Using the Salesloft API to Create or Update People in PHP
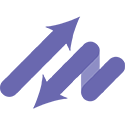
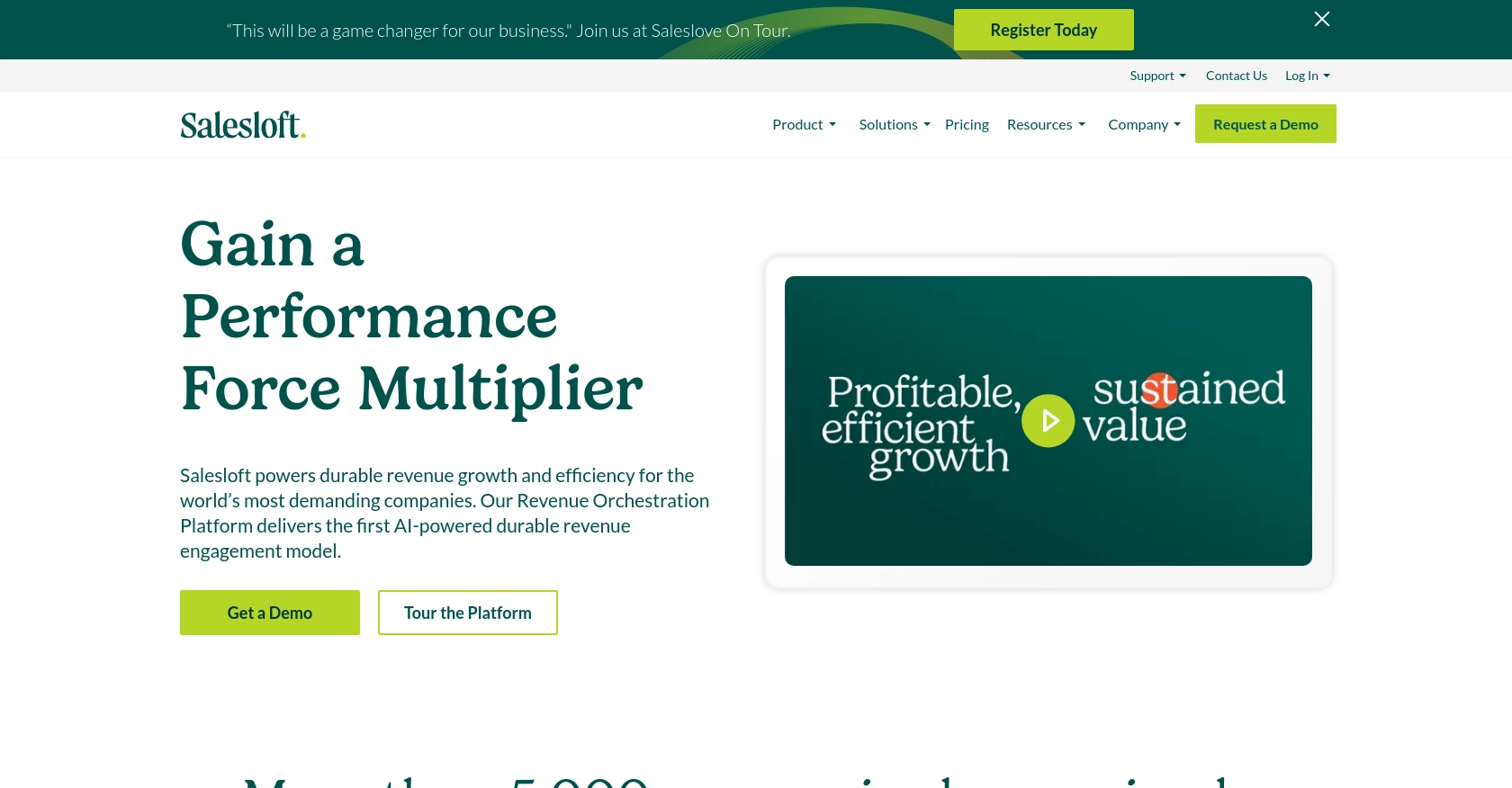
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform that enables sales teams to connect with prospects more effectively. It offers a suite of tools designed to enhance sales productivity, streamline workflows, and improve communication with potential clients.
Integrating with the Salesloft API allows developers to automate and enhance sales processes by programmatically managing data within the platform. For example, developers can create or update people records in Salesloft using PHP, enabling seamless synchronization of contact information from external systems.
This article will guide you through the process of using PHP to interact with the Salesloft API, focusing on creating or updating people records. By following this tutorial, you can efficiently manage your sales data and improve your team's engagement strategies.
Setting Up a Salesloft Test Account for API Integration
Before you can start integrating with the Salesloft API using PHP, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Salesloft provides a straightforward process to create an OAuth application, which is essential for authenticating your API requests.
Creating a Salesloft OAuth Application
- Log in to your Salesloft account. If you don't have one, you can sign up for a free trial on the Salesloft website.
- Navigate to Your Applications under the account settings.
- Select OAuth Applications and click on Create New.
- Fill out the required fields, including the application name and redirect URI. Once completed, click Save.
- After saving, you'll receive your Client ID and Client Secret. Make sure to store these securely as they are necessary for the authorization process.
Obtaining Authorization Code and Access Tokens
Salesloft uses OAuth 2.0 for authentication, which involves obtaining an authorization code and exchanging it for access tokens. Follow these steps to complete the process:
- Direct your users to the following authorization endpoint, replacing
YOUR_CLIENT_ID
andYOUR_REDIRECT_URI
with your app's details: - Upon user approval, the redirect URI will receive a
code
parameter. Use this code to request access and refresh tokens by making a POST request to the token endpoint: - The response will include an
access_token
and arefresh_token
. Store these tokens securely for future API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
With your OAuth application set up and tokens obtained, you're ready to start making authenticated requests to the Salesloft API. This setup ensures that your integration is secure and compliant with Salesloft's authentication standards.
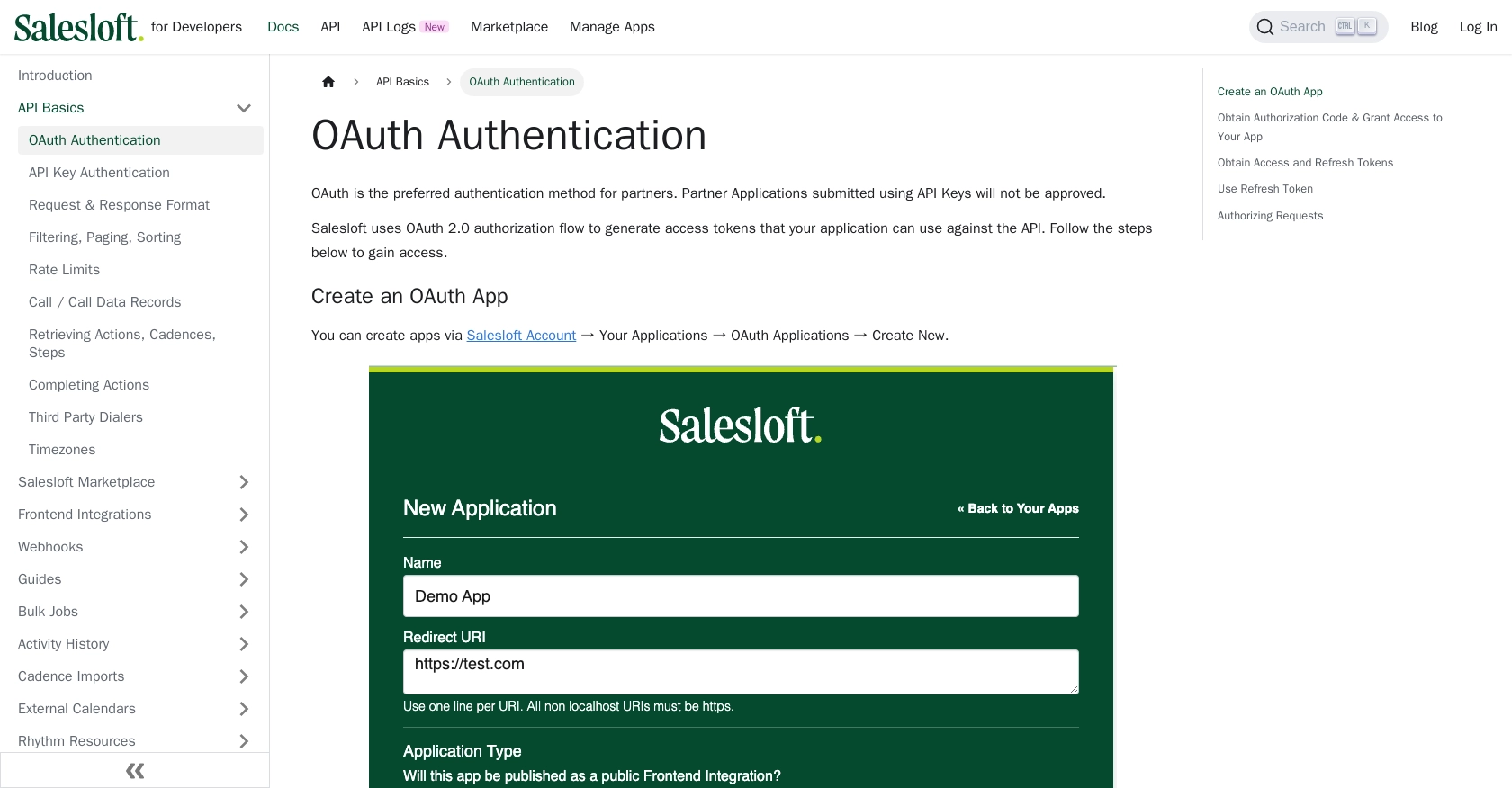
sbb-itb-96038d7
Making API Calls to Salesloft Using PHP
With your OAuth setup complete, you can now proceed to make API calls to Salesloft using PHP. This section will guide you through the process of creating or updating people records in Salesloft, leveraging the PHP programming language.
Prerequisites for PHP Integration with Salesloft API
Before diving into the code, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer for dependency management
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install Guzzle:
composer require guzzlehttp/guzzle
Creating or Updating People Records in Salesloft
To create or update people records in Salesloft, you'll need to make a POST request to the Salesloft API endpoint. Below is a sample PHP script demonstrating how to achieve this:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN'; // Replace with your access token
$response = $client->post('https://api.salesloft.com/v2/people', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
'Accept' => 'application/json'
],
'json' => [
'email_address' => 'example@example.com',
'first_name' => 'John',
'last_name' => 'Doe',
'phone' => '+1234567890'
]
]);
$data = json_decode($response->getBody(), true);
if ($response->getStatusCode() === 200) {
echo "Person created or updated successfully. ID: " . $data['data']['id'];
} else {
echo "Failed to create or update person.";
}
In this script, replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup. The script uses Guzzle to send a POST request to the Salesloft API, including the necessary headers and JSON payload.
Verifying API Call Success in Salesloft
After running the script, you can verify the success of the API call by checking the response status code. A status code of 200 indicates that the person record was successfully created or updated. You can also log in to your Salesloft account and check the people records to confirm the changes.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The Salesloft API may return various error codes, such as:
- 403 Forbidden: Indicates that the request is not authorized. Check your access token and permissions.
- 404 Not Found: The requested resource could not be found. Verify the endpoint URL.
- 422 Unprocessable Entity: The request was well-formed but could not be processed due to validation errors. Check the error messages for details.
Implement error handling in your code to manage these scenarios and provide meaningful feedback to users.
For more details on error handling, refer to the Salesloft API documentation.
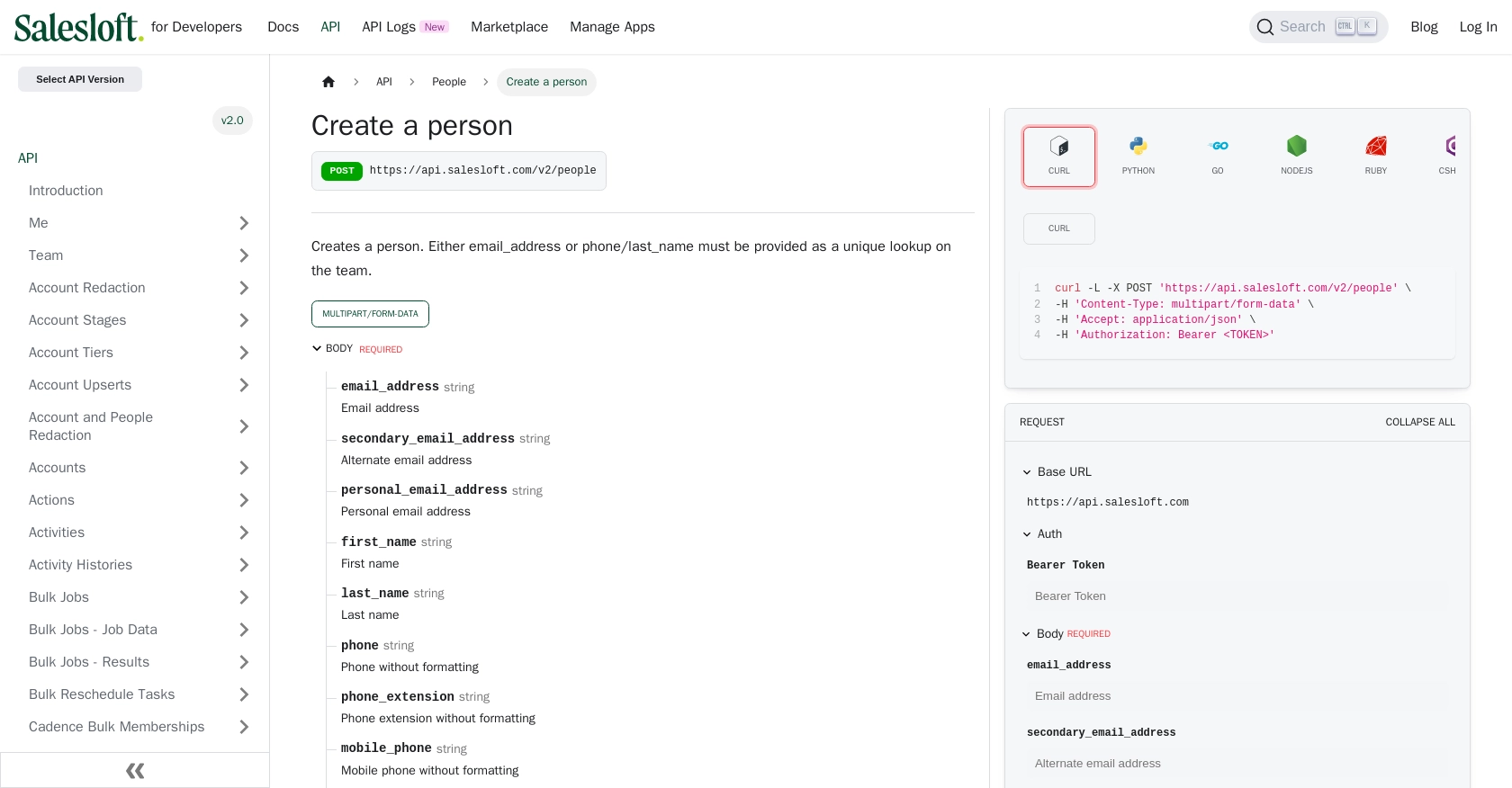
Conclusion and Best Practices for Salesloft API Integration with PHP
Integrating with the Salesloft API using PHP can significantly enhance your sales processes by automating the management of people records. By following the steps outlined in this guide, you can efficiently create or update contacts, ensuring your sales team has access to the most current data.
Best Practices for Secure and Efficient API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limits: Be mindful of Salesloft's rate limits, which are set at 600 cost per minute. Implement logic to handle rate limit responses and retry requests as needed. For more details, refer to the Salesloft API rate limits documentation.
- Implement Error Handling: Ensure your application gracefully handles errors by checking response codes and providing meaningful feedback. This includes managing common errors like 403, 404, and 422.
- Optimize Data Management: Standardize and transform data fields to align with your internal systems, ensuring consistency and accuracy across platforms.
Enhance Your Integration Strategy with Endgrate
While building integrations with the Salesloft API is a powerful way to enhance your sales processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Salesloft.
With Endgrate, you can focus on your core product while outsourcing integration management. This approach allows you to build once for each use case, providing an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/people-create/
Ready to get started?