How to Get Companies with the Pipeliner CRM API in Python
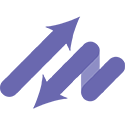
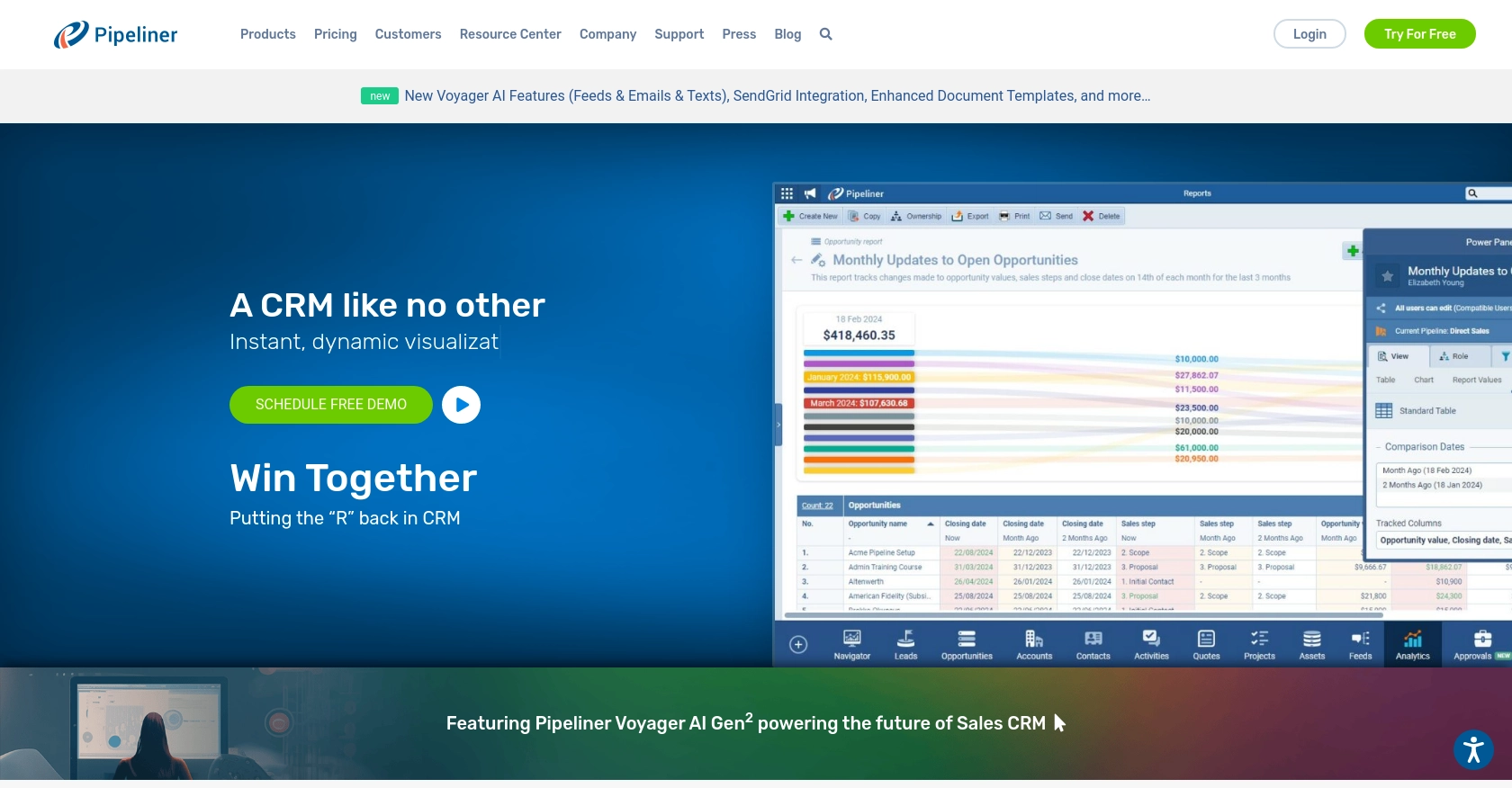
Introduction to Pipeliner CRM
Pipeliner CRM is a robust customer relationship management platform designed to enhance sales processes and improve business efficiency. It offers a comprehensive suite of tools for managing leads, opportunities, and customer interactions, making it an ideal choice for businesses aiming to streamline their sales operations.
Integrating with the Pipeliner CRM API allows developers to access and manage company data programmatically, enabling automation and customization of sales workflows. For example, a developer might use the Pipeliner CRM API to retrieve a list of companies and integrate this data into a custom reporting tool, providing sales teams with valuable insights into their client base.
Setting Up Your Pipeliner CRM Test Account
Before you can start interacting with the Pipeliner CRM API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Pipeliner CRM Account
If you don't already have a Pipeliner CRM account, follow these steps to create one:
- Visit the Pipeliner CRM website and sign up for an account.
- Once registered, log in to your Pipeliner CRM account.
Generating Pipeliner CRM API Keys for Authentication
Pipeliner CRM uses Basic authentication, which requires a username and password generated as API keys. Follow these steps to obtain them:
- Log in to your Pipeliner CRM account and select your team space.
- Navigate to the administration section.
- Under the "Unit, Users & Roles" tab, open "Applications."
- Create a new application.
- Click "Show API Access" to view your API username and password.
- Store these credentials securely, as they are generated only once.
These credentials will be used for authenticating your API requests.
Understanding Pipeliner CRM API URL Components
Each API request requires a Space ID and Service URL, which are part of the request URL. Ensure you have these details ready when making API calls.
With your test account and API keys set up, you're ready to start making API calls to Pipeliner CRM.
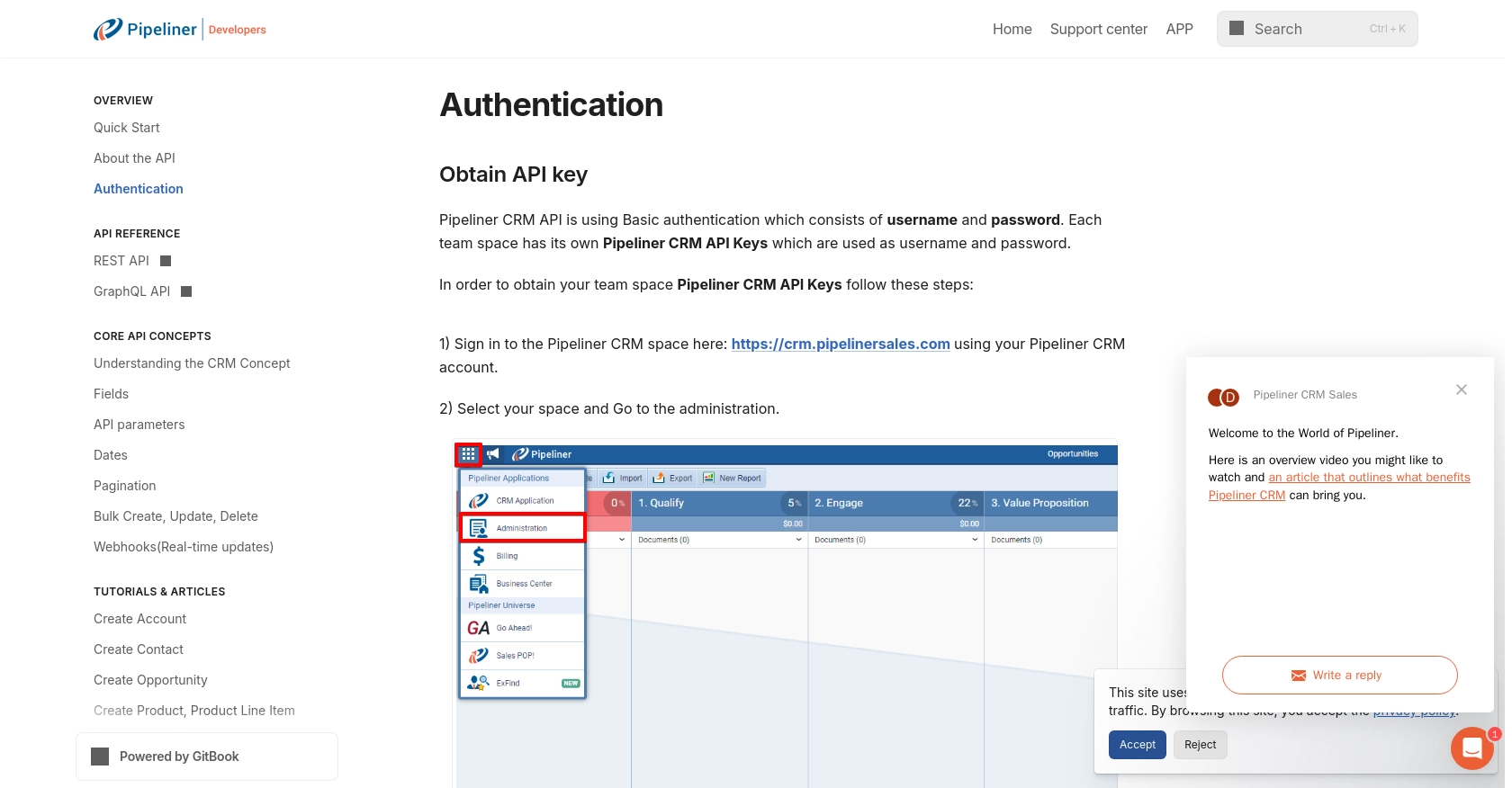
sbb-itb-96038d7
Making API Calls to Retrieve Companies from Pipeliner CRM Using Python
To interact with the Pipeliner CRM API and retrieve company data, you'll need to use Python. This section will guide you through the process of setting up your environment, writing the necessary code, and executing API calls to fetch company information.
Setting Up Your Python Environment for Pipeliner CRM API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Fetch Companies from Pipeliner CRM
Create a new Python file named get_pipeliner_companies.py
and add the following code:
import requests
# Define the API endpoint and authentication credentials
space_id = "your_space_id"
service_url = "your_service_url"
username = "your_api_username"
password = "your_api_password"
# Set the API endpoint URL
endpoint = f"{service_url}/entities/Accounts"
# Set up basic authentication
auth = (username, password)
# Make a GET request to the API
response = requests.get(endpoint, auth=auth)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for company in data['data']:
print(company)
else:
print(f"Failed to retrieve companies: {response.status_code} - {response.text}")
Replace your_space_id
, your_service_url
, your_api_username
, and your_api_password
with the credentials you obtained during the setup process.
Executing the Python Script to Retrieve Company Data
Run the script from your terminal or command prompt using the following command:
python get_pipeliner_companies.py
If successful, the script will output a list of companies retrieved from your Pipeliner CRM test account.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The example code checks the response status code and prints an error message if the request fails. You can further enhance error handling by implementing retries or logging errors for debugging purposes.
To verify the success of your API call, you can cross-check the returned data with the companies listed in your Pipeliner CRM test account.
For more detailed information on error codes and pagination, refer to the Pipeliner CRM API documentation.
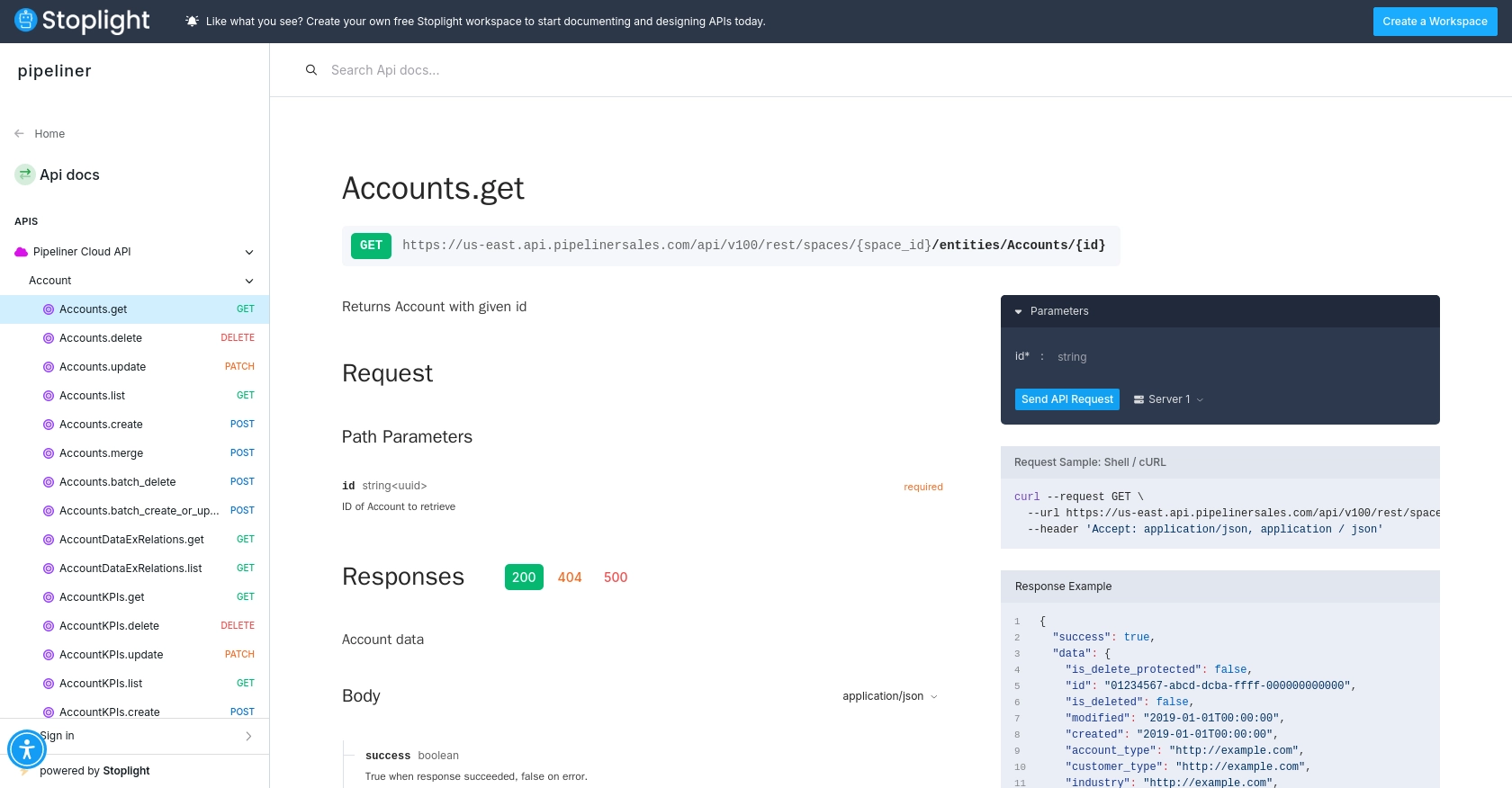
Conclusion and Best Practices for Using Pipeliner CRM API with Python
Integrating with the Pipeliner CRM API using Python provides a powerful way to automate and customize your sales processes. By following the steps outlined in this guide, you can efficiently retrieve company data and enhance your sales team's capabilities.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or a secure vault service to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from Pipeliner CRM is standardized and transformed as needed to fit your application's requirements.
- Pagination Management: Use pagination effectively to handle large datasets. Refer to the Pipeliner CRM API documentation for guidance on implementing pagination with cursors.
Enhance Your Integration Strategy with Endgrate
While building integrations with Pipeliner CRM can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution by providing a unified API endpoint for multiple platforms, including Pipeliner CRM. This allows you to focus on your core product while outsourcing integration complexities.
Explore how Endgrate can simplify your integration process, save time, and enhance the experience for your customers by visiting Endgrate.
Read More
Ready to get started?