How to Create Document Texts with the Google Docs API in Python
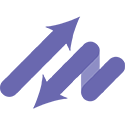
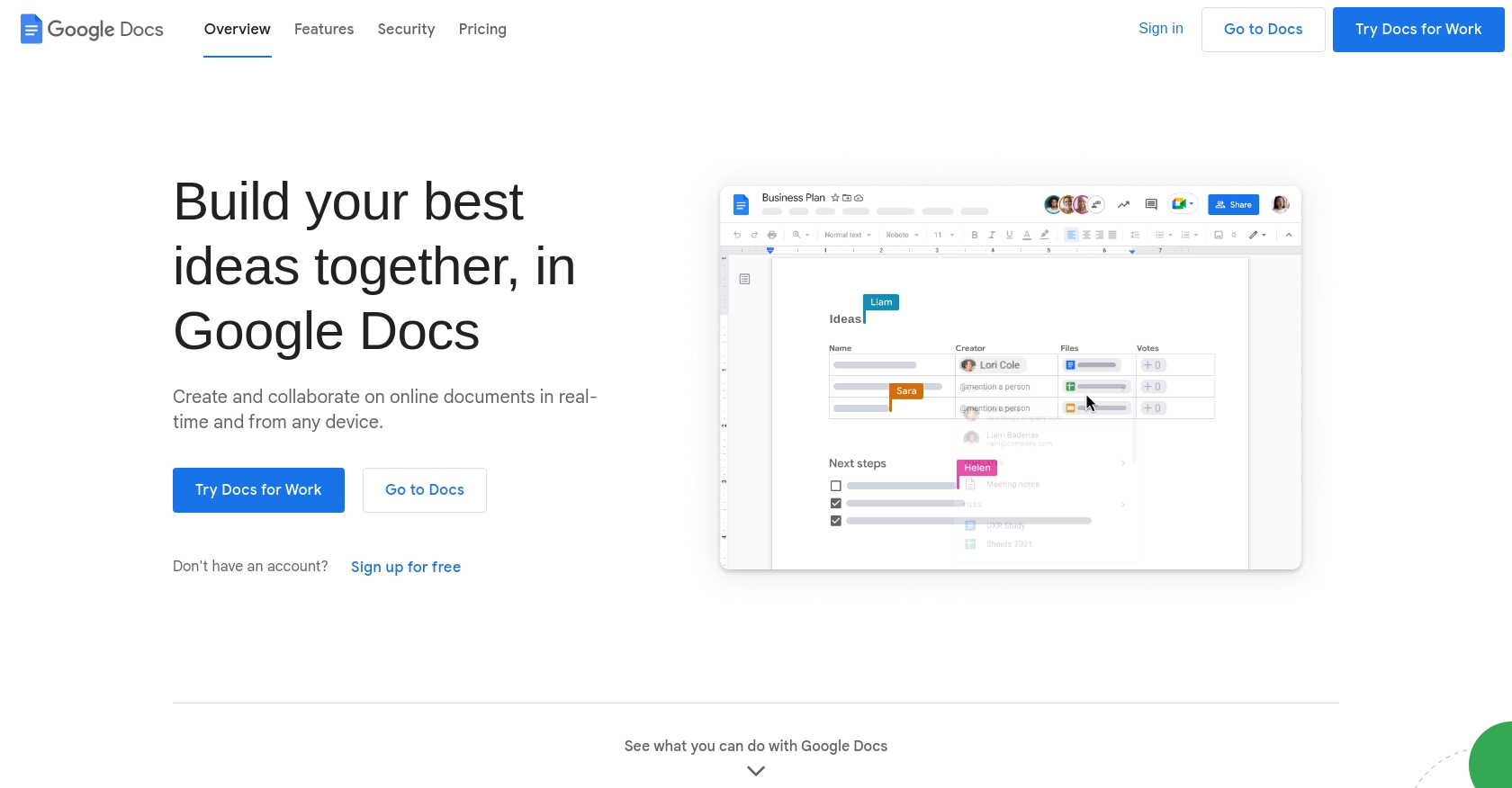
Introduction to Google Docs API
Google Docs is a widely used cloud-based word processing application that allows users to create, edit, and collaborate on documents in real-time. Its seamless integration with other Google Workspace tools makes it a preferred choice for businesses and individuals alike.
For developers, integrating with the Google Docs API opens up a world of possibilities for automating document creation and management. By leveraging this API, developers can programmatically create, edit, and format documents, enabling efficient workflows and enhanced productivity.
A practical use case for the Google Docs API is generating dynamic reports or documents from data stored in other applications. For example, a developer could automate the creation of monthly sales reports by pulling data from a database and formatting it into a structured document in Google Docs.
Setting Up Your Google Docs API Test Environment
Before you can start creating document texts with the Google Docs API, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows your application to interact securely with Google services.
Create a Google Cloud Project for Google Docs API
To begin, you need a Google Cloud project. Follow these steps to create one:
- Go to the Google Cloud Console.
- Click on the project dropdown and select "New Project."
- Enter a name for your project and click "Create."
Enable Google Docs API in Your Project
Once your project is created, you need to enable the Google Docs API:
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for "Google Docs API" and click on it.
- Click "Enable" to activate the API for your project.
For more details, refer to the Google Workspace API enabling guide.
Configure OAuth 2.0 Consent Screen
Next, configure the OAuth consent screen to define how your app requests access:
- Go to APIs & Services > OAuth consent screen in the Google Cloud Console.
- Select the user type for your app and click "Create."
- Fill out the app registration form and click "Save and Continue."
For more information, see the OAuth consent configuration guide.
Create OAuth 2.0 Credentials for Google Docs API
To authenticate requests, create OAuth 2.0 credentials:
- Navigate to APIs & Services > Credentials.
- Click "Create Credentials" and select "OAuth client ID."
- Choose "Desktop app" as the application type and click "Create."
- Note the Client ID and Client Secret, as you'll need them later.
For detailed steps, visit the Google Workspace credentials guide.
Install Required Python Libraries
Ensure you have Python installed on your machine. Then, install the necessary libraries:
pip install google-auth google-auth-oauthlib google-auth-httplib2 google-api-python-client
With your Google Cloud project set up and OAuth credentials ready, you're now prepared to start interacting with the Google Docs API using Python.
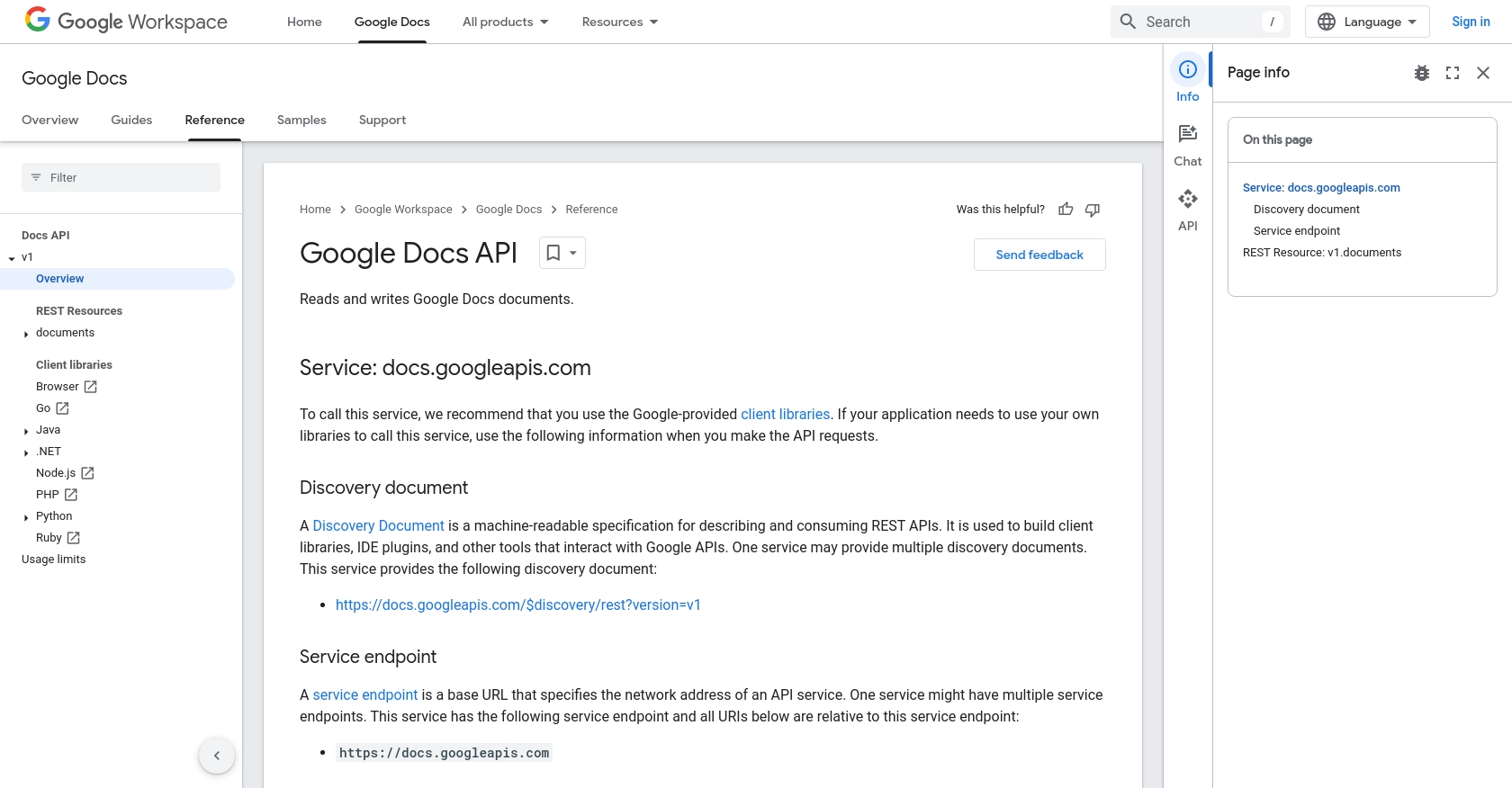
sbb-itb-96038d7
Making API Calls to Create Document Texts with Google Docs API in Python
With your Google Cloud project and OAuth credentials set up, you can now proceed to make API calls to create document texts using the Google Docs API in Python. This section will guide you through the necessary steps to achieve this, including setting up your Python environment and executing the API requests.
Setting Up Your Python Environment for Google Docs API
Before making API calls, ensure you have the correct version of Python installed. This tutorial uses Python 3.11.1. Additionally, verify that the required libraries are installed:
pip install google-auth google-auth-oauthlib google-auth-httplib2 google-api-python-client
Authenticating with Google Docs API Using OAuth 2.0
To interact with the Google Docs API, you need to authenticate your application using OAuth 2.0. The following code snippet demonstrates how to set up the authentication flow:
from google.oauth2.credentials import Credentials
from google_auth_oauthlib.flow import InstalledAppFlow
from google.auth.transport.requests import Request
import os.path
# Define the scopes
SCOPES = ['https://www.googleapis.com/auth/documents']
# Authenticate and get credentials
def authenticate():
creds = None
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials.json', SCOPES)
creds = flow.run_local_server(port=0)
with open('token.json', 'w') as token:
token.write(creds.to_json())
return creds
credentials = authenticate()
Creating a New Document with Google Docs API
Once authenticated, you can create a new document using the Google Docs API. The following code demonstrates how to create a blank document and retrieve its ID:
from googleapiclient.discovery import build
# Build the service
service = build('docs', 'v1', credentials=credentials)
# Create a new document
document = service.documents().create(body={'title': 'New Document'}).execute()
document_id = document.get('documentId')
print(f'Document created with ID: {document_id}')
Inserting Text into a Google Document
To insert text into the newly created document, use the batchUpdate
method with an InsertTextRequest
. Here’s how you can add text at a specific location:
# Define the requests for inserting text
requests = [
{
'insertText': {
'location': {
'index': 1,
},
'text': 'Hello, this is a sample text inserted into the document!'
}
}
]
# Execute the batch update
result = service.documents().batchUpdate(
documentId=document_id, body={'requests': requests}).execute()
print('Text inserted successfully')
Verifying the API Call Success
To verify that the text was successfully inserted, you can retrieve the document content and check the inserted text:
# Retrieve the document content
doc = service.documents().get(documentId=document_id).execute()
content = doc.get('body').get('content')
# Print the document content
for element in content:
if 'paragraph' in element:
text_run = element.get('paragraph').get('elements')[0].get('textRun')
if text_run:
print(text_run.get('content'))
Handling Errors and Troubleshooting
When making API calls, it's essential to handle potential errors. The Google Docs API may return various error codes, such as 400 for bad requests or 403 for permission errors. Implement error handling to manage these scenarios:
try:
# Your API call here
pass
except Exception as e:
print(f'An error occurred: {e}')
For more detailed information on error codes, refer to the Google Docs API documentation.
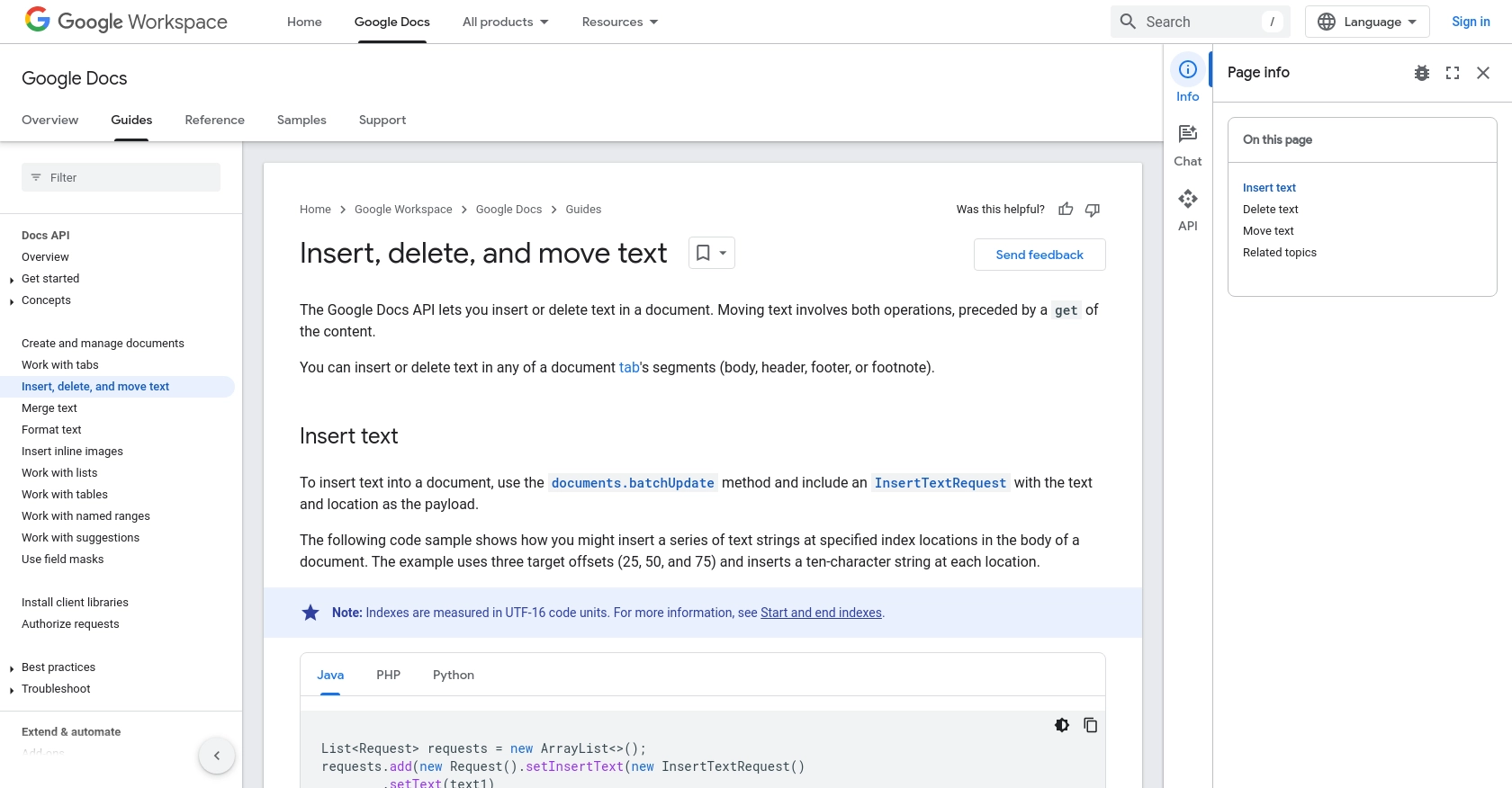
Best Practices for Using Google Docs API in Python
When working with the Google Docs API, it's crucial to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your application. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Google APIs have usage limits. Implement logic to handle rate limiting by checking response headers for rate limit information and using exponential backoff strategies for retries.
- Optimize API Calls: Minimize the number of API calls by batching requests when possible. This reduces latency and improves performance.
- Use Appropriate Scopes: Request only the necessary OAuth scopes to limit access and enhance security. Review the OAuth consent configuration guide for more details.
- Log and Monitor API Usage: Implement logging to track API usage and monitor for any unusual activity. This helps in troubleshooting and optimizing your integration.
Conclusion: Streamline Your Integrations with Endgrate
Integrating with the Google Docs API using Python allows developers to automate document creation and management, enhancing productivity and efficiency. By following the steps outlined in this guide, you can seamlessly create and manipulate Google Docs programmatically.
However, managing multiple integrations can be complex and time-consuming. That's where Endgrate comes in. With Endgrate, you can streamline your integration processes, saving time and resources. Focus on your core product while Endgrate handles the complexities of integration, providing a unified API experience for your customers.
Explore how Endgrate can simplify your integration needs and help you scale efficiently. Visit Endgrate to learn more and get started today.
Read More
- https://endgrate.com/provider/googledocs
- https://developers.google.com/docs/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/docs/api/how-tos/move-text
Ready to get started?