How to Create or Update Contacts with the Salesflare API in Python
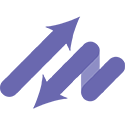
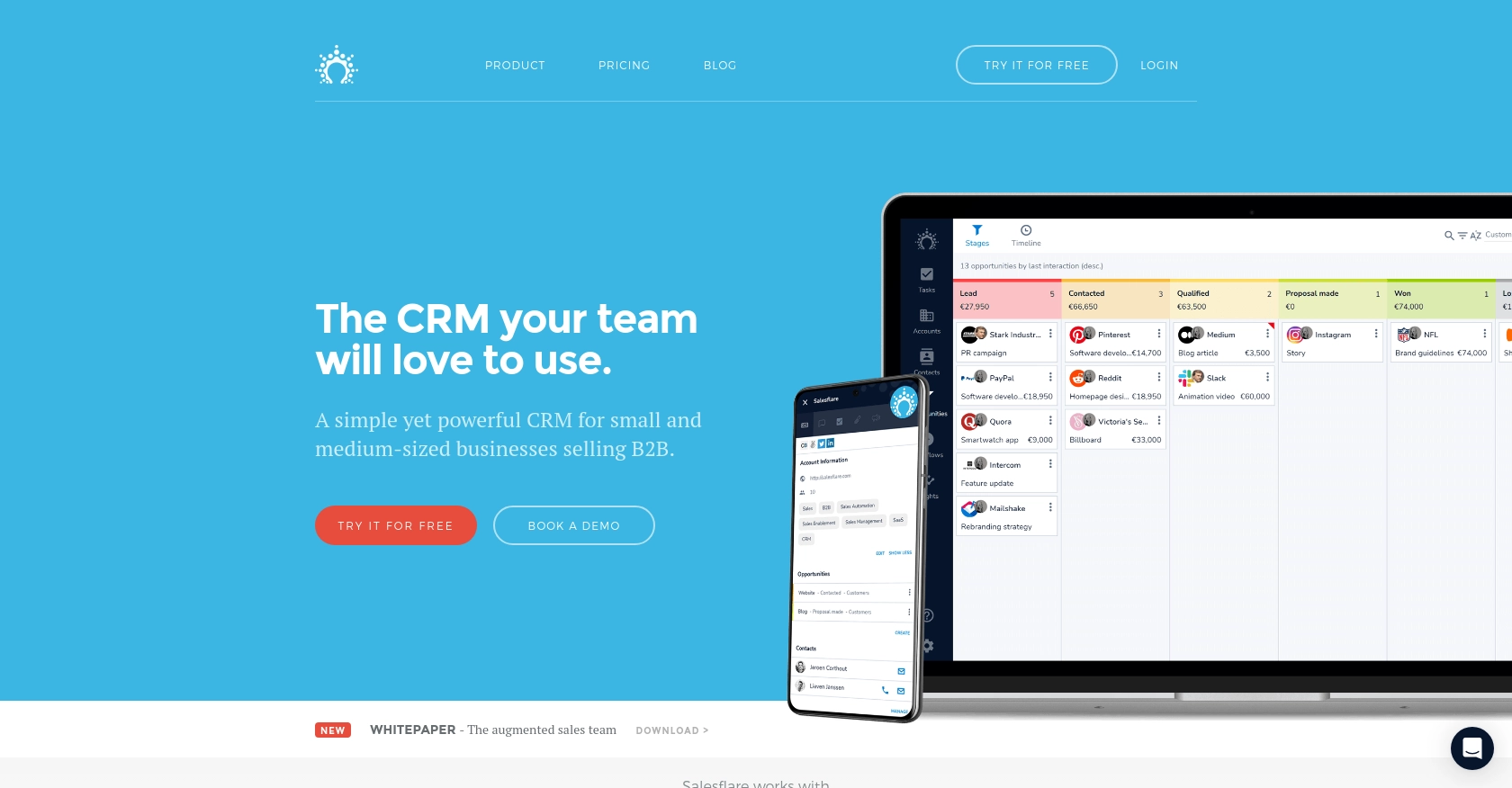
Introduction to Salesflare API Integration
Salesflare is a powerful CRM platform designed to simplify sales processes for small and medium-sized businesses. It offers a range of features that help sales teams manage customer relationships, track interactions, and automate repetitive tasks.
Integrating with Salesflare's API allows developers to enhance their applications by automating the management of contacts. For example, a developer can use the Salesflare API to create or update contact information directly from their application, ensuring that sales teams have access to the most up-to-date customer data.
This article will guide you through the process of using Python to interact with the Salesflare API, enabling you to efficiently create or update contacts within the Salesflare platform.
Setting Up Your Salesflare Test Account for API Integration
Before you can start integrating with the Salesflare API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Salesflare Account
If you don't already have a Salesflare account, you can sign up for a free trial on the Salesflare website. This trial will give you access to the necessary features to test API interactions.
- Visit the Salesflare website and click on the "Try for Free" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Salesflare dashboard.
Generating an API Key for Salesflare
Salesflare uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your Salesflare account and navigate to the settings page.
- Locate the "API" section in the settings menu.
- Click on "Generate API Key" and copy the key provided. Keep this key secure, as it will be used to authenticate your API requests.
Configuring Your Development Environment
With your API key in hand, you're ready to configure your development environment to interact with the Salesflare API using Python. Ensure you have Python installed on your machine, along with the necessary libraries for making HTTP requests.
- Ensure Python 3.x is installed on your system. You can download it from the official Python website.
- Install the
requests
library, which will be used to make API calls. Run the following command in your terminal:
pip install requests
With your test account and development environment set up, you're now ready to start making API calls to create or update contacts in Salesflare.
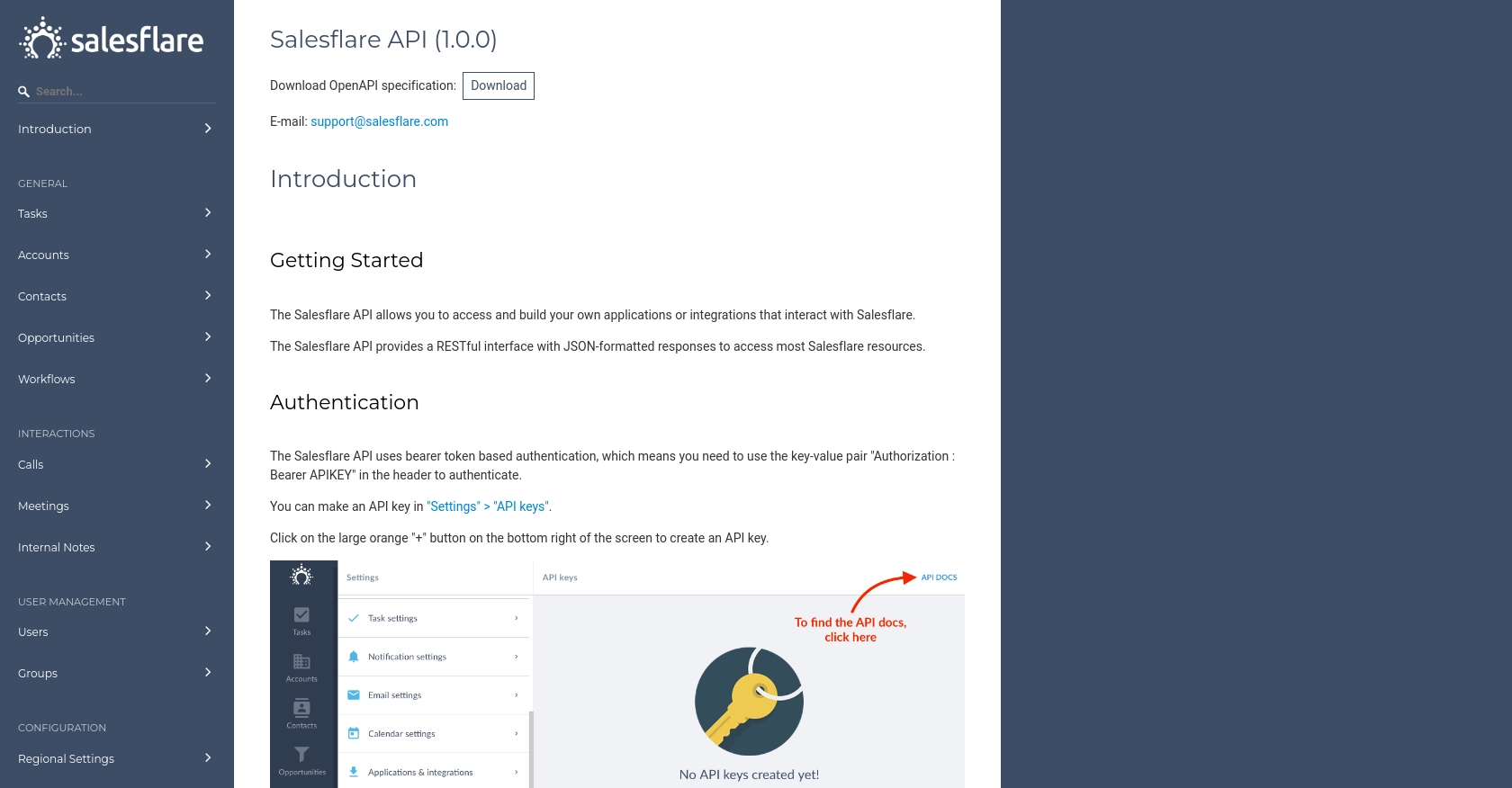
sbb-itb-96038d7
Making API Calls to Create or Update Contacts with Salesflare Using Python
With your Salesflare test account and API key ready, you can now proceed to make API calls to create or update contacts. This section will guide you through the process of setting up your Python environment and executing the necessary API requests.
Setting Up Your Python Environment for Salesflare API Integration
Before making API calls, ensure your Python environment is correctly configured. You'll need Python 3.x and the requests
library to handle HTTP requests.
- Verify Python 3.x is installed on your machine. If not, download it from the official Python website.
- Install the
requests
library by running the following command in your terminal:
pip install requests
Creating a Contact with the Salesflare API in Python
To create a new contact in Salesflare, you'll need to send a POST request to the appropriate endpoint. Below is a sample Python script to create a contact:
import requests
# Define the API endpoint and headers
url = "https://api.salesflare.com/v1/contacts"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_API_Key"
}
# Define the contact data
contact_data = {
"email": "example@salesflare.com",
"firstName": "John",
"lastName": "Doe"
}
# Send the POST request
response = requests.post(url, json=contact_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Contact created successfully.")
else:
print(f"Failed to create contact: {response.status_code} - {response.text}")
Replace Your_API_Key
with the API key you generated earlier. This script sends a POST request to create a contact with the specified email, first name, and last name. If successful, you'll receive a confirmation message.
Updating a Contact with the Salesflare API in Python
To update an existing contact, you'll need to send a PUT request. Here's an example script to update a contact:
import requests
# Define the API endpoint and headers
contact_id = "contact_id_here" # Replace with the actual contact ID
url = f"https://api.salesflare.com/v1/contacts/{contact_id}"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_API_Key"
}
# Define the updated contact data
updated_data = {
"firstName": "Jane",
"lastName": "Smith"
}
# Send the PUT request
response = requests.put(url, json=updated_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Contact updated successfully.")
else:
print(f"Failed to update contact: {response.status_code} - {response.text}")
Replace contact_id_here
with the ID of the contact you wish to update and Your_API_Key
with your API key. This script updates the contact's first and last name.
Verifying API Call Success in Salesflare
After executing the API calls, verify the changes in your Salesflare test account. Check the contacts list to ensure the new contact is created or the existing contact is updated as expected.
Handling Errors and Troubleshooting API Requests
When making API calls, it's essential to handle potential errors. The response status codes can help identify issues:
- 201 Created: The contact was successfully created.
- 200 OK: The contact was successfully updated.
- 400 Bad Request: The request was invalid. Check your data and headers.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 404 Not Found: The specified contact ID does not exist.
By understanding these status codes, you can troubleshoot and resolve issues effectively.
Conclusion and Best Practices for Salesflare API Integration
Integrating with the Salesflare API using Python can significantly enhance your application's ability to manage contacts efficiently. By automating the creation and updating of contact information, you ensure that your sales team always has access to the most current data, improving overall productivity and customer relationship management.
Best Practices for Secure and Efficient API Integration with Salesflare
- Securely Store API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Salesflare API to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data fields you send and receive are standardized to maintain consistency across your application.
- Error Handling: Implement robust error handling to manage different response status codes effectively, ensuring that your application can recover from failures smoothly.
Streamline Your Integration Process with Endgrate
While integrating with individual APIs like Salesflare can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with various platforms, including Salesflare.
By leveraging Endgrate, you can save time and resources, allowing your development team to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?