Using the Younium API to Get Orders (with Python examples)
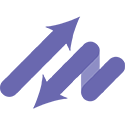
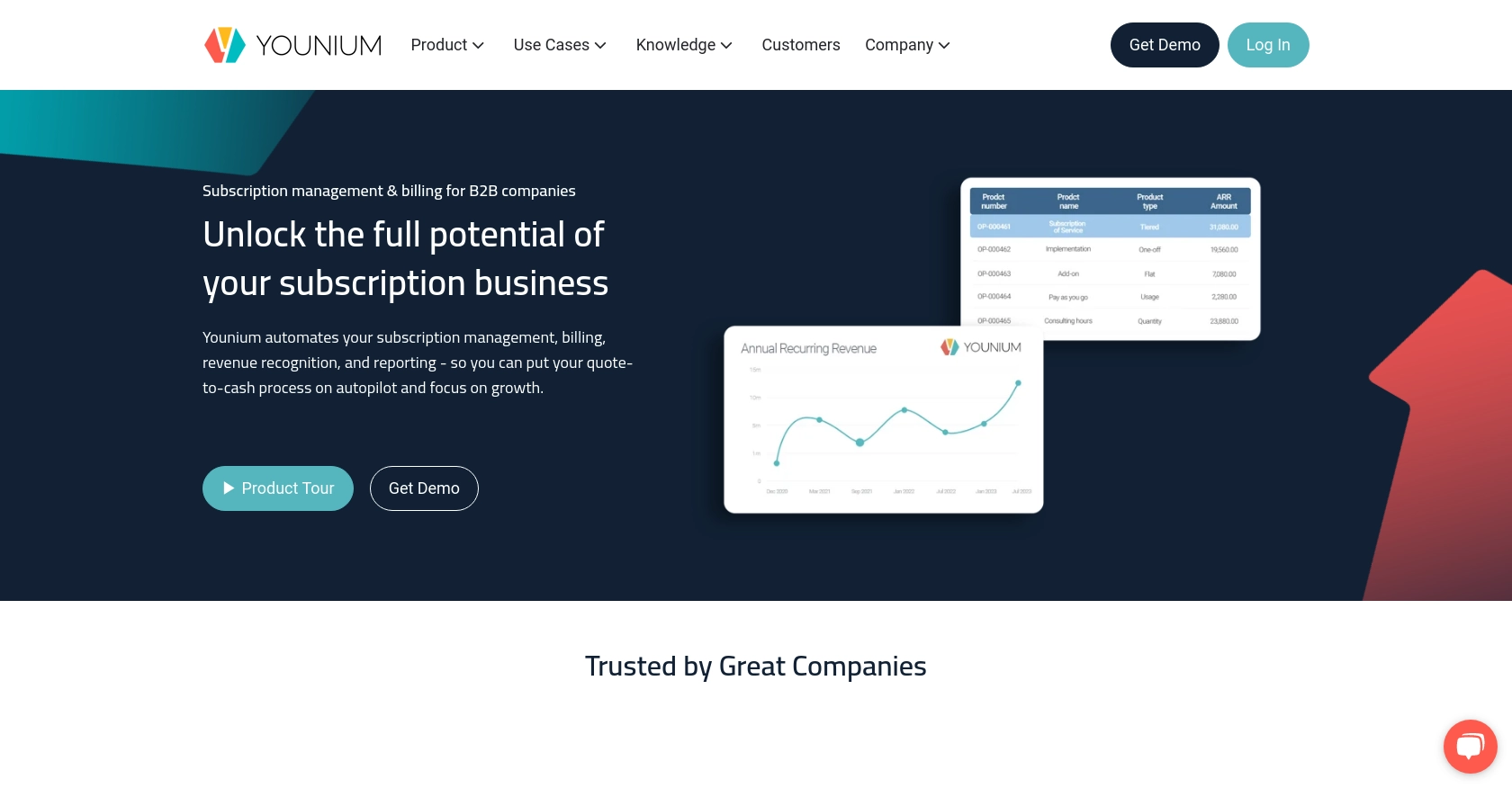
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform that empowers B2B SaaS companies to streamline their billing and revenue operations. With its robust features, Younium helps businesses manage subscriptions, automate billing processes, and gain valuable insights into their financial data.
Integrating with the Younium API allows developers to efficiently access and manage order data, enhancing the automation and accuracy of financial operations. For example, a developer might use the Younium API to retrieve sales orders and integrate them into a custom reporting tool, providing real-time insights into sales performance.
Setting Up Your Younium Test/Sandbox Account
Before you can start integrating with the Younium API, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting live data.
Creating a Younium Sandbox Account
To begin, you'll need to create a sandbox account on the Younium platform. This will give you access to a testing environment where you can make API calls and test your integration.
- Visit the Younium Developer Portal.
- Sign up for a sandbox account if you haven't already. Follow the on-screen instructions to complete the registration process.
- Once registered, log in to your sandbox account to access the dashboard.
Generating API Credentials for Younium
To authenticate your API requests, you'll need to generate API credentials. Follow these steps to obtain your Client ID and Secret Key:
- In your Younium dashboard, click on your user profile in the top right corner and select “Privacy & Security.”
- Navigate to “Personal Tokens” and click “Generate Token.”
- Provide a relevant description for your token and click “Create.”
- Copy the generated Client ID and Secret Key. These credentials will not be visible again, so store them securely.
Acquiring a JWT Access Token
With your API credentials ready, you can now generate a JWT access token, which is required for making authenticated API calls:
import requests
# Set the endpoint for token generation
url = "https://api.sandbox.younium.com/auth/token"
# Define the headers and body for the request
headers = {"Content-Type": "application/json"}
body = {
"clientId": "Your_Client_ID",
"secret": "Your_Secret_Key"
}
# Make the POST request to acquire the JWT token
response = requests.post(url, headers=headers, json=body)
# Parse the response to get the access token
token_data = response.json()
access_token = token_data.get("accessToken")
print("Access Token:", access_token)
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. This script will return a JWT access token, valid for 24 hours, which you will use to authenticate your API requests.
For more details on authentication, refer to the Younium Authentication Guide.
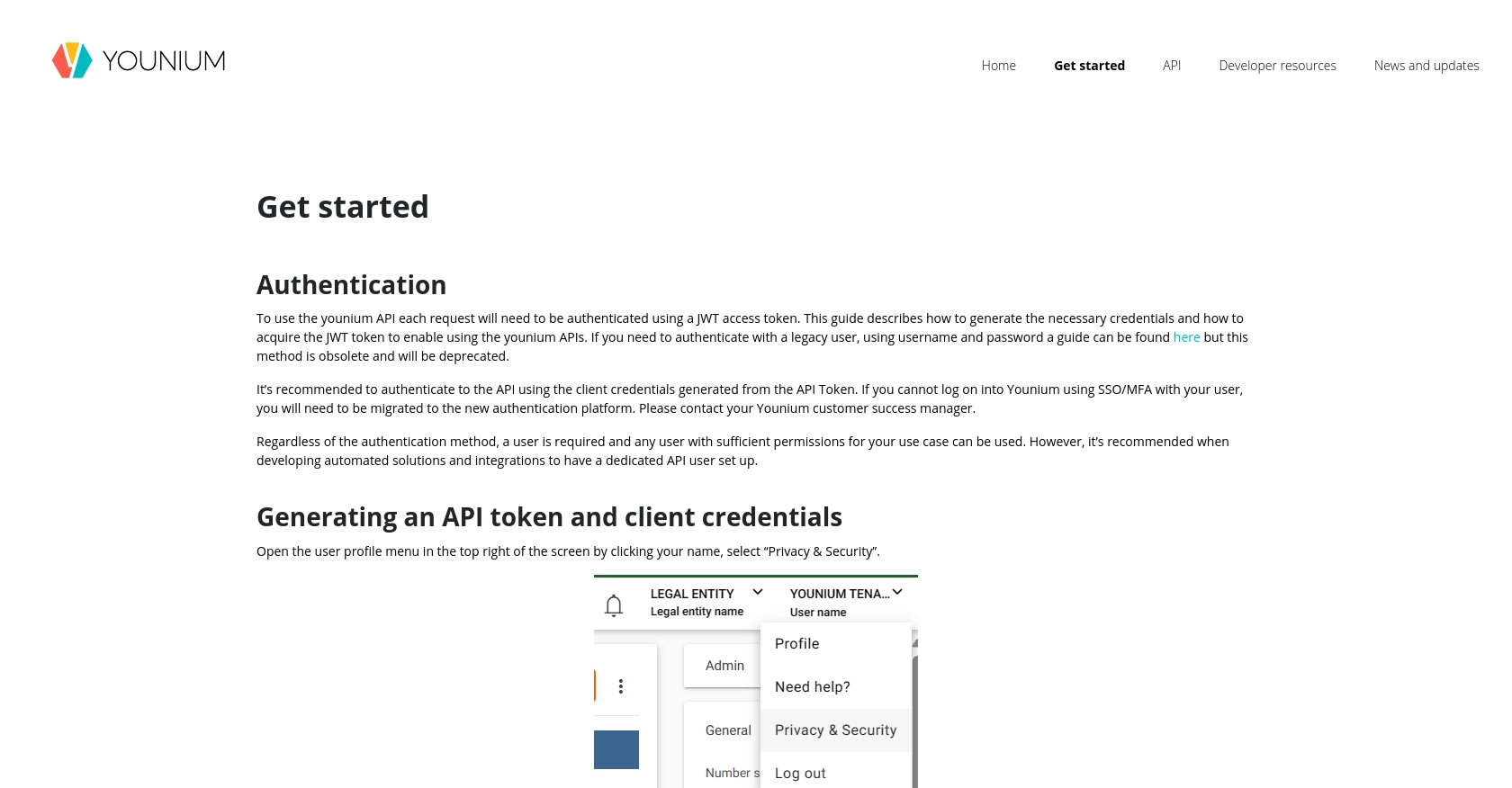
sbb-itb-96038d7
Making API Calls to Retrieve Younium Sales Orders Using Python
With your Younium sandbox account and JWT access token ready, you can now proceed to make API calls to retrieve sales orders. This section will guide you through the process using Python, ensuring you have the necessary tools and code to interact with the Younium API effectively.
Setting Up Your Python Environment for Younium API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Retrieving Sales Orders from Younium API
To retrieve sales orders, you'll need to make a GET request to the Younium API endpoint. Below is a sample Python script to help you get started:
import requests
# Define the API endpoint for retrieving sales orders
url = "https://api.sandbox.younium.com/salesorders"
# Set the request headers, including the JWT access token
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1"
}
# Make the GET request to the Younium API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
sales_orders = response.json()
print("Sales Orders:", sales_orders)
else:
print("Failed to retrieve sales orders. Status Code:", response.status_code)
Replace Your_Access_Token
with the JWT token obtained earlier. This script sends a GET request to the Younium API to fetch sales orders and prints them if the request is successful.
Verifying API Call Success and Handling Errors
Upon executing the script, you should see the sales orders data printed in the console. If the request fails, the script will output the status code, helping you diagnose the issue. Common error codes include:
- 401 Unauthorized: Indicates an expired or incorrect access token.
- 403 Forbidden: Suggests insufficient permissions or incorrect legal entity specified.
For more error handling details, refer to the Younium API Documentation.
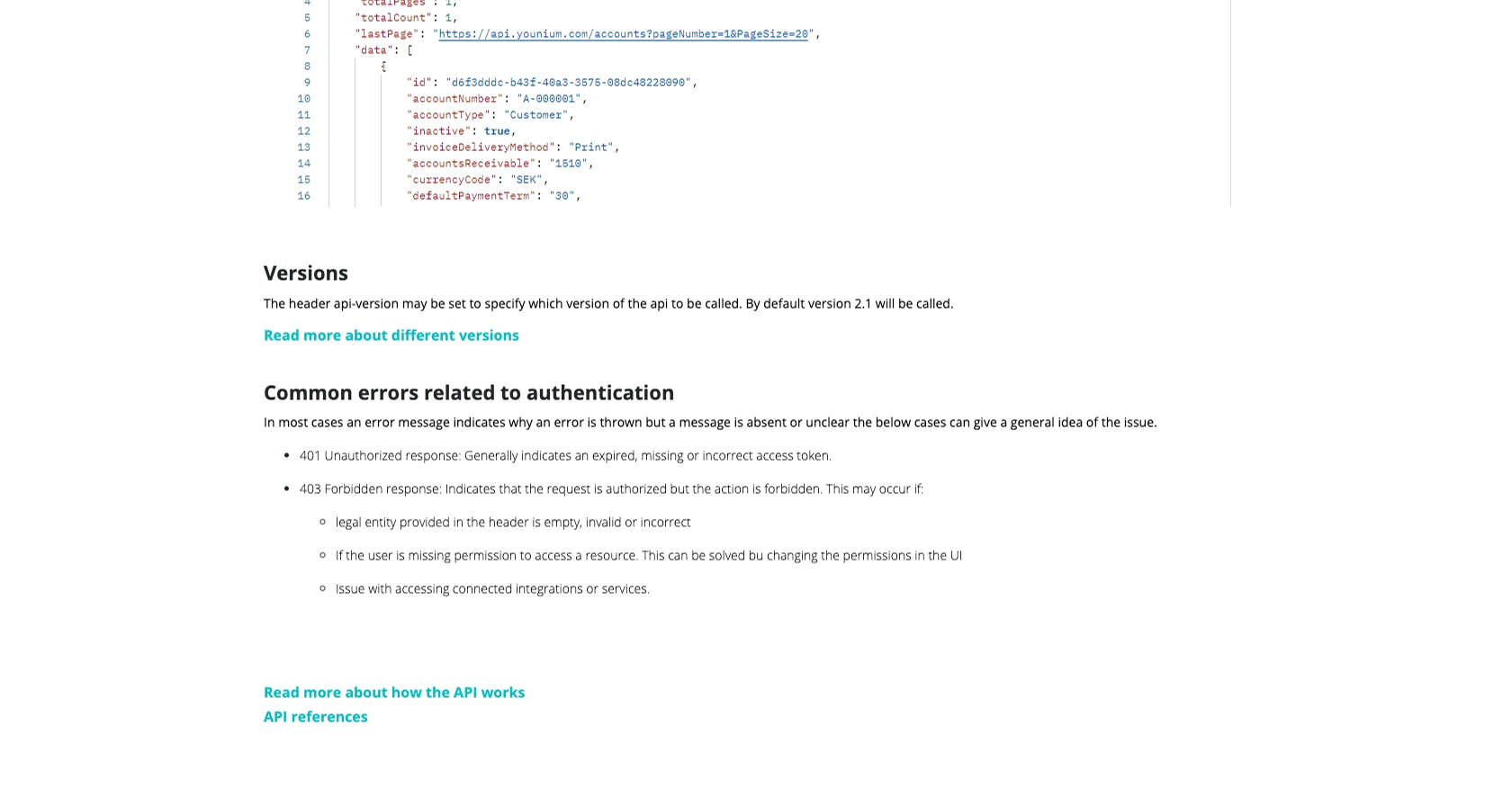
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API provides a powerful way to automate and enhance your financial operations by accessing and managing sales orders efficiently. By following the steps outlined in this guide, you can seamlessly connect your applications with Younium, ensuring real-time data synchronization and improved business insights.
Best Practices for Secure and Efficient Younium API Usage
- Securely Store Credentials: Always store your Client ID, Secret Key, and JWT access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle retries gracefully.
- Standardize Data Fields: Ensure consistent data formats when integrating Younium data with other systems. This helps maintain data integrity and simplifies reporting.
- Regularly Refresh Tokens: Since JWT tokens expire after 24 hours, automate the token refresh process to maintain uninterrupted API access.
Streamlining Integrations with Endgrate
While integrating with Younium can significantly enhance your operations, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including Younium.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Whether you need to build once for multiple integrations or provide an intuitive integration experience for your customers, Endgrate can help streamline your efforts.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a unified API approach.
Read More
Ready to get started?