How to Get Users with the Hubspot API in Python
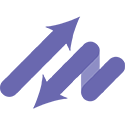
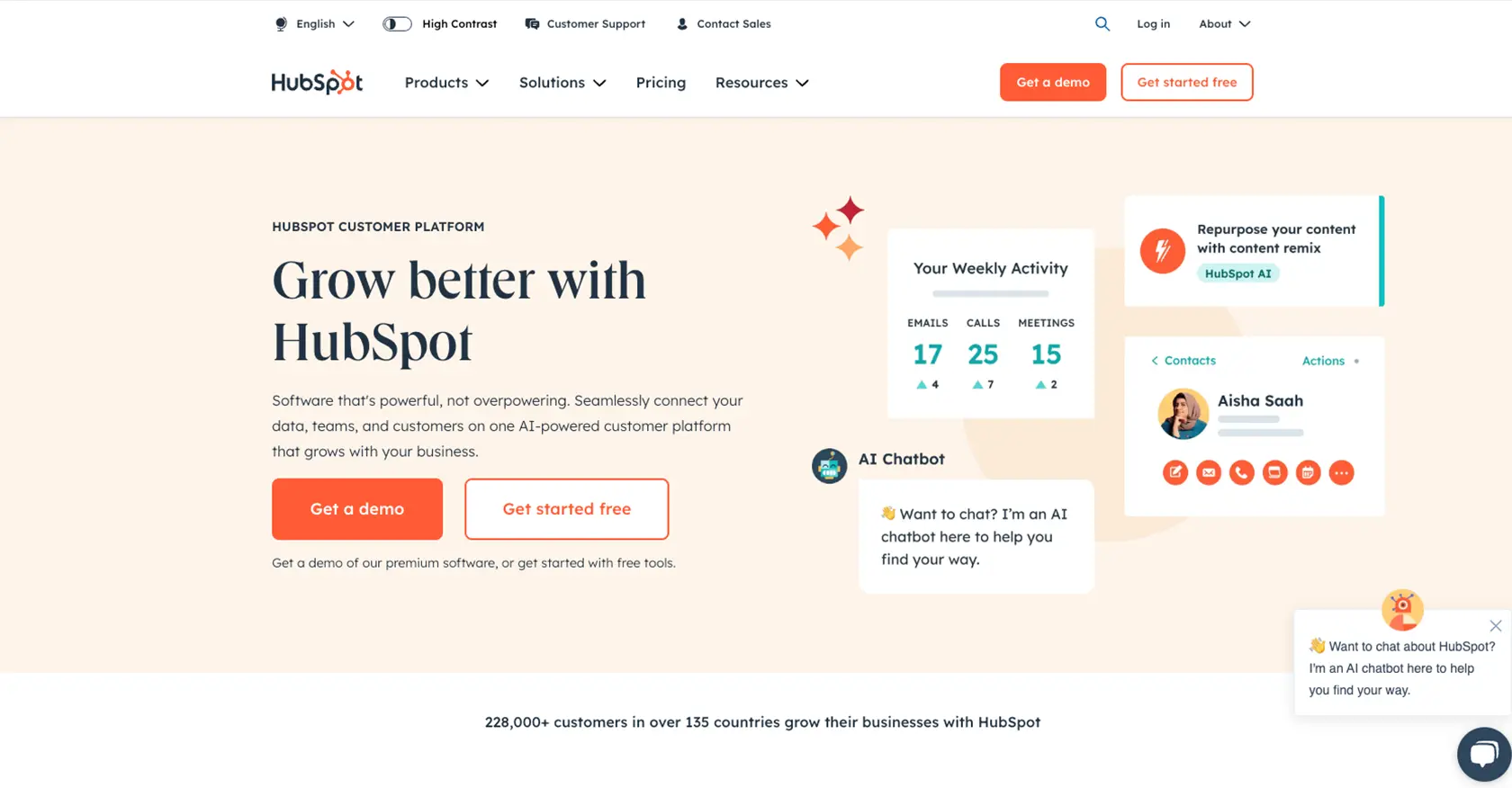
Introduction to HubSpot API Integration
HubSpot is a powerful CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for companies looking to streamline their operations and enhance customer relationships.
Integrating with HubSpot's API allows developers to access and manipulate user data, enabling seamless synchronization with external systems. For example, a developer might use the HubSpot API to retrieve user information and update an external workforce management tool, ensuring that user data remains consistent across platforms.
This article will guide you through the process of using Python to interact with the HubSpot API, specifically focusing on retrieving user data. By following this tutorial, you'll learn how to efficiently access and manage user information within the HubSpot ecosystem.
Setting Up Your HubSpot Test Account for API Integration
Before you can begin interacting with the HubSpot API using Python, you'll need to set up a HubSpot test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to create a test environment and obtain the necessary credentials for OAuth authentication.
Create a HubSpot Developer Account
If you don't already have a HubSpot developer account, you'll need to create one. This account will enable you to build and manage apps that interact with HubSpot's API.
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and follow the registration process.
- Once registered, log in to your developer account.
Set Up a HubSpot Test Account
With your developer account ready, you can now set up a test account to simulate real-world scenarios without impacting actual data.
- Navigate to the "Test Accounts" section in your developer dashboard.
- Create a new test account by following the on-screen instructions.
- Use this test account to perform API calls and verify their outcomes.
Create a HubSpot App for OAuth Authentication
To interact with the HubSpot API, you'll need to create an app that uses OAuth for authentication. This process will provide you with a client ID and client secret, which are essential for making authorized API requests.
- In your developer account, go to the "Apps" section and click "Create app."
- Fill in the required details, such as the app name and description.
- Under "Auth," select "OAuth 2.0" as the authentication method.
- Specify the necessary scopes for accessing user data. For retrieving user information, ensure you include scopes like
crm.objects.contacts.read
. - Save your app and note down the client ID and client secret provided.
Generate an OAuth Access Token
With your app set up, you can now generate an OAuth access token to authenticate your API requests.
- Direct users to the authorization URL generated by your app to obtain an authorization code.
- Exchange the authorization code for an access token by making a POST request to HubSpot's token endpoint.
- Include the client ID, client secret, and authorization code in your request.
import requests
# Define the token endpoint and payload
token_url = "https://api.hubapi.com/oauth/v1/token"
payload = {
"grant_type": "authorization_code",
"client_id": "Your_Client_ID",
"client_secret": "Your_Client_Secret",
"redirect_uri": "Your_Redirect_URI",
"code": "Authorization_Code"
}
# Make the POST request to obtain the access token
response = requests.post(token_url, data=payload)
access_token = response.json().get("access_token")
print("Access Token:", access_token)
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, and Authorization_Code
with your actual app credentials and authorization code.
With your test account and OAuth credentials set up, you're ready to start making API calls to retrieve user data from HubSpot using Python.
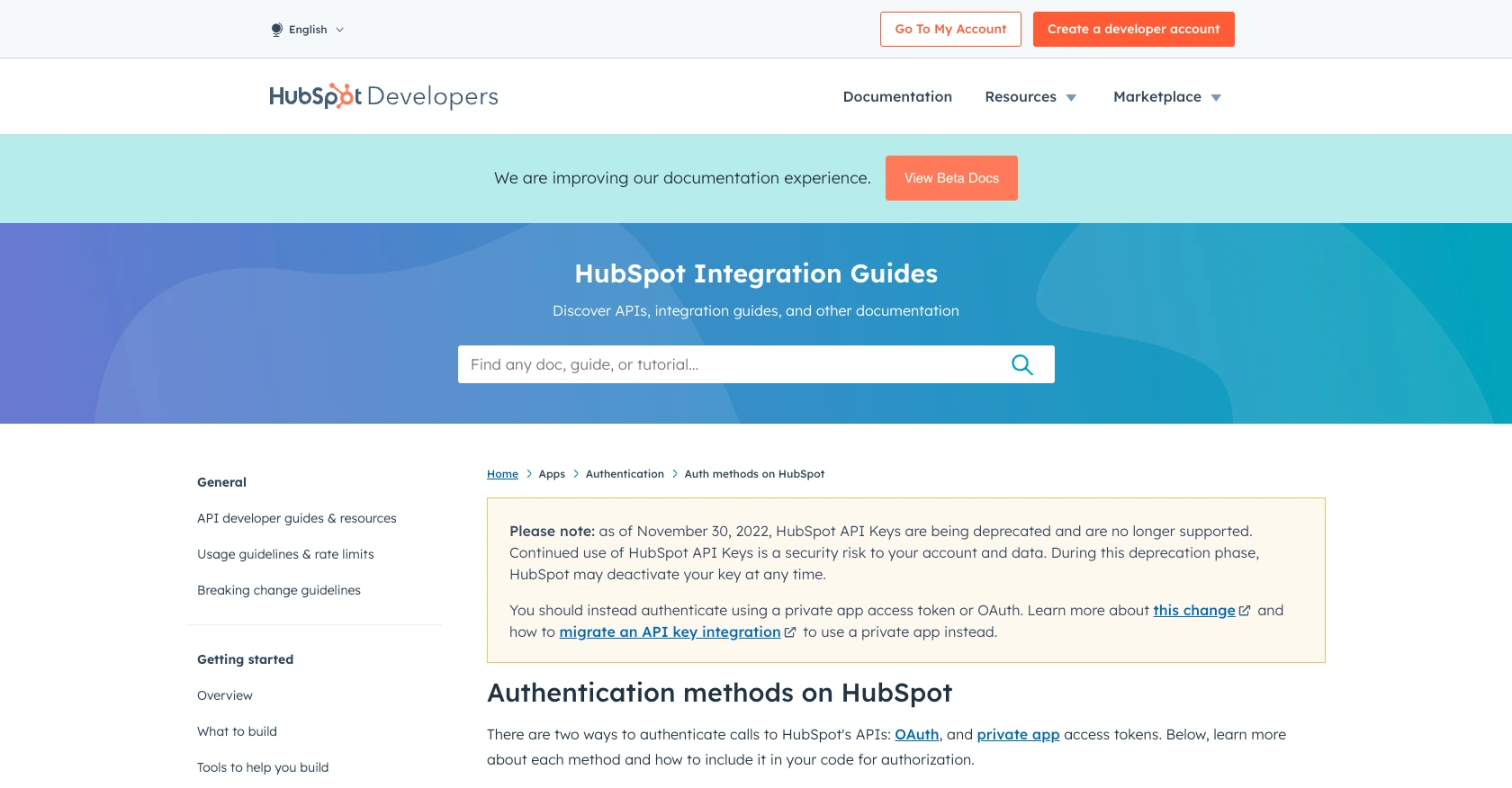
sbb-itb-96038d7
Making API Calls to Retrieve Users with HubSpot API in Python
To interact with the HubSpot API and retrieve user data, you'll need to use Python to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Python Environment for HubSpot API Integration
Before making API calls, ensure that your Python environment is correctly set up. You'll need Python 3.11.1 and the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Retrieve Users from HubSpot
With your environment ready, you can now write the Python code to make API calls to HubSpot and retrieve user data. Follow these steps to create a script that fetches user information.
import requests
# Define the API endpoint and headers
endpoint = "https://api.hubapi.com/crm/v3/objects/users"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
users = response.json().get("results", [])
for user in users:
print(f"User ID: {user['id']}, Created At: {user['createdAt']}")
else:
print(f"Failed to retrieve users: {response.status_code}")
Replace Your_Access_Token
with the OAuth access token obtained in the previous steps. This script makes a GET request to the HubSpot API to retrieve user data and prints out the user ID and creation date for each user.
Verifying API Call Success and Handling Errors
After running your script, you should verify that the API call was successful by checking the output and your HubSpot test account. If the request is successful, the user data should match the information in your test account.
In case of errors, the script will print the HTTP status code. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and has not expired.
- 429 Too Many Requests: You have exceeded the rate limit. HubSpot allows 100 requests every 10 seconds for OAuth apps. Consider implementing rate limiting in your application.
For more detailed error information, refer to the HubSpot Users API documentation.
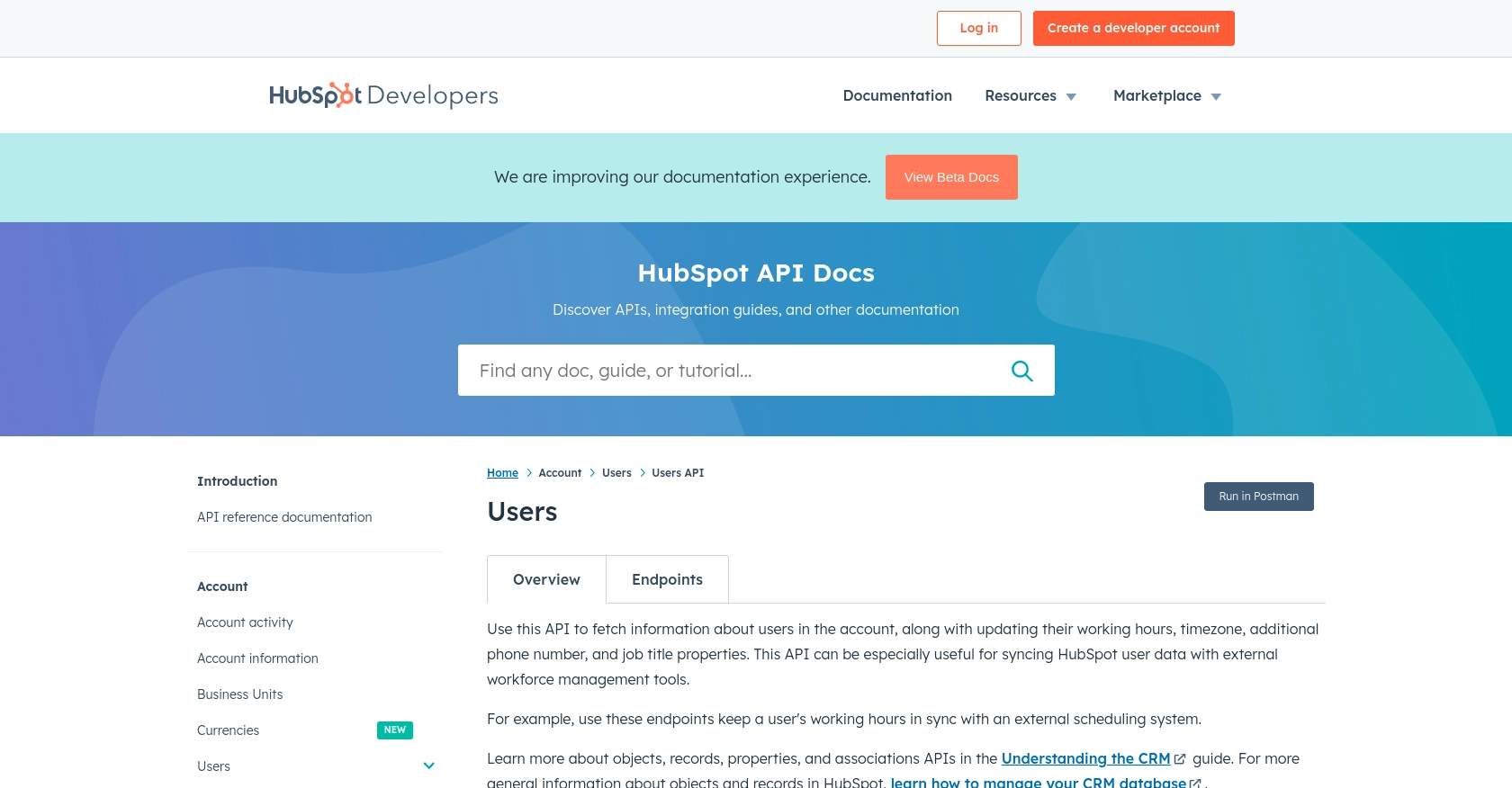
Conclusion and Best Practices for HubSpot API Integration Using Python
Integrating with the HubSpot API using Python allows developers to efficiently manage user data and synchronize it with external systems. By following the steps outlined in this article, you can set up a secure and effective connection to retrieve user information from HubSpot.
Best Practices for Secure and Efficient HubSpot API Integration
- Secure Storage of Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Implement Rate Limiting: HubSpot enforces a rate limit of 100 requests every 10 seconds for OAuth apps. Ensure your application handles rate limiting gracefully to avoid hitting these limits.
- Data Standardization: When retrieving user data, consider transforming and standardizing fields to maintain consistency across different systems.
- Error Handling: Implement robust error handling to manage common issues such as expired tokens or rate limit breaches. This will enhance the reliability of your integration.
Streamline Your Integrations with Endgrate
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?