Using the FreshService API to Create or Update Departments (with Javascript examples)
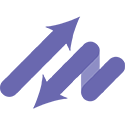
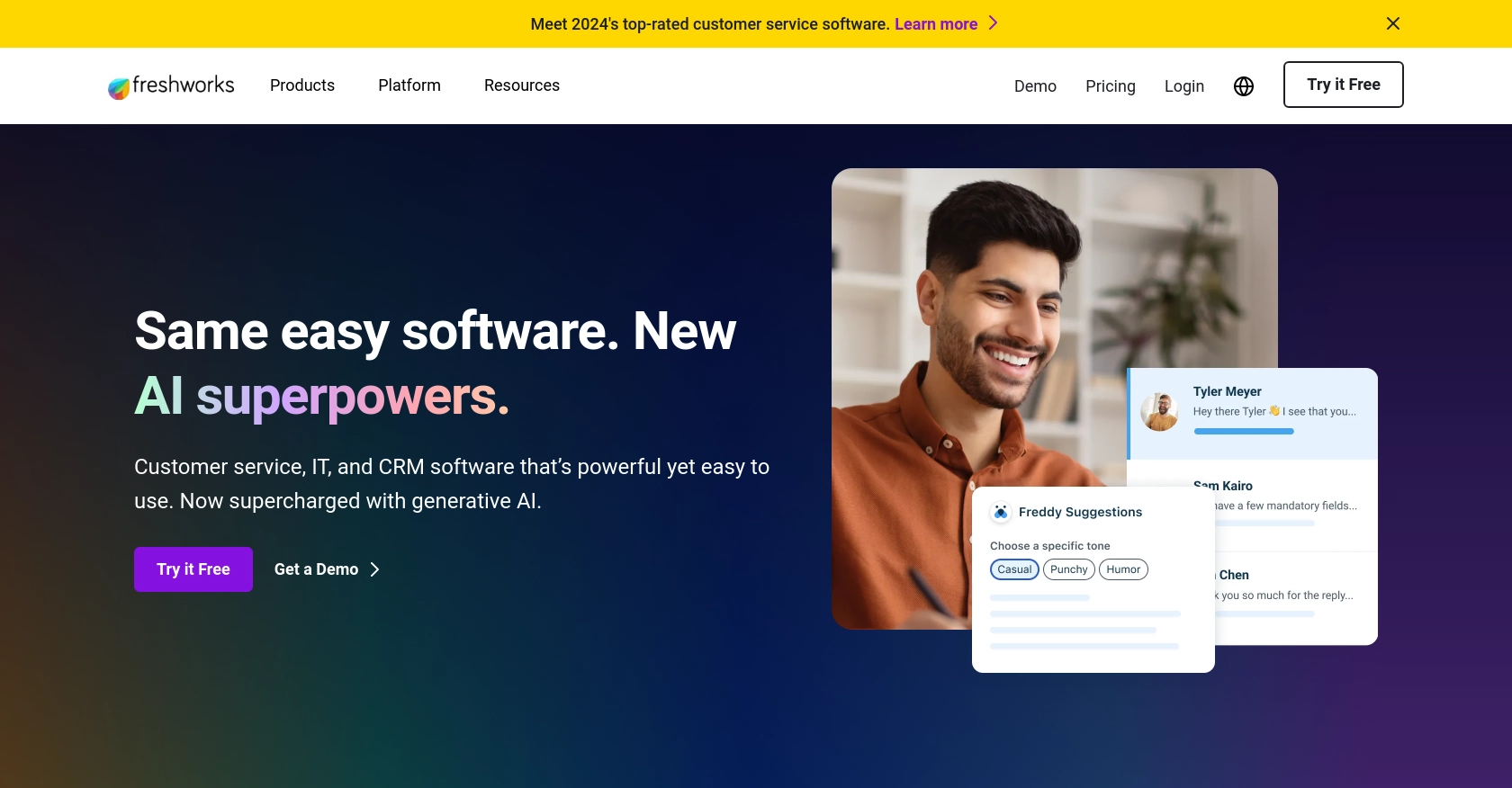
Introduction to FreshService API
FreshService is a robust IT service management (ITSM) solution designed to streamline IT operations for businesses of all sizes. It offers a comprehensive suite of tools for incident management, asset management, change management, and more, making it a popular choice for organizations looking to enhance their IT service delivery.
Integrating with the FreshService API allows developers to automate and optimize various IT processes. For example, you can use the API to create or update department records, ensuring that your organization's departmental information is always up-to-date and accurately reflected in your ITSM system.
This article will guide you through using JavaScript to interact with the FreshService API, focusing on creating or updating departments. By following this tutorial, you'll learn how to efficiently manage department data within FreshService, enhancing your organization's IT service management capabilities.
Setting Up Your FreshService Test or Sandbox Account
Before you can start interacting with the FreshService API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. FreshService provides a free trial that you can use to explore its features and test your integrations.
Creating a FreshService Free Trial Account
To get started, visit the FreshService signup page and register for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is created, you'll have access to the FreshService dashboard where you can manage your IT operations.
Generating API Key for FreshService Authentication
FreshService uses a custom authentication method that requires an API key. Follow these steps to generate your API key:
- Log in to your FreshService account.
- Navigate to your profile icon in the top-right corner and select Profile Settings.
- Under the API Key section, you'll find your unique API key. Copy this key and store it securely, as you'll need it to authenticate your API requests.
Configuring Your FreshService App for API Access
To interact with the FreshService API, you may need to configure your app settings to ensure proper access and permissions:
- Go to the Admin section in the FreshService dashboard.
- Select Apps from the sidebar menu.
- Ensure that the necessary permissions are enabled for your app to create or update departments.
With your FreshService account set up and your API key ready, you're now prepared to start making API calls to create or update departments using JavaScript. In the next section, we'll walk through the process of making these API calls with detailed code examples.
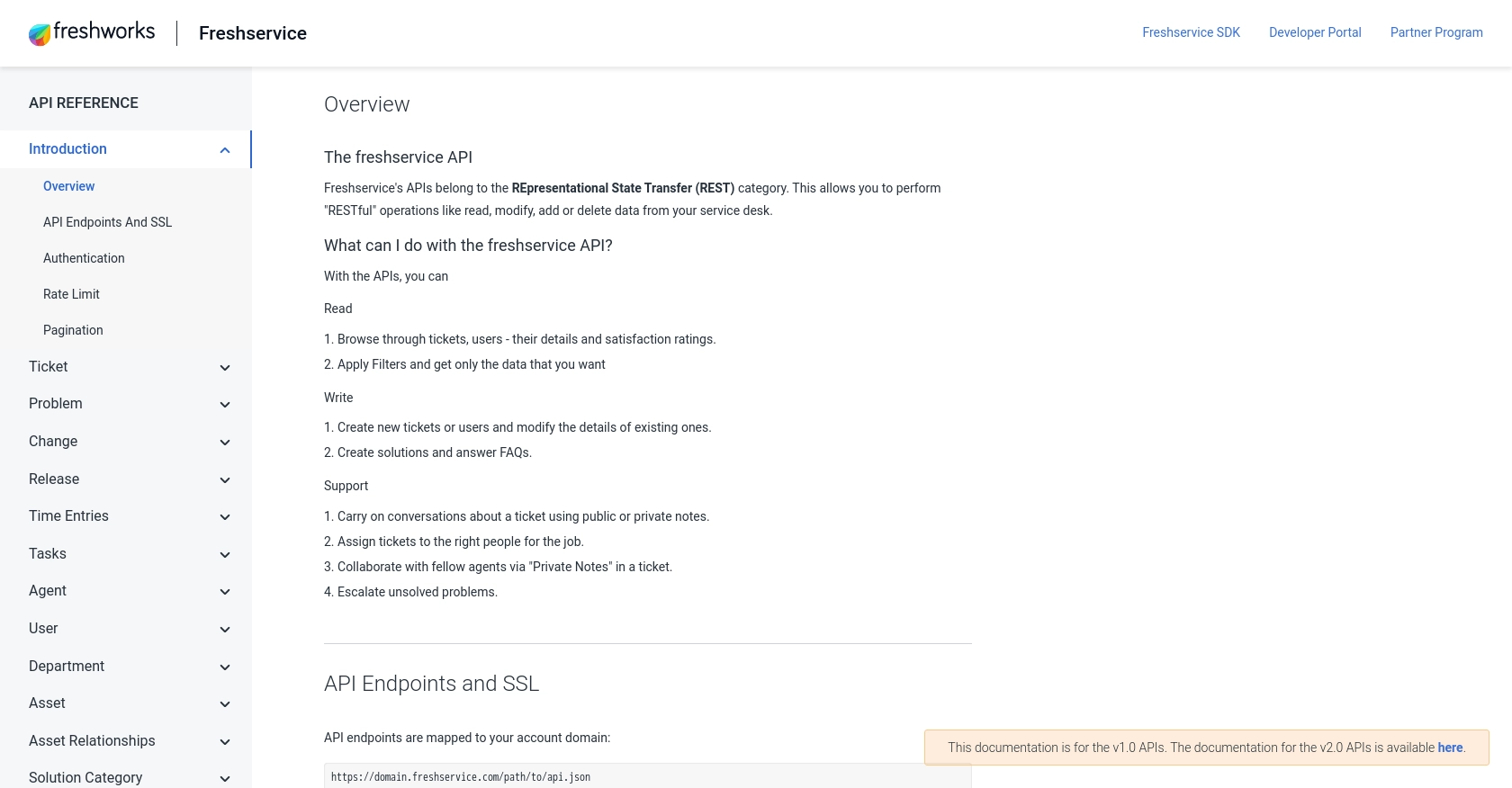
sbb-itb-96038d7
Making API Calls to FreshService with JavaScript
To interact with the FreshService API using JavaScript, you'll need to set up your environment and write code that can send HTTP requests to the API endpoints. This section will guide you through the process of creating or updating departments in FreshService using JavaScript.
Setting Up Your JavaScript Environment
Before you begin, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side scripting and API interactions.
- Download and install Node.js from the official website.
- Once installed, open your terminal or command prompt and verify the installation by running
node -v
andnpm -v
. - Create a new directory for your project and navigate into it using
cd your_project_directory
. - Initialize a new Node.js project by running
npm init -y
. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Creating or Updating Departments with FreshService API
With your environment set up, you can now write JavaScript code to interact with the FreshService API. Below are examples of how to create or update departments.
Example: Creating a Department
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://yourdomain.freshservice.com/api/v2/departments';
const apiKey = 'Your_API_Key';
const headers = {
'Content-Type': 'application/json',
'Authorization': `Basic ${Buffer.from(apiKey + ':X').toString('base64')}`
};
// Define the department data
const departmentData = {
department: {
name: 'New Department',
description: 'Description of the new department'
}
};
// Make a POST request to create a department
axios.post(endpoint, departmentData, { headers })
.then(response => {
console.log('Department Created:', response.data);
})
.catch(error => {
console.error('Error Creating Department:', error.response.data);
});
Replace Your_API_Key
with your actual FreshService API key. This code sends a POST request to the FreshService API to create a new department. If successful, it logs the created department's details.
Example: Updating a Department
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://yourdomain.freshservice.com/api/v2/departments/department_id';
const apiKey = 'Your_API_Key';
const headers = {
'Content-Type': 'application/json',
'Authorization': `Basic ${Buffer.from(apiKey + ':X').toString('base64')}`
};
// Define the updated department data
const updatedDepartmentData = {
department: {
name: 'Updated Department Name',
description: 'Updated description of the department'
}
};
// Make a PUT request to update the department
axios.put(endpoint, updatedDepartmentData, { headers })
.then(response => {
console.log('Department Updated:', response.data);
})
.catch(error => {
console.error('Error Updating Department:', error.response.data);
});
In this example, replace department_id
with the ID of the department you wish to update. This code sends a PUT request to update the department's information.
Verifying API Requests in FreshService
After executing the API calls, you can verify the changes by logging into your FreshService account and checking the departments section. Any new or updated departments should be reflected there.
Handling Errors and Troubleshooting
When making API calls, it's crucial to handle potential errors. The examples above include basic error handling that logs the error response. Ensure you check the error messages for any issues related to authentication, permissions, or data validation.
Conclusion: Best Practices for Using FreshService API with JavaScript
Integrating with the FreshService API using JavaScript can significantly enhance your IT service management capabilities by automating the creation and updating of department records. To ensure a smooth and efficient integration process, consider the following best practices:
Securely Storing FreshService API Credentials
Always store your FreshService API key securely. Avoid hardcoding it directly into your codebase. Instead, use environment variables or secure vaults to manage sensitive information. This practice helps prevent unauthorized access and protects your organization's data.
Handling FreshService API Rate Limits
Be mindful of FreshService API rate limits to avoid throttling. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay. This ensures your application remains responsive and compliant with FreshService's usage policies.
Standardizing and Transforming Data for FreshService
When interacting with the FreshService API, ensure that your data is standardized and properly formatted. This includes validating department names, descriptions, and other fields before sending requests. Consistent data formatting helps maintain data integrity within your ITSM system.
Leveraging Endgrate for Streamlined Integrations
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including FreshService, allowing you to focus on your core product while outsourcing integration complexities.
By following these best practices, you can effectively manage department data within FreshService, enhancing your organization's IT service management capabilities. For more information on how Endgrate can assist with your integration needs, visit Endgrate.
Read More
Ready to get started?