Using the Shopify API to Get Product Variants in Javascript
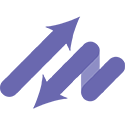
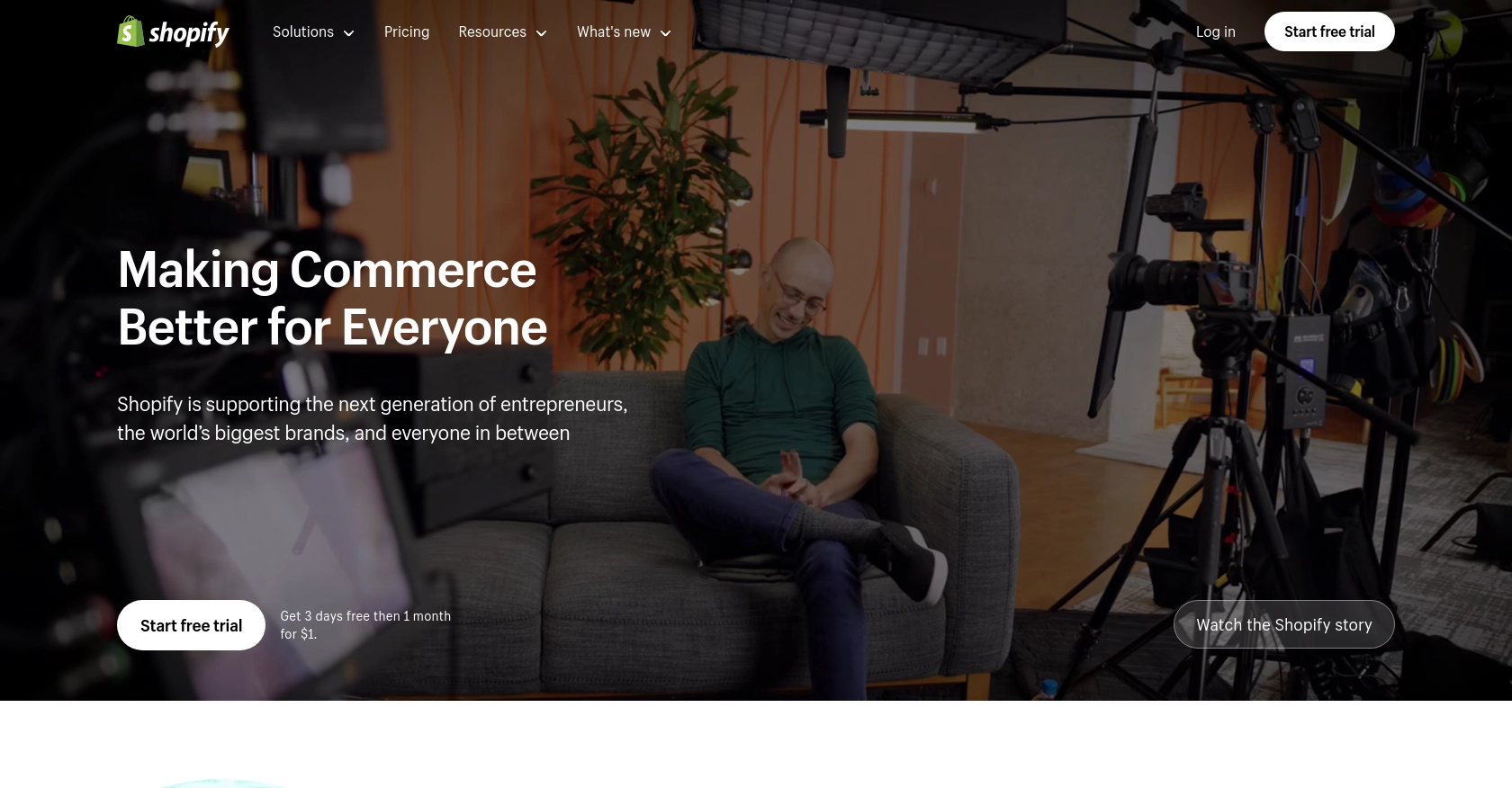
Introduction to Shopify API for Product Variants
Shopify is a leading e-commerce platform that empowers businesses to create and manage their online stores with ease. It offers a robust set of tools and APIs that allow developers to extend and customize the functionality of Shopify stores.
Integrating with Shopify's API enables developers to access and manipulate store data programmatically. One common use case is retrieving product variants, which are different versions of a product, such as size or color options. By accessing product variants through the Shopify API, developers can efficiently manage inventory, update product details, and enhance the shopping experience for customers.
This article will guide you through using JavaScript to interact with the Shopify API to retrieve product variants, providing a practical example of how to leverage this powerful tool in your e-commerce applications.
Setting Up Your Shopify Test Account for API Access
Before you can start interacting with the Shopify API to retrieve product variants, you'll need to set up a test account. Shopify provides developers with the ability to create a development store, which is ideal for testing and building applications without affecting a live store.
Creating a Shopify Development Store
- Visit the Shopify Partners page and sign up for a free account if you haven't already.
- Once logged in, navigate to the "Stores" section in your Shopify Partners dashboard.
- Click on "Add store" and select "Development store" as the store type.
- Fill in the required details, such as store name and password, and click "Save."
Your development store is now ready, and you can use it to test API interactions without any risk to a live environment.
Setting Up OAuth Authentication for Shopify API
Shopify uses OAuth 2.0 for authentication, which requires you to create a private app to obtain the necessary credentials.
- In your development store's admin panel, go to "Apps" and click on "Develop apps for your store."
- Click "Create an app" and provide a name for your app.
- Under "Configuration," select the necessary API scopes that your app will require. For retrieving product variants, ensure you have access to the "Products" scope.
- Click "Save" to generate your API credentials, including the API key and secret.
These credentials will be used to authenticate your API requests.
Generating Access Tokens
To make authenticated requests to the Shopify API, you'll need to exchange your API key and secret for an access token.
- Use the following URL format to initiate the OAuth flow:
https://{store_name}.myshopify.com/admin/oauth/authorize?client_id={api_key}&scope={scopes}&redirect_uri={redirect_uri}
. - After authorization, Shopify will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request to
https://{store_name}.myshopify.com/admin/oauth/access_token
with your API key, secret, and the code received.
Once you have the access token, include it in your API requests as a header: X-Shopify-Access-Token: {access_token}
.
For more detailed information on setting up OAuth, refer to the Shopify authentication documentation.
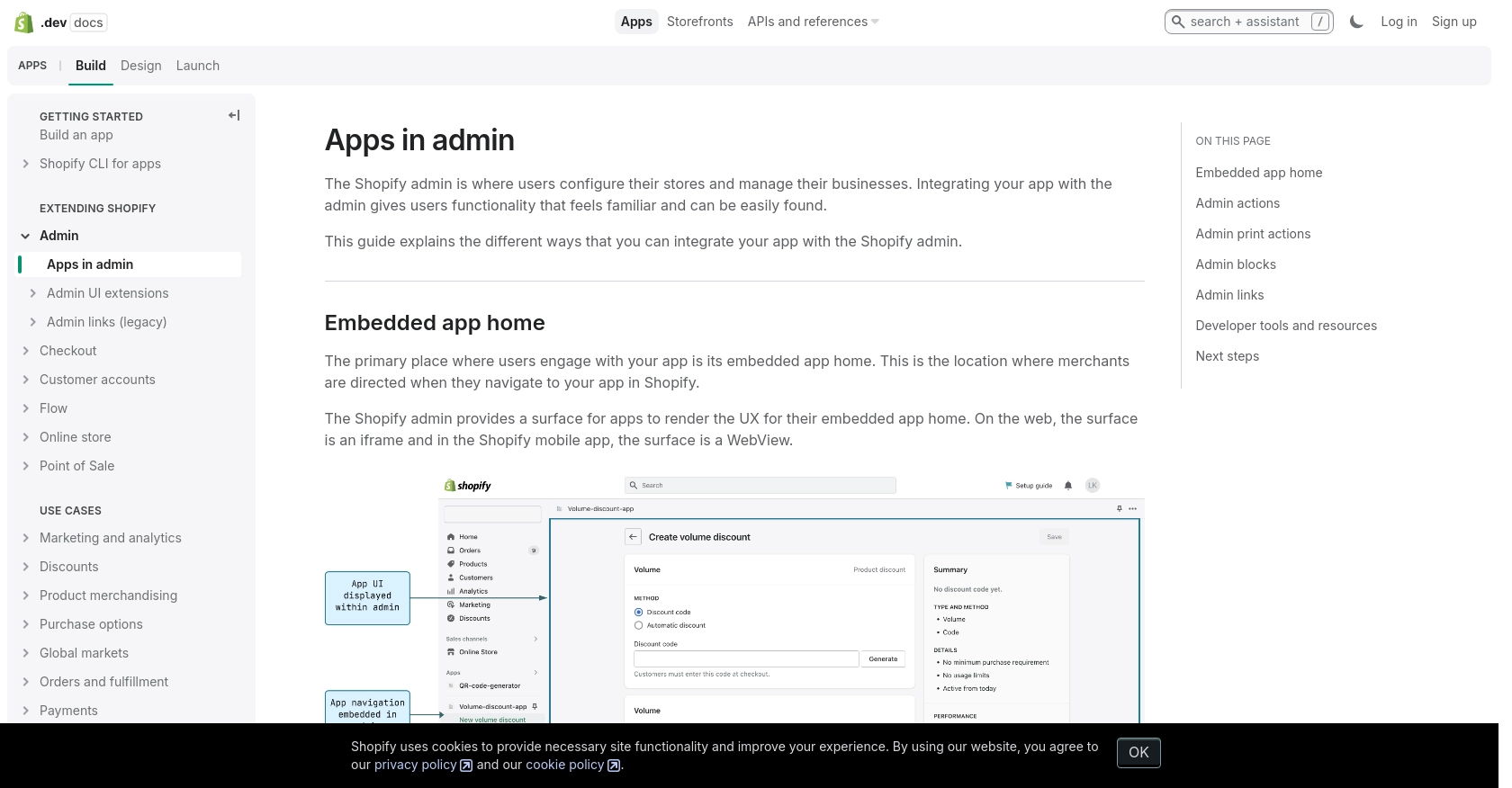
sbb-itb-96038d7
Making API Calls to Retrieve Shopify Product Variants Using JavaScript
To interact with the Shopify API and retrieve product variants, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Shopify API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside a browser.
- Download and install Node.js from the official website.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the
axios
library to simplify HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Retrieve Shopify Product Variants
With your environment set up, you can now write the JavaScript code to interact with the Shopify API and retrieve product variants.
const axios = require('axios');
// Define the API endpoint and headers
const storeName = 'your-development-store';
const productId = '632910392';
const accessToken = 'your_access_token';
const endpoint = `https://${storeName}.myshopify.com/admin/api/2024-07/products/${productId}/variants.json`;
const headers = {
'X-Shopify-Access-Token': accessToken,
'Content-Type': 'application/json'
};
// Function to get product variants
async function getProductVariants() {
try {
const response = await axios.get(endpoint, { headers });
const variants = response.data.variants;
console.log('Product Variants:', variants);
} catch (error) {
console.error('Error fetching product variants:', error.response.data);
}
}
// Call the function
getProductVariants();
Replace your-development-store
and your_access_token
with your actual store name and access token. This script uses the axios
library to send a GET request to the Shopify API endpoint for product variants. If successful, it logs the variants to the console.
Verifying API Call Success and Handling Errors
After running the script, you should see the product variants printed in the console. To verify the success of your API call, cross-check the output with the product variants listed in your Shopify development store.
In case of errors, the script will log the error details. Common error codes include:
- 401 Unauthorized: Check your access token and store name.
- 403 Forbidden: Ensure your app has the necessary permissions.
- 429 Too Many Requests: You have exceeded the rate limit. Refer to the Shopify rate limits documentation for more information.
For a complete list of error codes, refer to the Shopify status and error codes documentation.
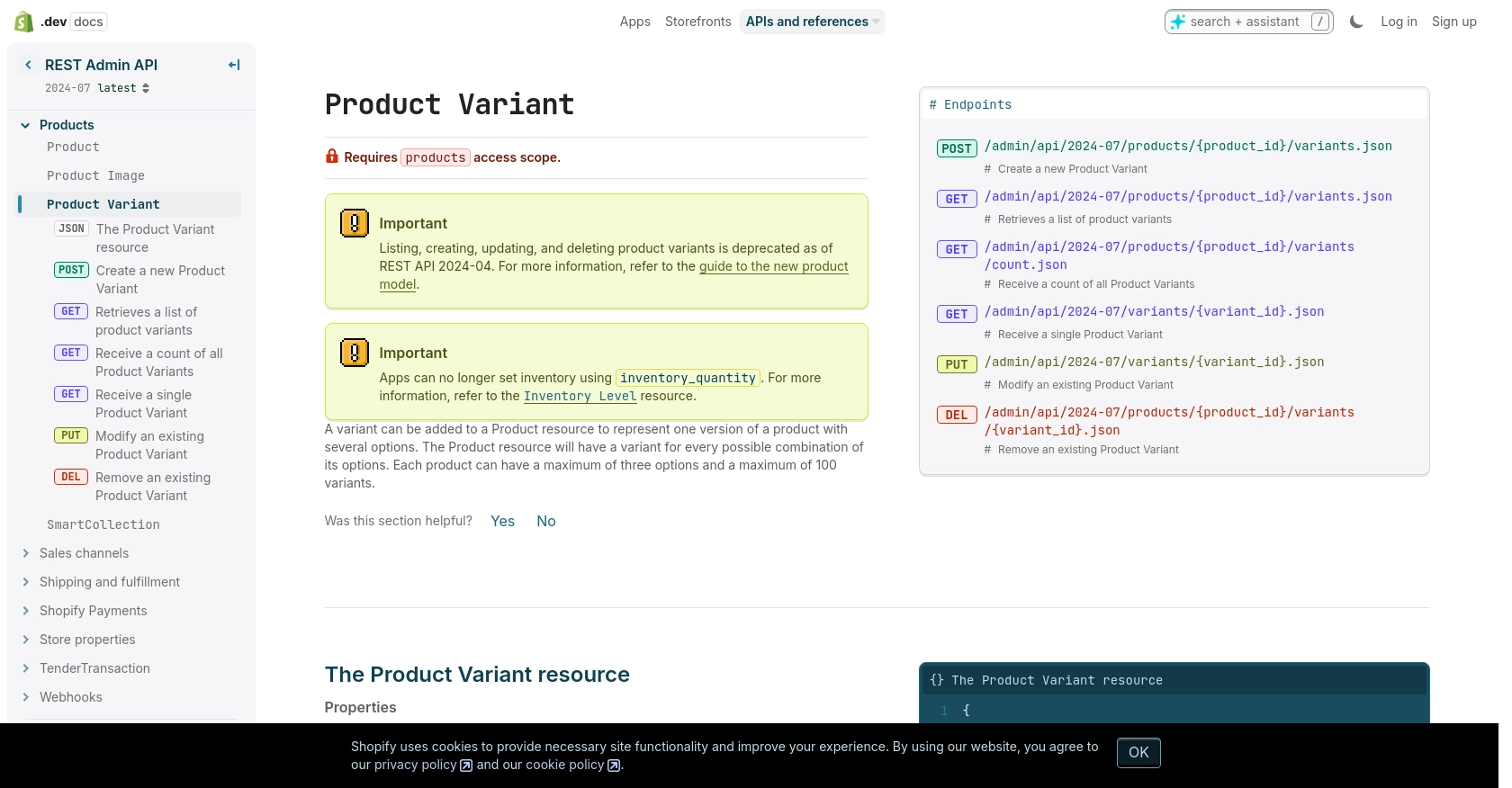
Conclusion and Best Practices for Using Shopify API with JavaScript
Integrating with the Shopify API to retrieve product variants using JavaScript offers a powerful way to manage and enhance your e-commerce store. By following the steps outlined in this article, you can efficiently access product data, streamline inventory management, and provide a better shopping experience for your customers.
Best Practices for Secure and Efficient Shopify API Integration
- Securely Store Credentials: Always store your API credentials securely and avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Shopify imposes a rate limit of 40 requests per app per store per minute. Implement error handling to manage 429 errors and use the
Retry-After
header to determine when to retry requests. For more details, refer to the Shopify rate limits documentation. - Optimize Data Handling: When retrieving large datasets, use pagination to manage data efficiently and reduce load times.
- Regularly Update API Versions: Shopify's API is versioned, with updates released quarterly. Ensure your application uses the latest supported version to take advantage of new features and security improvements.
Enhancing Your Integration Strategy with Endgrate
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing multiple integrations, allowing you to focus on your core product. With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Discover how Endgrate can save you time and resources by visiting Endgrate.
By leveraging the Shopify API and following best practices, you can create robust and scalable solutions that enhance your e-commerce operations. Whether you're managing product variants or exploring other Shopify functionalities, the possibilities are vast and exciting.
Read More
Ready to get started?