Using the Sugar Sell API to Create Records in Javascript
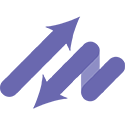
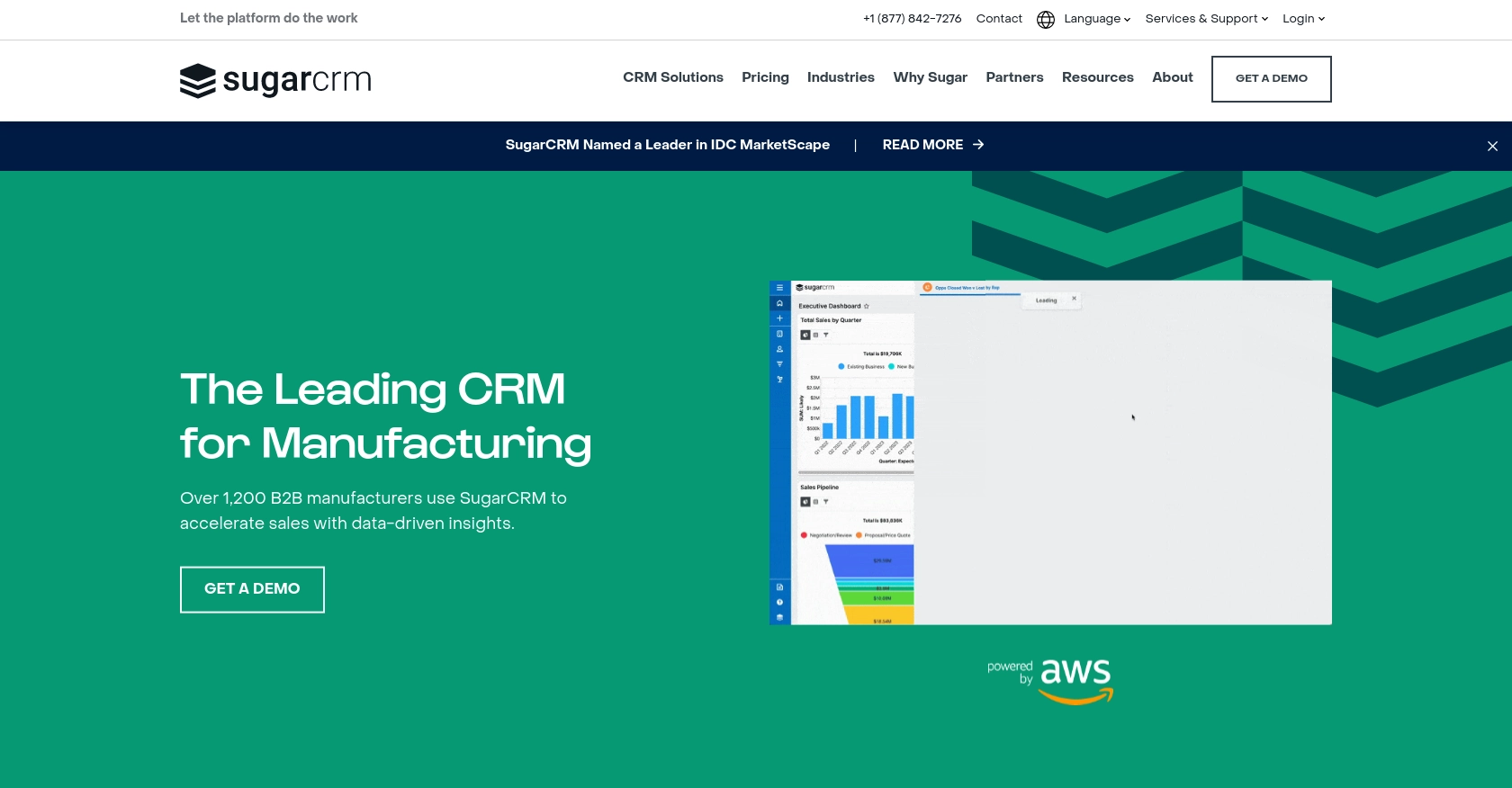
Introduction to Sugar Sell API
Sugar Sell is a robust customer relationship management (CRM) platform designed to enhance sales processes and customer interactions. It offers a comprehensive suite of tools that enable businesses to manage leads, opportunities, and customer data effectively.
Integrating with the Sugar Sell API allows developers to automate and streamline CRM tasks, such as creating and managing records. For example, a developer might use the Sugar Sell API to automatically create new customer records from an external data source, ensuring that the sales team has up-to-date information at their fingertips.
Setting Up a Sugar Sell Test or Sandbox Account
Before you can start integrating with the Sugar Sell API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a Sugar Sell Sandbox Account
To begin, visit the SugarCRM website and sign up for a free trial or request access to a sandbox account. This will provide you with a dedicated environment to test your API integrations.
- Go to the SugarCRM website.
- Navigate to the trial or sandbox section.
- Fill out the necessary information to create your account.
- Once your account is set up, log in to access your dashboard.
Configuring OAuth2 Authentication for Sugar Sell API
Sugar Sell uses OAuth2 for authentication. Follow these steps to configure your OAuth2 credentials:
- Log in to your Sugar Sell account.
- Navigate to the Administration section and select "OAuth Keys."
- Create a new OAuth Key with the following details:
- Client ID: Use "sugar" for standard authentication.
- Client Secret: Leave this blank unless specified otherwise.
- Platform: Set to "custom" to avoid login conflicts.
- Save your OAuth Key configuration.
Generating Access Tokens for API Requests
With your OAuth2 credentials ready, you can now generate access tokens to authenticate API requests:
// Example of generating an access token using JavaScript
const axios = require('axios');
const getToken = async () => {
try {
const response = await axios.post('https:///rest//oauth2/token', {
grant_type: 'password',
client_id: 'sugar',
client_secret: '',
username: '',
password: '',
platform: 'custom'
});
console.log('Access Token:', response.data.access_token);
} catch (error) {
console.error('Error fetching token:', error);
}
};
getToken();
Replace <site_url>
, <version>
, <your_username>
, and <your_password>
with your actual Sugar Sell account details.
Once you have your access token, you can proceed to make API calls to create and manage records within your Sugar Sell sandbox environment.
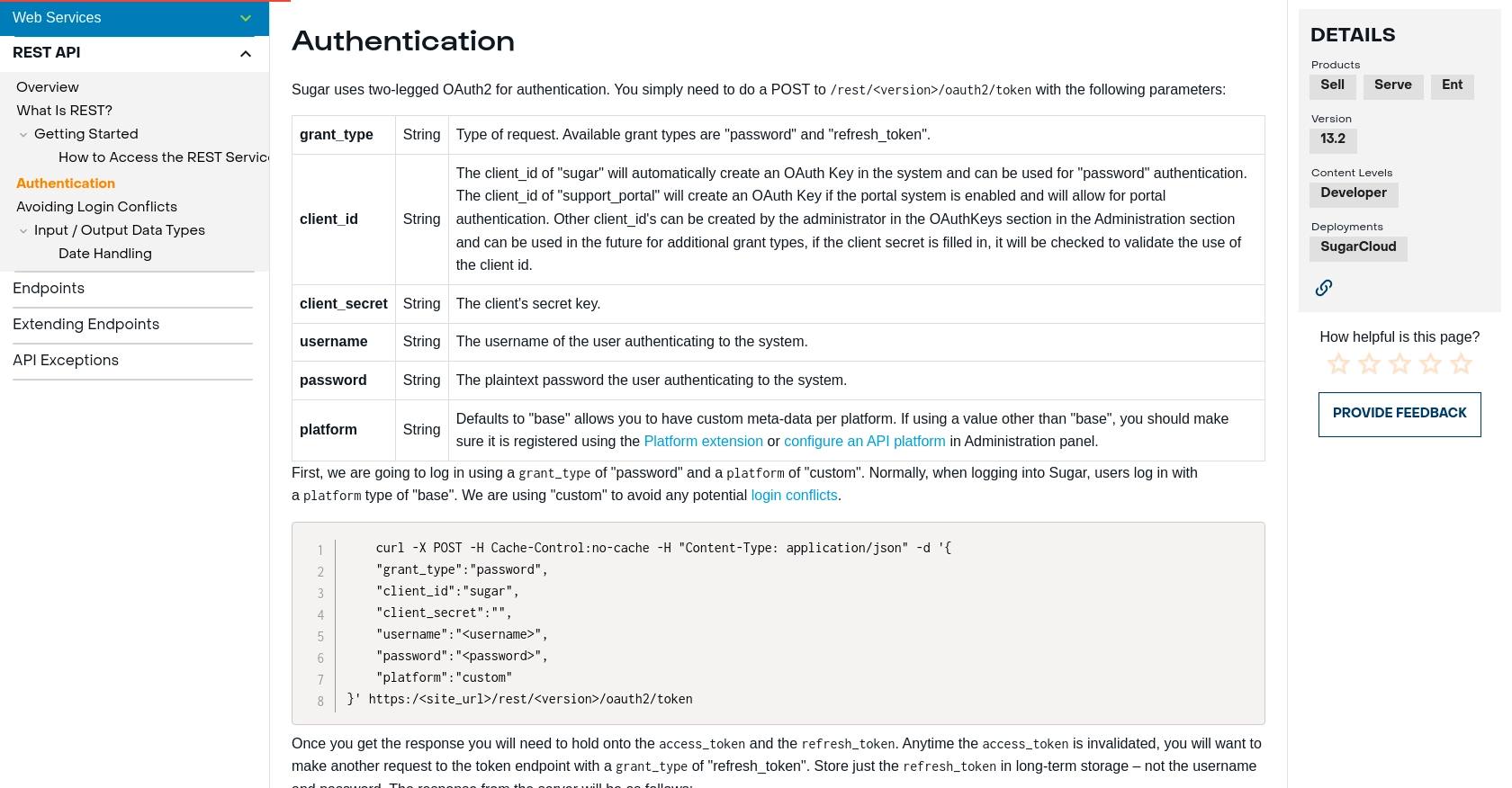
sbb-itb-96038d7
Making API Calls to Create Records in Sugar Sell Using JavaScript
With your access token in hand, you can now proceed to make API calls to create records in Sugar Sell. This section will guide you through the process of setting up your JavaScript environment and executing API requests to interact with Sugar Sell's CRM functionalities.
Setting Up Your JavaScript Environment for Sugar Sell API
Before making API calls, ensure you have Node.js installed on your machine. You will also need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Creating a Record in Sugar Sell Using JavaScript
To create a record in Sugar Sell, you'll need to make a POST request to the appropriate endpoint. Below is an example of how to create a new contact record using JavaScript:
const axios = require('axios');
const createRecord = async () => {
const accessToken = '';
const siteUrl = '';
const version = '';
const data = {
data: {
type: 'Contacts',
attributes: {
first_name: 'John',
last_name: 'Doe',
email: 'john.doe@example.com'
}
}
};
try {
const response = await axios.post(`https://${siteUrl}/rest/${version}/Contacts`, data, {
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${accessToken}`
}
});
console.log('Record Created:', response.data);
} catch (error) {
console.error('Error creating record:', error.response ? error.response.data : error.message);
}
};
createRecord();
Replace <your_access_token>
, <site_url>
, and <version>
with your actual access token, site URL, and API version. The data
object contains the details of the record you wish to create.
Verifying Record Creation in Sugar Sell
After executing the code, you can verify the creation of the new record by logging into your Sugar Sell sandbox account and navigating to the Contacts module. The newly created contact should appear in the list.
Handling Errors and Troubleshooting API Requests
When making API calls, it's crucial to handle potential errors gracefully. Common error codes include:
- 400 Bad Request: The request was invalid. Check your request syntax and data.
- 401 Unauthorized: The access token is missing or invalid. Ensure your token is correct and hasn't expired.
- 500 Internal Server Error: An error occurred on the server. Try again later or contact support.
For more detailed error information, refer to the SugarCRM API documentation.
Best Practices for Using Sugar Sell API in JavaScript
When integrating with the Sugar Sell API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth2 credentials and access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized and validated before making API requests. This helps in maintaining data integrity and consistency.
- Use Error Handling: Implement robust error handling to manage API errors effectively. Log errors for troubleshooting and provide user-friendly messages where applicable.
Leveraging Endgrate for Seamless Sugar Sell Integrations
Integrating with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Sugar Sell. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
- https://endgrate.com/provider/sugarsell
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/#Authentication
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/endpoints/
Ready to get started?