Using the Xero API to Create or Update Items (with Javascript examples)
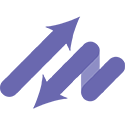
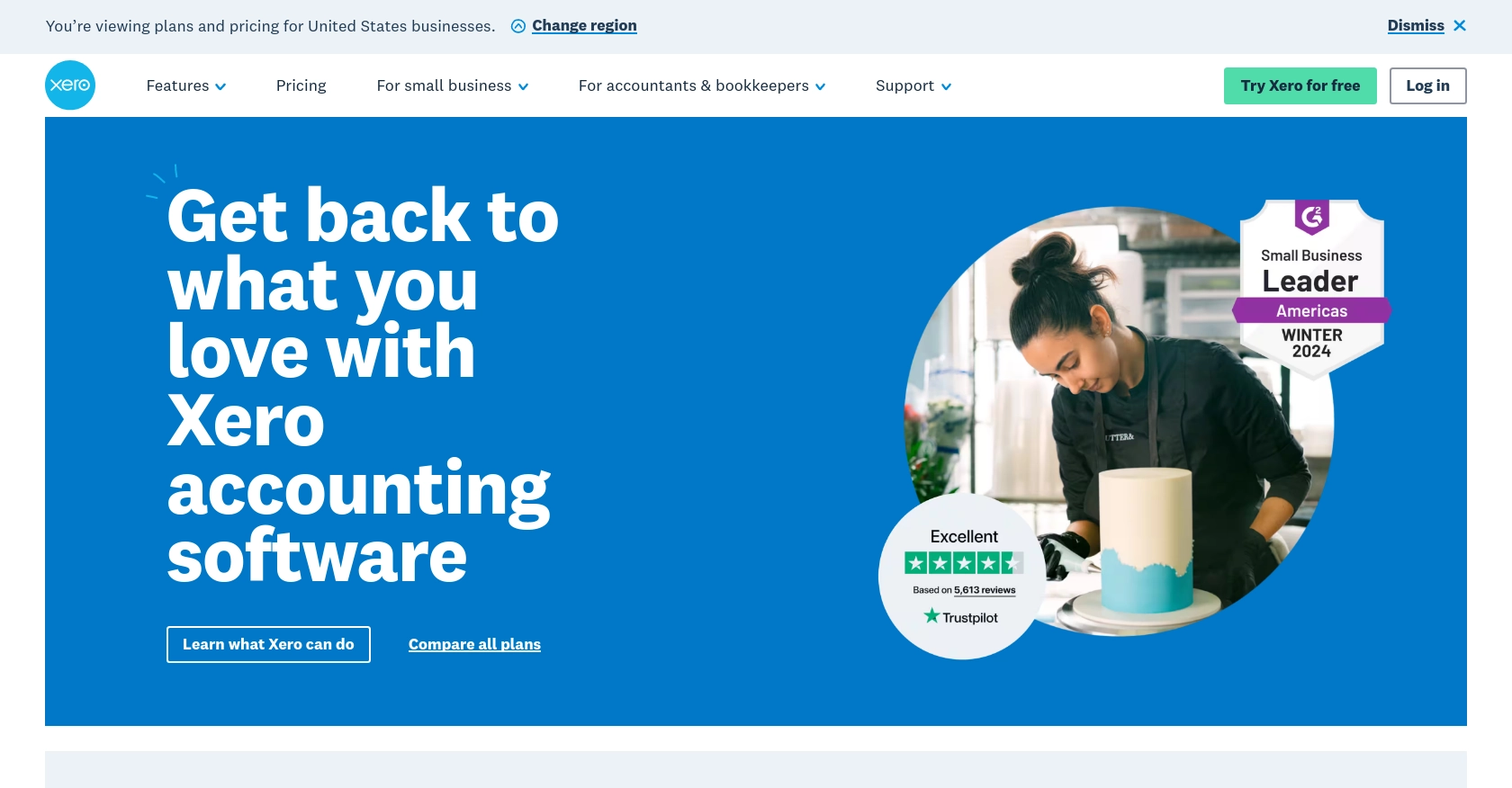
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help businesses manage their finances efficiently. With features like invoicing, payroll, and inventory management, Xero is a popular choice for small to medium-sized enterprises looking to streamline their financial operations.
Integrating with Xero's API allows developers to automate and enhance accounting processes, such as creating or updating inventory items. For example, a developer might use the Xero API to automatically update stock levels in Xero when a sale is made on an e-commerce platform, ensuring accurate inventory tracking and financial reporting.
Setting Up Your Xero Test/Sandbox Account for API Integration
Before you can start integrating with the Xero API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Xero Developer Account
If you don't have a Xero developer account, you'll need to create one. Visit the Xero Developer Portal and sign up for a free account. This account will give you access to the tools and resources needed for API integration.
Set Up a Xero Sandbox Organization
Once your developer account is ready, you can create a sandbox organization. This is a simulated environment where you can test your API interactions:
- Log in to your Xero developer account.
- Navigate to the "My Apps" section and click on "Create App."
- Fill in the required details, such as the app name and company URL.
- Select the "Accounting" API and any other APIs you plan to use.
- Click "Create App" to generate your sandbox organization.
Configure OAuth 2.0 Authentication
Xero uses OAuth 2.0 for authentication, which requires setting up an app to obtain the necessary credentials:
- In your Xero app settings, navigate to the "OAuth 2.0 Credentials" section.
- Note down the Client ID and Client Secret provided.
- Set up a redirect URI, which is the URL to which users will be redirected after authentication.
- Ensure you have the necessary scopes for accessing items, as detailed in the Xero OAuth 2.0 Scopes documentation.
Authorize Your Application
To interact with the Xero API, you'll need to authorize your application:
- Direct users to the Xero authorization URL, including your Client ID and requested scopes.
- After users log in and approve access, they'll be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to the Xero token endpoint.
For more details, refer to the Xero OAuth 2.0 Authorization Flow documentation.
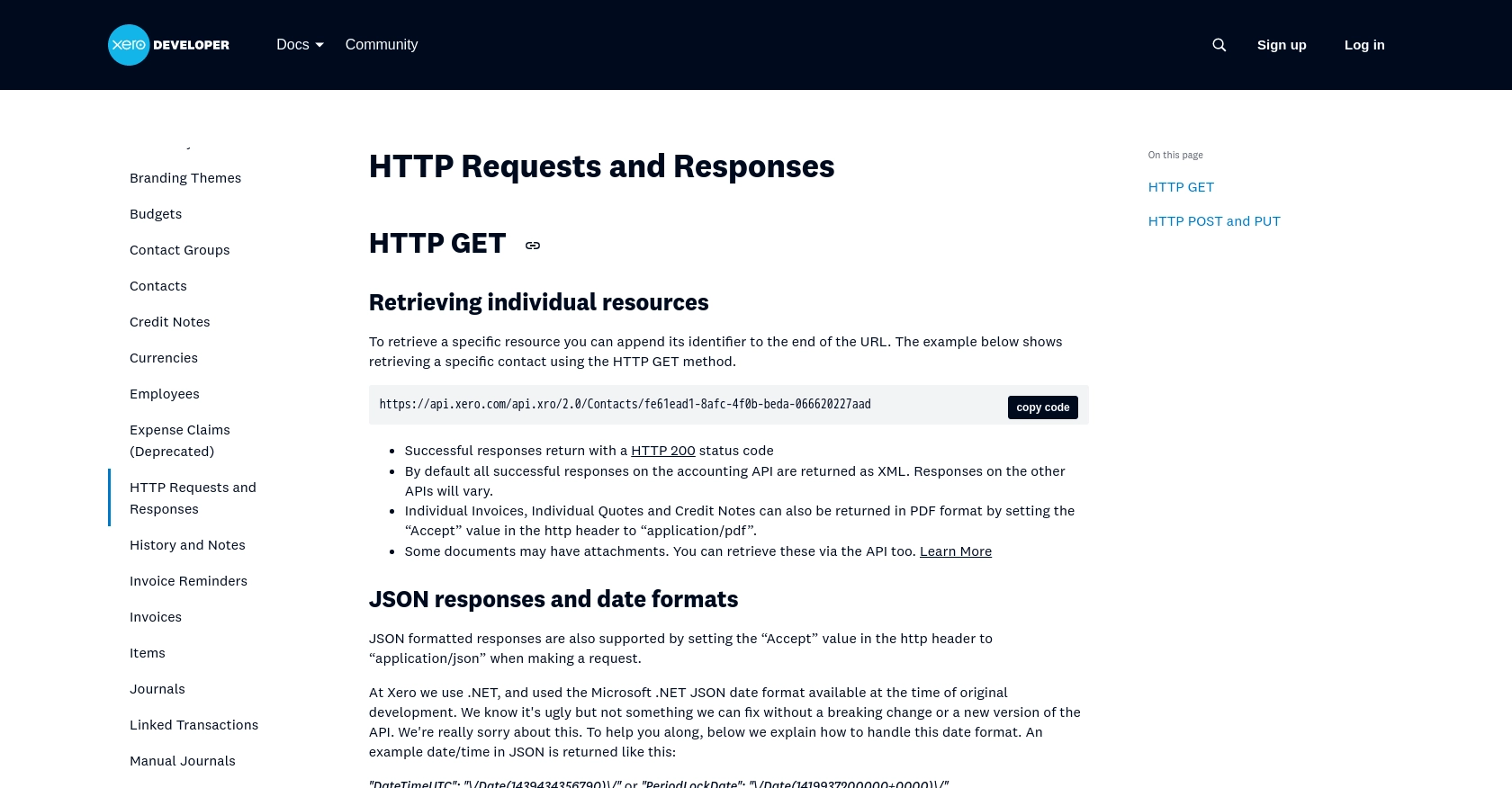
sbb-itb-96038d7
Making API Calls to Xero for Creating or Updating Items Using JavaScript
To interact with the Xero API for creating or updating items, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side operations.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
in your terminal to check the version.
Next, you'll need to install the Axios library, which simplifies making HTTP requests:
npm install axios
Writing JavaScript Code to Create or Update Items in Xero
With your environment set up, you can now write the JavaScript code to interact with the Xero API. Below is an example of how to create or update an item:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.xero.com/api.xro/2.0/Items';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Define the item data
const itemData = {
"Code": "ITEM001",
"Description": "Sample Item",
"PurchaseDetails": {
"UnitPrice": 20.00,
"AccountCode": "500"
},
"SalesDetails": {
"UnitPrice": 30.00,
"AccountCode": "200"
}
};
// Function to create or update an item
async function createOrUpdateItem() {
try {
const response = await axios.post(endpoint, itemData, { headers });
console.log('Item created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating item:', error.response.data);
}
}
createOrUpdateItem();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth 2.0 authentication process.
Verifying API Call Success and Handling Errors
After running the code, you should verify the success of the API call:
- Check the console output for a success message and the returned data.
- Log in to your Xero sandbox account and navigate to the items section to confirm the item was created or updated.
Handling errors is crucial for robust integration. The example code above includes a try-catch
block to catch and log any errors. Refer to the Xero API documentation for detailed error codes and their meanings.
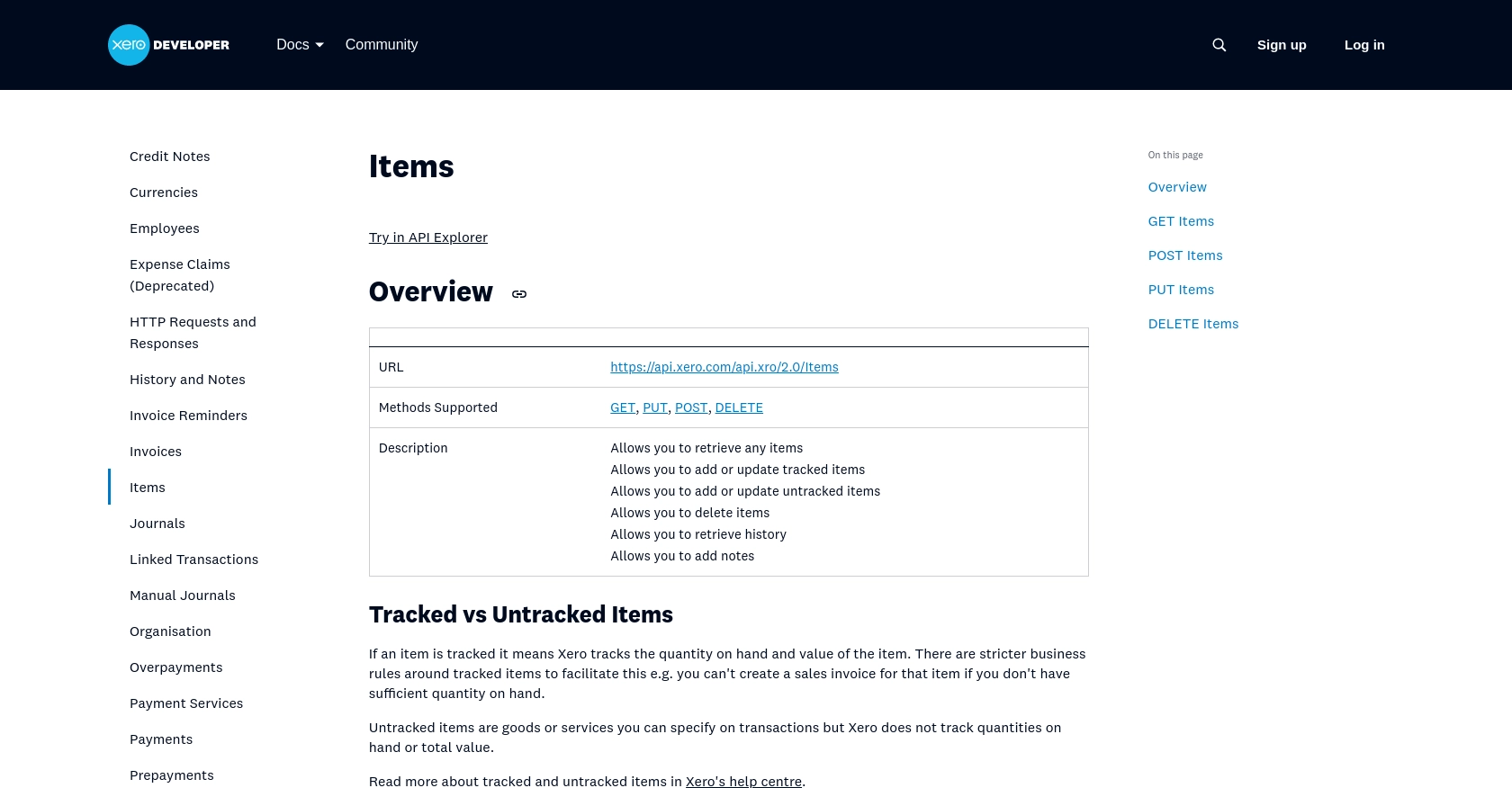
Conclusion and Best Practices for Xero API Integration Using JavaScript
Integrating with the Xero API to create or update items using JavaScript can significantly enhance your business's accounting processes. By automating inventory management, you ensure accurate financial reporting and streamline operations. However, to make the most of this integration, it's essential to follow best practices.
Best Practices for Secure and Efficient Xero API Integration
- Securely Store Credentials: Always store your OAuth 2.0 credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be sure to implement logic to handle rate limit responses gracefully. For more information, refer to the Xero API rate limits documentation.
- Standardize Data Fields: Ensure that the data fields you send to Xero are standardized and validated. This minimizes errors and ensures data consistency across platforms.
- Implement Error Handling: Use robust error handling to manage API call failures. Log errors for debugging and provide meaningful feedback to users.
Streamlining Integrations with Endgrate
While integrating with Xero's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can help. By providing a unified API endpoint, Endgrate allows you to connect with multiple platforms, including Xero, through a single integration point.
With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover a more efficient way to manage your business integrations.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/items
Ready to get started?