Using the Zoho CRM API to Get Deals (with Javascript examples)
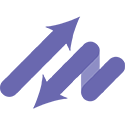
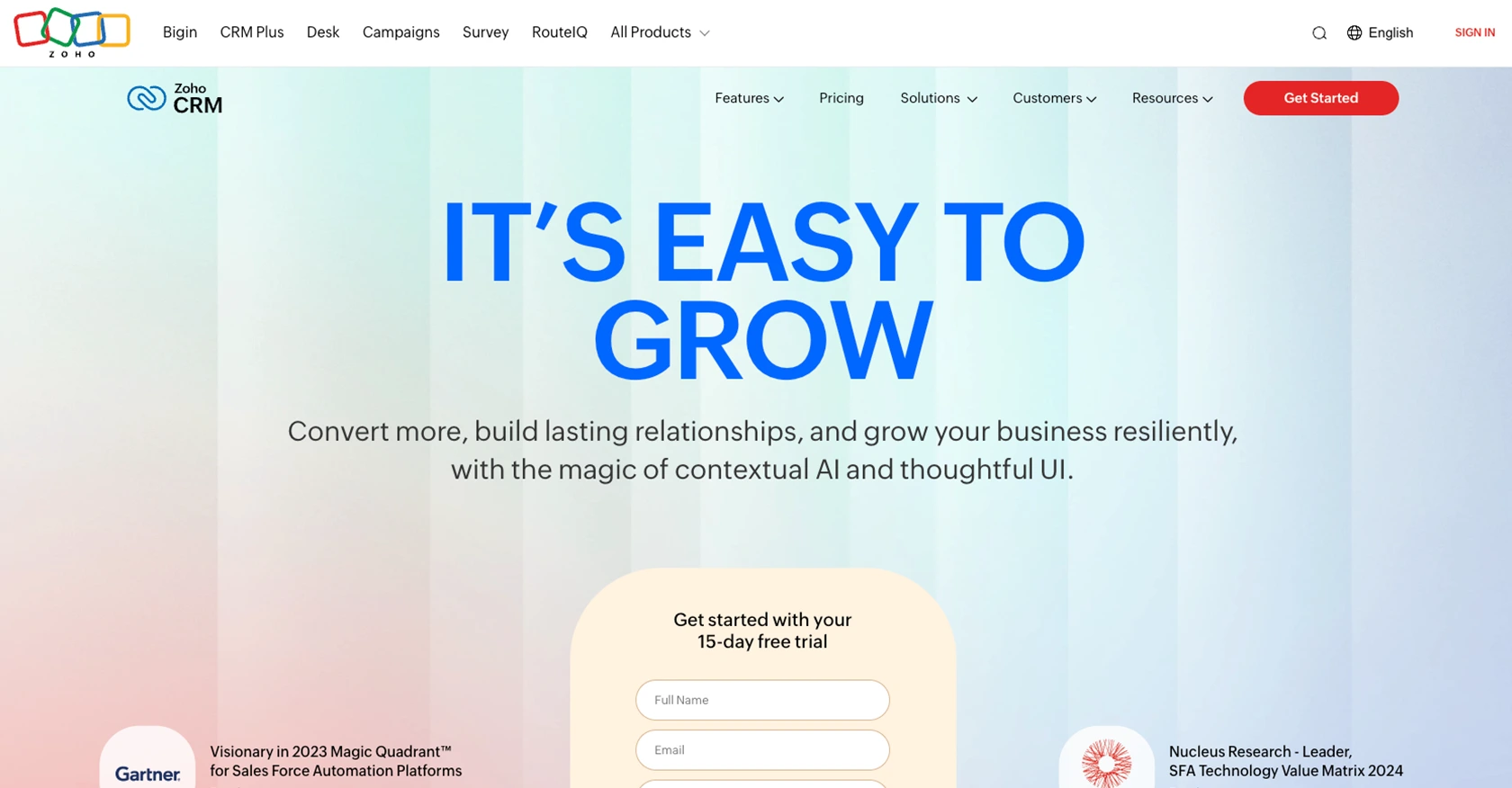
Introduction to Zoho CRM and Its API Integration
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a single system. Known for its flexibility and robust features, Zoho CRM is a popular choice for organizations looking to enhance their customer engagement and streamline operations.
Developers often integrate with Zoho CRM to automate and optimize various business processes. By leveraging the Zoho CRM API, developers can access and manipulate data such as deals, contacts, and leads, enabling seamless integration with other business tools and systems.
For example, a developer might use the Zoho CRM API to retrieve deal information and integrate it with a custom analytics dashboard, providing real-time insights into sales performance and helping teams make data-driven decisions.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you can start integrating with the Zoho CRM API, you need to set up a test or sandbox account. This allows you to safely test your API calls without affecting live data. Zoho CRM offers a developer sandbox environment that mirrors your production setup, making it ideal for testing integrations.
Step-by-Step Guide to Creating a Zoho CRM Sandbox Account
- Sign Up for Zoho CRM: If you don't already have a Zoho CRM account, visit the Zoho CRM sign-up page and create a free account. Follow the on-screen instructions to complete the registration process.
- Access the Developer Console: Once logged in, navigate to the Zoho Developer Console by selecting Setup from the top navigation bar, then choose Developer Space and click on Sandbox.
- Create a Sandbox: Click on Create Sandbox and provide a name and description for your sandbox environment. This will create a replica of your current CRM setup for testing purposes.
Configuring OAuth Authentication for Zoho CRM API
Zoho CRM uses OAuth 2.0 for authentication, which is a secure and industry-standard protocol. Follow these steps to set up OAuth authentication:
- Register Your Application: Go to the Zoho Developer Console and click on Register Client. Choose the client type as Web Based and fill in the required details such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Obtain Client ID and Secret: After registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for making API calls.
-
Set Scopes: Define the scopes for your application to specify the type of resources it can access. For accessing deals, use the scope
ZohoCRM.modules.deals.ALL
. - Generate Access Tokens: Use the Client ID and Secret to generate access and refresh tokens by making an authorization request. Refer to the Zoho CRM OAuth documentation for detailed steps.
Testing Your Zoho CRM API Setup
Once your sandbox and OAuth setup are complete, you can begin testing API calls. Use the sandbox environment to ensure your integration works as expected without impacting your live data.
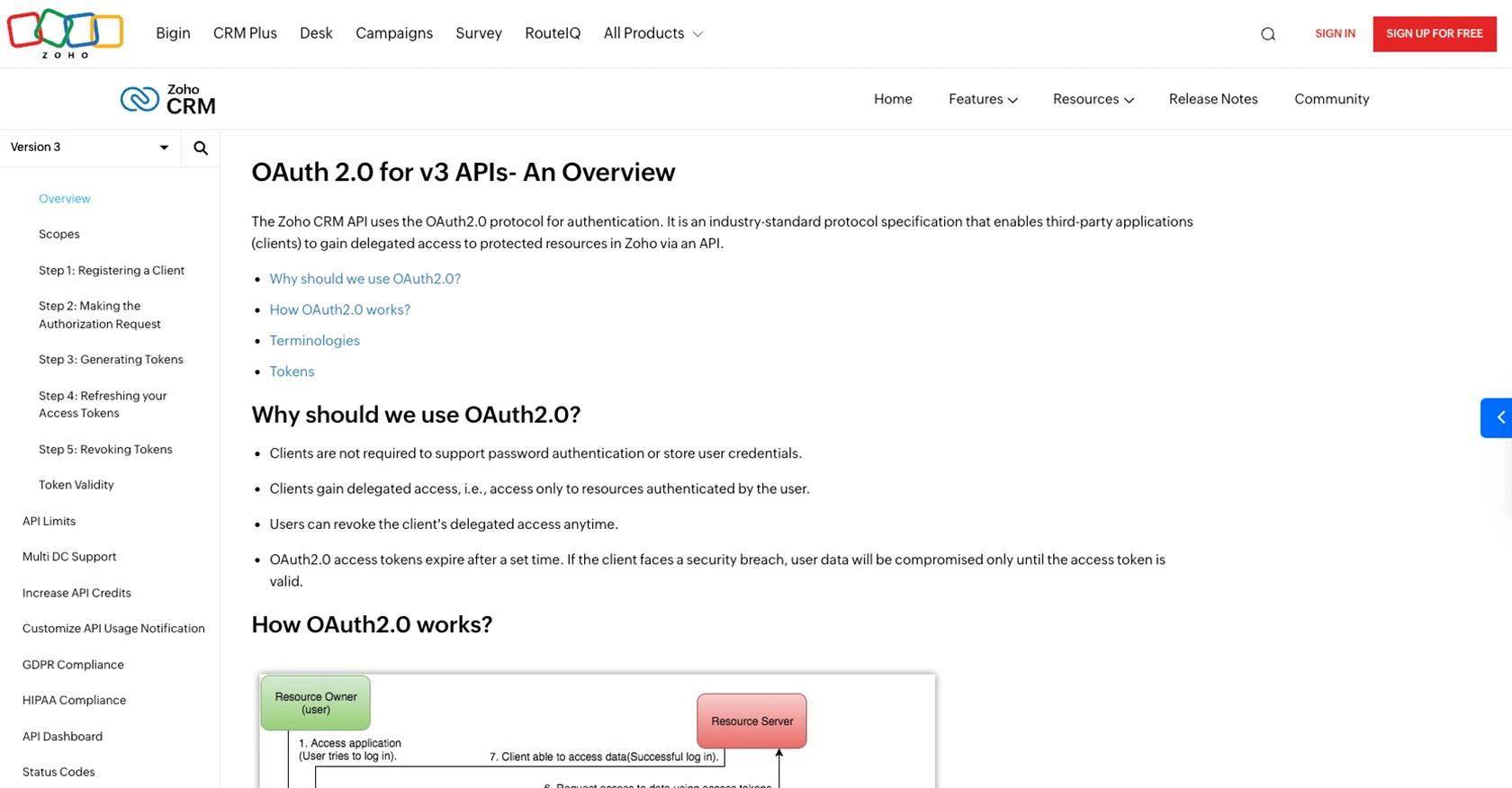
sbb-itb-96038d7
Making API Calls to Retrieve Deals from Zoho CRM Using JavaScript
To interact with the Zoho CRM API and retrieve deals, you'll need to set up your environment with the necessary tools and libraries. This section will guide you through the process of making API calls using JavaScript, ensuring you can efficiently access and manage deal data.
Setting Up Your JavaScript Environment for Zoho CRM API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a browser.
- Install Node.js: Download and install Node.js from the official website. This will also install npm, the Node package manager.
-
Initialize Your Project: Create a new directory for your project and run
npm init -y
to initialize a new Node.js project. -
Install Axios: Use Axios, a promise-based HTTP client, to make API requests. Install it by running
npm install axios
.
Example Code for Fetching Deals from Zoho CRM
With your environment set up, you can now write JavaScript code to fetch deals from Zoho CRM. Below is an example of how to make a GET request to the Zoho CRM API to retrieve deals.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://www.zohoapis.com/crm/v3/Deals';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
};
// Function to fetch deals
async function getDeals() {
try {
const response = await axios.get(endpoint, { headers });
const deals = response.data.data;
console.log('Deals:', deals);
} catch (error) {
console.error('Error fetching deals:', error.response ? error.response.data : error.message);
}
}
// Call the function
getDeals();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. This token is crucial for authenticating your API requests.
Understanding the API Response and Handling Errors
When you make a successful API call, the response will contain an array of deal objects. You can iterate over this array to access individual deal details. Here's how you can handle potential errors:
- Check Response Status: Ensure the response status code is 200, indicating a successful request.
- Handle API Errors: Use try-catch blocks to manage errors. Log error messages to understand issues such as invalid tokens or network problems.
Verifying API Call Success in Zoho CRM Sandbox
After running your code, verify the retrieved deals in your Zoho CRM sandbox environment. This ensures that your integration is functioning correctly and that the data matches your expectations.
For further details on error codes and handling, refer to the Zoho CRM API Status Codes documentation.
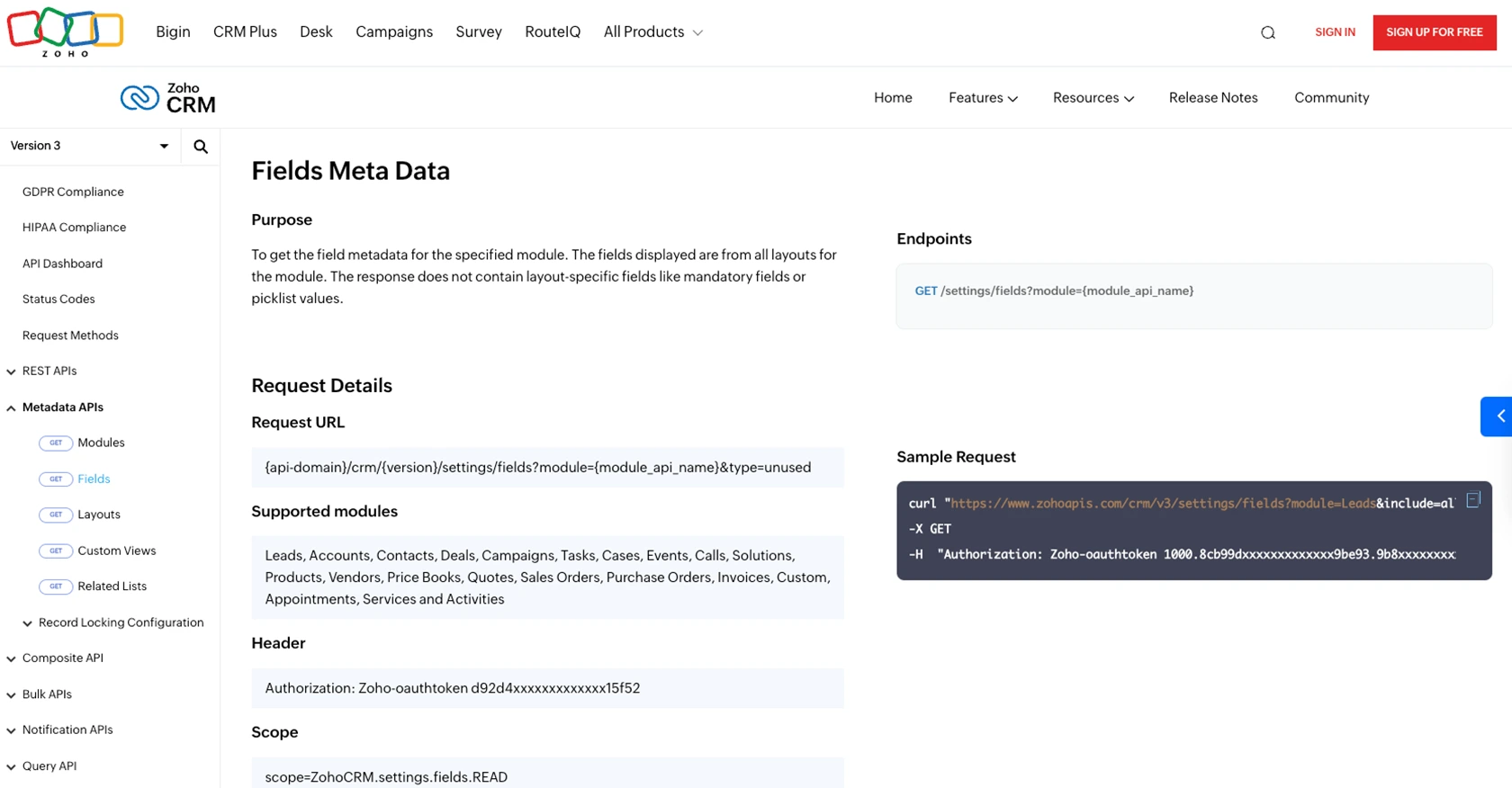
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API allows developers to enhance their applications by accessing and managing crucial business data such as deals, contacts, and leads. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth, and make API calls to retrieve deal information.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Securely Store Credentials: Always keep your Client ID, Client Secret, and access tokens secure. Avoid exposing them in client-side code or public repositories.
- Handle Rate Limiting: Zoho CRM imposes rate limits on API requests. Be mindful of these limits to avoid disruptions. Refer to the API Limits documentation for details.
- Implement Error Handling: Use robust error handling to manage API errors gracefully. This includes checking response status codes and handling exceptions.
- Optimize Data Handling: Transform and standardize data fields as needed to ensure consistency across your application.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline their integration processes, consider leveraging Endgrate. By using Endgrate, you can save time and resources, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies the integration experience across multiple platforms, including Zoho CRM.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a seamless integration experience.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?