Using the Google Ads API to Get Leads (with Javascript examples)
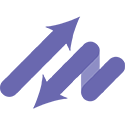
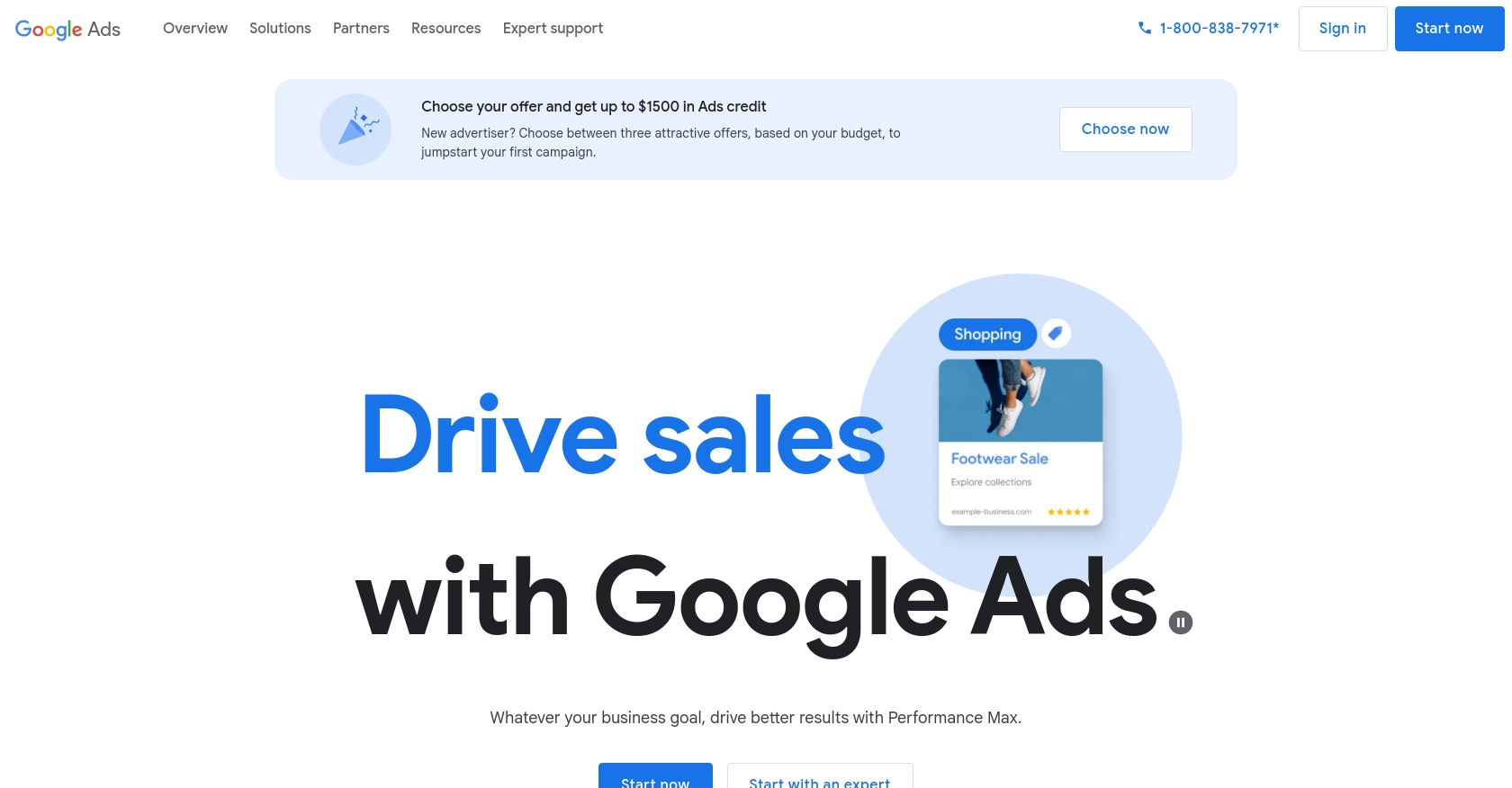
Introduction to Google Ads API
The Google Ads API is a powerful tool that allows developers to manage large and complex Google Ads accounts programmatically. It provides the flexibility to automate account management, create custom reports, and manage ad campaigns efficiently. This API is particularly beneficial for businesses looking to optimize their advertising strategies and streamline their marketing efforts.
Developers might want to connect with the Google Ads API to access lead data directly from their advertising campaigns. By retrieving leads through the API, businesses can integrate this data into their customer relationship management (CRM) systems, enabling real-time lead management and enhancing marketing automation.
For example, a developer could use the Google Ads API to automatically pull lead form submissions into a CRM, allowing sales teams to follow up with potential customers promptly. This integration not only saves time but also ensures that no lead is missed, ultimately improving conversion rates.
Setting Up Your Google Ads Test Account for API Integration
Before you can start using the Google Ads API to retrieve leads, you'll need to set up a test account. This involves creating a Google Ads manager account, obtaining a developer token, and setting up a Google API Console project. These steps will ensure you have the necessary credentials and permissions to interact with the API.
Create a Google Ads Manager Account
If you don't already have a Google Ads manager account, you'll need to create one. This account will allow you to manage multiple Google Ads accounts and is essential for accessing the Google Ads API.
- Visit the Google Ads Manager Accounts page.
- Follow the instructions to set up your manager account.
- Once your account is created, note down your manager account ID for future reference.
Obtain a Developer Token
A developer token is required to access the Google Ads API. This token is linked to your manager account and controls the number of API calls you can make.
- Log in to your Google Ads manager account.
- Navigate to the Tools & Settings menu and select API Center.
- Apply for a developer token by following the on-screen instructions.
- Once approved, your developer token will be available in the API Center.
Set Up a Google API Console Project
To use OAuth 2.0 for authentication, you'll need to create a project in the Google API Console. This project will generate the client ID and client secret required for API calls.
- Go to the Google API Console.
- Create a new project and give it a meaningful name.
- Enable the Google Ads API for your project by navigating to the Library and searching for "Google Ads API".
- In the Credentials section, click on Create Credentials and select OAuth client ID.
- Configure the consent screen and set the application type to Web application.
- Note down the generated client ID and client secret.
Authorize Your Application Using OAuth 2.0
With your client ID and client secret, you can now authorize your application to access the Google Ads API.
- Use the OAuth 2.0 Playground to generate an access token.
- Select the Google Ads API scopes you need, such as
https://www.googleapis.com/auth/adwords
. - Exchange the authorization code for an access token using your client ID and client secret.
Once you have completed these steps, your test account is set up, and you are ready to make API calls to retrieve leads from Google Ads. For more detailed information, refer to the Google Ads API documentation.
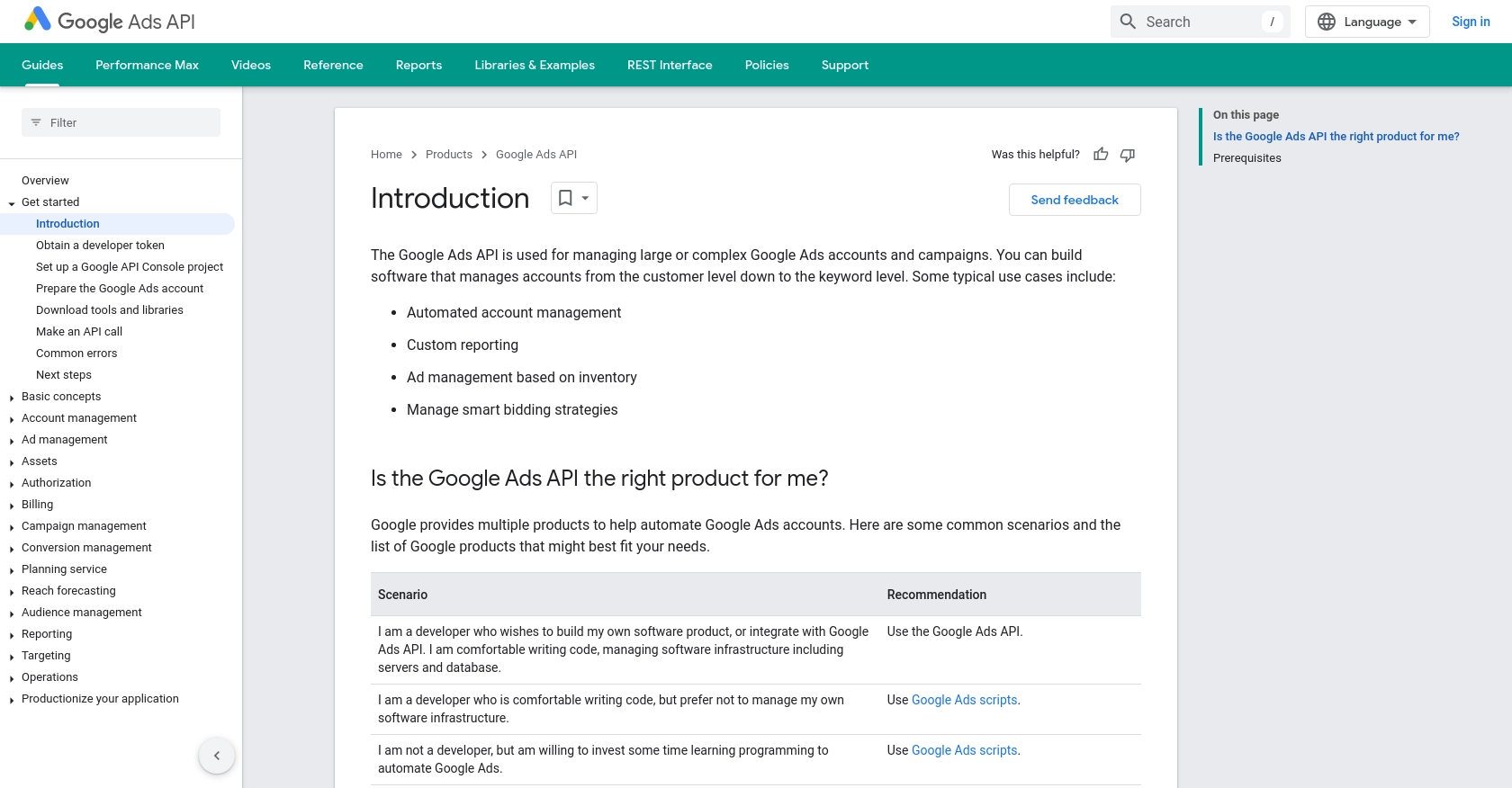
sbb-itb-96038d7
Making API Calls to Retrieve Leads Using Google Ads API with JavaScript
To interact with the Google Ads API and retrieve leads, you'll need to use JavaScript. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A text editor or IDE such as Visual Studio Code.
- Google Ads API client library for JavaScript. Install it using npm:
npm install google-ads-api
Writing the JavaScript Code to Retrieve Leads
Now, let's write the code to make an API call to retrieve leads from Google Ads:
const { GoogleAdsApi } = require('google-ads-api');
// Initialize the Google Ads API client
const client = new GoogleAdsApi({
client_id: 'YOUR_CLIENT_ID',
client_secret: 'YOUR_CLIENT_SECRET',
developer_token: 'YOUR_DEVELOPER_TOKEN',
refresh_token: 'YOUR_REFRESH_TOKEN',
});
// Define the customer ID and query to retrieve leads
const customerId = 'YOUR_CUSTOMER_ID';
const query = `
SELECT
lead_form_submission_data.resource_name,
lead_form_submission_data.id,
lead_form_submission_data.asset,
lead_form_submission_data.campaign,
lead_form_submission_data.lead_form_submission_fields
FROM
lead_form_submission_data
WHERE
segments.date DURING LAST_30_DAYS
`;
// Function to fetch leads
async function fetchLeads() {
try {
const response = await client.customer(customerId).query(query);
console.log('Leads retrieved successfully:', response);
} catch (error) {
console.error('Error retrieving leads:', error);
}
}
// Execute the function
fetchLeads();
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_DEVELOPER_TOKEN
, YOUR_REFRESH_TOKEN
, and YOUR_CUSTOMER_ID
with your actual credentials.
Handling API Responses and Errors
After executing the code, you should see the leads retrieved from your Google Ads account. If the request is successful, the response will contain the lead data, which you can then integrate into your CRM or other systems.
In case of errors, the catch block will log the error details. Common error codes include:
- 401 Unauthorized: Check your OAuth credentials.
- 403 Forbidden: Ensure your developer token is approved for production use.
- 429 Too Many Requests: You have exceeded the API rate limits.
For more detailed error handling, refer to the Google Ads API error handling documentation.
Verifying API Call Success
To verify that your API call was successful, check the response data for the expected lead information. Additionally, you can log into your Google Ads account and confirm that the leads match the data retrieved by your API call.
For further information on making API calls, consult the Google Ads API reference documentation.
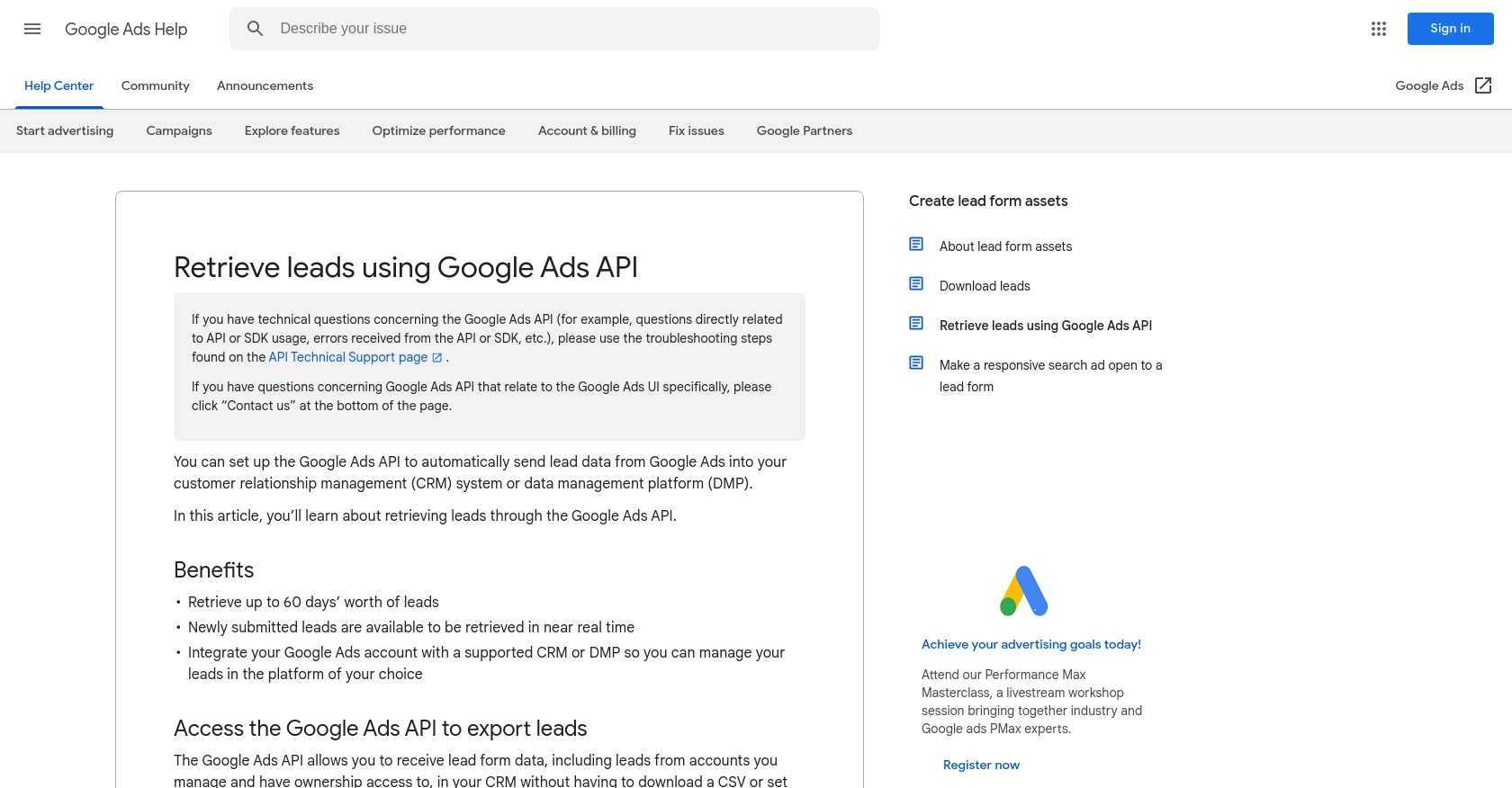
Conclusion: Best Practices for Using Google Ads API to Retrieve Leads
Integrating the Google Ads API into your systems can significantly enhance your lead management process by providing real-time access to lead data. However, to maximize the benefits of this integration, it's essential to follow best practices.
Securely Storing User Credentials
Ensure that all sensitive information, such as client IDs, client secrets, and refresh tokens, are stored securely. Use environment variables or secure vaults to manage these credentials and avoid hardcoding them in your source code.
Handling Google Ads API Rate Limits
The Google Ads API has rate limits that you must adhere to in order to avoid disruptions. Implement exponential backoff strategies to handle 429 Too Many Requests
errors gracefully. For more details, refer to the Google Ads API rate limits documentation.
Transforming and Standardizing Lead Data
When retrieving lead data, ensure that it is transformed and standardized to fit your CRM or data management platform's requirements. This will facilitate seamless integration and improve data consistency across your systems.
Leverage Endgrate for Simplified Integrations
If managing multiple integrations is becoming a challenge, consider using Endgrate. With Endgrate, you can streamline your integration process by connecting to multiple platforms through a single API endpoint. This allows you to focus on your core product while ensuring a smooth integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs.
Read More
Ready to get started?