Using the Xero API to Get Tax Rates in Javascript
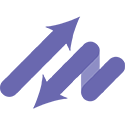
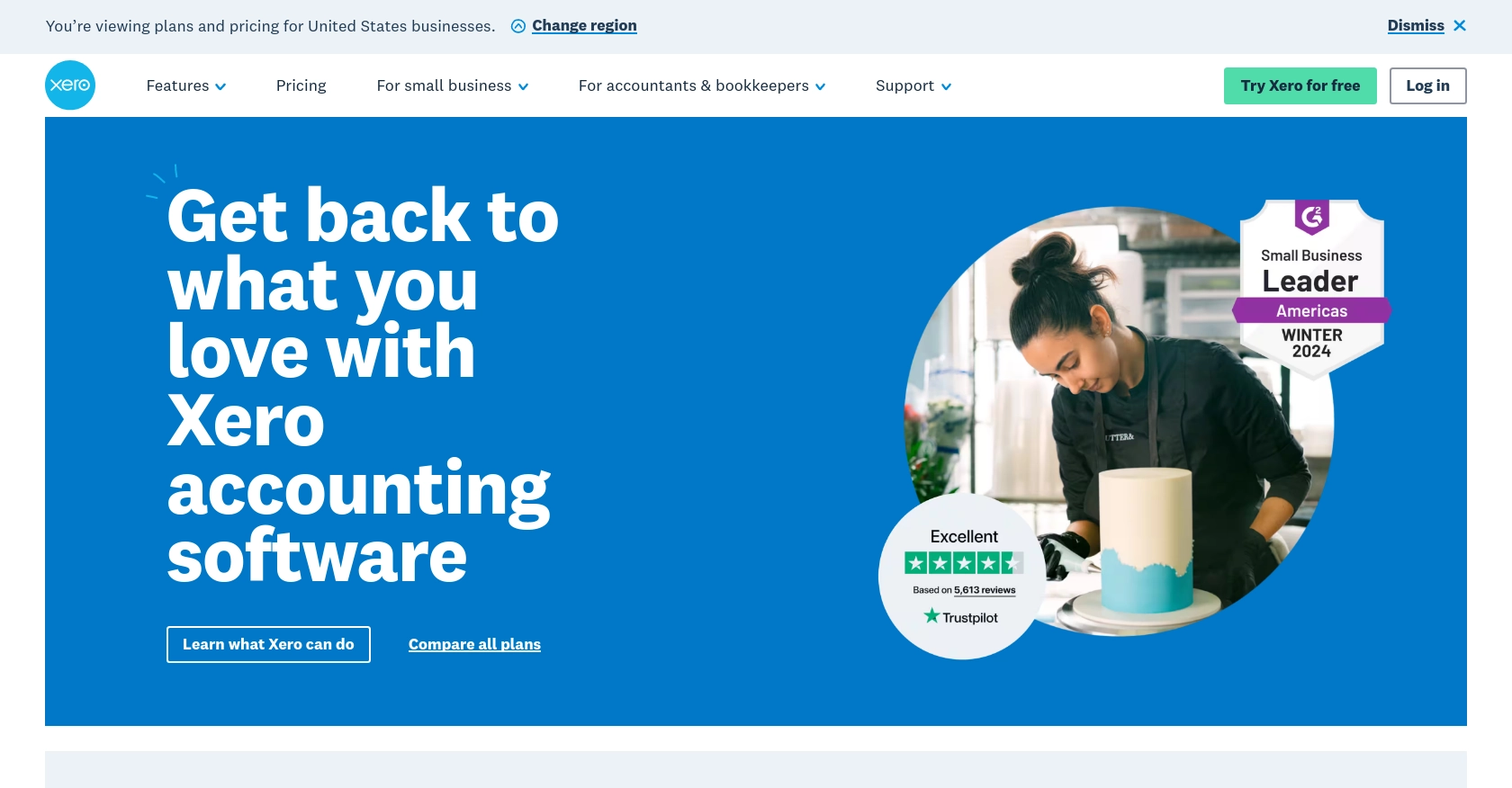
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help businesses manage their finances efficiently. With features like invoicing, payroll, and expense tracking, Xero is a popular choice for small to medium-sized enterprises looking to streamline their financial operations.
Integrating with Xero's API allows developers to access and manipulate financial data programmatically, enabling automation and enhanced functionality. For example, a developer might use the Xero API to retrieve tax rates, which can be crucial for applications that need to calculate taxes accurately for various jurisdictions.
This article will guide you through using JavaScript to interact with the Xero API, specifically focusing on retrieving tax rates. By following this tutorial, you'll learn how to set up your environment, authenticate with Xero, and make API calls to access tax rate information.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with the Xero API using JavaScript, you'll need to set up a Xero sandbox account. This allows you to test your integration without affecting live data. Xero provides a free trial or demo account that developers can use for testing purposes.
Creating a Xero Sandbox Account
To begin, visit the Xero sign-up page and create a new account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you can log in to the Xero dashboard.
Setting Up OAuth 2.0 Authentication for Xero API
Xero uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to set up OAuth 2.0:
- Navigate to the Xero Developer Portal and log in with your Xero account.
- Click on "New App" to create a new application.
- Fill in the required details, such as the app name and company URL.
- Under the "OAuth 2.0 Credentials" section, note down the Client ID and Client Secret. These will be used to authenticate your API requests.
- Set the redirect URI to a valid URL where Xero can send the authorization code. This is typically a URL on your server that will handle the OAuth callback.
Generating Access Tokens for Xero API
With your app created, you can now generate access tokens to authenticate API requests. Follow these steps:
- Use the Client ID and Client Secret to request an authorization code from Xero. This involves redirecting the user to Xero's authorization URL.
- Once the user authorizes the app, Xero will redirect them back to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to Xero's token endpoint.
- Store the access token securely, as it will be used to authenticate your API requests.
For more detailed information on OAuth 2.0 authentication with Xero, refer to the Xero OAuth 2.0 documentation.
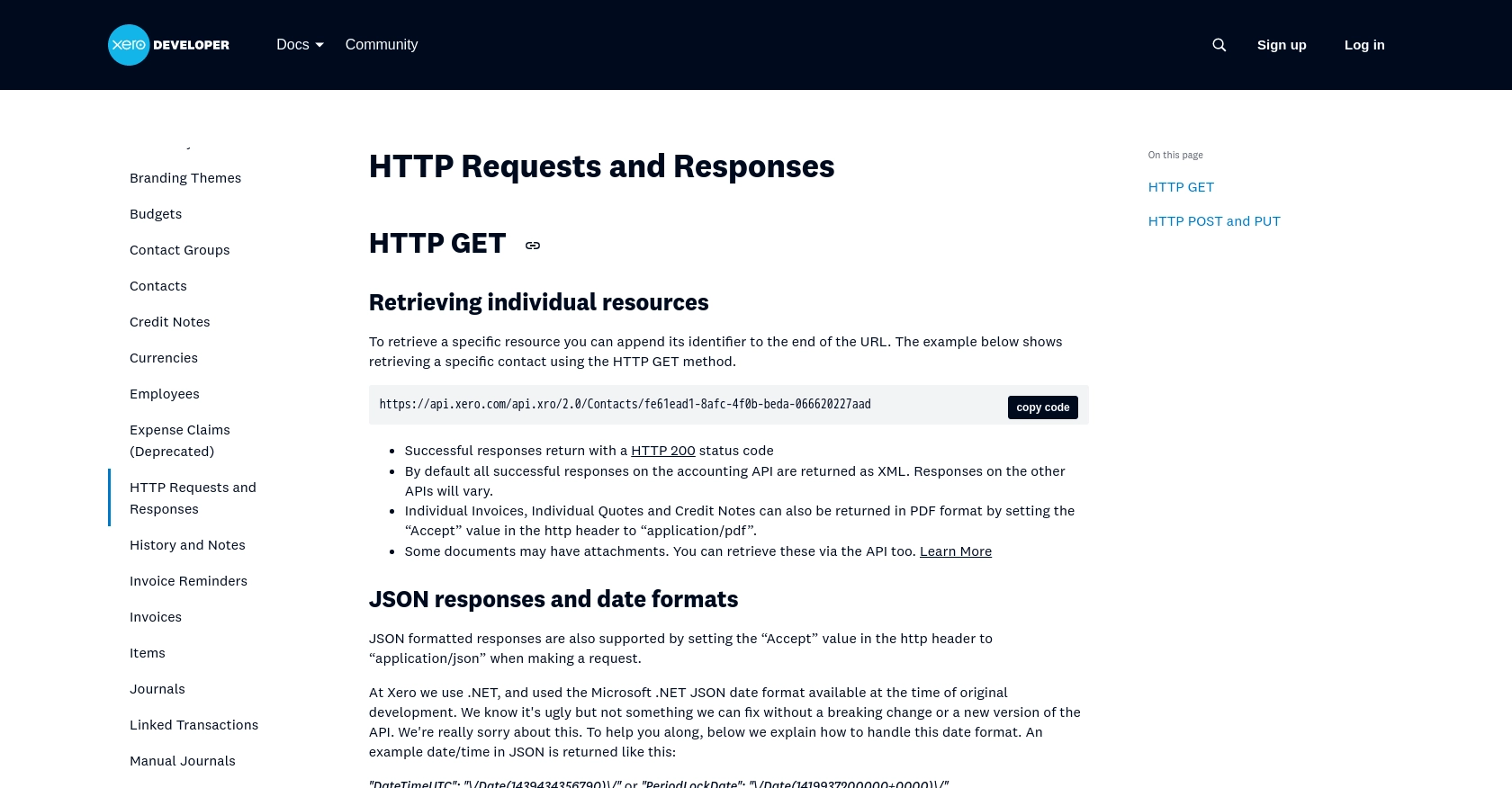
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates from Xero Using JavaScript
With your Xero sandbox account and OAuth 2.0 authentication set up, you are now ready to make API calls to retrieve tax rates using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Xero API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side applications.
- Download and install Node.js from the official website.
- Create a new directory for your project and navigate into it using the terminal.
- Initialize a new Node.js project by running
npm init -y
to create apackage.json
file. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Fetch Tax Rates from Xero API
Now that your environment is set up, you can write the JavaScript code to interact with the Xero API and retrieve tax rates. Create a new file named getTaxRates.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.xero.com/api.xro/2.0/TaxRates';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to fetch tax rates
async function getTaxRates() {
try {
const response = await axios.get(endpoint, { headers });
const taxRates = response.data.TaxRates;
console.log('Tax Rates:', taxRates);
} catch (error) {
console.error('Error fetching tax rates:', error.response ? error.response.data : error.message);
}
}
// Call the function
getTaxRates();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth 2.0 setup. This code uses the axios
library to send a GET request to the Xero API endpoint for tax rates. If successful, it logs the tax rates to the console.
Verifying API Call Success and Handling Errors
After running the script, you should see the tax rates printed in the console. To verify the success of your API call, cross-check the returned data with the tax rates in your Xero sandbox account.
Handling errors is crucial for robust API integration. The code above includes error handling to log any issues that occur during the API call. Common errors might include invalid access tokens or network issues. For more detailed error information, refer to the Xero API documentation.
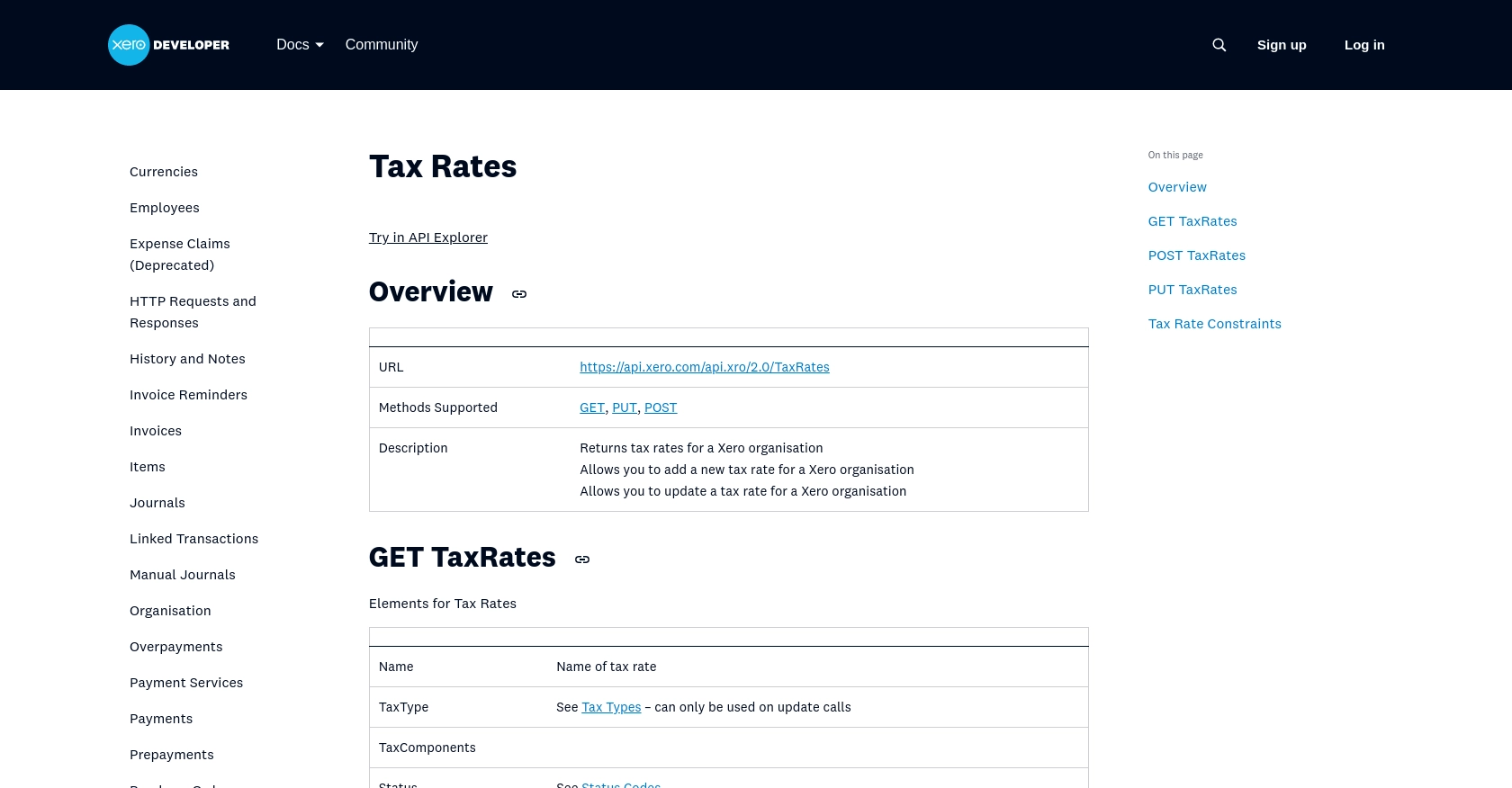
Conclusion: Best Practices for Xero API Integration in JavaScript
Integrating with the Xero API to retrieve tax rates using JavaScript can significantly enhance your application's financial capabilities. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate with Xero, and make API calls to access crucial tax information.
Here are some best practices to consider when working with the Xero API:
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be sure to implement logic to handle rate limit responses gracefully. For more information, refer to the Xero API rate limits documentation.
- Standardize Data: When retrieving tax rates, ensure that the data is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage potential issues such as expired tokens or network errors. This will ensure a seamless user experience.
By leveraging the Xero API, you can automate and streamline financial processes, providing a more efficient experience for your users. However, managing multiple integrations can be complex and time-consuming.
This is where Endgrate can assist. By using Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs and enhance your application's capabilities today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/taxrates
Ready to get started?