Using the Sap Business One API to Get Purchase Orders (with Javascript examples)
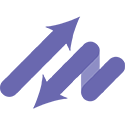
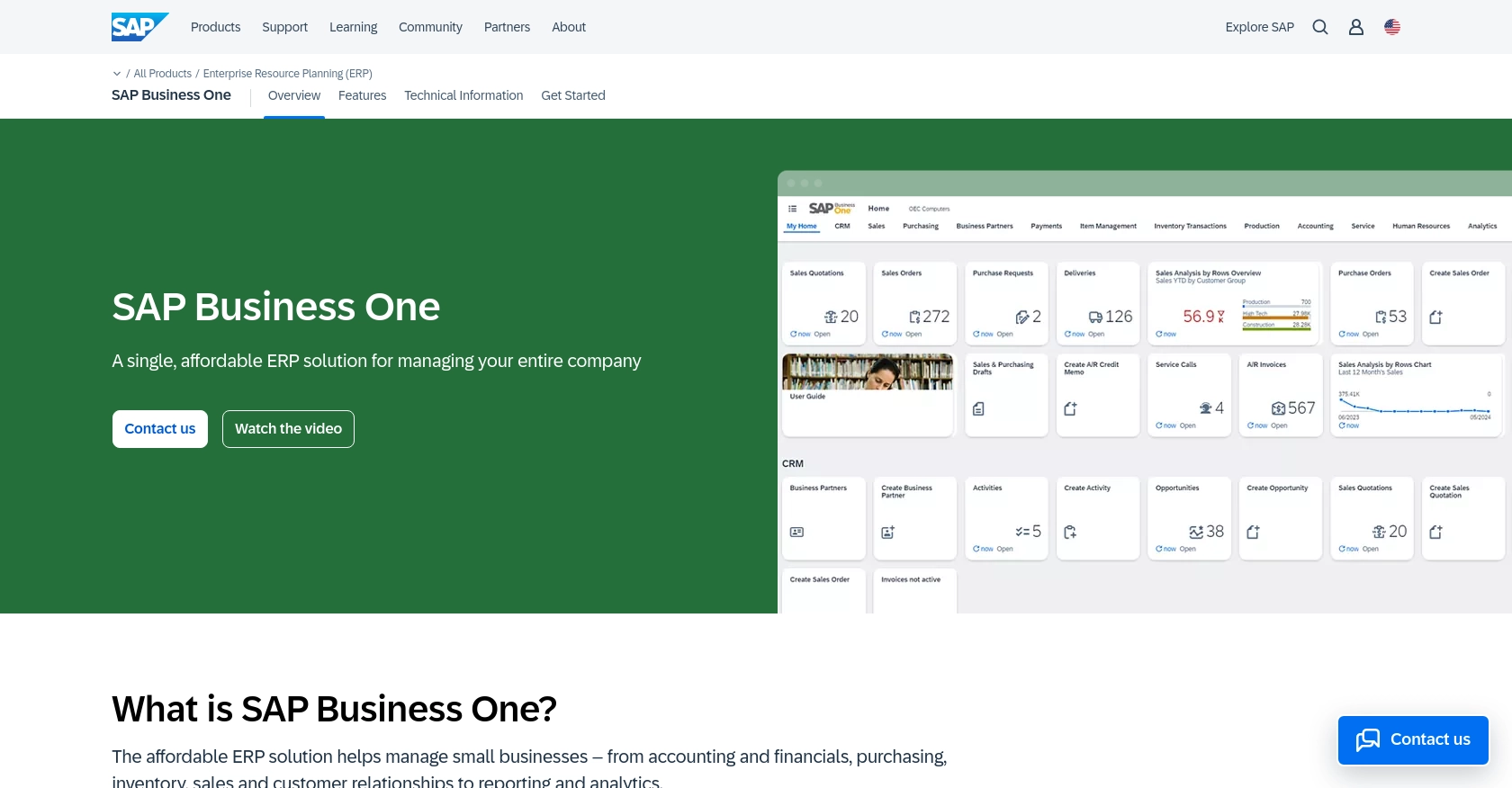
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed specifically for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, inventory, and operations.
Integrating with the SAP Business One API allows developers to streamline business processes by automating tasks such as retrieving purchase orders. For example, a developer might use the SAP Business One API to automatically fetch purchase order data and integrate it into a custom reporting tool, enhancing decision-making and operational efficiency.
Setting Up Your SAP Business One Test/Sandbox Account
Before you can start interacting with the SAP Business One API, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting your live data.
Creating a SAP Business One Sandbox Account
To begin, you'll need access to a SAP Business One environment. If you don't have one, you can request a demo or trial version through SAP's official website. This will provide you with a sandbox environment to test your API integrations.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method. To authenticate API requests, you need to create an app within your SAP Business One sandbox account. Follow these steps:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer section.
- Create a new application by providing necessary details such as app name and description.
- Once the app is created, note down the Client ID and Client Secret. These will be used to authenticate your API requests.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need API keys for certain operations. Here's how to generate them:
- Go to the API Management section in your SAP Business One account.
- Select Create API Key and fill out the required information.
- Save the generated API key securely, as it will be used in your API calls.
With your sandbox account set up and authentication credentials ready, you're now prepared to start making API calls to retrieve purchase orders using JavaScript.
For more detailed information on authentication, refer to the SAP Business One Service Layer documentation.
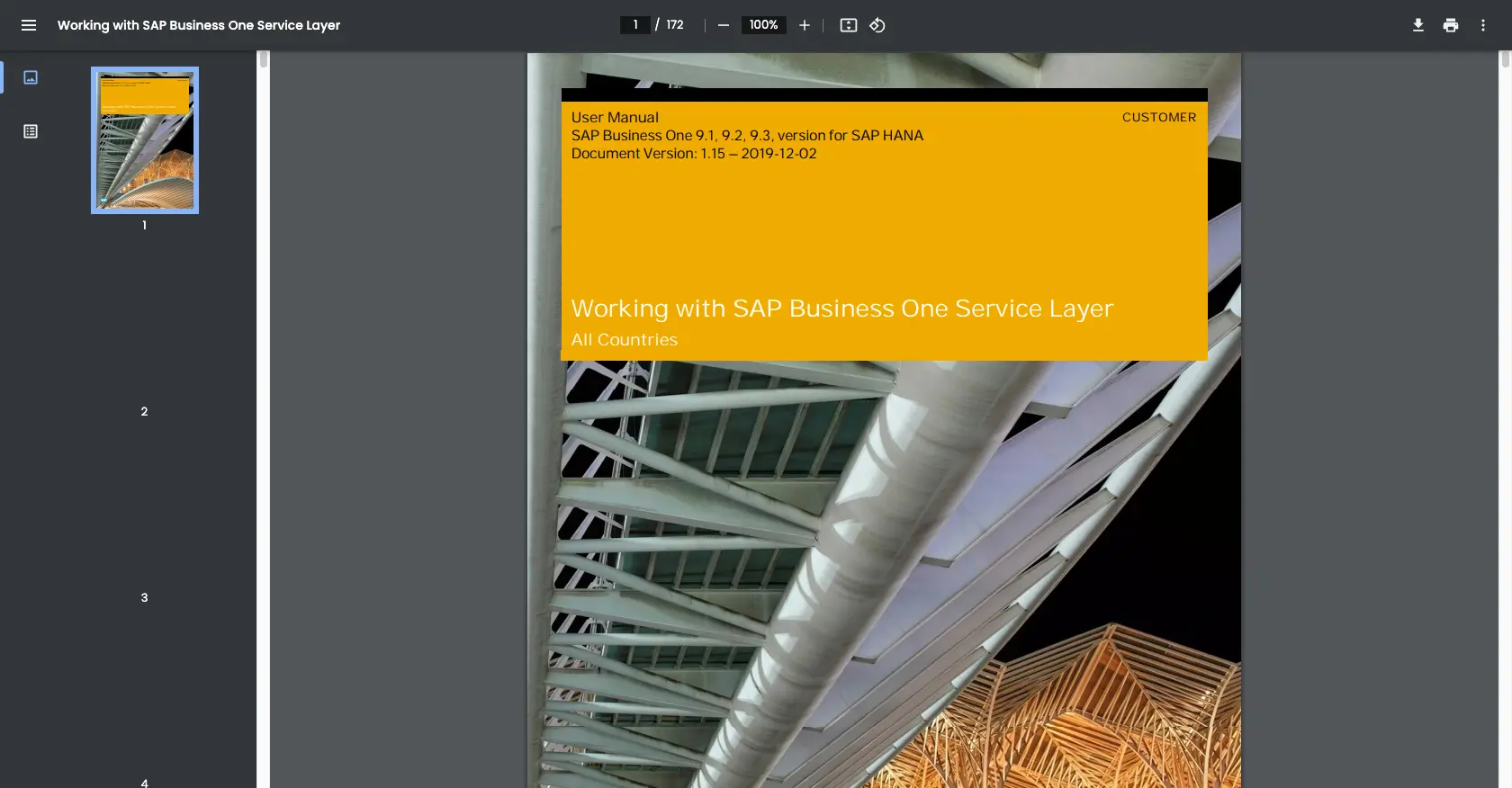
sbb-itb-96038d7
How to Make API Calls to Retrieve Purchase Orders Using JavaScript with SAP Business One
To interact with the SAP Business One API and retrieve purchase orders using JavaScript, you need to ensure that your development environment is properly set up. This section will guide you through the necessary steps, including setting up your JavaScript environment, writing the code to make API calls, and handling responses and errors.
Setting Up Your JavaScript Environment for SAP Business One API
Before making API calls, ensure you have the following prerequisites installed:
- Node.js (version 14.x or later)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, you can set up your project by creating a new directory and initializing it:
mkdir sap-business-one-api
cd sap-business-one-api
npm init -y
Next, install the necessary dependencies, such as the axios
library for making HTTP requests:
npm install axios
Writing JavaScript Code to Fetch Purchase Orders from SAP Business One
Create a new file named getPurchaseOrders.js
and add the following code to it:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/PurchaseOrders';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
// Function to fetch purchase orders
async function getPurchaseOrders() {
try {
const response = await axios.get(endpoint, { headers });
const purchaseOrders = response.data.value;
// Display purchase orders
purchaseOrders.forEach(order => {
console.log(`Order ID: ${order.DocEntry}, Supplier: ${order.CardName}`);
});
} catch (error) {
console.error('Error fetching purchase orders:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getPurchaseOrders();
Replace Your_Access_Token
with the token obtained during the authentication setup.
Running the JavaScript Code and Verifying API Call Success
To execute the code, run the following command in your terminal:
node getPurchaseOrders.js
If successful, you should see a list of purchase orders printed in the console. Verify the data by checking your SAP Business One sandbox account to ensure the retrieved orders match the expected results.
Handling Errors and Understanding SAP Business One API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The SAP Business One API may return various error codes, such as:
- 401 Unauthorized: Indicates invalid authentication credentials.
- 404 Not Found: The requested resource does not exist.
- 500 Internal Server Error: An unexpected error occurred on the server.
Ensure your code includes error handling logic to manage these scenarios and provide meaningful feedback to users.
Conclusion and Best Practices for Using SAP Business One API with JavaScript
Integrating with the SAP Business One API using JavaScript can significantly enhance your business processes by automating tasks such as retrieving purchase orders. By following the steps outlined in this article, you can efficiently set up your environment, authenticate your requests, and handle API interactions.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API keys and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement retry logic and exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed for your application. This will help maintain consistency and accuracy across your systems.
- Error Handling: Implement comprehensive error handling to manage different error codes returned by the API. Provide clear feedback to users and log errors for further analysis.
Streamlining Integrations with Endgrate
While integrating with SAP Business One API can be rewarding, it can also be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including SAP Business One. This allows you to focus on your core product while outsourcing the complexities of integration.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate today.
Read More
Ready to get started?