How to Create Sales Invoices with the Sage Accounting API in Javascript
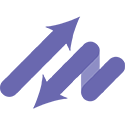
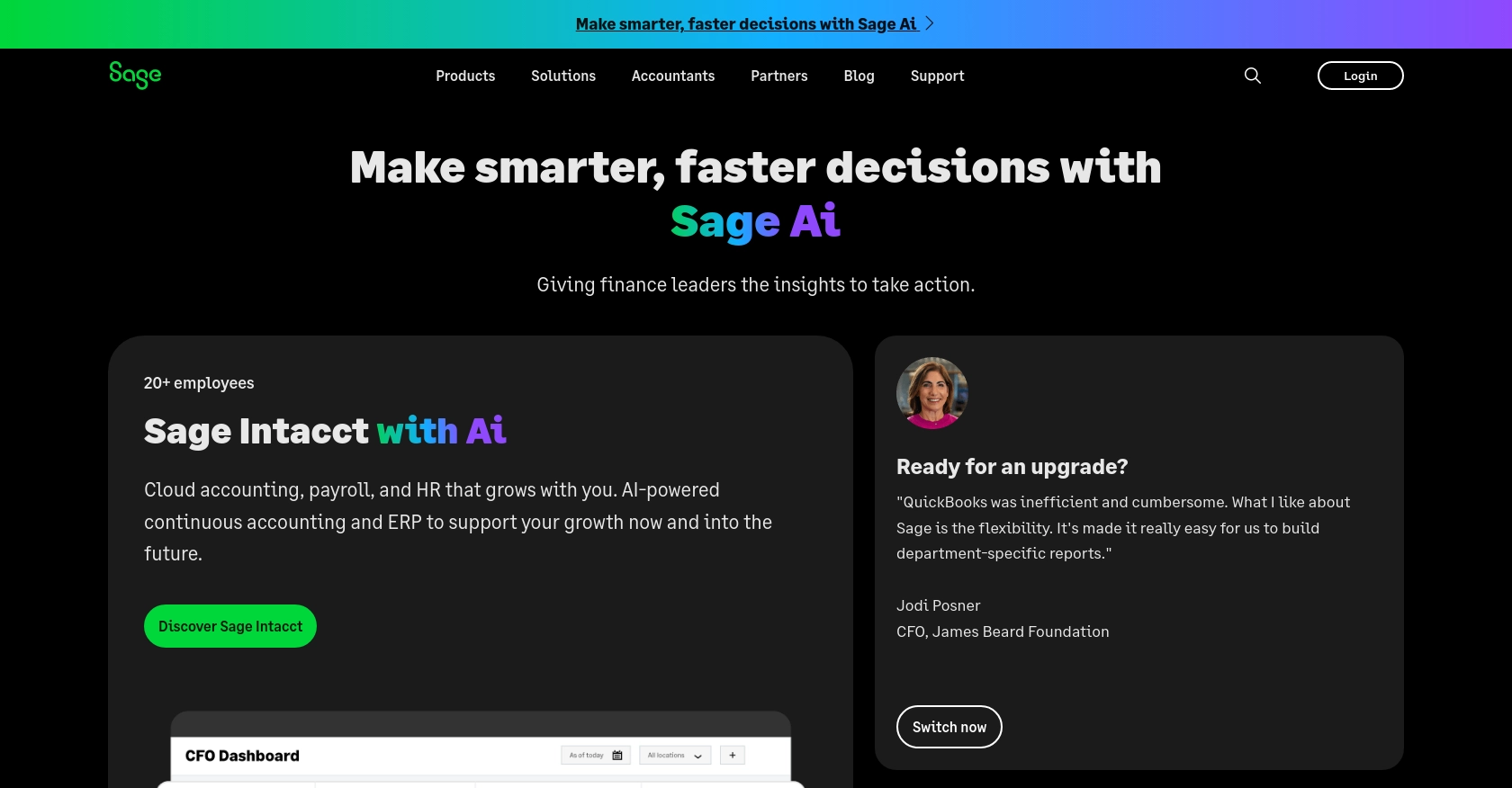
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small and medium-sized businesses. It offers a comprehensive suite of tools to manage finances, including invoicing, expense tracking, and financial reporting. Sage Accounting's flexibility and user-friendly interface make it a popular choice for businesses looking to streamline their financial operations.
Integrating with the Sage Accounting API allows developers to automate and enhance financial processes, such as creating sales invoices. For example, a developer might use the Sage Accounting API to automatically generate and send invoices to clients, reducing manual entry and improving efficiency.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you can start creating sales invoices with the Sage Accounting API, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting real data. Follow these steps to get started:
Create a Sage Developer Account
To begin, you'll need a Sage Developer account. This account will enable you to register and manage your applications, obtain client credentials, and specify key details such as callback URLs.
- Visit the Sage Developer Portal and sign up using your GitHub account or an email address.
- Follow the guide on creating an app to complete your registration.
Register a Trial Business for Development
Next, you'll need to set up a trial business account. This will allow you to test your integration with Sage Accounting.
- Choose the appropriate region and subscription tier for your trial business. You can find more details and sign-up links on the Sage Developer Portal.
- Ensure you understand the differences in functionality between subscription tiers to align with your integration's intended scope.
Create a Sage Accounting App
With your developer account and trial business set up, you can now create a Sage Accounting app to obtain the necessary credentials for API access.
- Log in to your Sage Developer account.
- Navigate to the "Create App" section and enter a name and callback URL for your app. Optionally, provide an alternative email address and homepage URL.
- Click "Save" to generate your Client ID and Client Secret, which you'll use for OAuth authentication.
Upgrade to a Developer Account
To fully utilize the Sage Accounting API, upgrade your trial account to a developer account. This grants you 12 months of free access for testing purposes.
- Submit the upgrade request form with details such as your name, email address, app name, Client ID, and region.
- Wait for confirmation from the Sage team, which typically takes 3-5 working days.
Once your developer account is set up, you'll be ready to start integrating with the Sage Accounting API and creating sales invoices programmatically.
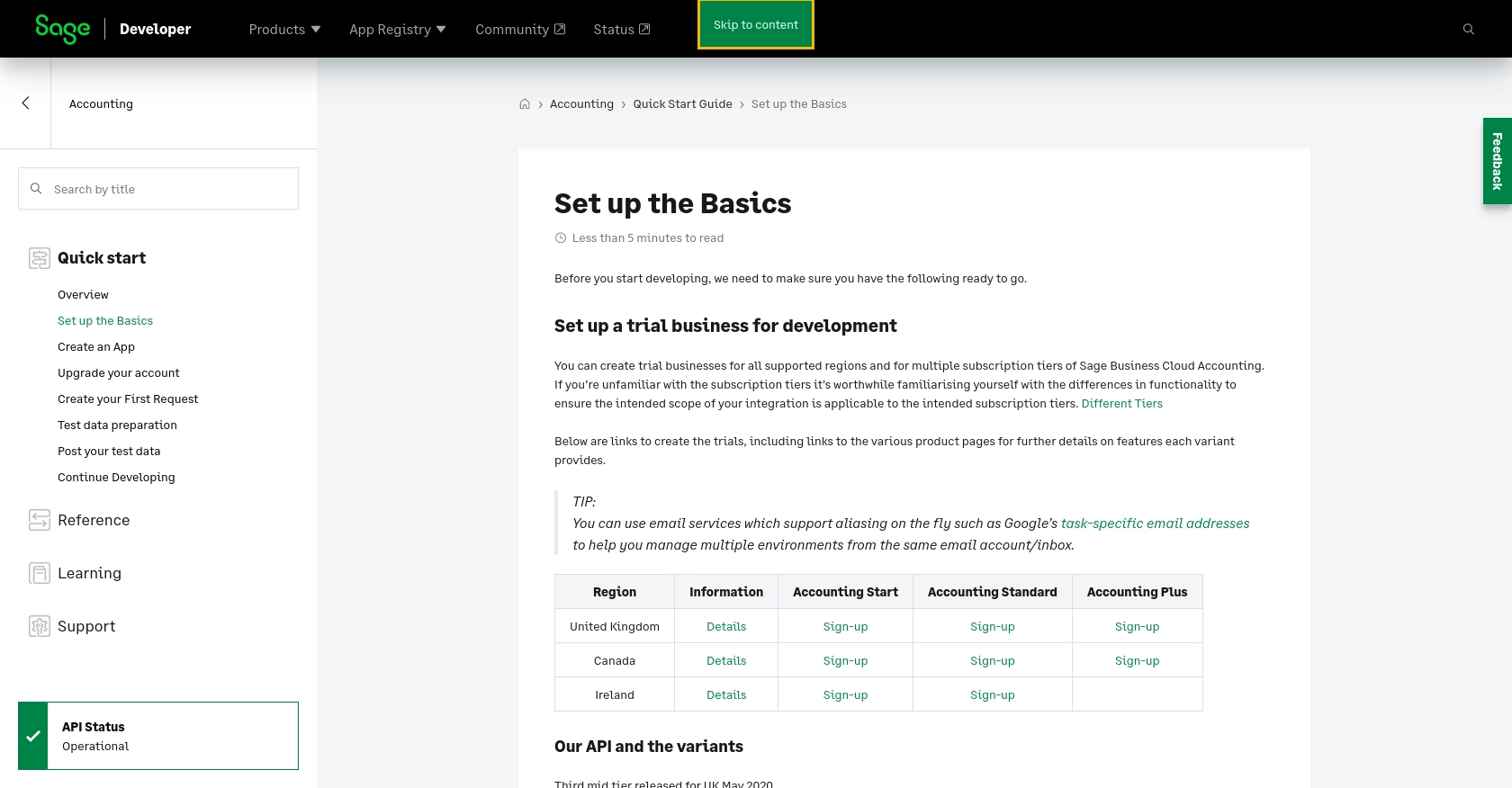
sbb-itb-96038d7
How to Make the Actual API Call to Create Sales Invoices with Sage Accounting API in JavaScript
Setting Up Your JavaScript Environment for Sage Accounting API
Before making API calls, ensure your JavaScript environment is properly configured. You'll need Node.js installed on your machine. If you haven't already, download and install Node.js from the official website.
Once Node.js is installed, create a new project directory and initialize it:
mkdir sage-invoice-project
cd sage-invoice-project
npm init -y
Installing Required Dependencies
To interact with the Sage Accounting API, you'll need to use the Axios library for making HTTP requests. Install it using npm:
npm install axios
Creating a Sales Invoice with Sage Accounting API
Now, let's create a script to make an API call to Sage Accounting to create a sales invoice. Create a new file named createInvoice.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.sage.com/accounting/v3/sales_invoices';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
// Define the invoice data
const invoiceData = {
"contact_id": "123456",
"date": "2023-10-01",
"due_date": "2023-10-15",
"line_items": [
{
"description": "Web Development Services",
"quantity": 10,
"unit_price": 100
}
]
};
// Make a POST request to create the invoice
axios.post(endpoint, invoiceData, { headers })
.then(response => {
console.log('Invoice Created Successfully:', response.data);
})
.catch(error => {
console.error('Error Creating Invoice:', error.response.data);
});
Replace Your_Access_Token
with the OAuth token you obtained during the setup process.
Running the Script and Verifying the API Call
Run the script using Node.js:
node createInvoice.js
If successful, you should see a message confirming the invoice creation along with the invoice details. Verify the creation by checking your Sage Accounting sandbox account.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. Common error codes include:
- 400 Bad Request: The request was invalid. Check your data format.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The endpoint or resource could not be found.
Ensure your error handling logic captures these scenarios to provide meaningful feedback to users.
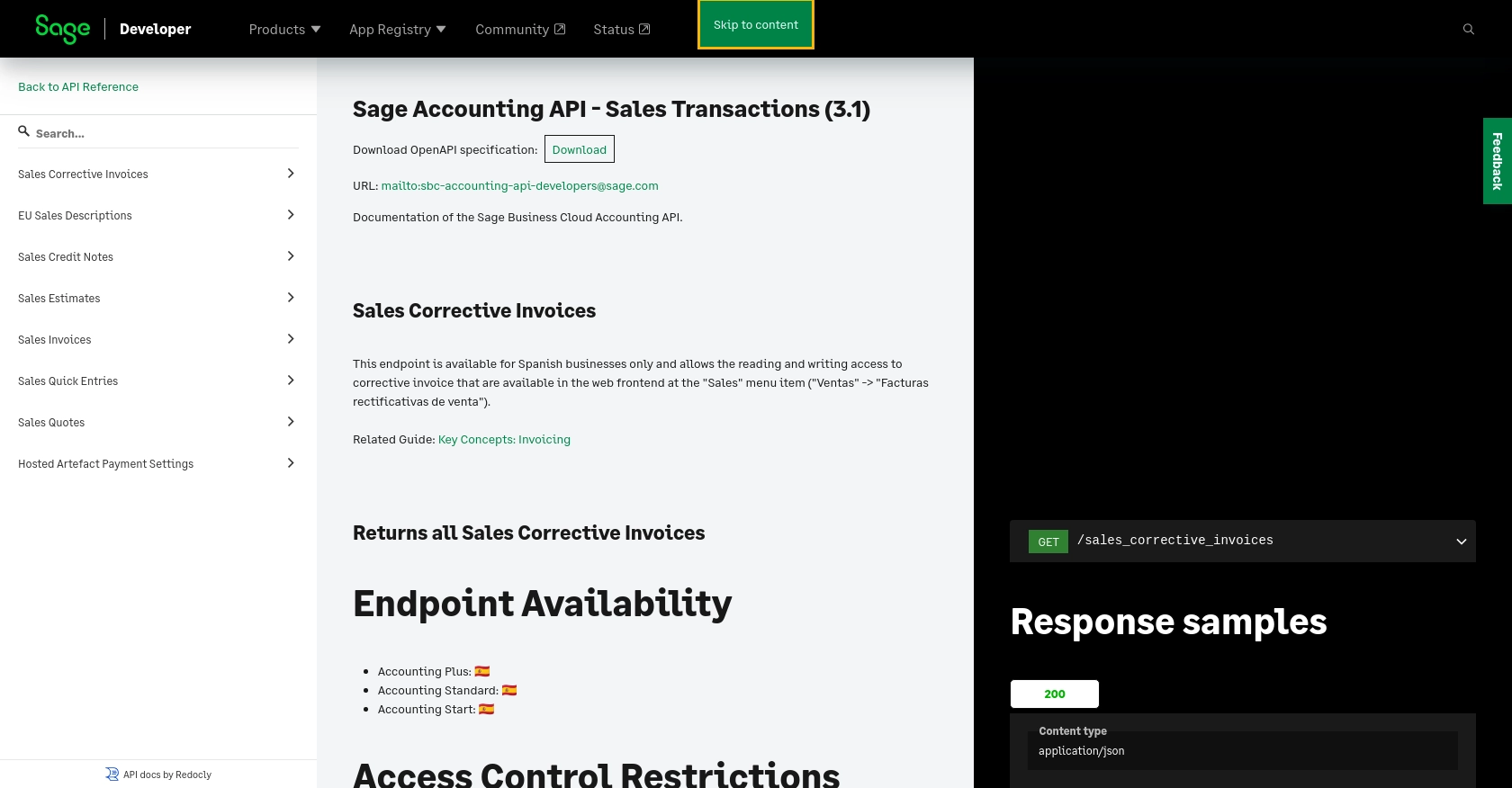
Conclusion and Best Practices for Using Sage Accounting API
Integrating with the Sage Accounting API to create sales invoices in JavaScript can significantly streamline your financial processes, reducing manual effort and increasing efficiency. By following the steps outlined in this guide, you can automate invoice generation and ensure seamless financial operations.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth tokens and client credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send and receive is standardized to maintain consistency across your systems. This includes formatting dates, currency, and other fields appropriately.
- Error Handling: Implement robust error handling to capture and respond to common API errors, providing clear feedback to users and maintaining application stability.
Enhance Your Integration Capabilities with Endgrate
While integrating with Sage Accounting API can be powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover how you can save time and resources while enhancing your product's capabilities.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/invoicing-sales/
Ready to get started?