Using the Cloze API to Get Users in Javascript
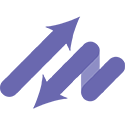
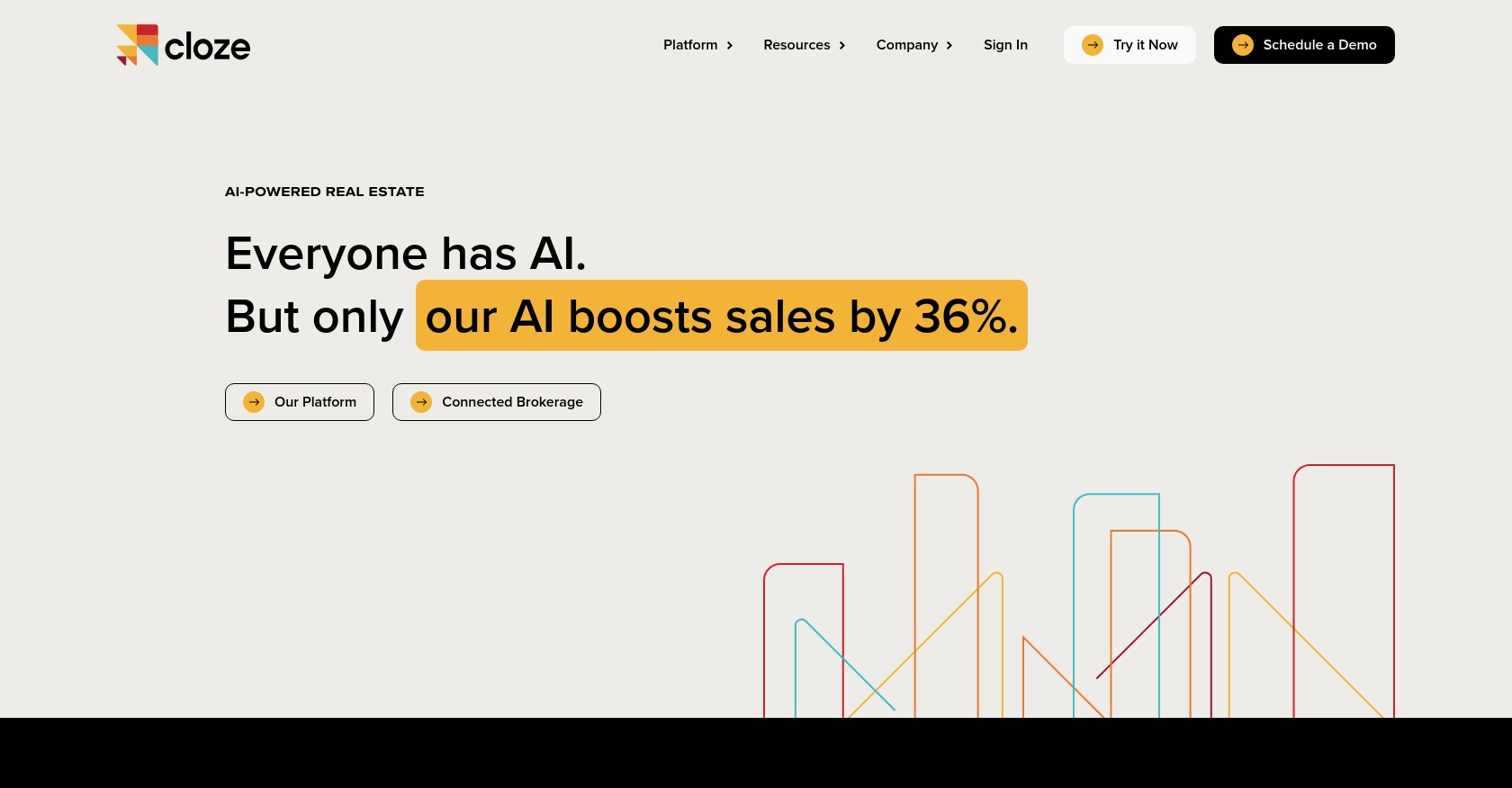
Introduction to Cloze API
Cloze is a powerful relationship management platform designed to help businesses manage their interactions with clients, partners, and team members. It offers a comprehensive suite of tools for managing contacts, projects, and communications, making it an essential tool for businesses looking to streamline their relationship management processes.
Integrating with the Cloze API allows developers to access and manage user data programmatically, enabling automation and enhanced functionality within their applications. For example, developers can use the Cloze API to retrieve a list of team members, facilitating seamless integration with other business tools and improving team collaboration.
This article will guide you through the process of using JavaScript to interact with the Cloze API, specifically focusing on retrieving user information. By following this tutorial, you'll learn how to set up your environment, authenticate with the API, and execute API calls to access user data efficiently.
Setting Up Your Cloze API Test Account
Before you can start interacting with the Cloze API using JavaScript, you'll need to set up a test account. This involves creating a Cloze account and obtaining the necessary API credentials to authenticate your requests.
Creating a Cloze Account
If you don't already have a Cloze account, you can sign up for a free trial on the Cloze website. Follow these steps to get started:
- Visit the Cloze website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email address, and password.
- Follow the on-screen instructions to complete the registration process.
Once your account is created, you'll be able to log in to the Cloze dashboard.
Generating an API Key for Cloze
To authenticate your API requests, you'll need to generate an API key. Here's how you can do it:
- Log in to your Cloze account and navigate to the settings page.
- Locate the "API Keys" section under the integrations tab.
- Click on "Create API Key" and provide a name for your key to help you identify it later.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
Understanding Cloze API Authentication
The Cloze API supports both API key and OAuth2 authentication methods. For initial development and testing, using an API key is recommended. You'll need to include your Cloze account email and the API key in your requests. Here's a brief overview:
- API Key Authentication: Include your Cloze account email as the
user
parameter and the API key as theapi_key
parameter in your requests. - OAuth2 Authentication: If you're building a public integration, you'll need to use OAuth2. Contact Cloze support to get started with OAuth2.
For more details on authentication, refer to the Cloze API documentation.
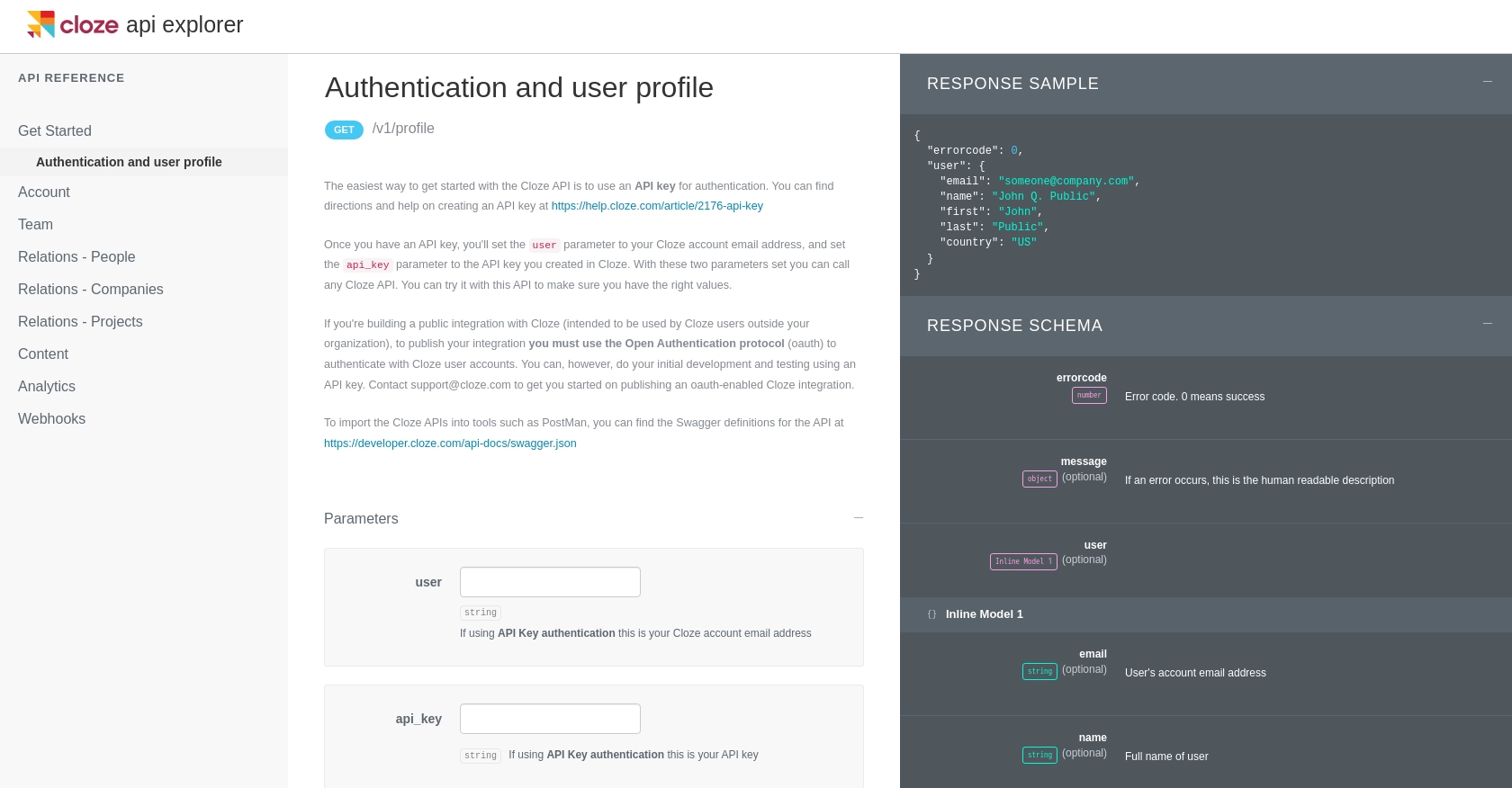
sbb-itb-96038d7
Making API Calls to Retrieve Cloze Users with JavaScript
To interact with the Cloze API using JavaScript, you'll need to set up your environment and execute API calls to retrieve user information. This section will guide you through the necessary steps, including setting up your JavaScript environment, writing the code to make API requests, and handling responses effectively.
Setting Up Your JavaScript Environment for Cloze API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a browser. Follow these steps to set up your environment:
- Download and install Node.js from the official website.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Call the Cloze API
With your environment set up, you can now write the JavaScript code to interact with the Cloze API. The following example demonstrates how to retrieve a list of team members using the Cloze API:
const axios = require('axios');
// Define the API endpoint and your credentials
const endpoint = 'https://api.cloze.com/v1/team/members';
const userEmail = 'your-email@domain.com';
const apiKey = 'your-api-key';
// Function to get Cloze team members
async function getClozeTeamMembers() {
try {
const response = await axios.get(endpoint, {
params: {
user: userEmail,
api_key: apiKey
}
});
// Check if the request was successful
if (response.data.errorcode === 0) {
console.log('Team Members:', response.data.list);
} else {
console.error('Error:', response.data.message);
}
} catch (error) {
console.error('Request failed:', error.message);
}
}
// Execute the function
getClozeTeamMembers();
Replace your-email@domain.com
and your-api-key
with your actual Cloze account email and API key. This code uses the axios
library to send a GET request to the Cloze API endpoint for team members. It checks for a successful response and logs the list of team members to the console.
Verifying API Call Success and Handling Errors
After executing the API call, verify the success by checking the response data. The Cloze API returns an errorcode
of 0 for successful requests. If an error occurs, the response will include a human-readable message
describing the issue.
To handle errors effectively, ensure your code includes error handling logic, such as try-catch blocks, to manage exceptions and provide meaningful feedback to users.
For more information on error codes and handling, refer to the Cloze API documentation.
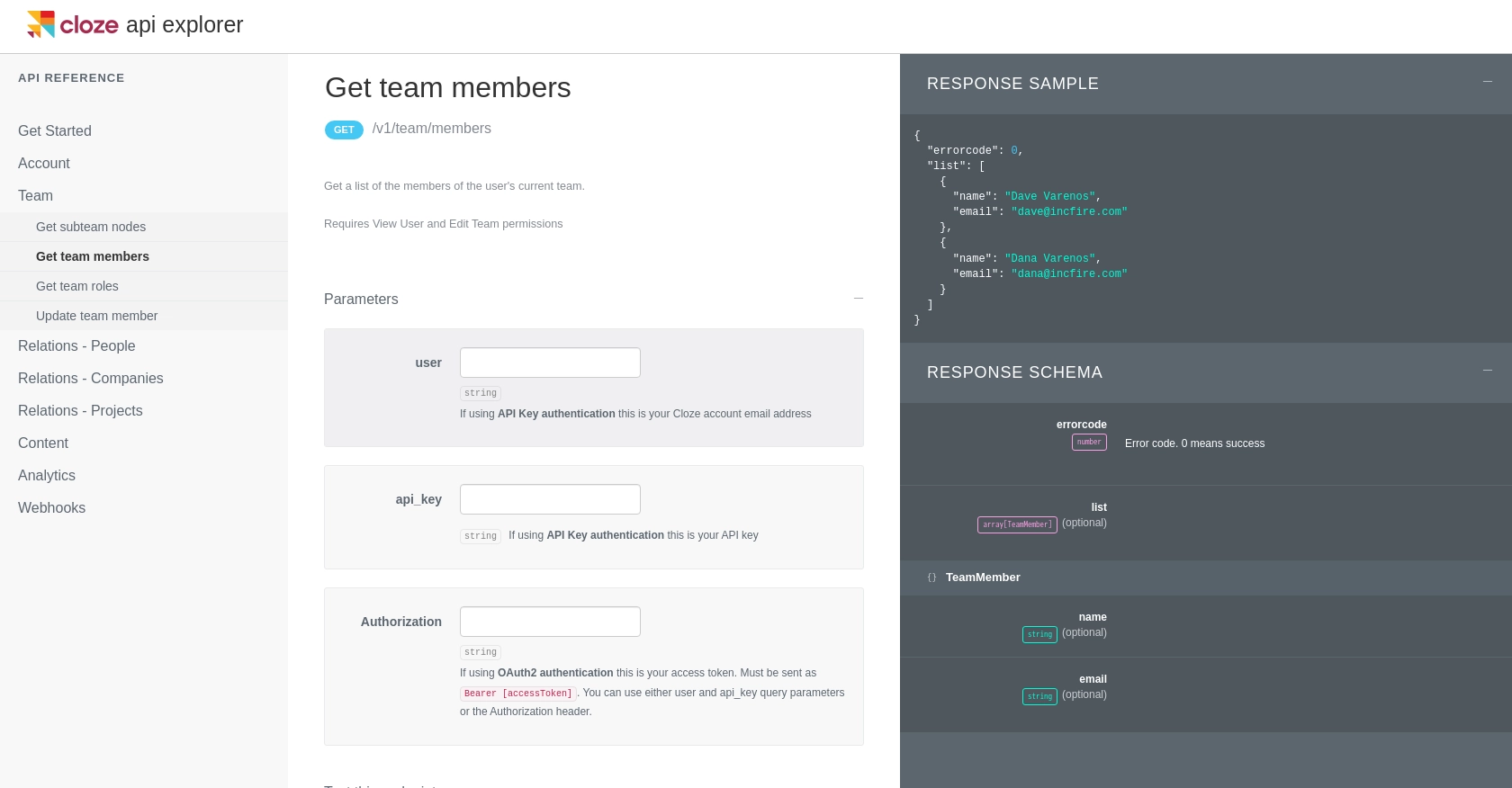
Conclusion and Best Practices for Using Cloze API with JavaScript
Integrating with the Cloze API using JavaScript provides a powerful way to enhance your application's capabilities by accessing and managing user data programmatically. By following the steps outlined in this article, you can efficiently set up your environment, authenticate with the API, and retrieve user information.
Best Practices for Secure and Efficient Cloze API Integration
- Secure API Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API call failures and provide meaningful feedback to users.
By adhering to these best practices, you can ensure a secure and efficient integration with the Cloze API, enhancing your application's functionality and improving user experience.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including Cloze, allowing you to build once and integrate seamlessly across different services. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration processes and focus on your core product development.
Read More
Ready to get started?