Using the Zoho Books API to Get Tax Rates (with Python examples)
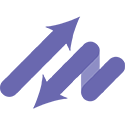
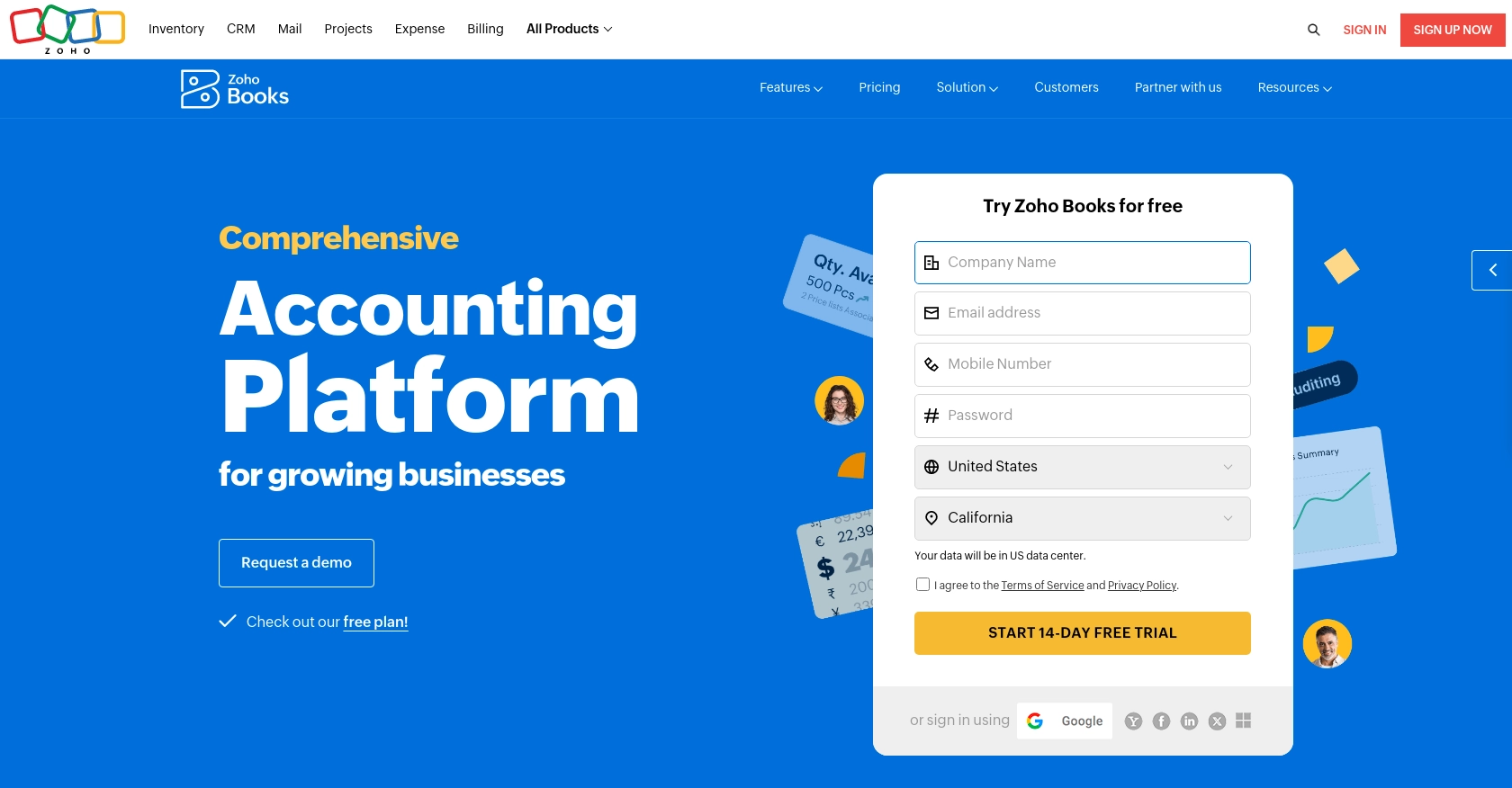
Introduction to Zoho Books API
Zoho Books is a comprehensive cloud-based accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and tax management, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to automate financial processes and access critical financial data programmatically. For example, developers can use the API to retrieve tax rates, which can be essential for calculating taxes on transactions in real-time, ensuring compliance and accuracy in financial reporting.
This article will guide you through using Python to interact with the Zoho Books API to get tax rates, providing you with practical examples and insights into efficient API integration.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start using the Zoho Books API to retrieve tax rates, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Create a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. This trial will give you access to all the features you need to test the API.
- Visit the Zoho Books website.
- Click on "Free Trial" and follow the instructions to create your account.
- Once your account is created, log in to access the Zoho Books dashboard.
Register Your Application in Zoho's Developer Console
To interact with the Zoho Books API, you need to register your application to obtain the necessary OAuth credentials.
- Go to the Zoho Developer Console.
- Click on "Add Client ID" to register your application.
- Fill in the required details, such as the application name and redirect URI.
- After registration, you'll receive a Client ID and Client Secret. Keep these credentials secure.
Generate OAuth Tokens for Zoho Books API Access
Zoho Books uses OAuth 2.0 for authentication. Follow these steps to generate the necessary tokens:
- Redirect users to the following authorization URL to obtain a grant token:
- After the user consents, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- Store the access_token and refresh_token securely for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.settings.READ&client_id=Your_Client_ID&response_type=code&redirect_uri=Your_Redirect_URI&access_type=offline
import requests
url = "https://accounts.zoho.com/oauth/v2/token"
payload = {
'code': 'Your_Grant_Code',
'client_id': 'Your_Client_ID',
'client_secret': 'Your_Client_Secret',
'redirect_uri': 'Your_Redirect_URI',
'grant_type': 'authorization_code'
}
response = requests.post(url, data=payload)
tokens = response.json()
print(tokens)
By following these steps, you'll have your Zoho Books test account and OAuth credentials ready, allowing you to proceed with making API calls to retrieve tax rates.
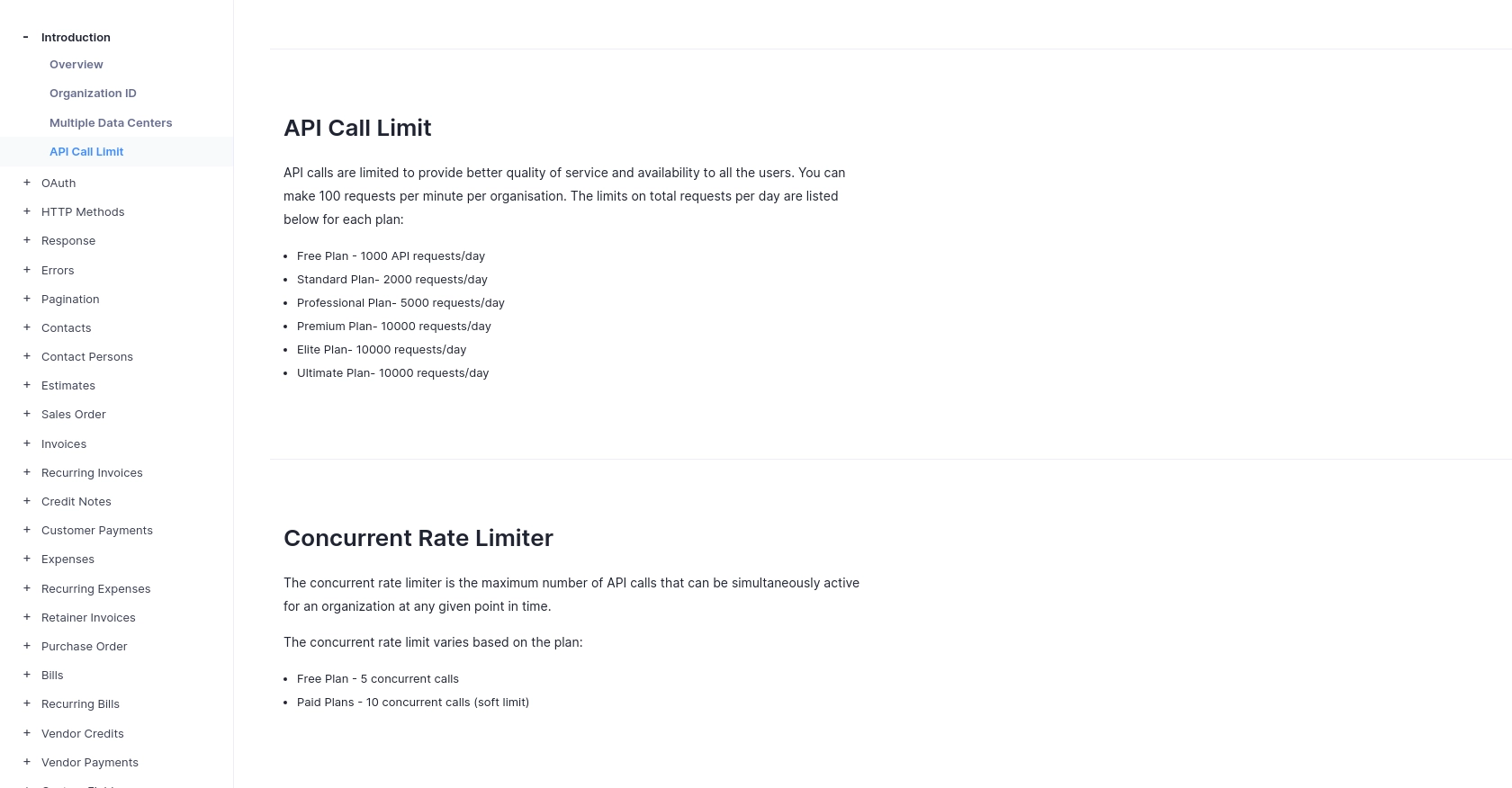
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates from Zoho Books Using Python
Now that you have set up your Zoho Books account and obtained the necessary OAuth credentials, you can proceed to make API calls to retrieve tax rates. This section will guide you through the process of using Python to interact with the Zoho Books API.
Prerequisites for Using Python with Zoho Books API
Before making API calls, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Install the requests
library using the following command:
pip install requests
Example Code to Retrieve Tax Rates from Zoho Books
Create a Python file named get_tax_rates.py
and add the following code to retrieve tax rates:
import requests
# Set the API endpoint and headers
endpoint = "https://www.zohoapis.com/books/v3/settings/taxes?organization_id=Your_Organization_ID"
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
for tax in data["taxes"]:
print(f"Tax Name: {tax['tax_name']}, Tax Percentage: {tax['tax_percentage']}")
else:
print(f"Failed to retrieve tax rates. Status Code: {response.status_code}")
Replace Your_Organization_ID
and Your_Access_Token
with your actual organization ID and access token.
Running the Python Script and Verifying the Output
Run the script from the terminal using the following command:
python get_tax_rates.py
You should see the tax rates printed in the terminal. If the request fails, ensure your access token is valid and check the status code for more information.
Handling Errors and Understanding Response Codes
Zoho Books API uses HTTP status codes to indicate the result of an API call. Here are some common codes you might encounter:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: The access token is invalid or expired.
- 429 Rate Limit Exceeded: You have exceeded the API call limit.
For more details on error codes, refer to the Zoho Books API documentation.
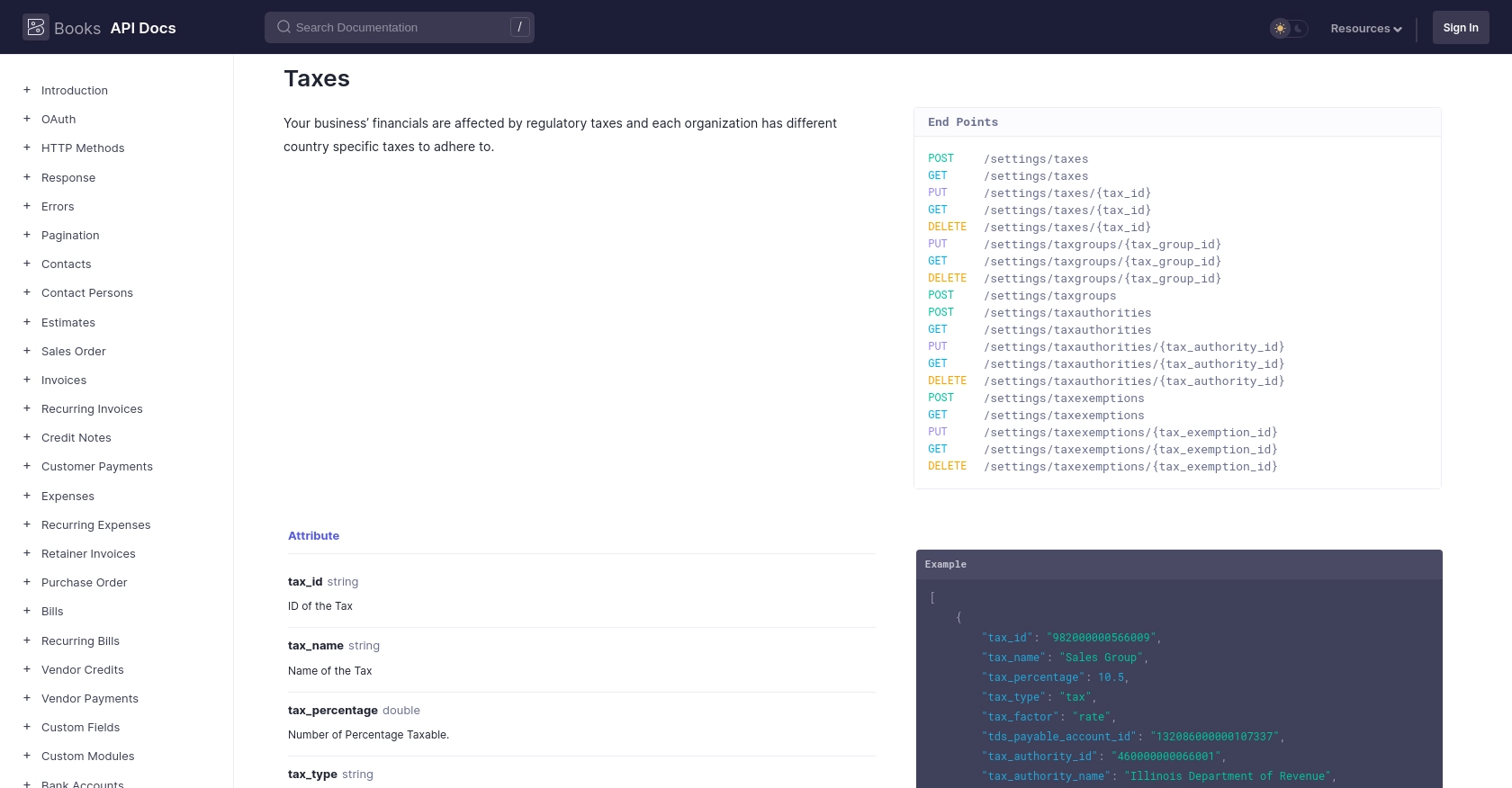
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API to retrieve tax rates using Python can significantly streamline your financial processes. By automating the retrieval of tax data, you ensure accuracy and compliance in your financial reporting, saving time and reducing manual errors.
Best Practices for Secure and Efficient Zoho Books API Usage
- Secure Storage of Credentials: Always store your OAuth tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Zoho Books API has a rate limit of 100 requests per minute per organization. Implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the API call limit documentation.
- Data Transformation and Standardization: Ensure that the tax data retrieved from Zoho Books is transformed and standardized to fit your application's data model. This will facilitate seamless integration with other systems.
Enhancing Your Integration Strategy with Endgrate
While integrating with Zoho Books API offers numerous benefits, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho Books.
By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/taxes/#overview
Ready to get started?