Using the Teamleader API to Get Companies in PHP
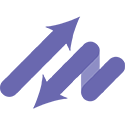
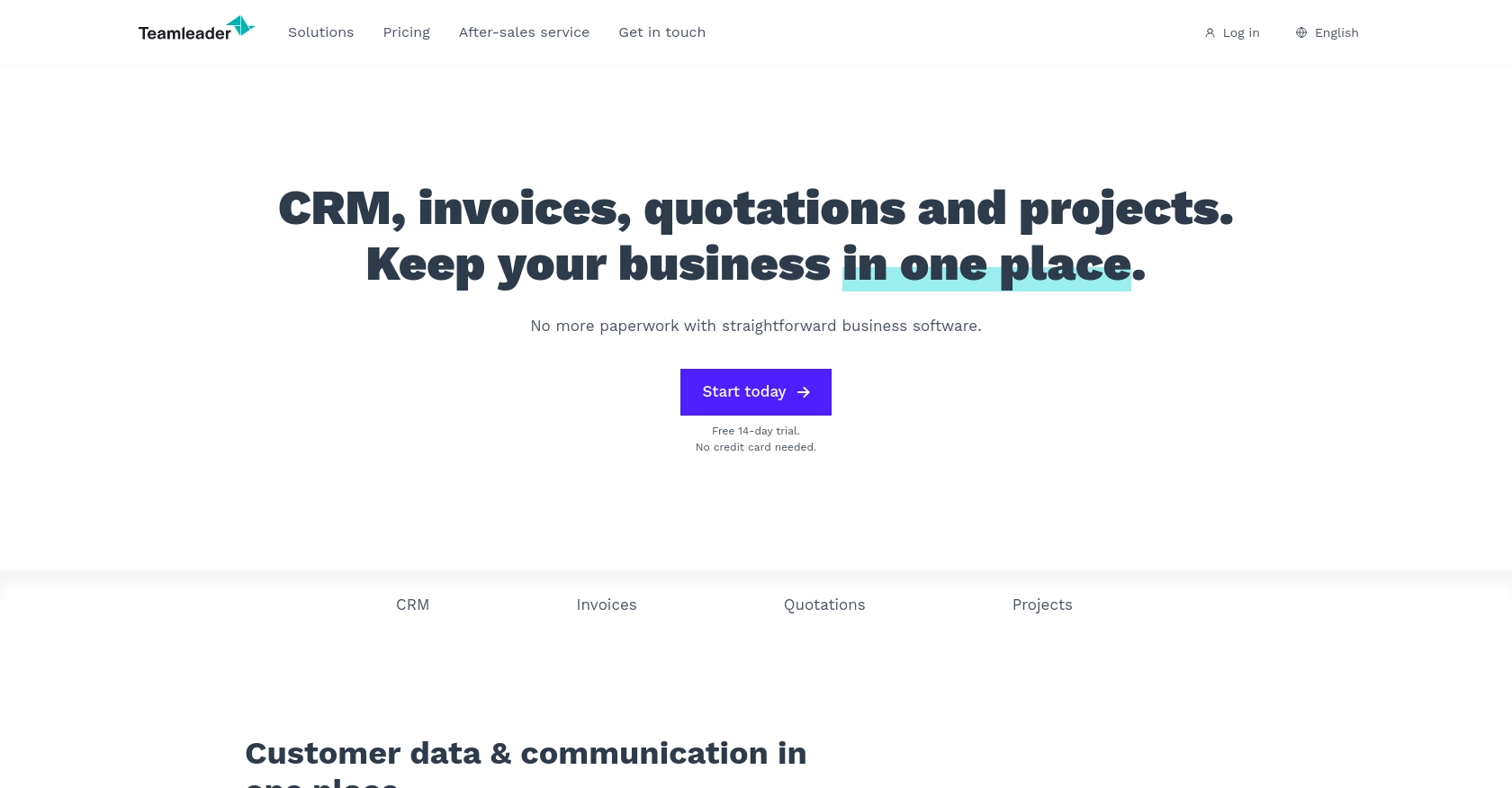
Introduction to Teamleader API
Teamleader is a versatile CRM and project management platform designed to help businesses streamline their operations. It offers a comprehensive suite of tools for managing contacts, projects, and invoices, making it a popular choice for companies looking to enhance productivity and organization.
Integrating with the Teamleader API allows developers to access and manage company data efficiently. For example, you can use the API to retrieve a list of companies, enabling seamless integration of business data into your applications. This can be particularly useful for automating workflows and enhancing data-driven decision-making processes.
Setting Up Your Teamleader Test/Sandbox Account
Before you can start interacting with the Teamleader API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Teamleader Account
If you don't already have a Teamleader account, you can sign up for a free trial on the Teamleader website. Follow the instructions to create your account. If you already have an account, simply log in.
Setting Up OAuth Authentication for Teamleader API
The Teamleader API uses OAuth 2.0 for authentication, which provides a secure way to authorize API requests. Follow these steps to set up OAuth authentication:
- Log in to your Teamleader account and navigate to the developer section.
- Create a new application by providing the necessary details such as the application name and redirect URI.
- Once the application is created, you will receive a client ID and client secret. Keep these credentials safe as you will need them to authenticate API requests.
- Set the required scopes for your application to access company data. Ensure you have the necessary permissions to read company information.
Generating Access Tokens
With your client ID and client secret, you can now generate access tokens to authenticate your API requests:
- Direct users to the Teamleader authorization URL, where they can log in and authorize your application.
- Once authorized, you will receive an authorization code. Use this code to request an access token from the Teamleader token endpoint.
- Store the access token securely, as it will be used to authenticate your API calls.
For more detailed information on OAuth setup, refer to the Teamleader API Documentation.
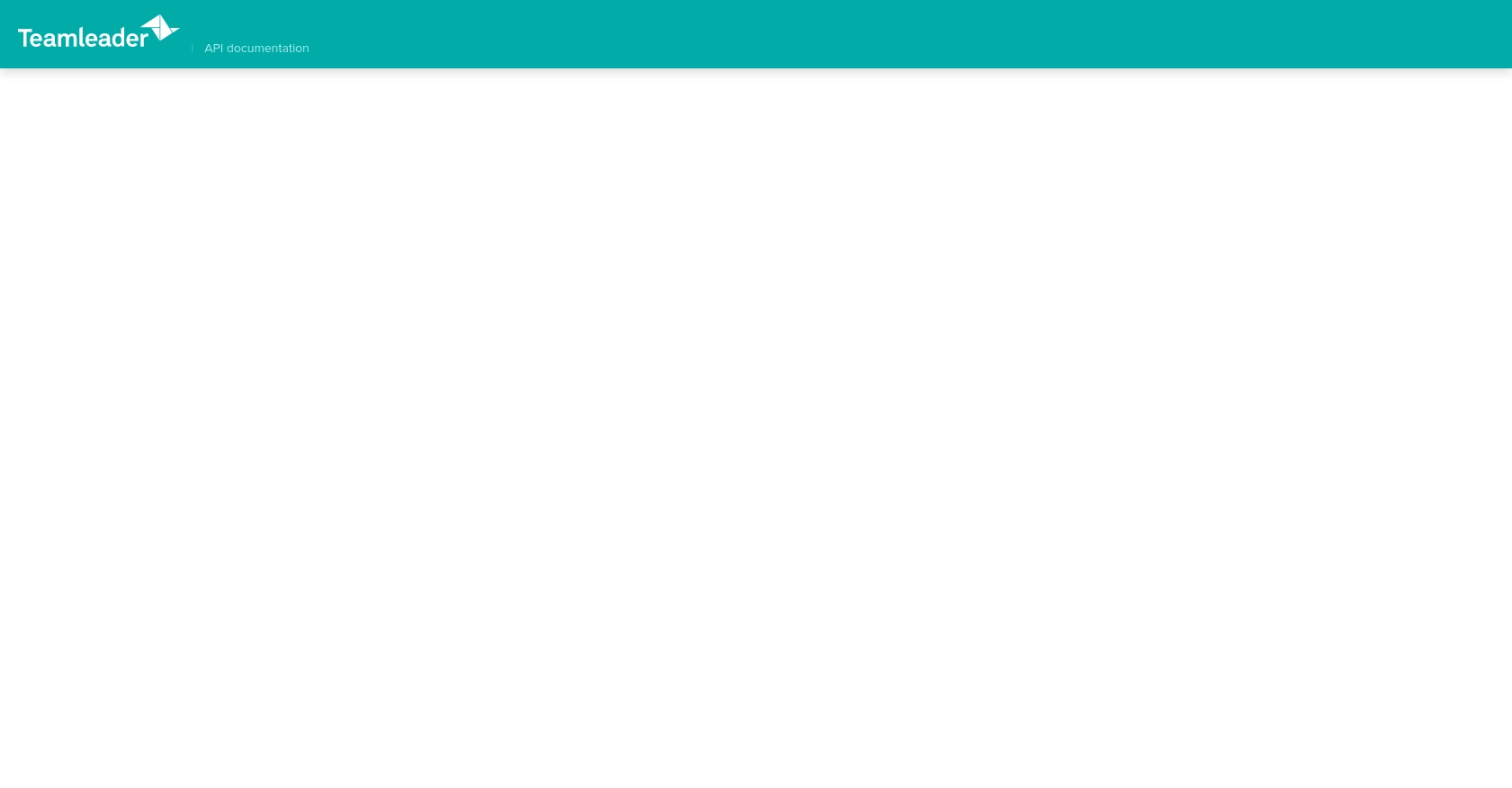
sbb-itb-96038d7
Making API Calls to Retrieve Companies Using Teamleader API in PHP
To interact with the Teamleader API and retrieve a list of companies, you'll need to set up your PHP environment and make the appropriate API calls. This section will guide you through the process, ensuring you have everything you need to successfully access company data.
Setting Up Your PHP Environment for Teamleader API Integration
Before making API calls, ensure your development environment is ready. You'll need PHP installed on your machine, along with the Composer package manager to handle dependencies.
- Ensure you have PHP 7.4 or higher installed. You can download it from the PHP official website.
- Install Composer by following the instructions on the Composer website.
Installing Required PHP Dependencies
To make HTTP requests, you'll need to install the Guzzle HTTP client, a popular PHP library for handling HTTP requests.
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Companies from Teamleader API
With your environment set up, you can now write the PHP code to make the API call. Create a new PHP file named get_companies.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
try {
$response = $client->request('GET', 'https://api.teamleader.eu/companies.list', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
]
]);
$companies = json_decode($response->getBody(), true);
foreach ($companies['data'] as $company) {
echo 'Company Name: ' . $company['name'] . "<br>";
}
} catch (\Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token you obtained during the OAuth setup. This code uses Guzzle to send a GET request to the Teamleader API endpoint for retrieving companies. It then parses the JSON response and prints the names of the companies.
Running Your PHP Script and Verifying Results
Run the script from your terminal or command line using the following command:
php get_companies.php
You should see a list of company names printed as output. This confirms that your API call was successful and that you have retrieved the company data from Teamleader.
Handling Errors and Troubleshooting API Requests
When making API calls, it's important to handle potential errors. The Teamleader API may return various error codes, such as 401 for unauthorized access or 404 if the endpoint is incorrect. Ensure you catch exceptions and handle them appropriately in your code.
For more information on error codes and handling, refer to the Teamleader API Documentation.
Conclusion and Best Practices for Teamleader API Integration
Integrating with the Teamleader API using PHP allows developers to efficiently manage and access company data, facilitating seamless automation and data-driven decision-making. By following the steps outlined in this guide, you can set up OAuth authentication, make API calls, and handle responses effectively.
Best Practices for Secure and Efficient API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: When integrating data from Teamleader into your applications, ensure that data fields are standardized and transformed as needed to maintain consistency.
- Error Handling: Implement robust error handling to manage different HTTP status codes and exceptions. This will help in diagnosing issues and maintaining a smooth integration experience.
Enhance Your Integration Strategy with Endgrate
While integrating with Teamleader API directly is a powerful approach, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API endpoint that simplifies integration processes across various platforms, including Teamleader.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
Ready to get started?