Using the Insightly API to Create or Update Organizations in Python
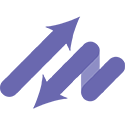
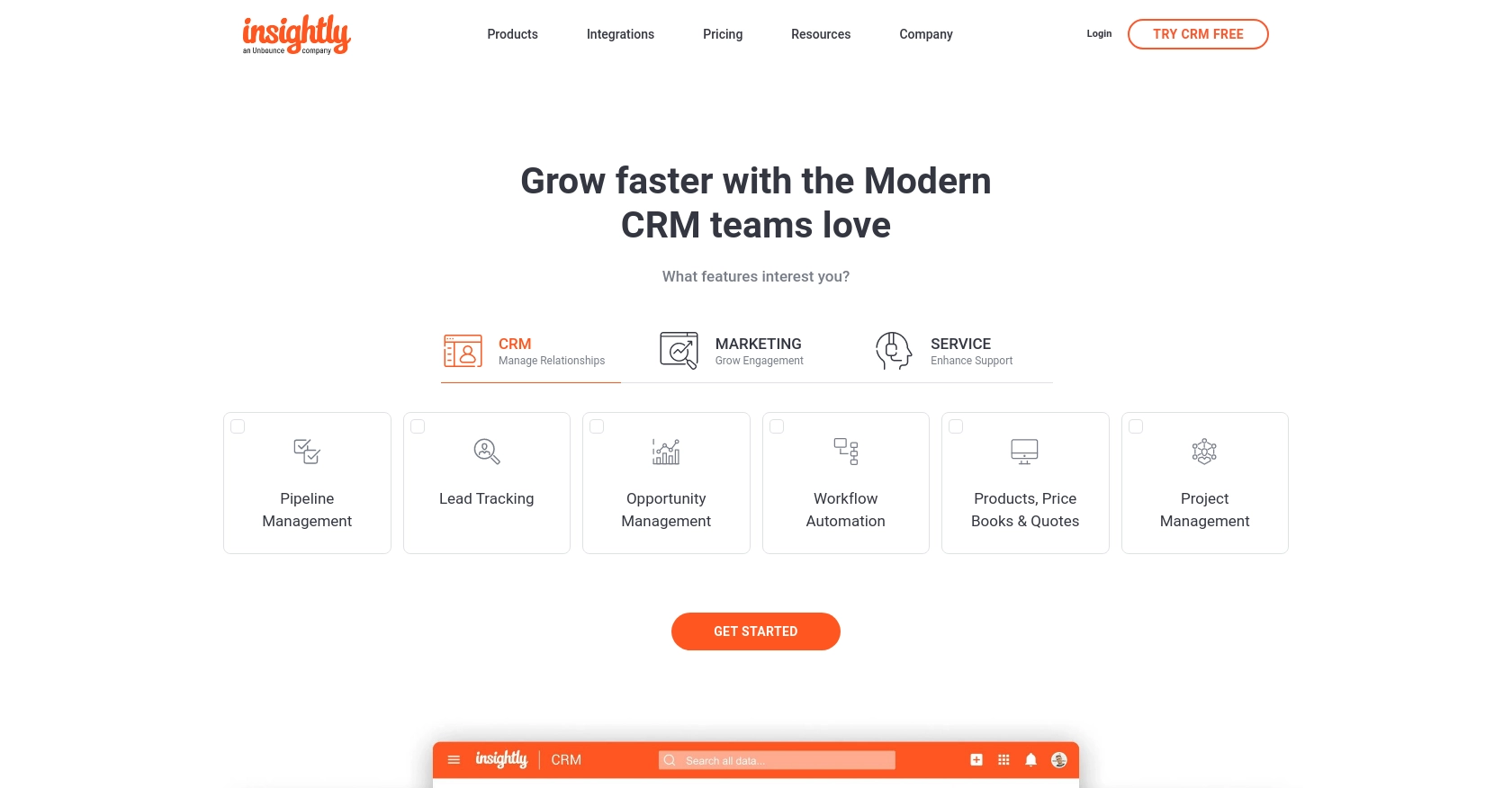
Introduction to Insightly CRM Integration
Insightly is a powerful CRM platform that offers a suite of tools to help businesses manage customer relationships, projects, and sales pipelines. With its robust API, developers can seamlessly integrate Insightly with other applications, enhancing productivity and data management capabilities.
Connecting with the Insightly API allows developers to automate and streamline various business processes. For example, you might want to create or update organization records in Insightly using Python, enabling real-time synchronization of company data across multiple platforms.
This article will guide you through using the Insightly API to efficiently create or update organizations in Python, providing step-by-step instructions and code examples to simplify the integration process.
Setting Up Your Insightly Test Account
Before you can start integrating with the Insightly API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on the Insightly website. Follow the on-screen instructions to complete the registration process.
Accessing Your API Key
Once your account is set up, you'll need to obtain your API key to authenticate your requests. Here's how to find it:
- Log in to your Insightly account.
- Click on your user profile in the upper right corner and select User Settings.
- Navigate to the API Key and URL section.
- Copy your API key from this section. This key will be used to authenticate your API requests.
For more detailed instructions, you can refer to the Insightly support article.
Understanding Insightly API Authentication
Insightly uses HTTP Basic authentication, requiring you to include your API key as the Base64-encoded username, leaving the password blank. This ensures that your API calls are securely authenticated.
For testing purposes, you can paste your API key directly into the API key field without Base64 encoding when using the sandbox environment.
For more information, visit the Insightly API documentation.
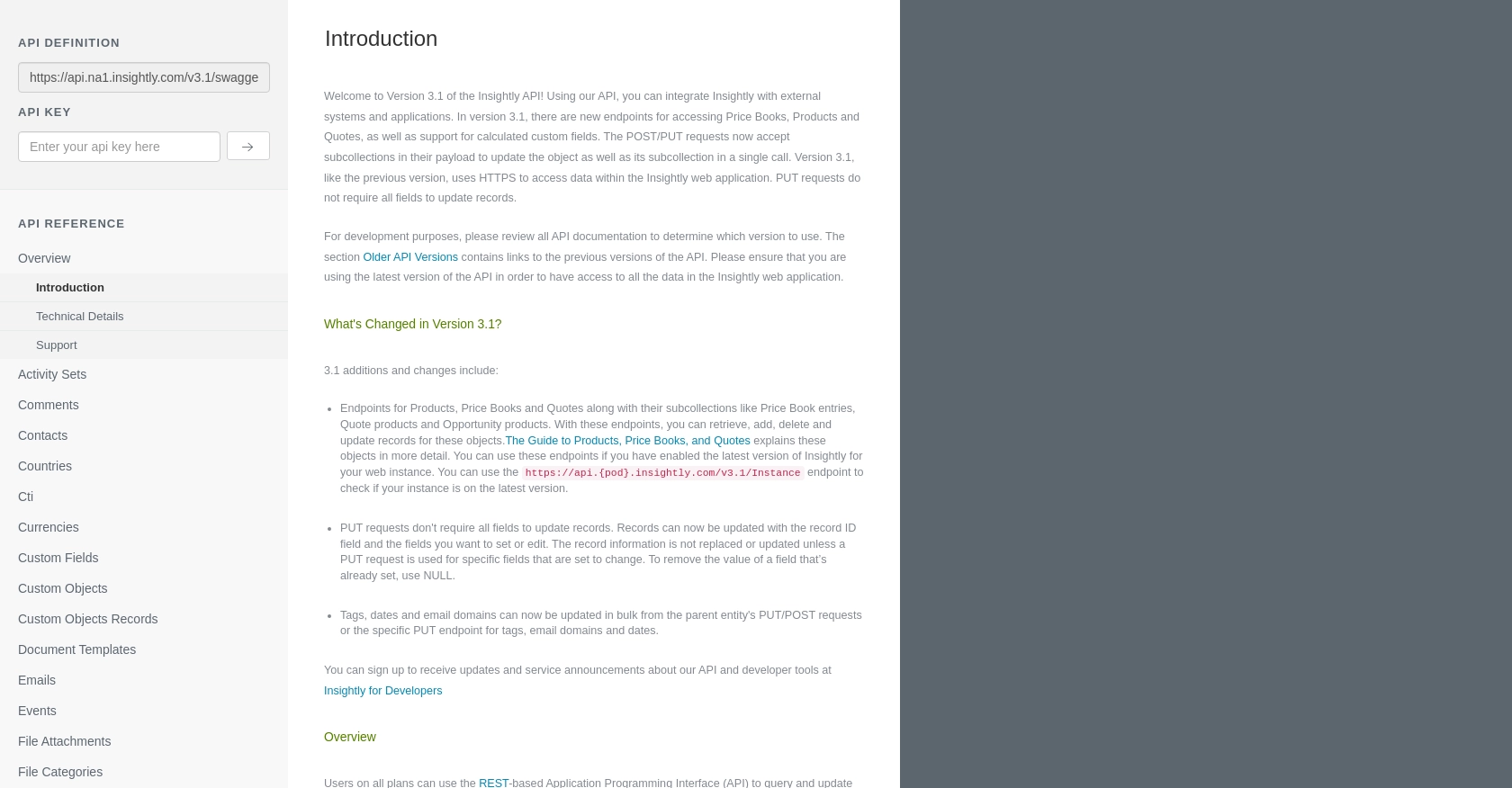
sbb-itb-96038d7
Making API Calls to Create or Update Organizations in Insightly Using Python
To interact with the Insightly API and manage organization records, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Insightly API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You'll also need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Install the
requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Create or Update Organizations in Insightly
Now that your environment is set up, you can write the Python script to create or update organizations in Insightly. Here's a step-by-step guide:
import requests
import base64
# Set your Insightly API key
api_key = 'Your_API_Key'
# Encode the API key for HTTP Basic Authentication
encoded_api_key = base64.b64encode(api_key.encode()).decode()
# Define the API endpoint for organizations
url = 'https://api.na1.insightly.com/v3.1/Organisations'
# Set the headers for the request
headers = {
'Authorization': f'Basic {encoded_api_key}',
'Content-Type': 'application/json'
}
# Define the organization data
organization_data = {
'ORGANISATION_NAME': 'New Organization',
'CUSTOMFIELDS': [
{
'FIELD_NAME': 'Custom_Field_Name',
'FIELD_VALUE': 'Custom Value'
}
]
}
# Make a POST request to create a new organization
response = requests.post(url, json=organization_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print('Organization created successfully:', response.json())
else:
print('Failed to create organization:', response.status_code, response.text)
Replace Your_API_Key
with your actual Insightly API key. This script sends a POST request to the Insightly API to create a new organization. If you need to update an existing organization, use a PUT request instead, and include the organization's ID in the URL.
Verifying API Call Success and Handling Errors
After running the script, you should verify that the organization was created or updated successfully in your Insightly test account. Check the response from the API call for confirmation.
If the API call fails, the response will include an error code. Common error codes include:
- 401 Unauthorized: Check your API key and ensure it is correctly encoded.
- 429 Too Many Requests: You have exceeded the rate limit. Wait and try again later.
For more details on error codes, refer to the Insightly API documentation.
Conclusion and Best Practices for Insightly API Integration
Integrating with the Insightly API to create or update organizations in Python can significantly enhance your business processes by automating data management tasks. By following the steps outlined in this guide, you can efficiently manage organization records within Insightly, ensuring real-time data synchronization across platforms.
Best Practices for Secure and Efficient Insightly API Usage
- Secure Storage of API Keys: Always store your Insightly API key securely. Consider using environment variables or a secure vault to keep your credentials safe.
- Handling Rate Limits: Be mindful of Insightly's rate limits, which allow up to 10 requests per second and vary daily based on your plan. Implement error handling to manage HTTP 429 status codes and retry requests after a delay.
- Data Standardization: Ensure that data fields are standardized and consistent across your applications to facilitate seamless integration and data accuracy.
Enhance Your Integration Strategy with Endgrate
While integrating with Insightly directly can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Insightly. This allows you to focus on your core product while outsourcing integration management.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can simplify your integration strategy and enhance your business operations.
Read More
Ready to get started?