How to Create or Update People with the Copper API in PHP
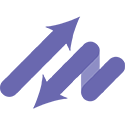
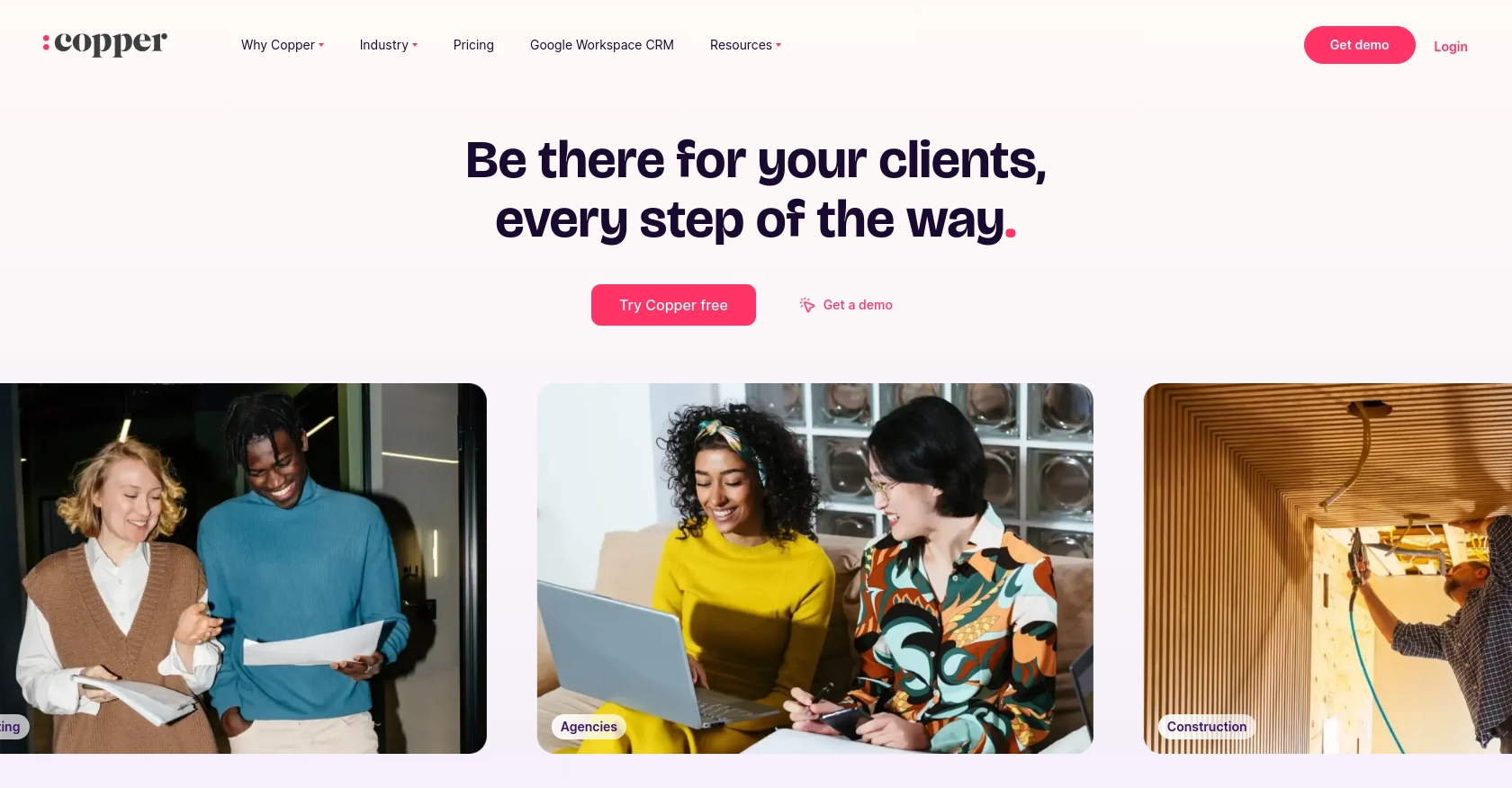
Introduction to Copper API for People Management
Copper is a powerful CRM platform that integrates seamlessly with G Suite, providing businesses with a streamlined way to manage customer relationships. Its intuitive interface and robust features make it an ideal choice for companies looking to enhance their CRM capabilities.
Developers may want to integrate with Copper's API to automate and manage customer data efficiently. For example, using the Copper API, you can create or update people records directly from your application, ensuring that your CRM data is always up-to-date and accurate.
This article will guide you through the process of creating or updating people using the Copper API in PHP, providing step-by-step instructions and code examples to help you get started quickly and effectively.
Setting Up Your Copper API Test or Sandbox Account
Before you can start creating or updating people with the Copper API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your live data.
Creating a Copper Account
If you don't already have a Copper account, you can sign up for a free trial on the Copper website. This will give you access to the necessary tools and features to begin integrating with the Copper API.
- Visit the Copper signup page.
- Follow the instructions to create your account.
- Once your account is created, log in to access the Copper dashboard.
Generating API Keys for Copper Integration
To interact with the Copper API, you'll need to generate API keys. These keys will allow your application to authenticate and communicate with Copper's services.
- Navigate to the Copper dashboard and click on your profile icon.
- Select Settings from the dropdown menu.
- Under the Integrations section, find and click on API Keys.
- Click on Create API Key and fill in the required information.
- Copy the generated API key and secret. Store them securely as you'll need them for authentication.
Configuring OAuth for Copper API Access
Copper uses OAuth 2.0 for authentication, which requires setting up an application within your Copper account.
- In the Copper dashboard, go to Settings and select Integrations.
- Click on OAuth Apps and then Create OAuth App.
- Provide a name and description for your app.
- Enter the redirect URI, which is the URL where users will be redirected after authentication.
- Save the app to generate a client ID and client secret.
- Use these credentials to authenticate API requests.
Testing Your Copper API Setup
With your API keys and OAuth app configured, you can now test your setup by making a simple API call to ensure everything is working correctly.
// Example PHP code to test Copper API connection
$apiKey = 'your_api_key';
$clientSecret = 'your_client_secret';
$endpoint = 'https://api.copper.com/v1/people';
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Close cURL session
curl_close($ch);
// Output the response
echo $response;
By following these steps, you'll have a fully configured test environment to begin integrating with the Copper API in PHP.
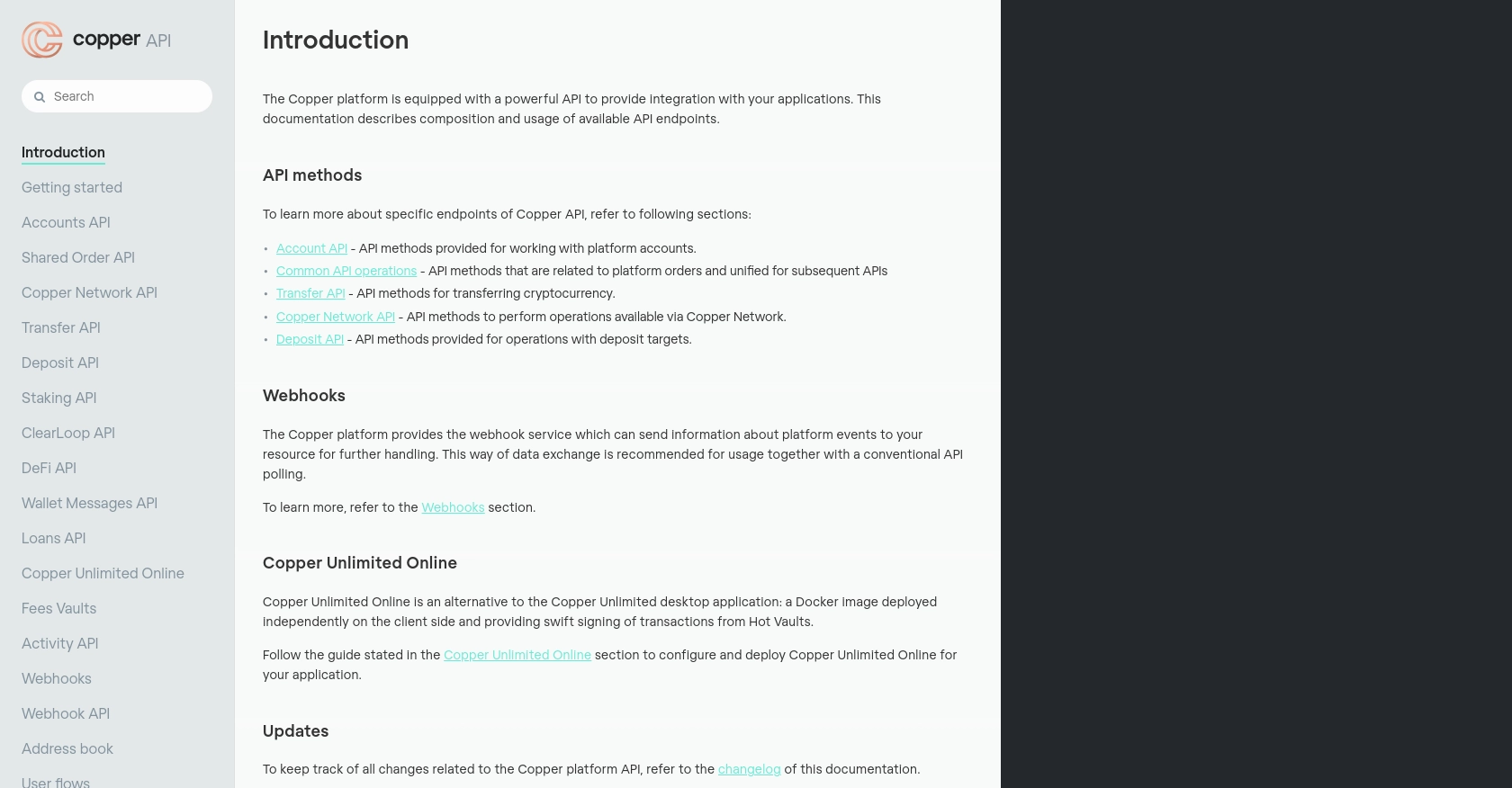
sbb-itb-96038d7
Making API Calls to Create or Update People with Copper API in PHP
To effectively interact with the Copper API using PHP, you'll need to ensure your environment is set up correctly. This involves using the right PHP version and installing necessary dependencies.
Setting Up PHP Environment for Copper API Integration
Ensure you have PHP 7.4 or later installed on your system. You will also need the cURL
extension enabled to make HTTP requests.
- Check your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file.
Installing Required PHP Dependencies for Copper API
Use Composer, a dependency manager for PHP, to install any required libraries. If you haven't installed Composer yet, you can do so by following the instructions on the Composer website.
composer require guzzlehttp/guzzle
This command will install Guzzle, a PHP HTTP client that simplifies making HTTP requests.
Example Code to Create or Update People Using Copper API
Below is a sample PHP script to create or update a person in Copper using the API. Replace your_api_key
and your_client_secret
with your actual API credentials.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'your_api_key';
$clientSecret = 'your_client_secret';
$endpoint = 'https://api.copper.com/v1/people';
$data = [
'name' => 'John Doe',
'email' => 'john.doe@example.com',
'phone_number' => '123-456-7890'
];
$response = $client->post($endpoint, [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Content-Type' => 'application/json'
],
'json' => $data
]);
echo $response->getBody();
This script initializes a Guzzle client, sets the necessary headers for authentication, and sends a POST request to the Copper API to create or update a person. The response from the API is then printed to the console.
Verifying Successful API Requests in Copper
After executing the script, verify that the person was successfully created or updated by checking your Copper dashboard. If the request was successful, the new or updated person should appear in your list of people.
Handling Errors and API Response Codes
It's important to handle potential errors when making API calls. Copper API may return various HTTP status codes indicating the success or failure of your request. Here are some common codes:
- 200 OK: The request was successful.
- 400 Bad Request: The request was malformed or missing required parameters.
- 401 Unauthorized: Authentication failed. Check your API key and secret.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
Implement error handling in your code to manage these responses appropriately.
Best Practices for Using Copper API in PHP
When working with the Copper API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Secure API Credentials: Always store your API key and client secret securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Copper API has a rate limit of 30000 requests per 5 minutes. Implement logic to handle HTTP 429 errors and retry requests after a delay.
- Validate API Responses: Always check the response status codes and handle errors appropriately to ensure your application can recover gracefully from failures.
- Data Standardization: Ensure that data fields are consistent and standardized before sending them to the API to avoid validation errors.
Streamlining Copper API Integrations with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Copper. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Deploy Anywhere: Create a single integration that works across multiple platforms, reducing development overhead.
- Enhance User Experience: Provide your users with a seamless and intuitive integration experience.
Visit Endgrate to learn more about how you can optimize your integration strategy.
Read More
Ready to get started?