Using the Teamleader API to Create Or Update Contacts in Javascript
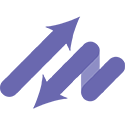
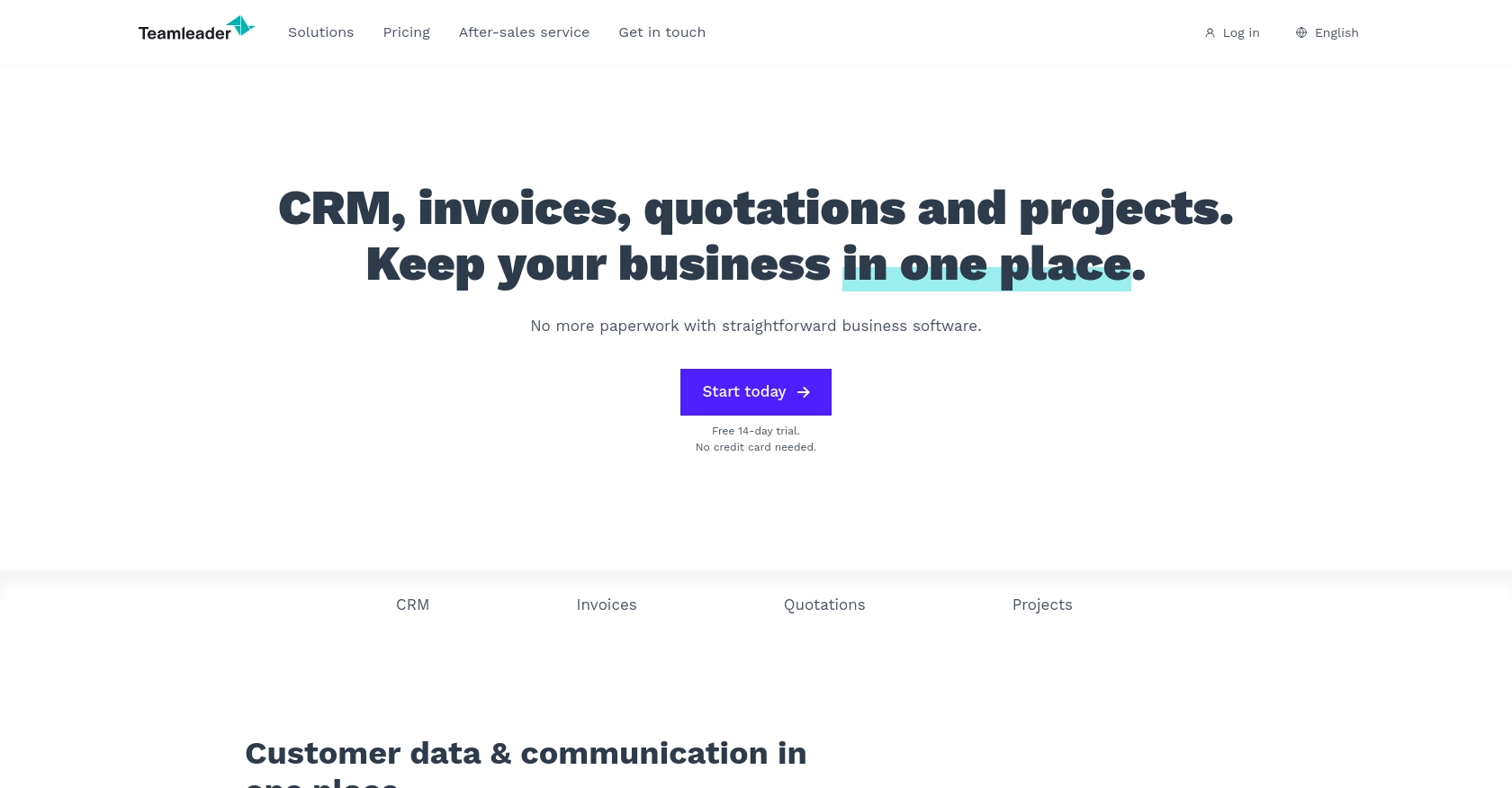
Introduction to Teamleader API Integration
Teamleader is a versatile business management software that helps companies streamline their operations by combining CRM, project management, and invoicing into one intuitive platform. It is designed to enhance productivity and efficiency for businesses of all sizes by providing a centralized hub for managing customer relationships, projects, and financials.
Integrating with the Teamleader API allows developers to automate and enhance business processes by interacting directly with the platform's features. For example, developers can use the API to create or update contact information, ensuring that customer data is always up-to-date and accessible across various systems. This can be particularly useful for businesses looking to synchronize their CRM data with other applications or services.
Setting Up Your Teamleader Test Account for API Integration
Before you can start integrating with the Teamleader API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data. Teamleader offers a free trial that developers can use to explore the platform's features and test API interactions.
Creating a Teamleader Account
To get started, visit the Teamleader website and sign up for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is created, you'll have access to the Teamleader dashboard, where you can manage your settings and data.
Setting Up OAuth Authentication for Teamleader API
The Teamleader API uses OAuth 2.0 for authentication, which requires you to create an app within your Teamleader account. This app will provide you with the necessary credentials to authenticate API requests.
- Log in to your Teamleader account and navigate to the "Integrations" section.
- Select "Create a new app" and fill in the required details, such as the app name and description.
- Once the app is created, you'll receive a client ID and client secret. Make sure to store these securely, as you'll need them to authenticate your API requests.
- Configure the redirect URI for your app. This is the URL where users will be redirected after they authorize your app.
Obtaining Access Tokens for API Requests
With your app set up, you can now obtain access tokens to authenticate your API requests. Follow these steps to generate an access token:
- Direct users to the Teamleader authorization URL, including your client ID and redirect URI as query parameters.
- After users authorize your app, they will be redirected to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to the Teamleader token endpoint, including your client ID, client secret, and authorization code.
- Store the access token securely, as it will be used to authenticate your API requests.
For more detailed information on OAuth authentication, refer to the Teamleader API Documentation.
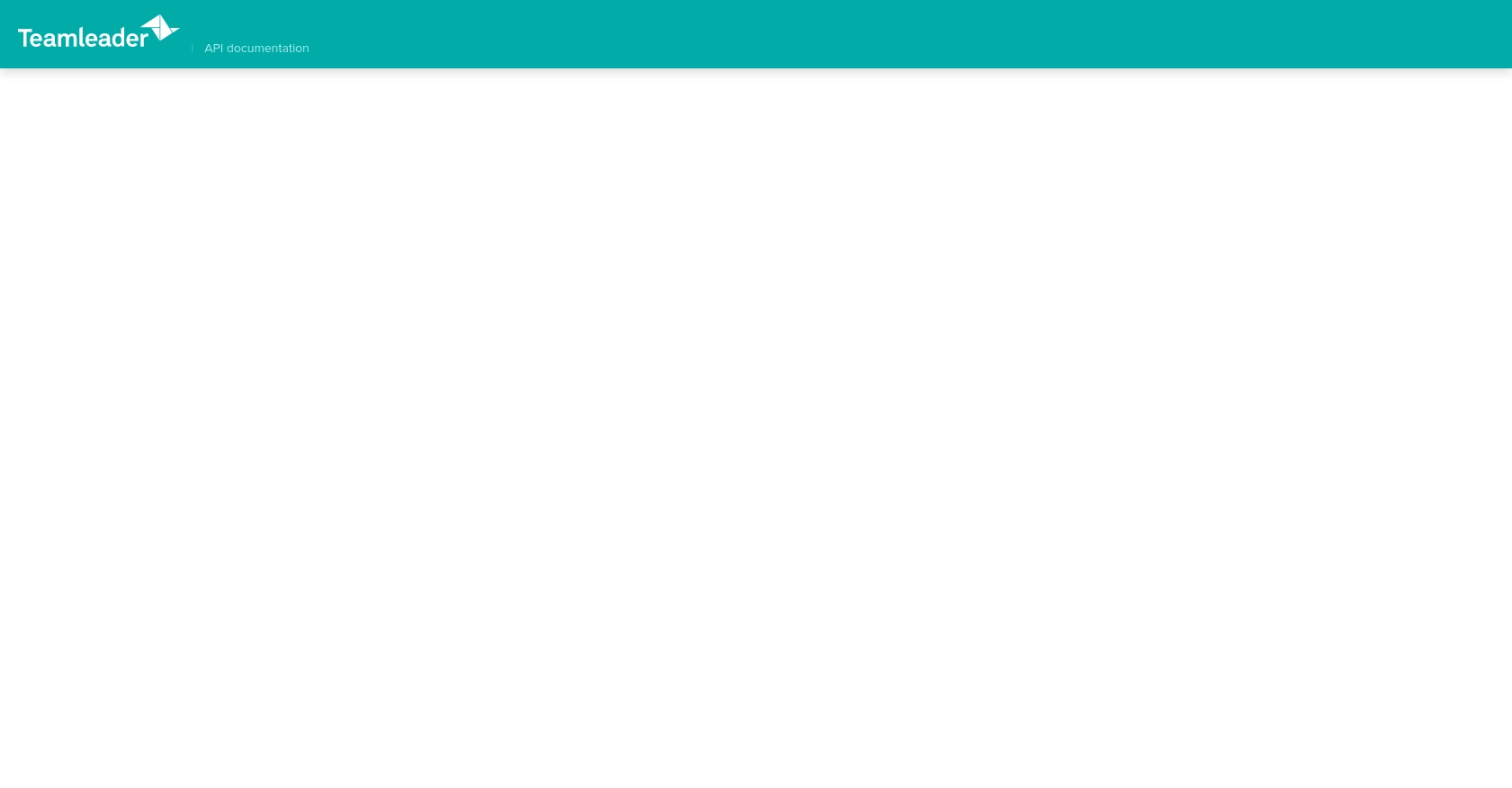
sbb-itb-96038d7
Making API Calls with Teamleader Using JavaScript
To interact with the Teamleader API using JavaScript, you need to ensure you have the necessary environment set up. This section will guide you through the process of making API calls to create or update contacts in Teamleader.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side operations like API requests. You can download Node.js from the official website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the required dependencies. For this tutorial, we'll use the axios
library to handle HTTP requests. Run the following command in your terminal to install axios:
npm install axios
Creating or Updating Contacts with Teamleader API
With the environment set up, you can now create a JavaScript file to interact with the Teamleader API. Follow these steps to create or update contacts:
const axios = require('axios');
// Replace with your actual access token
const accessToken = 'Your_Access_Token';
// Function to create or update a contact
async function createOrUpdateContact(contactData) {
try {
const response = await axios.post('https://api.teamleader.eu/contacts.createOrUpdate', contactData, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Contact created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating contact:', error.response ? error.response.data : error.message);
}
}
// Sample contact data
const contactData = {
first_name: 'John',
last_name: 'Doe',
email: 'john.doe@example.com'
};
// Call the function
createOrUpdateContact(contactData);
In the code above, replace Your_Access_Token
with the access token obtained during the OAuth authentication process. The createOrUpdateContact
function sends a POST request to the Teamleader API endpoint to create or update a contact. The contact data is passed as a JSON object, and the response is logged to the console.
Verifying API Call Success and Handling Errors
After running the code, you should verify the success of the API call by checking the response data. If the contact is created or updated successfully, the response will contain the contact details. In case of an error, the error message will be logged to the console.
Common error codes include:
- 401 Unauthorized: Check if the access token is valid and not expired.
- 400 Bad Request: Ensure that the contact data is correctly formatted and all required fields are included.
For more detailed error information, refer to the Teamleader API Documentation.
Conclusion and Best Practices for Teamleader API Integration
Integrating with the Teamleader API using JavaScript can significantly enhance your business operations by automating contact management and ensuring data consistency across platforms. By following the steps outlined in this guide, you can efficiently create or update contacts, leveraging the power of Teamleader's comprehensive CRM capabilities.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handling Rate Limits: Be aware of any rate limits imposed by the Teamleader API to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Validation and Error Handling: Validate contact data before making API requests to minimize errors. Implement robust error handling to manage API response errors effectively.
- Regular Token Refresh: Ensure that your access tokens are refreshed regularly to maintain uninterrupted API access. Monitor token expiration and automate the refresh process if possible.
Streamlining Integrations with Endgrate
While integrating with the Teamleader API offers numerous benefits, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Teamleader. By using Endgrate, you can focus on your core product development while outsourcing integration management, saving time and resources.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover an intuitive integration experience for your customers.
Read More
Ready to get started?