Using the Insightly API to Get Contacts in PHP
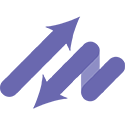
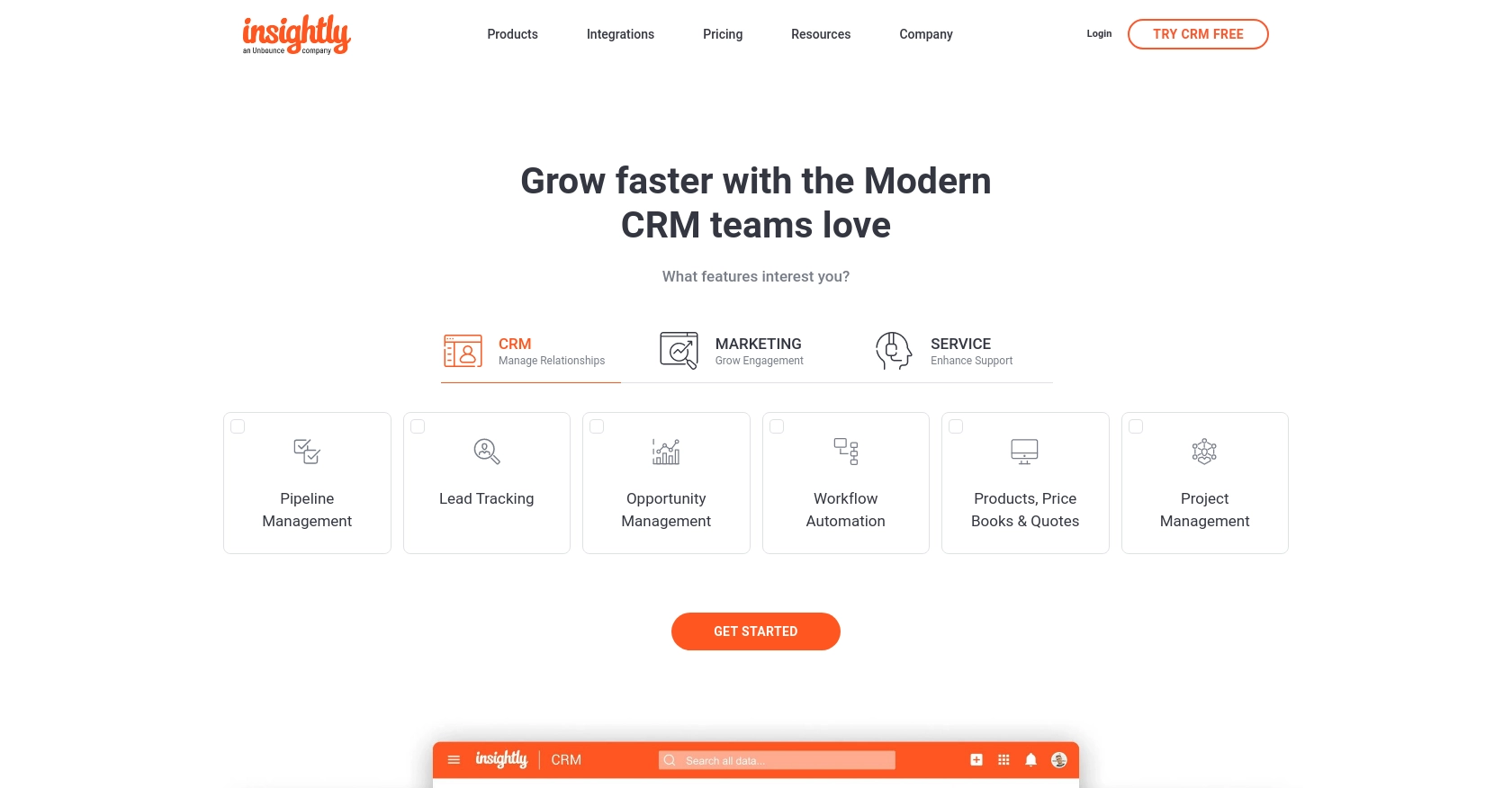
Introduction to Insightly CRM
Insightly is a powerful CRM platform designed to help businesses manage their customer relationships and streamline their sales processes. With features like project management, contact management, and workflow automation, Insightly is a popular choice for businesses looking to enhance their customer interactions.
Integrating with the Insightly API allows developers to access and manipulate CRM data programmatically, enabling automation and customization of CRM workflows. For example, a developer might use the Insightly API to retrieve contact information and synchronize it with another system, ensuring that all customer data is up-to-date across platforms.
This article will guide you through using PHP to interact with the Insightly API, specifically focusing on retrieving contact information. By the end of this tutorial, you'll be able to efficiently access and manage contacts within the Insightly platform using PHP.
Setting Up Your Insightly Test Account
Before you can start interacting with the Insightly API using PHP, you need to set up a test account. Insightly provides a straightforward way to access their API through an API key, which allows you to authenticate your requests and access your CRM data.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on the Insightly website. Follow the instructions to create your account and log in to the Insightly dashboard.
Locating Your Insightly API Key
To authenticate your API requests, you'll need your Insightly API key. Follow these steps to find it:
- Log in to your Insightly account.
- Click on your user profile icon in the upper right corner and select User Settings.
- Navigate to the API Key and URL section.
- Copy your API key from this section. You will use this key to authenticate your API requests.
For more detailed instructions, you can refer to the Insightly support page.
Understanding Insightly API Authentication
Insightly uses HTTP Basic authentication. When making API calls, include your API key as the Base64-encoded username, leaving the password blank. This ensures that your requests are authenticated and authorized to access your CRM data.
For testing purposes, you can paste your API key directly into the API key field without Base64 encoding when using the sandbox environment.
Rate Limiting Considerations
Insightly imposes rate limits on API requests to prevent abuse. The free plan allows up to 1,000 requests per day per instance, with a maximum of 10 requests per second. Be mindful of these limits when developing and testing your integration.
If you exceed the rate limits, you will receive an HTTP Status Code 429 response. Plan your API usage accordingly to avoid disruptions.
For more information on rate limits, visit the Insightly API documentation.
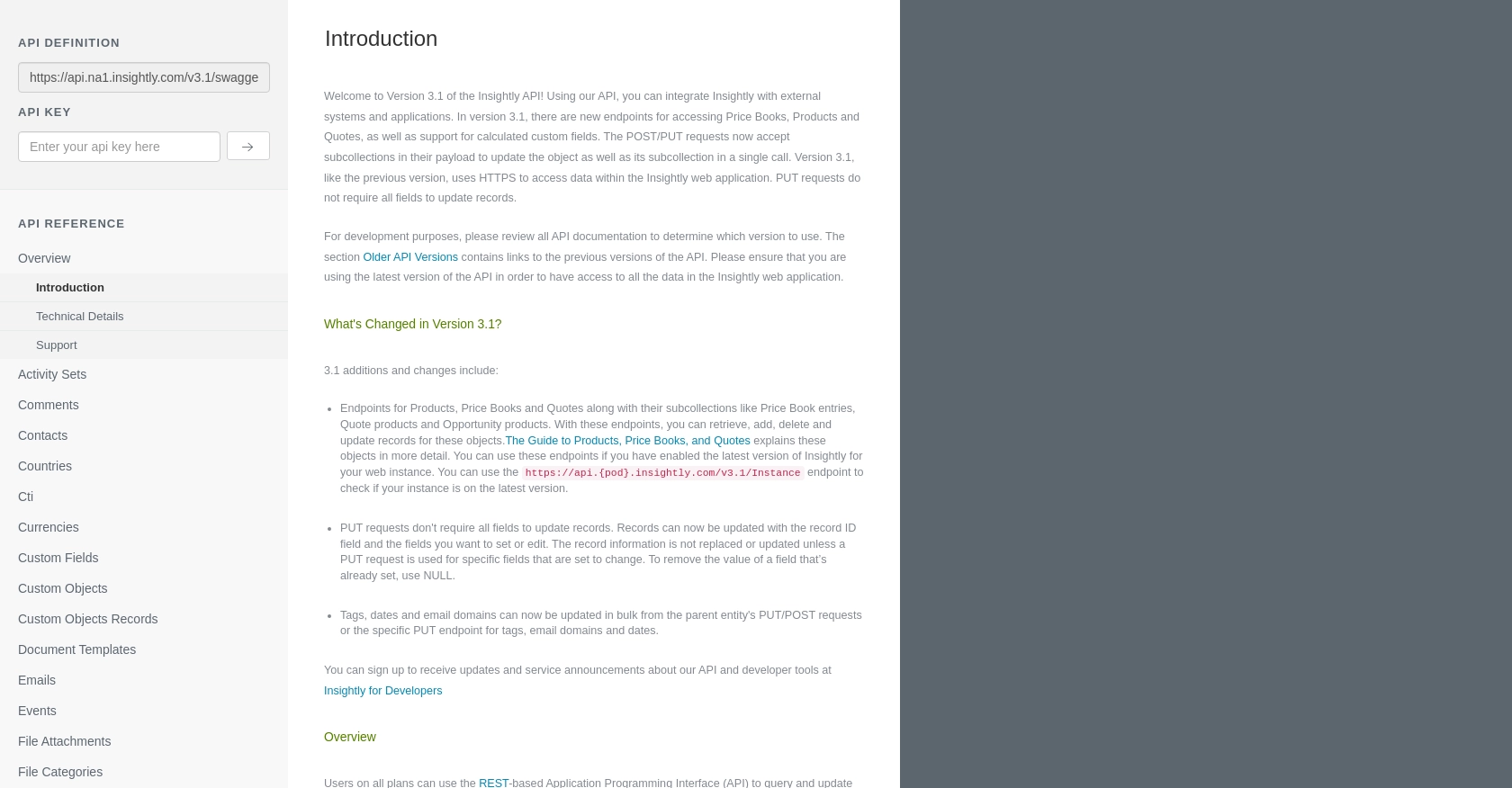
sbb-itb-96038d7
Making API Calls to Retrieve Insightly Contacts Using PHP
To interact with the Insightly API using PHP, you'll need to set up your development environment and write code to make HTTP requests. This section will guide you through the process of retrieving contact information from Insightly using PHP.
Setting Up Your PHP Environment
Before you begin, ensure you have the following installed on your machine:
- PHP 7.4 or higher
- Composer (for managing dependencies)
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install Guzzle:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Insightly Contacts
Create a new PHP file named get_insightly_contacts.php
and add the following code to it:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key'; // Replace with your Insightly API key
$base64ApiKey = base64_encode($apiKey . ':');
$response = $client->request('GET', 'https://api.na1.insightly.com/v3.1/Contacts', [
'headers' => [
'Authorization' => 'Basic ' . $base64ApiKey,
'Accept-Encoding' => 'gzip'
]
]);
if ($response->getStatusCode() === 200) {
$contacts = json_decode($response->getBody(), true);
foreach ($contacts as $contact) {
echo 'Contact Name: ' . $contact['FIRST_NAME'] . ' ' . $contact['LAST_NAME'] . "\n";
}
} else {
echo 'Failed to retrieve contacts. Status code: ' . $response->getStatusCode();
}
In this code, you start by importing the necessary Guzzle classes and setting up the HTTP client. You then encode your API key using Base64 and make a GET request to the Insightly Contacts endpoint. If the request is successful, the code will loop through the contacts and print their names.
Running the PHP Script and Verifying Results
To execute the script, run the following command in your terminal:
php get_insightly_contacts.php
If successful, you should see a list of contact names printed in the terminal. You can verify the retrieved data by cross-referencing it with the contacts in your Insightly account.
Handling Errors and Insightly API Error Codes
When making API calls, it's important to handle potential errors. The Insightly API may return various HTTP status codes to indicate issues:
- 401 Unauthorized: Check if your API key is correct and properly encoded.
- 429 Too Many Requests: You've exceeded the rate limit. Wait and try again later.
- 500 Internal Server Error: There might be an issue with the Insightly server. Try again later.
Implement error handling in your code to manage these scenarios gracefully and ensure a robust integration.
Best Practices for Using the Insightly API in PHP
When working with the Insightly API, it's crucial to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store API Credentials: Always store your API key securely. Avoid hardcoding it directly in your code. Consider using environment variables or a secure vault.
- Implement Rate Limiting: Be mindful of Insightly's rate limits. Implement logic to handle HTTP 429 responses and retry requests after a delay.
- Data Standardization: Ensure that data retrieved from Insightly is standardized before integrating it with other systems. This helps maintain consistency across platforms.
- Error Handling: Implement robust error handling to manage different HTTP status codes and potential network issues.
Leveraging Endgrate for Seamless API Integrations
While integrating with Insightly's API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Insightly.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of multiple integrations.
- Build Once, Deploy Everywhere: Develop a single integration that works across different platforms, reducing redundancy.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can simplify your integration processes by visiting Endgrate.
Read More
Ready to get started?