Using the Xero API to Create or Update Sales Orders (with PHP examples)
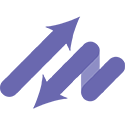
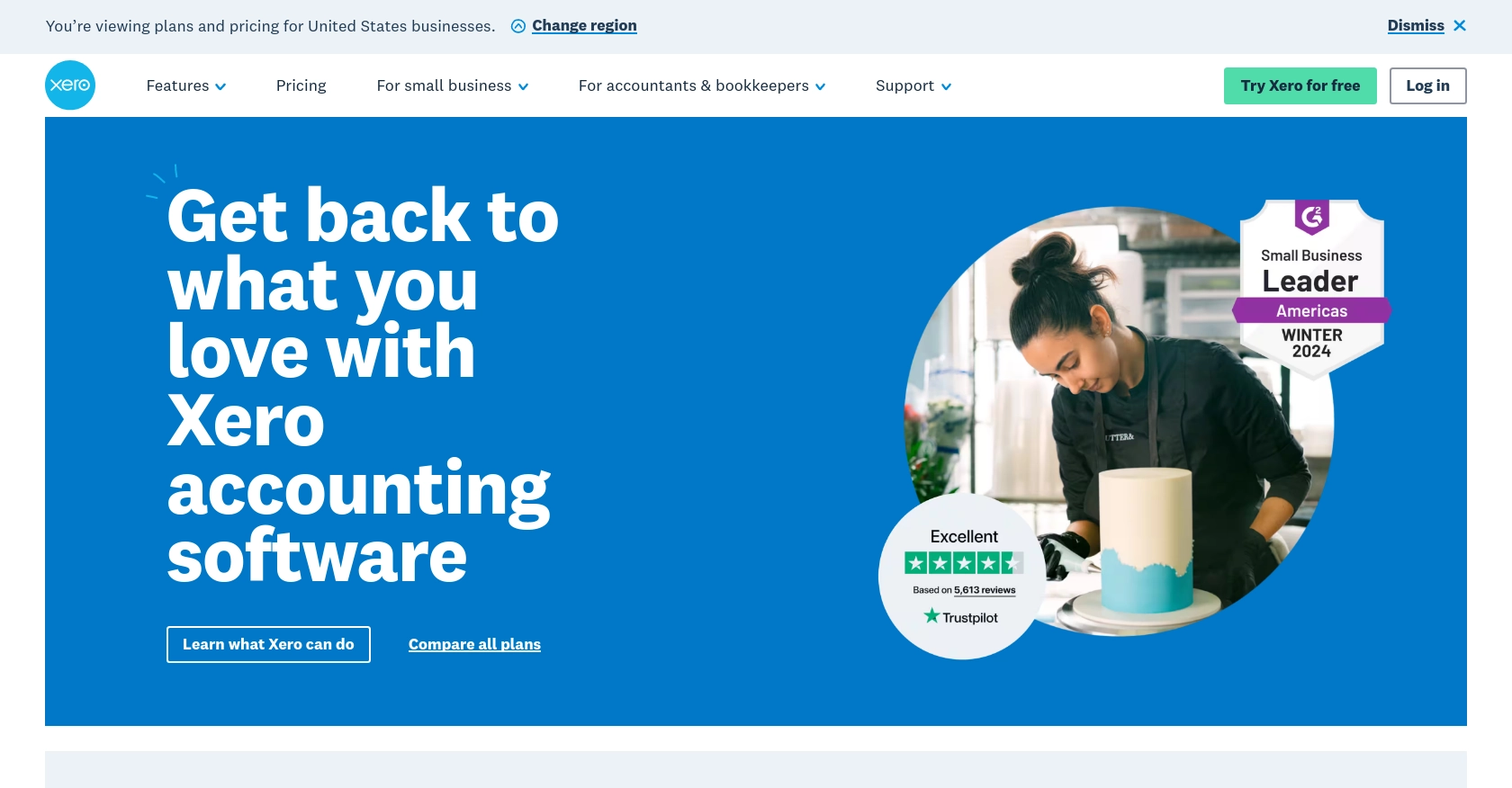
Introduction to Xero API for Sales Orders
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, payroll, and inventory management, Xero offers a comprehensive suite of tools to streamline financial operations.
For developers, integrating with the Xero API can unlock a range of possibilities, such as automating the creation and updating of sales orders. This can be particularly useful for businesses looking to synchronize their sales data with their accounting system, ensuring accuracy and saving time. For example, a developer might use the Xero API to automatically update sales orders from an e-commerce platform, keeping financial records up-to-date without manual intervention.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start integrating with the Xero API to create or update sales orders, you'll need to set up a sandbox account. This environment allows you to test your API interactions without affecting live data, ensuring a smooth development process.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. If you don't have one, follow these steps:
- Visit the Xero Developer Portal and sign up for a free developer account.
- Once registered, log in to your account to access the developer dashboard.
Setting Up a Xero Sandbox Organization
After creating your developer account, the next step is to set up a sandbox organization:
- Navigate to the "My Apps" section in the developer dashboard.
- Select "Add a new app" and fill in the required details, such as the app name and company URL.
- Choose "Web app" as the app type to enable OAuth 2.0 authentication.
- Once your app is created, you'll receive a client ID and client secret, which are essential for API authentication.
Configuring OAuth 2.0 Authentication
Xero uses OAuth 2.0 for secure API authentication. Follow these steps to configure it:
- In your app settings, set the redirect URI to a valid endpoint in your application where users will be redirected after authentication.
- Ensure you have the necessary scopes for accessing sales orders. You can manage scopes in the "Scopes" section of your app settings.
- Use the client ID and client secret to implement the OAuth 2.0 authorization code flow in your application. For more details, refer to the Xero OAuth 2.0 documentation.
Testing API Calls in the Sandbox
With your sandbox organization and OAuth 2.0 authentication set up, you can now test API calls:
- Use the client ID and client secret to obtain an access token.
- Make API requests to the sandbox environment using the access token. Ensure you handle responses and errors appropriately.
- Verify the results in your sandbox organization to confirm that sales orders are being created or updated as expected.
By following these steps, you'll be well-prepared to integrate with the Xero API and automate sales order management efficiently.
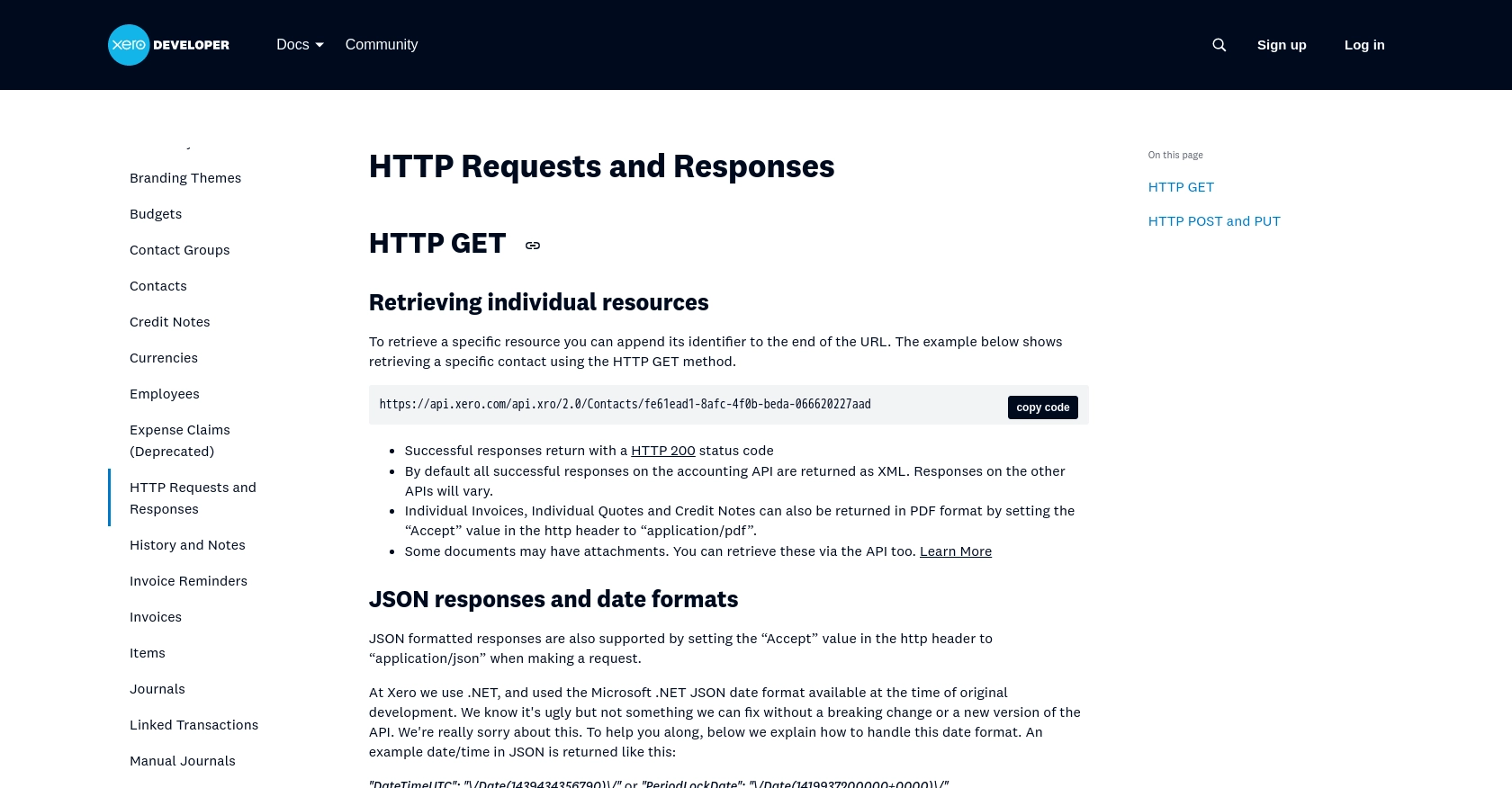
sbb-itb-96038d7
Making API Calls to Xero for Sales Orders Using PHP
To interact with the Xero API for creating or updating sales orders, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment
Before you begin, ensure you have the following installed on your machine:
- PHP 7.4 or higher
- Composer for managing dependencies
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install it:
composer require guzzlehttp/guzzle
Creating and Updating Sales Orders with Xero API
Now, let's write the PHP code to create or update sales orders using the Xero API. You'll need to replace Your_Access_Token
with the access token obtained during the OAuth 2.0 authentication process.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$headers = [
'Authorization' => 'Bearer Your_Access_Token',
'Content-Type' => 'application/json'
];
$salesOrderData = [
'SalesOrders' => [
[
'Contact' => [
'Name' => 'Example Customer'
],
'Date' => '2023-10-01',
'LineItems' => [
[
'Description' => 'Product Description',
'Quantity' => 1,
'UnitAmount' => 100.00
]
]
]
]
];
$response = $client->post('https://api.xero.com/api.xro/2.0/SalesOrders', [
'headers' => $headers,
'json' => $salesOrderData
]);
if ($response->getStatusCode() == 200) {
echo "Sales Order Created/Updated Successfully";
} else {
echo "Failed to Create/Update Sales Order: " . $response->getBody();
}
Verifying API Call Success in Xero Sandbox
After running the code, you should verify the success of your API call by checking the sales orders in your Xero sandbox organization. If the sales order appears as expected, the integration is working correctly.
Handling Errors and Response Codes
It's crucial to handle potential errors when making API calls. The Xero API provides various HTTP status codes to indicate the result of your request. Common codes include:
- 200: Success
- 400: Bad Request
- 401: Unauthorized
- 403: Forbidden
- 404: Not Found
- 500: Internal Server Error
Ensure your application gracefully handles these errors by checking the response status code and implementing appropriate error handling logic.
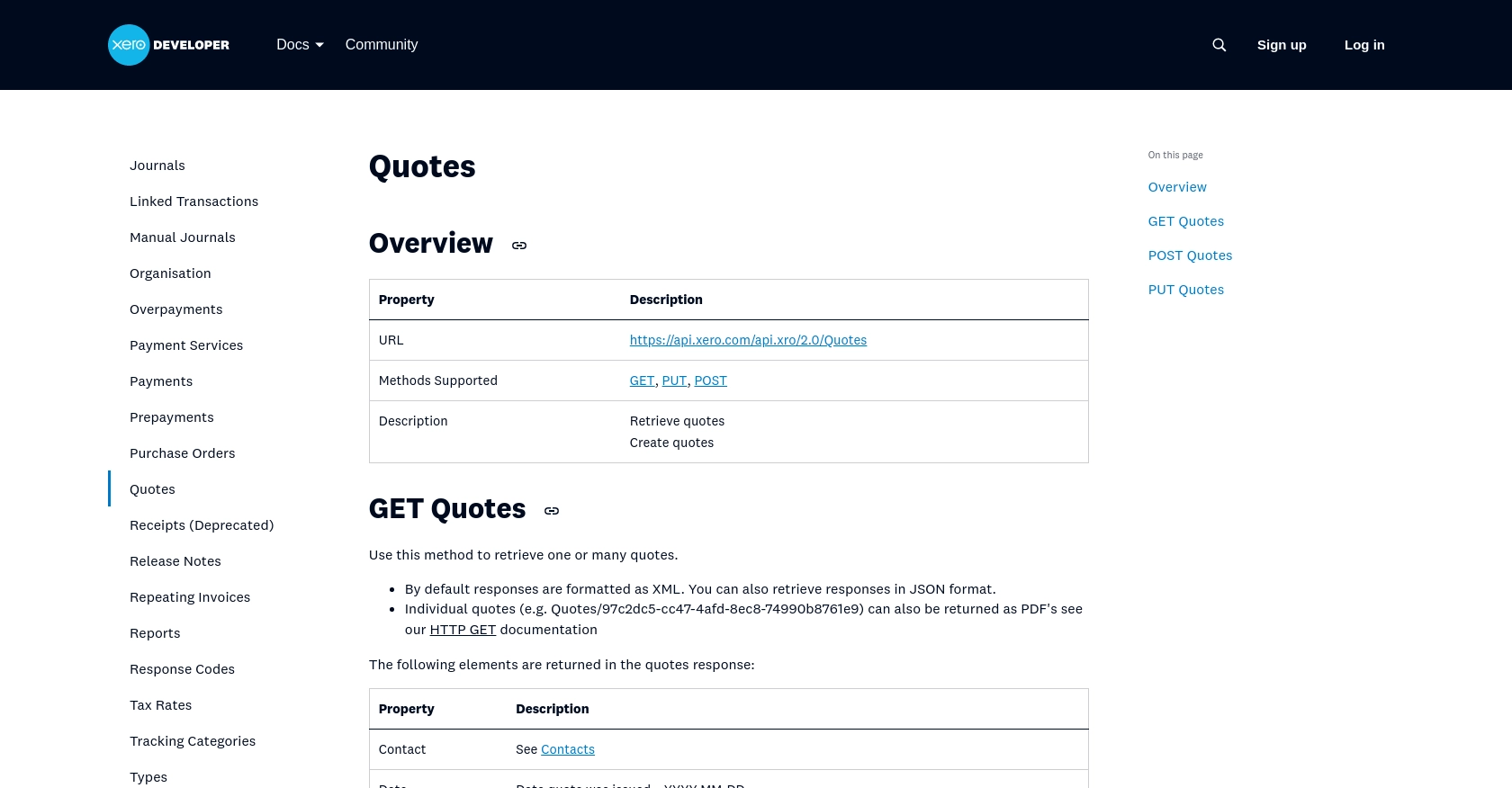
Best Practices for Xero API Integration
Successfully integrating with the Xero API requires adhering to best practices to ensure security, efficiency, and reliability. Here are some key recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be sure to implement logic to handle rate limits gracefully, such as retrying requests after a delay. For more details, refer to the Xero API rate limits documentation.
- Data Transformation and Standardization: Ensure that data being sent to and received from the Xero API is properly formatted and standardized to match your application's requirements.
Streamline Your Integrations with Endgrate
Building and maintaining multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Xero.
With Endgrate, you can:
- Save time and resources by outsourcing integration development and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/quotes
Ready to get started?