Using the Sage 100 API to Create or Update Sales Orders in Python
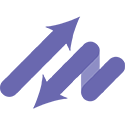
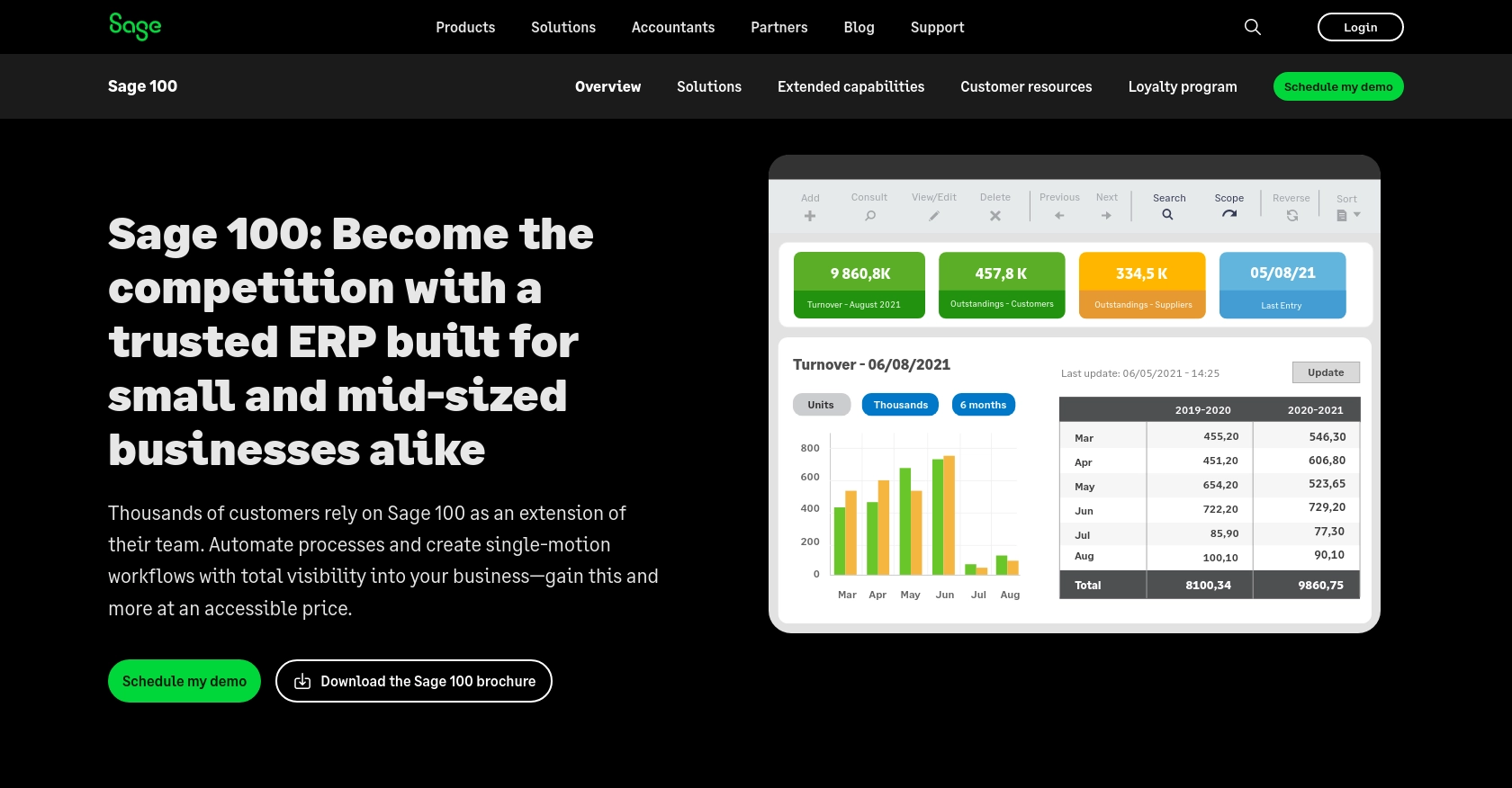
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution designed to streamline business operations, including accounting, inventory management, and sales order processing. It is widely used by small to medium-sized businesses to enhance efficiency and accuracy in their financial and operational workflows.
Integrating with the Sage 100 API allows developers to automate and manage sales orders effectively. For example, a developer might use the Sage 100 API to create or update sales orders directly from an e-commerce platform, ensuring seamless order processing and inventory updates.
Setting Up a Test Environment for Sage 100 API Integration
Before you can begin integrating with the Sage 100 API, it's essential to set up a test environment. This allows you to safely experiment with API calls without affecting live data. Sage 100 provides a robust ODBC driver that facilitates this integration process.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, you must first ensure that the Sage 100 ODBC driver is installed and configured correctly. Follow these steps to set up the ODBC driver:
- Access the ODBC Data Source Administrator on your system.
- Create a new DSN (Data Source Name) that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Refer to the official Sage 100 documentation for detailed instructions on configuring the ODBC driver.
Creating a DSN for Sage 100
Once the ODBC driver is installed, you need to create a DSN to establish a connection to the Sage 100 database:
- Open the ODBC Data Source Administrator.
- Navigate to the User DSN or System DSN tab and click on "Add."
- Select the Sage 100 ODBC driver from the list and click "Finish."
- Enter the DSN name and description, and provide the server and database details.
- Test the connection to ensure it is configured correctly.
Setting Up Authentication for Sage 100 API
Sage 100 uses a custom authentication method. Ensure that you have the necessary credentials and permissions to access the API:
- Verify that your user account has the appropriate access rights within the Sage 100 system.
- Ensure that the ODBC connection is configured to use the correct authentication settings.
With the test environment set up, you are now ready to start making API calls to create or update sales orders using Python. This setup ensures that you can safely test your integration without impacting live operations.
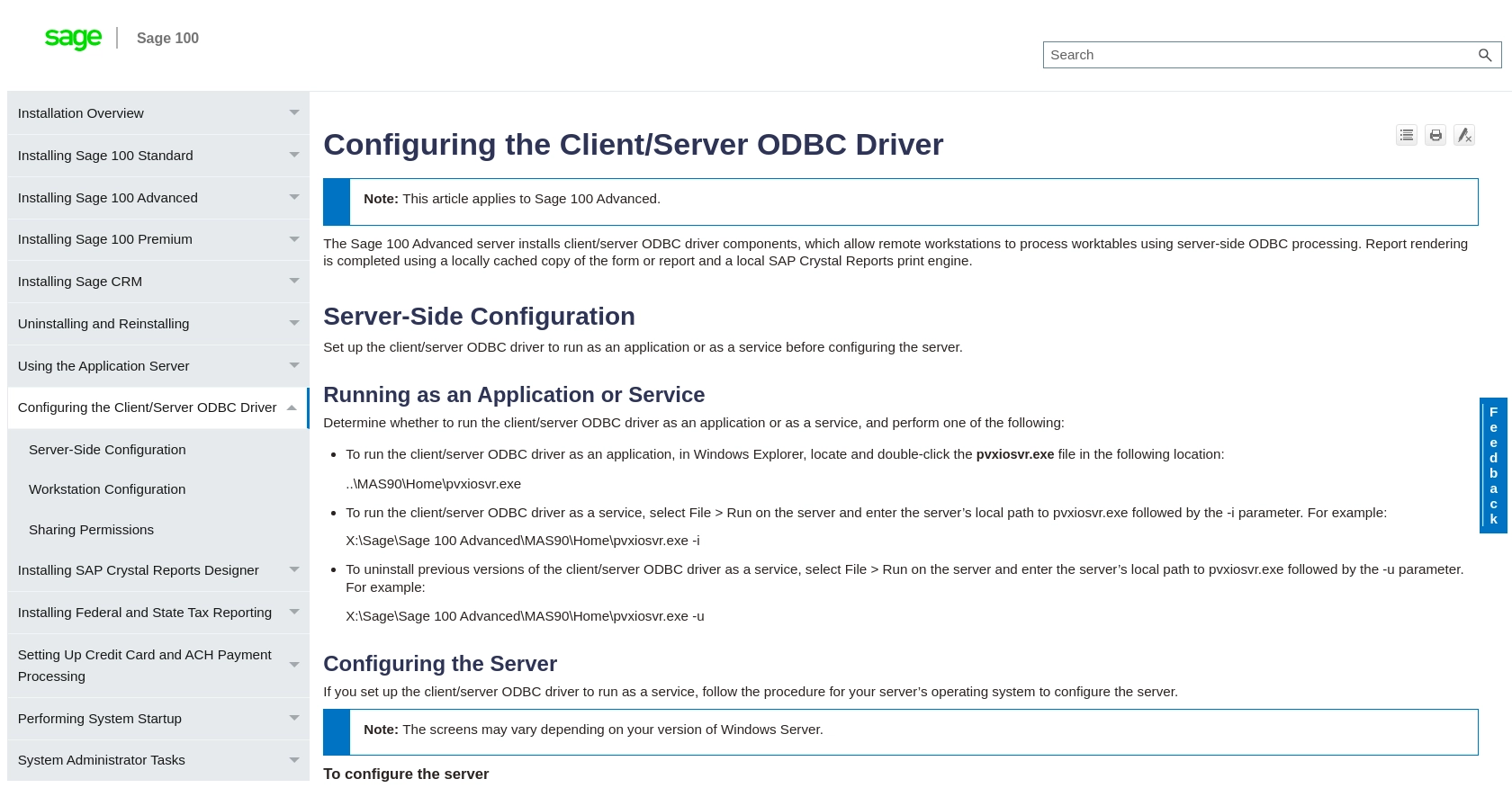
sbb-itb-96038d7
Making API Calls to Create or Update Sales Orders in Sage 100 Using Python
To interact with the Sage 100 API and manage sales orders, you'll need to use Python to establish a connection and execute the necessary API calls. This section will guide you through setting up your Python environment, writing the code to create or update sales orders, and handling potential errors.
Setting Up Your Python Environment for Sage 100 API Integration
Before you begin, ensure that you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the pyodbc
library to facilitate ODBC connections.
pip install pyodbc
Writing Python Code to Create or Update Sales Orders in Sage 100
Once your environment is set up, you can write the Python code to interact with the Sage 100 API. Below is a sample script to create or update sales orders:
import pyodbc
# Define the DSN and connection parameters
dsn = 'Your_DSN_Name'
user = 'Your_Username'
password = 'Your_Password'
# Establish a connection to the Sage 100 database
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
# Create a cursor object to execute SQL queries
cursor = connection.cursor()
# Define the SQL query to create or update a sales order
sql_query = """
INSERT INTO SO_SalesOrderHeader (SalesOrderNo, CustomerNo, OrderDate)
VALUES (?, ?, ?)
ON DUPLICATE KEY UPDATE
CustomerNo = VALUES(CustomerNo),
OrderDate = VALUES(OrderDate);
"""
# Define the sales order data
sales_order_data = ('SO12345', 'CUST001', '2023-10-01')
# Execute the query
cursor.execute(sql_query, sales_order_data)
# Commit the transaction
connection.commit()
# Close the connection
cursor.close()
connection.close()
print("Sales order created or updated successfully.")
In this script, replace Your_DSN_Name
, Your_Username
, and Your_Password
with your actual DSN, username, and password. The script connects to the Sage 100 database, executes an SQL query to create or update a sales order, and commits the transaction.
Verifying Successful API Calls and Handling Errors
After running the script, you can verify the success of the API call by checking the Sage 100 database for the updated sales order. If the order appears as expected, the integration is successful.
To handle errors, wrap your code in a try-except block to catch exceptions and log error messages. This will help you identify issues such as connection failures or SQL syntax errors.
try:
# Your code here
except pyodbc.Error as e:
print("Error occurred:", e)
By following these steps, you can efficiently create or update sales orders in Sage 100 using Python, ensuring seamless integration with your business processes.
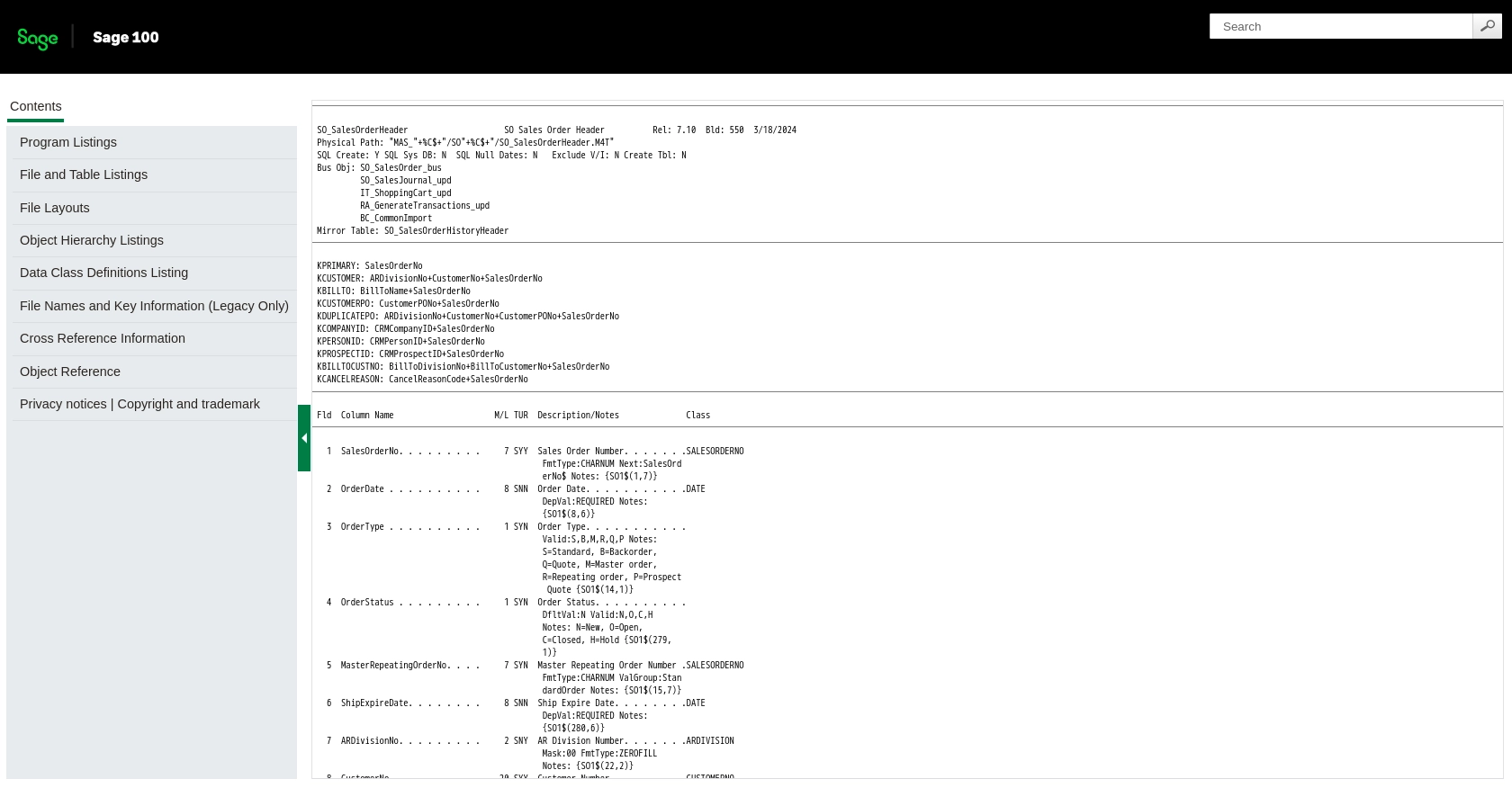
Conclusion and Best Practices for Sage 100 API Integration
Integrating with the Sage 100 API to create or update sales orders using Python can significantly enhance your business operations by automating order processing and ensuring data consistency across platforms. By following the steps outlined in this guide, you can efficiently set up your environment, execute API calls, and handle potential errors.
Best Practices for Secure and Efficient Sage 100 API Usage
- Secure Credentials: Always store your DSN, username, and password securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Validation: Validate all data before sending it to the API to prevent errors and ensure data integrity.
- Error Logging: Implement comprehensive logging to capture and analyze errors, which can help in troubleshooting and improving your integration.
Enhance Your Integration Strategy with Endgrate
While integrating with Sage 100 directly can be effective, using a tool like Endgrate can further streamline your integration process. Endgrate provides a unified API endpoint that connects to multiple platforms, allowing you to manage various integrations with ease. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover a more efficient way to manage your business integrations.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderHeader.htm?Highlight=SO_SalesOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderDetail.htm?Highlight=SO_SalesOrderDetail
Ready to get started?