Using the Webhook API to Push Data in Python
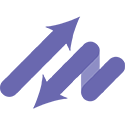
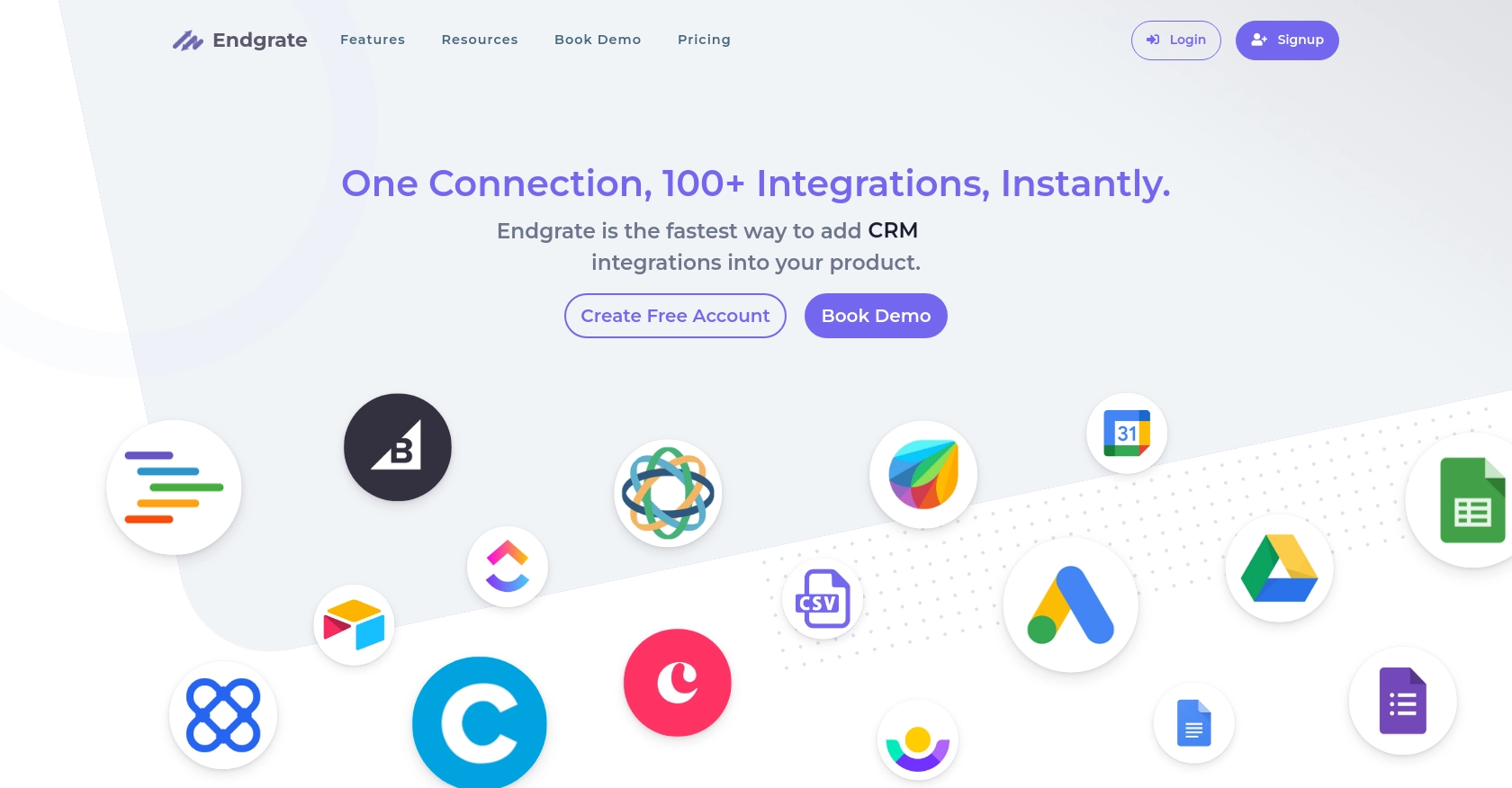
Introduction to Webhook API Integration
Webhooks are a powerful tool for enabling real-time data transfer between applications. They allow developers to send data to a specific URL, triggering actions in another system whenever an event occurs. This makes them an essential component for building responsive and interconnected software solutions.
Integrating with a Webhook API can significantly enhance a developer's ability to automate workflows and synchronize data across platforms. For example, a developer might use a Webhook API to push data from a customer relationship management (CRM) system to a marketing automation platform, ensuring that customer interactions are promptly reflected in marketing campaigns.
This article will guide you through the process of using Python to interact with a Webhook API, demonstrating how to efficiently push data and handle responses to ensure successful integration.
Setting Up a Test or Sandbox Environment for Webhook API Integration
Before you begin pushing data using the Webhook API, it's crucial to set up a test or sandbox environment. This allows you to experiment with the integration without affecting live data, ensuring that your implementation is both safe and effective.
Creating a Test Environment for Webhook API
To start, you'll need to establish a test environment where you can safely send and receive data. This typically involves setting up a local server or using a service that can receive webhook requests.
- Local Server: You can use tools like ngrok to expose your local server to the internet, allowing you to receive webhook requests.
- Online Services: Platforms like Webhook.site provide temporary URLs for testing webhook integrations.
Configuring the Webhook URL
Once your test environment is ready, you'll need to configure the Webhook URL. This is the endpoint where the data will be sent. Ensure that the URL is correctly set up to handle incoming POST requests.
For example, if you're using Flask to create a local server, your code might look like this:
from flask import Flask, request
app = Flask(__name__)
@app.route('/webhook', methods=['POST'])
def webhook():
data = request.json
print(data)
return 'Webhook received', 200
if __name__ == '__main__':
app.run(port=5000)
Testing the Webhook Configuration
Before proceeding with the full integration, it's essential to test the webhook configuration. Send a test request to your Webhook URL to ensure that it is functioning as expected. This step helps verify that the endpoint is correctly set up to receive and process data.
Use tools like Postman to send a sample POST request to your Webhook URL and check the response to confirm successful data reception.
Making API Calls to Webhook Using Python
To effectively push data to a Webhook API using Python, you'll need to understand the process of making HTTP requests. Python's simplicity and extensive libraries make it an excellent choice for interacting with web services.
Setting Up Python Environment for Webhook API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library, which simplifies HTTP requests.
pip install requests
Creating a Python Script to Push Data to Webhook
Once your environment is set up, you can create a Python script to send data to the Webhook API. Here's a basic example:
import requests
# Define the Webhook URL
webhook_url = 'https://your-webhook-url.com/webhook'
# Prepare the data to be sent
data = {
'event': 'new_customer',
'customer_id': 12345,
'name': 'John Doe',
'email': 'john.doe@example.com'
}
# Send the POST request
response = requests.post(webhook_url, json=data)
# Check the response status
if response.status_code == 200:
print('Data pushed successfully!')
else:
print(f'Failed to push data. Status code: {response.status_code}')
In this script, you define the Webhook URL and prepare the data in JSON format. The requests.post()
method sends the data to the Webhook, and the response status code is checked to confirm success.
Handling Responses and Errors in Webhook API Calls
Properly handling responses and errors is crucial for robust integration. The server's response will indicate whether the request was successful or if there was an error.
- Success: A status code in the 200 range indicates success. Log the response for confirmation.
- Error Handling: If the status code is outside the 200 range, log the error and implement retry mechanisms if necessary.
Consider using a try-except block to handle exceptions and ensure your application can recover from unexpected issues.
Verifying Webhook API Call Success
After making the API call, verify that the data was received correctly by checking the test or sandbox environment. This step ensures that your integration is functioning as expected.
Use logging to capture the response and any errors, which will help in troubleshooting and improving the integration process.
Conclusion: Best Practices for Webhook API Integration in Python
Integrating with a Webhook API using Python can greatly enhance your application's responsiveness and data synchronization capabilities. By following best practices, you can ensure a robust and efficient integration process.
Securely Storing Webhook Configuration and Credentials
It's crucial to securely store any configuration details and credentials associated with your Webhook API integration. Consider using environment variables or secure vaults to keep sensitive information safe and prevent unauthorized access.
Managing Webhook API Rate Limits and Data Batching
Understanding and adhering to any rate limits imposed by the Webhook API is essential to avoid throttling or service disruptions. Implement data batching strategies where applicable to optimize the number of requests and ensure efficient data transfer.
Implementing Error Handling and Retry Logic
Proper error handling is vital for maintaining a stable integration. Use try-except blocks to catch exceptions and implement retry mechanisms for transient errors. Logging errors and responses will aid in troubleshooting and improving the integration.
Transforming and Standardizing Data for Webhook API
Ensure that the data sent to the Webhook API is in the correct format and standardized according to the API's requirements. This step minimizes errors and ensures seamless data processing on the receiving end.
Leveraging Endgrate for Simplified Webhook Integrations
If managing multiple integrations becomes overwhelming, consider using Endgrate to streamline the process. Endgrate provides a unified API endpoint that connects to various platforms, allowing you to focus on your core product while outsourcing complex integrations.
By utilizing Endgrate, you can save time and resources, build once for each use case, and offer an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?