How to Get Records with the Snowflake API in PHP
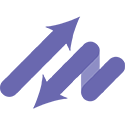
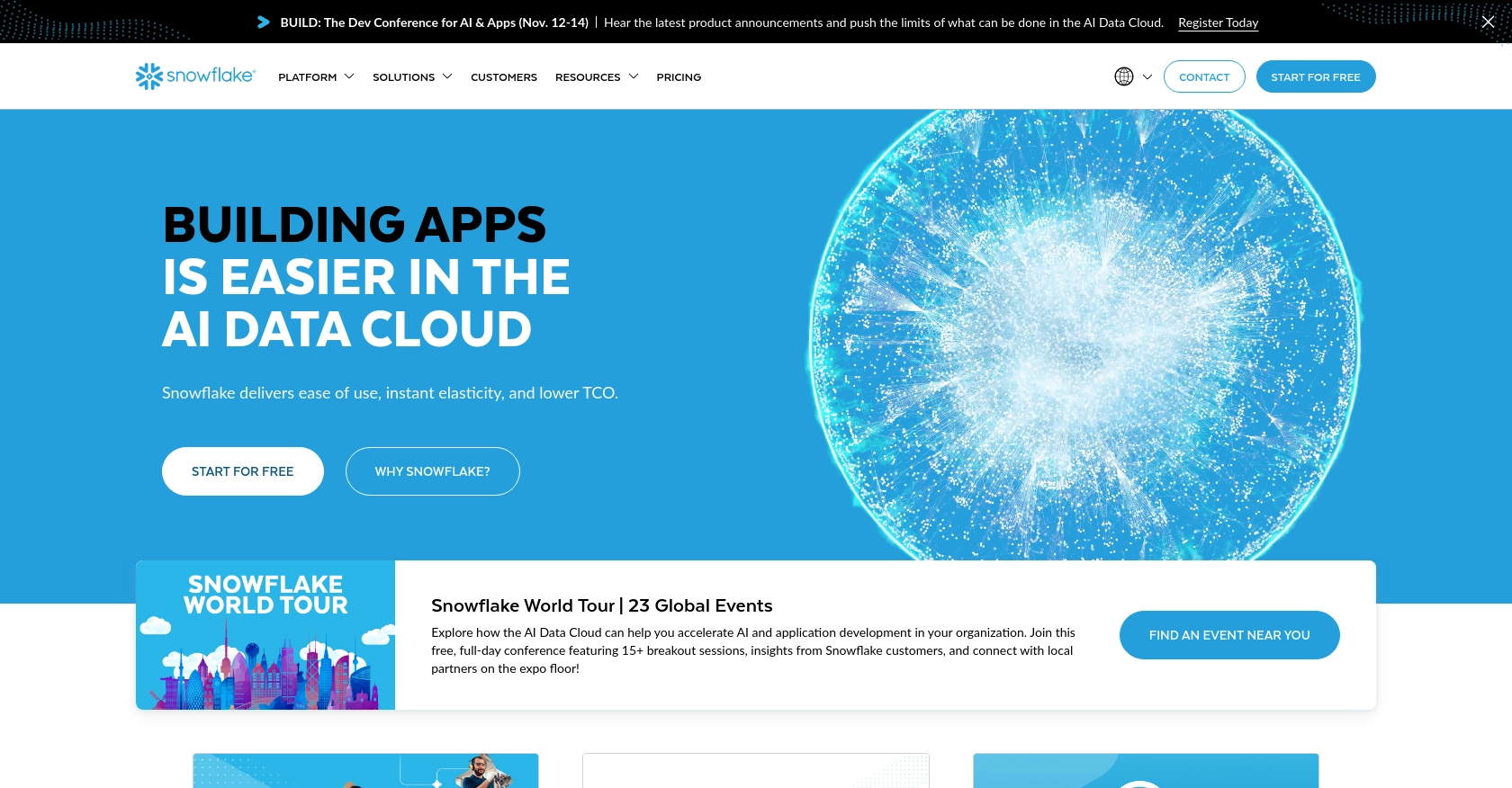
Introduction to Snowflake API Integration
Snowflake is a cloud-based data warehousing platform renowned for its scalability, flexibility, and ease of use. It enables businesses to store and analyze vast amounts of data efficiently, making it a popular choice for data-driven organizations.
Integrating with Snowflake's API allows developers to interact with their data programmatically, facilitating tasks such as querying data, managing resources, and automating workflows. For example, a developer might use the Snowflake API to retrieve sales records and generate real-time analytics dashboards, enhancing decision-making processes.
Setting Up Your Snowflake Test Account for API Integration
Before you can start interacting with the Snowflake API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your production data.
Creating a Snowflake Account
If you don't already have a Snowflake account, you can sign up for a free trial on the Snowflake website. This trial provides access to a sandbox environment where you can test API interactions.
- Visit the Snowflake website and click on "Start for Free."
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Snowflake dashboard.
Setting Up OAuth for Snowflake API Authentication
Snowflake uses OAuth for secure API authentication. Follow these steps to set up OAuth and obtain the necessary credentials:
- Navigate to the "Admin" section in your Snowflake dashboard.
- Under "Security," select "OAuth" to create a new OAuth integration.
- Fill in the required details, such as the name and description of your integration.
- Once created, note down the client ID and client secret, as you'll need these for authentication.
Generating an OAuth Token
With your OAuth integration set up, you can now generate an OAuth token to authenticate your API requests:
// Use the client ID and client secret to request an OAuth token
$client_id = 'your_client_id';
$client_secret = 'your_client_secret';
$token_url = 'https://your_account.snowflakecomputing.com/oauth/token';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $token_url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query([
'grant_type' => 'client_credentials',
'client_id' => $client_id,
'client_secret' => $client_secret
]));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$token_data = json_decode($response, true);
$access_token = $token_data['access_token'];
Replace your_client_id
and your_client_secret
with the values you obtained earlier. This script will return an OAuth token that you can use in your API requests.
Testing Your Snowflake API Connection
To ensure your setup is correct, test the connection by making a simple API call using the generated OAuth token:
// Example API call to list databases
$api_url = 'https://your_account.snowflakecomputing.com/api/v2/statements/';
$headers = [
'Authorization: Bearer ' . $access_token,
'Content-Type: application/json'
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $api_url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
This code snippet demonstrates how to authenticate and make a basic API call to Snowflake. Replace your_account
with your actual Snowflake account identifier.
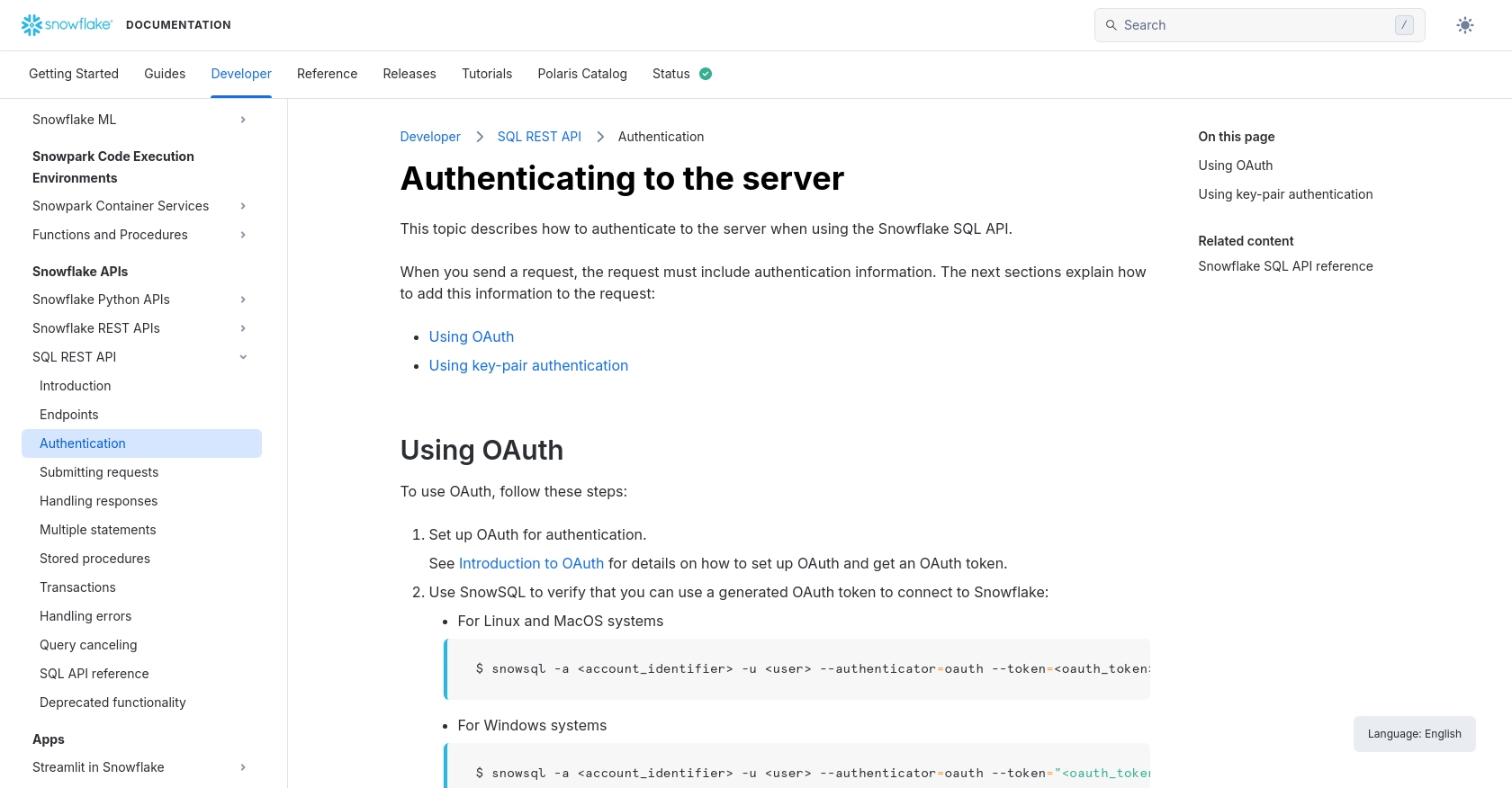
sbb-itb-96038d7
Executing Snowflake API Calls Using PHP
To interact with Snowflake's API using PHP, you need to ensure that your environment is set up correctly. This section will guide you through the process of making API calls to retrieve records from Snowflake, using PHP as the programming language.
Setting Up PHP Environment for Snowflake API
Before making API calls, ensure you have PHP installed on your machine. You can verify this by running php -v
in your terminal. Additionally, you need the cURL extension enabled, which is typically included in most PHP installations.
Installing Required PHP Dependencies
To make HTTP requests in PHP, we'll use the cURL library. Ensure that your PHP installation has cURL enabled. If not, you can enable it by uncommenting the line extension=curl
in your php.ini
file.
Making a Snowflake API Call to Retrieve Records
With your environment ready, you can now proceed to make an API call to Snowflake. The following PHP script demonstrates how to retrieve records using the Snowflake API:
$api_url = 'https://your_account.snowflakecomputing.com/api/v2/statements/';
$headers = [
'Authorization: Bearer ' . $access_token,
'Content-Type: application/json'
];
// Define the SQL query to execute
$sql_query = json_encode([
'statement' => 'SELECT * FROM your_database.your_schema.your_table LIMIT 10'
]);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $api_url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $sql_query);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response, true);
print_r($data);
Replace your_account
, your_database
, your_schema
, and your_table
with your actual Snowflake account identifier and database details. This script sends a SQL query to Snowflake and prints the retrieved records.
Verifying API Call Success and Handling Errors
After executing the API call, check the response to ensure the request was successful. Snowflake returns HTTP status codes to indicate the result of the request:
- 200 OK: The request was successful, and the data is returned.
- 408 Request Timeout: The request took longer than the timeout period.
- 422 Unprocessable Entity: There was an error processing the request.
For more detailed error information, refer to the Snowflake error handling documentation.
By following these steps, you can successfully retrieve records from Snowflake using PHP, allowing you to integrate Snowflake's powerful data capabilities into your applications.
Conclusion and Best Practices for Snowflake API Integration in PHP
Integrating with the Snowflake API using PHP allows developers to harness the full potential of Snowflake's data warehousing capabilities. By following the steps outlined in this guide, you can efficiently retrieve and manage data, enabling seamless integration into your applications.
Best Practices for Secure and Efficient Snowflake API Usage
- Securely Store Credentials: Always store your OAuth credentials securely. Consider using environment variables or secure vaults to manage sensitive information like client IDs and secrets.
- Handle Rate Limiting: Be mindful of Snowflake's rate limits to avoid throttling. Implement exponential backoff strategies to manage retries gracefully.
- Standardize Data Fields: Ensure that data retrieved from Snowflake is transformed and standardized to fit your application's data models, enhancing consistency and reliability.
Streamlining Integrations with Endgrate
While integrating with Snowflake's API directly can be powerful, it may not always be the most efficient approach, especially if you need to manage multiple integrations. Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Snowflake.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?