How to Get Calls with the Salesloft API in PHP
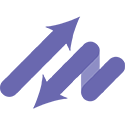
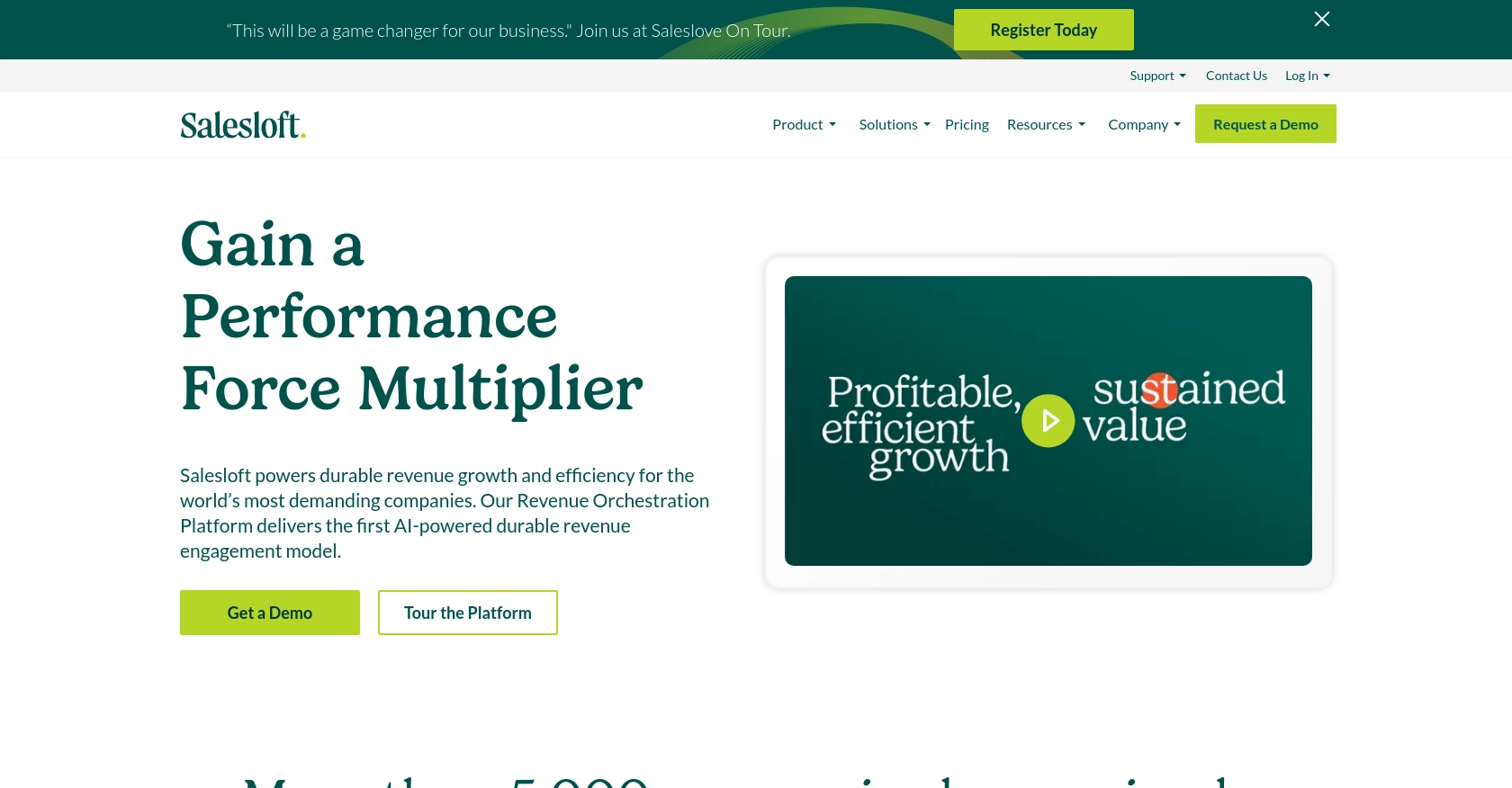
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform that helps sales teams optimize their processes and improve communication with prospects. With features like email tracking, call logging, and analytics, Salesloft enables sales professionals to engage more effectively and close deals faster.
Integrating with the Salesloft API allows developers to access and manage call data, which can be crucial for analyzing sales performance and enhancing customer interactions. For example, a developer might use the Salesloft API to retrieve call records and analyze call durations and outcomes, helping sales teams refine their strategies and improve conversion rates.
Setting Up Your Salesloft Test Account for API Integration
Before you can start interacting with the Salesloft API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Salesloft provides a sandbox environment for developers to test their integrations.
Creating a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial on the Salesloft website. This will give you access to the platform's features and allow you to create a sandbox environment for testing.
Setting Up OAuth Authentication for Salesloft API
The Salesloft API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth authentication:
- Log in to your Salesloft account and navigate to Your Applications under the account settings.
- Select OAuth Applications and click on Create New to create a new application.
- Fill out the required fields and click Save. You will receive an Application Id (Client Id) and Secret (Client Secret).
- Note down the Redirect URI (Callback URL) as you will need it for the authorization process.
Obtaining Authorization Code and Access Tokens
To obtain an access token, follow these steps:
- Generate a request to the Salesloft authorization endpoint using your Client Id and Redirect URI:
- Upon approval, you will receive a code in the redirect URI. Use this code to request an access token:
- You will receive a JSON response with the access_token and refresh_token. Store these tokens securely as they will be used to authenticate your API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "YOUR_AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
For more detailed information, refer to the Salesloft OAuth Authentication documentation.
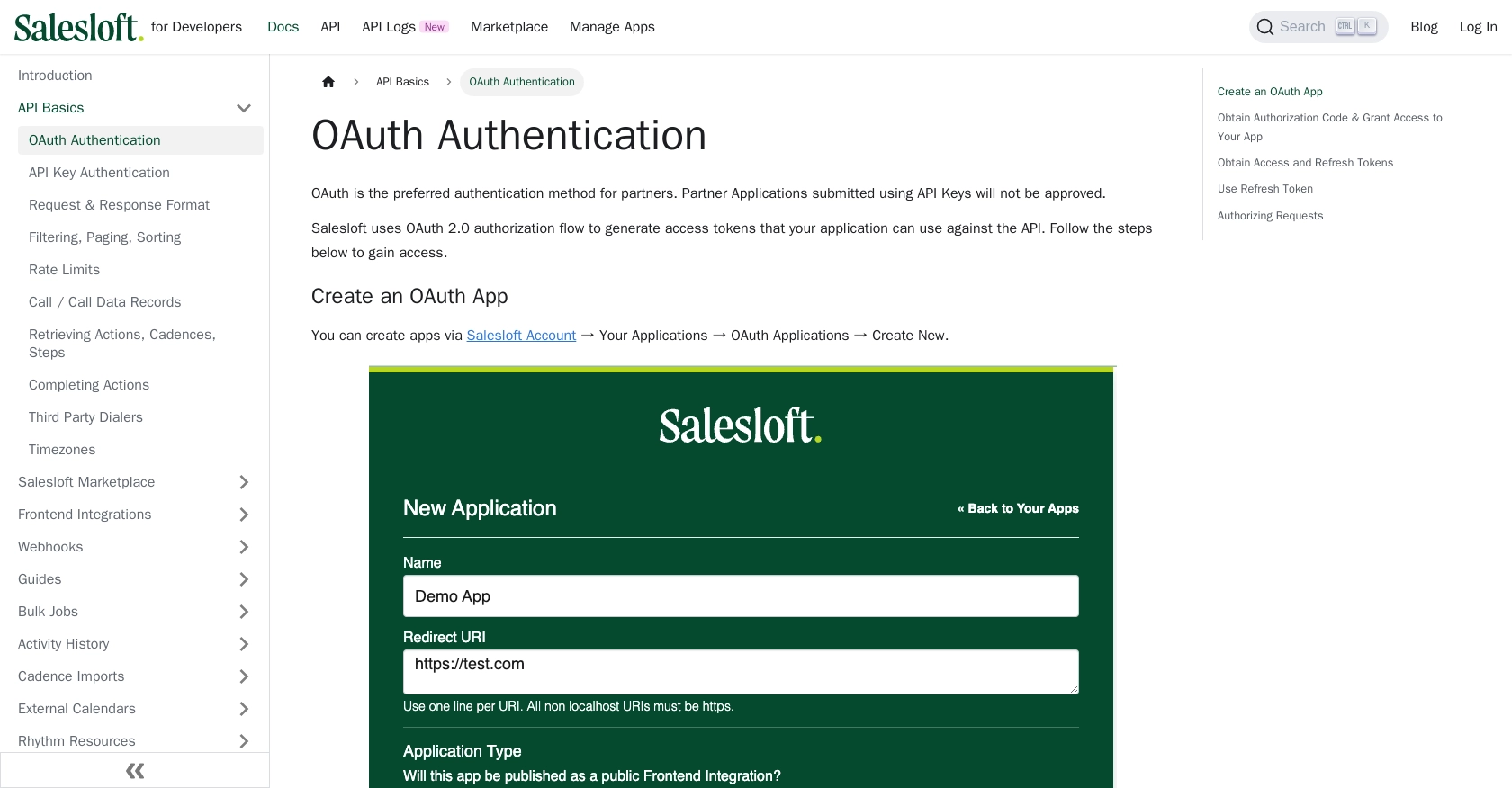
sbb-itb-96038d7
Making API Calls to Retrieve Salesloft Call Data Using PHP
To interact with the Salesloft API and retrieve call data, you'll need to use PHP to make HTTP requests. This section will guide you through setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Salesloft API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer for dependency management
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install Guzzle:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Salesloft Call Records
Create a new PHP file named get_salesloft_calls.php
and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://api.salesloft.com/v2/activities/calls';
$headers = [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Accept' => 'application/json'
];
// Make a GET request to the API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Parse the JSON data from the response
$data = json_decode($response->getBody(), true);
// Loop through the call records and print their information
foreach ($data['data'] as $call) {
echo 'Call ID: ' . $call['id'] . "\n";
echo 'To: ' . $call['to'] . "\n";
echo 'Duration: ' . $call['duration'] . " seconds\n";
echo 'Sentiment: ' . $call['sentiment'] . "\n";
echo 'Disposition: ' . $call['disposition'] . "\n";
echo '---' . "\n";
}
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication setup.
Running the PHP Script and Verifying the Output
Execute the script from the command line using the following command:
php get_salesloft_calls.php
You should see the call records printed in the console, including details such as call ID, recipient, duration, sentiment, and disposition.
Handling Errors and Validating API Responses
It's essential to handle potential errors when making API calls. Check the response status code to ensure the request was successful. Salesloft API uses standard HTTP status codes to indicate success or failure:
- 200 - Success
- 403 - Forbidden
- 404 - Not Found
- 422 - Unprocessable Entity
Modify the code to include error handling:
try {
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
if ($response->getStatusCode() === 200) {
$data = json_decode($response->getBody(), true);
// Process data
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
For more details on error codes, refer to the Salesloft Request & Response Format documentation.
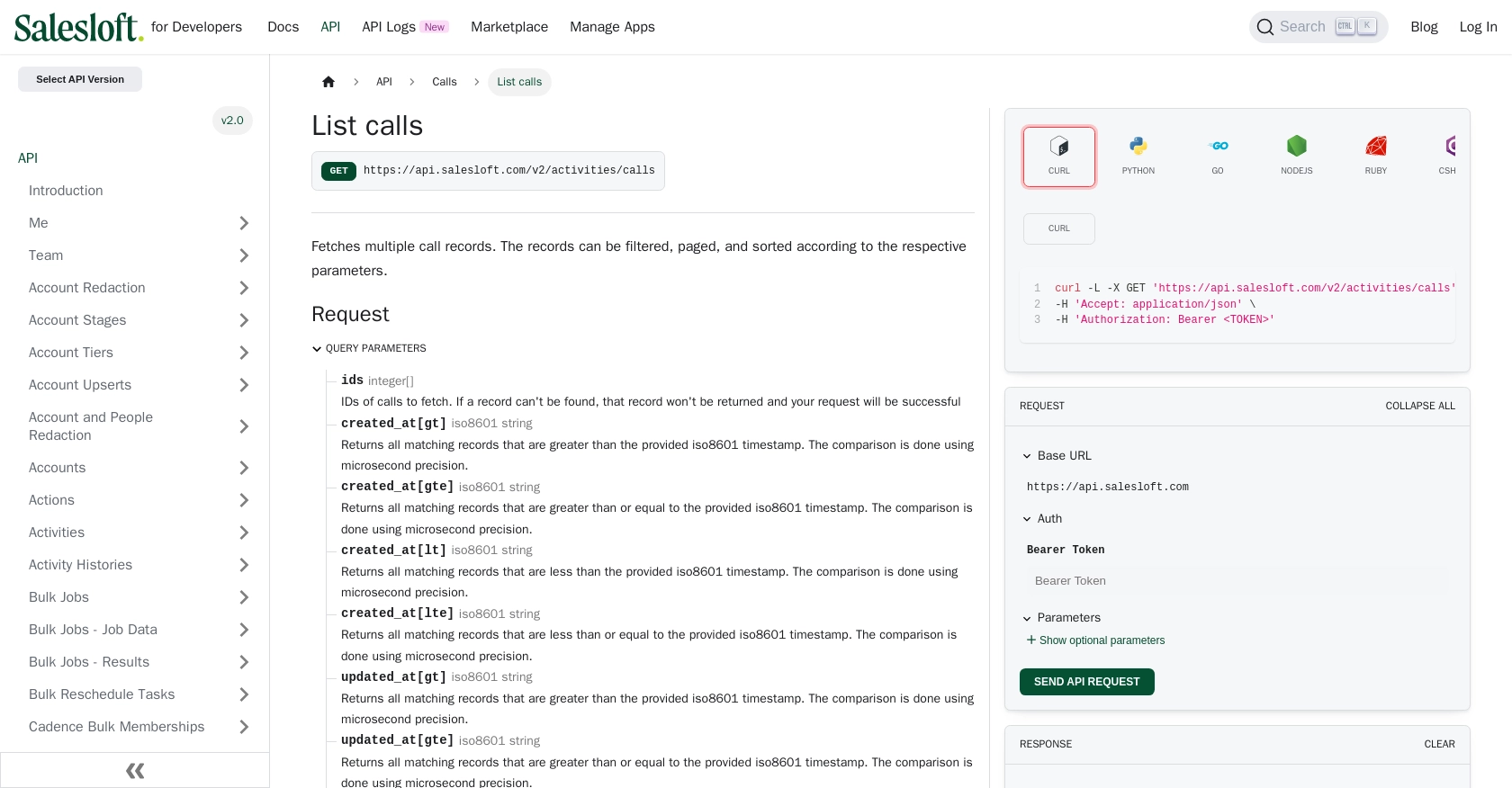
Conclusion and Best Practices for Salesloft API Integration Using PHP
Integrating with the Salesloft API using PHP provides a powerful way to access and manage call data, enabling sales teams to optimize their engagement strategies. By following the steps outlined in this guide, developers can efficiently retrieve call records and handle API responses.
Best Practices for Secure and Efficient Salesloft API Usage
- Secure Token Storage: Always store your access and refresh tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Salesloft's rate limits, which are set at 600 cost per minute. Implement logic to handle rate limit responses and consider using exponential backoff strategies for retries. For more details, refer to the Salesloft Rate Limits documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application and other integrated systems.
Enhancing Integration Capabilities with Endgrate
While integrating with Salesloft directly can be effective, leveraging a tool like Endgrate can streamline the process further. Endgrate offers a unified API endpoint that connects to multiple platforms, including Salesloft, allowing developers to build integrations once and apply them across various services.
By using Endgrate, you can save time and resources, focus on your core product, and provide an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/activities-calls-index/
Ready to get started?