Using the Mode API to Get Users (with Javascript examples)
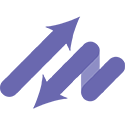
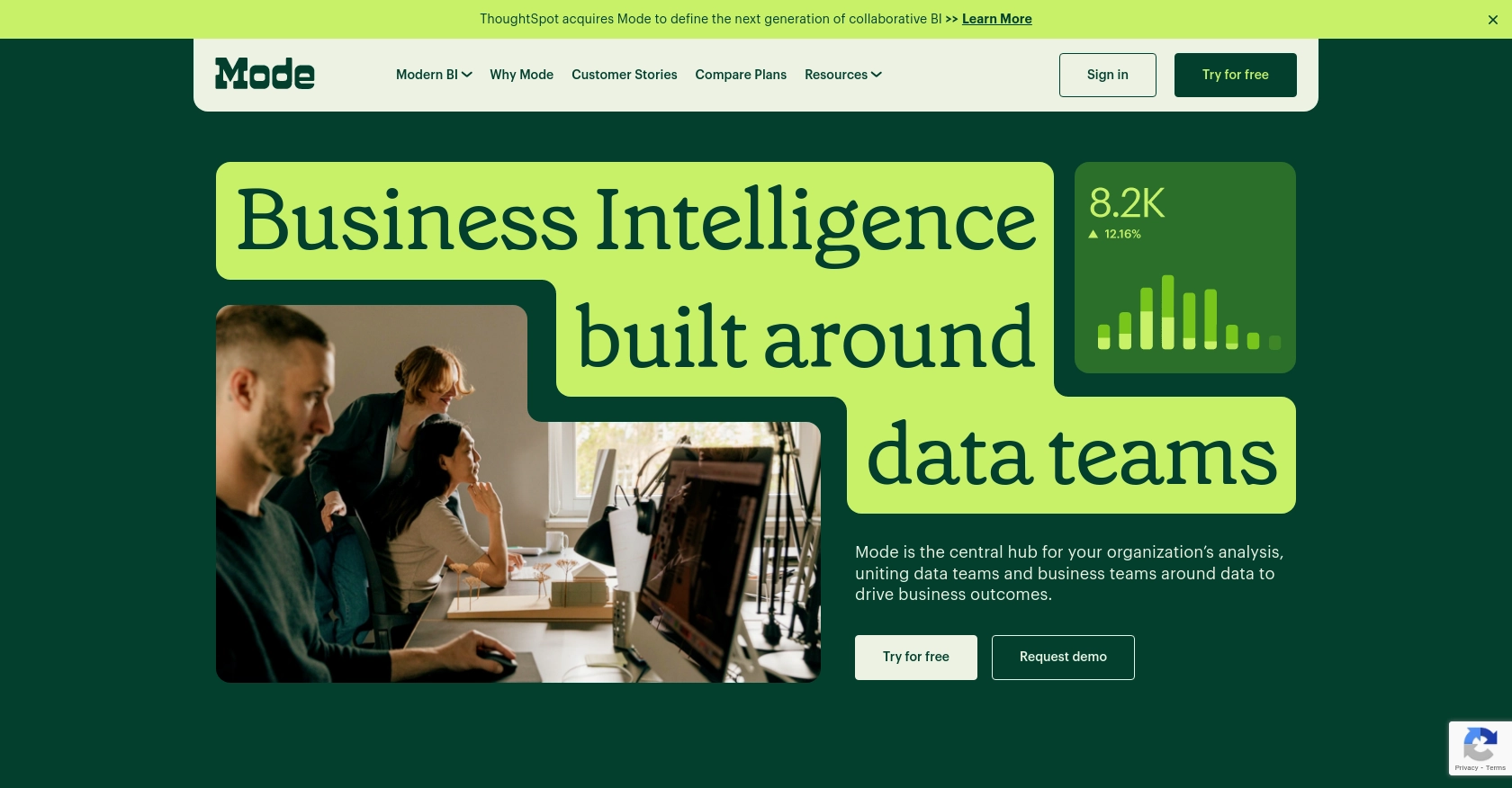
Introduction to Mode API for User Management
Mode is a collaborative analytics platform that empowers teams to make data-driven decisions through its robust suite of tools. By leveraging Mode's API, developers can programmatically access and manage various analytics functions, including user management within a Mode Workspace.
Integrating with the Mode API allows developers to efficiently manage user data and workspace memberships. For example, you might want to retrieve a list of users in a workspace to synchronize with an internal directory or automate user onboarding processes.
This guide will walk you through using JavaScript to interact with the Mode API, specifically focusing on retrieving user information from a Mode Workspace.
Setting Up Your Mode API Test Account
Before you can start interacting with the Mode API, you'll need to set up a Mode account and configure it for API access. This involves creating a Mode Workspace and obtaining the necessary API credentials for authentication.
Step 1: Create a Mode Account and Workspace
- Visit the Mode website and sign up for an account if you haven't already.
- Once registered, create a new Workspace. This Workspace will be the environment where you'll manage users and other resources.
- Connect a database to your Workspace or use available public data to start exploring Mode's capabilities.
Step 2: Generate Mode API Access Token
To interact with the Mode API, you'll need an API access token. Follow these steps to create one:
- Navigate to your Workspace Settings.
- Go to Privacy & Security and select API.
- Click the gear icon and choose Create new API token.
- Add a display name for your token and save it. Remember to securely store the token and secret, as they will only be shown once.
For more details on generating and managing API tokens, refer to the Mode API Authentication documentation.
Step 3: Test Your API Access
With your API token ready, you can test your access by making a simple API call. Here's a JavaScript example using the request
library:
const request = require('request');
const options = {
method: 'GET',
url: 'https://app.mode.com/api/your_workspace/memberships',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/hal+json',
'Authorization': 'Basic ' + Buffer.from('your_api_token:your_api_secret').toString('base64')
}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log('Status:', response.statusCode);
console.log('Response:', body);
});
Replace your_workspace
, your_api_token
, and your_api_secret
with your actual Workspace name and API credentials. This code will retrieve a list of memberships in your Workspace, confirming that your API setup is correct.
For more information on making API calls, visit the Mode API Workspace Memberships documentation.
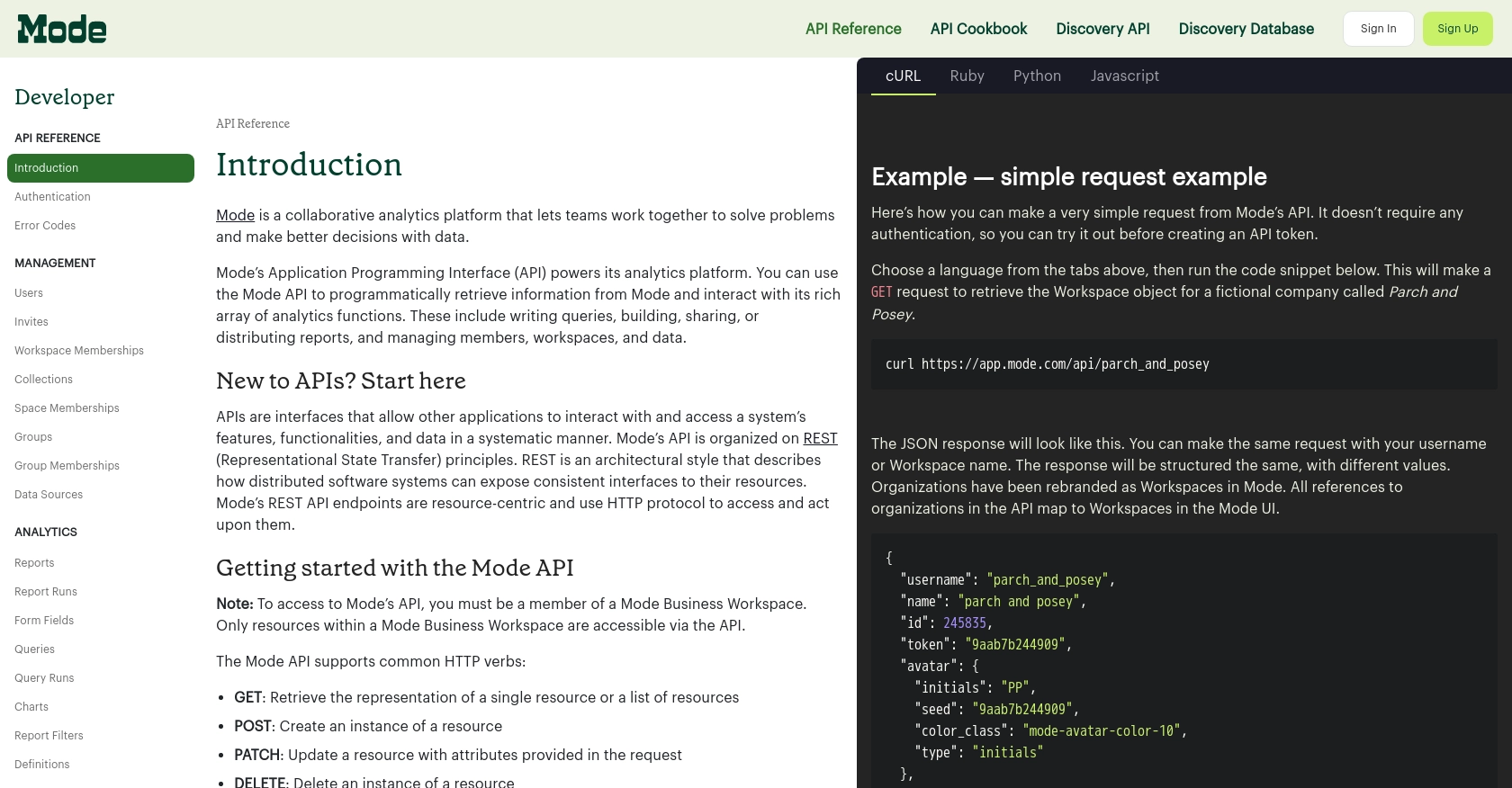
sbb-itb-96038d7
Making API Calls to Retrieve Users from Mode Workspace Using JavaScript
To effectively interact with the Mode API and retrieve user information, you'll need to use JavaScript to make API calls. This section will guide you through the process, ensuring you have the right setup and understanding to execute these calls successfully.
Prerequisites for JavaScript API Integration with Mode
Before diving into the code, ensure you have the following prerequisites:
- Node.js installed on your machine.
- The
request
library, which can be installed via npm using the command:npm install request
. - Your Mode API token and secret, securely stored from the setup process.
Executing a GET Request to Retrieve Users
To retrieve a list of users from your Mode Workspace, you'll need to make a GET request to the appropriate API endpoint. Here's how you can do it using JavaScript:
const request = require('request');
const options = {
method: 'GET',
url: 'https://app.mode.com/api/your_workspace/memberships',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/hal+json',
'Authorization': 'Basic ' + Buffer.from('your_api_token:your_api_secret').toString('base64')
}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log('Status:', response.statusCode);
console.log('Response:', body);
});
Replace your_workspace
, your_api_token
, and your_api_secret
with your actual Workspace name and API credentials. This script will fetch the list of users in your Workspace.
Understanding the API Response and Error Handling
Upon executing the API call, you should receive a response containing user data. If the request is successful, the response will include user details such as usernames and membership status.
It's crucial to handle potential errors gracefully. Common HTTP error codes you might encounter include:
- 400 Bad Request: The request was malformed or contained invalid parameters.
- 401 Unauthorized: Authentication failed due to incorrect credentials.
- 403 Forbidden: The request was valid, but you don't have permission to access the resource.
- 404 Not Found: The requested resource could not be found.
For more details on error codes, refer to the Mode API Error Codes documentation.
Verifying Successful API Requests in Mode
After making the API call, verify the results by checking the returned data against your Mode Workspace. Ensure that the user information matches the data in your Workspace.
By following these steps, you can efficiently retrieve and manage user data from Mode using JavaScript, streamlining your integration processes.
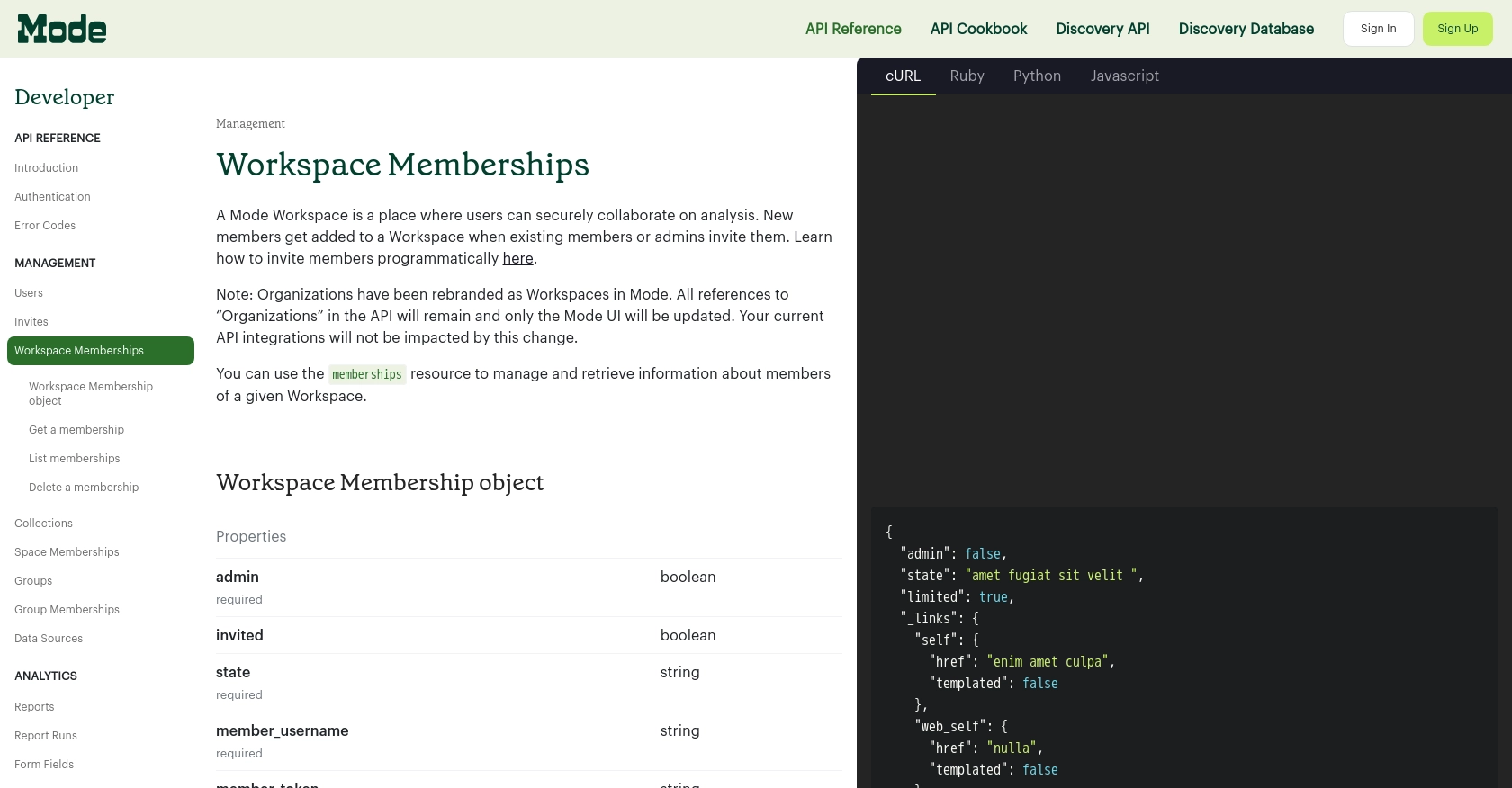
Conclusion and Best Practices for Using Mode API with JavaScript
Integrating with the Mode API using JavaScript provides a powerful way to manage user data and workspace memberships efficiently. By following the steps outlined in this guide, you can seamlessly retrieve user information and automate various processes within your Mode Workspace.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API tokens and secrets securely. Avoid exposing them in client-side code or public repositories.
- Handle Rate Limiting: Be mindful of API rate limits to prevent service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application’s requirements.
- Error Handling: Implement robust error handling to manage different HTTP response codes effectively, ensuring a smooth user experience.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Mode. By using Endgrate, you can save time and resources, allowing you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?