How to Create or Update Sales Orders with the Younium API in Python
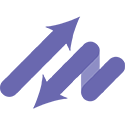
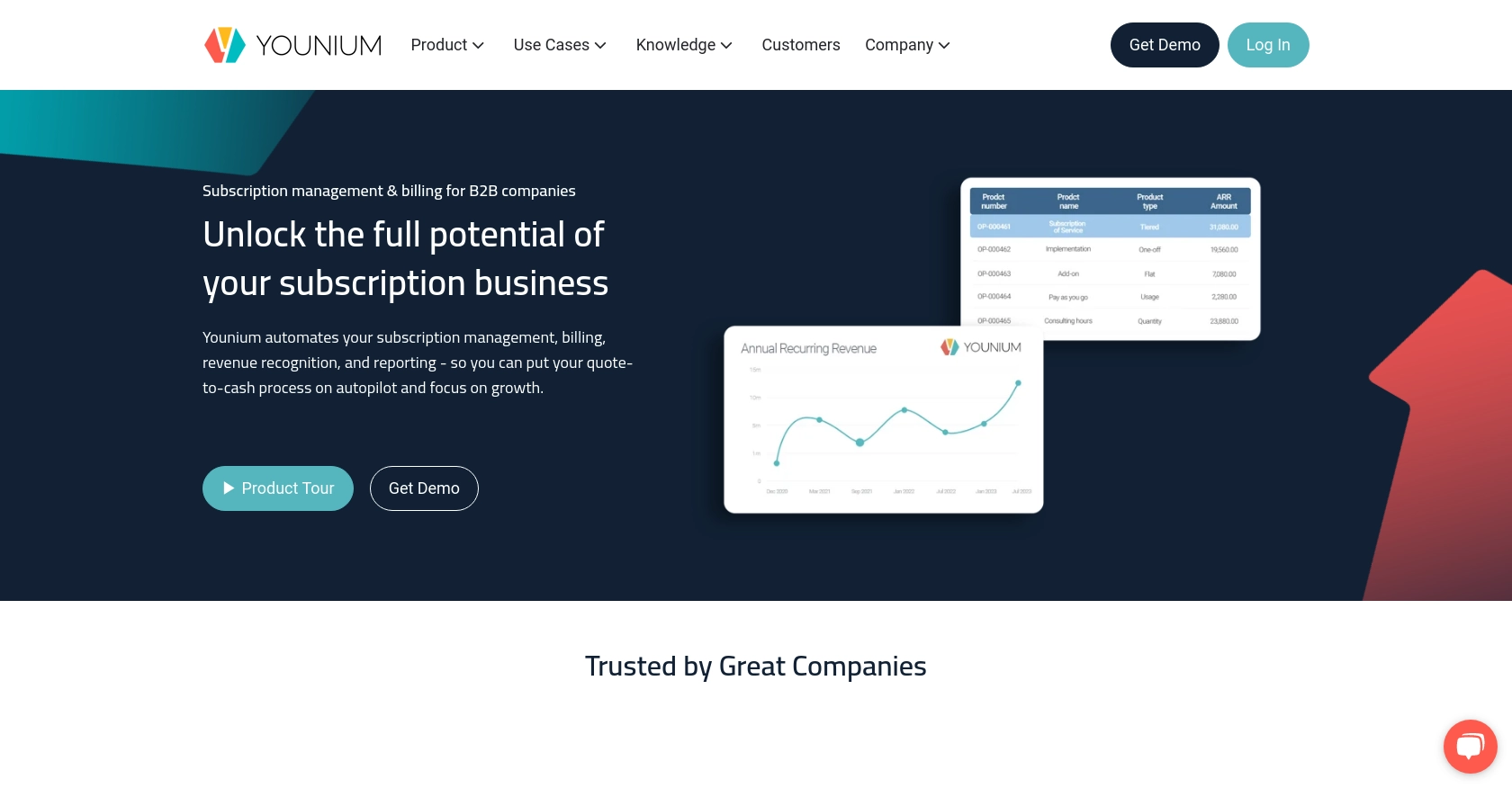
Introduction to Younium API for Sales Order Management
Younium is a comprehensive subscription management platform that empowers B2B SaaS companies to streamline their billing and financial operations. With its robust API, Younium offers developers the ability to automate and enhance various business processes, including sales order management.
Integrating with the Younium API allows developers to efficiently create or update sales orders, facilitating seamless order processing and inventory management. For example, a developer might use the Younium API to automate the creation of sales orders from an external CRM system, ensuring that all sales data is synchronized and up-to-date.
Setting Up Your Younium Test/Sandbox Account
Before you can begin creating or updating sales orders with the Younium API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with the API without affecting your production data.
Creating a Younium Sandbox Account
If you don't already have a Younium account, you can sign up for a sandbox account on the Younium website. This will provide you with a safe environment to test your API integrations.
- Visit the Younium website and navigate to the sandbox account registration page.
- Follow the instructions to create your account, providing the necessary details.
- Once your account is created, log in to access the sandbox environment.
Generating API Tokens and Client Credentials
To interact with the Younium API, you'll need to authenticate your requests using a JWT access token. Follow these steps to generate the necessary credentials:
- Log in to your Younium account and open the user profile menu by clicking your name in the top right corner.
- Select "Privacy & Security" from the dropdown menu.
- In the left panel, click on "Personal Tokens" and then "Generate Token".
- Provide a relevant description for the token and click "Create".
- Copy the Client ID and Secret key that are generated. These will not be visible again, so ensure you store them securely.
Acquiring a JWT Access Token
With your client credentials in hand, you can now generate a JWT access token. This token will be used to authenticate your API requests:
import requests
# Set the endpoint for acquiring the JWT token
url = "https://api.sandbox.younium.com/auth/token"
# Define the request headers and body
headers = {"Content-Type": "application/json"}
body = {
"clientId": "Your_Client_ID",
"secret": "Your_Secret_Key"
}
# Make a POST request to acquire the token
response = requests.post(url, headers=headers, json=body)
# Parse the response to get the access token
token_data = response.json()
access_token = token_data.get("accessToken")
print("Access Token:", access_token)
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. If successful, this code will print your access token, which is valid for 24 hours.
For more details on authentication, refer to the Younium documentation.
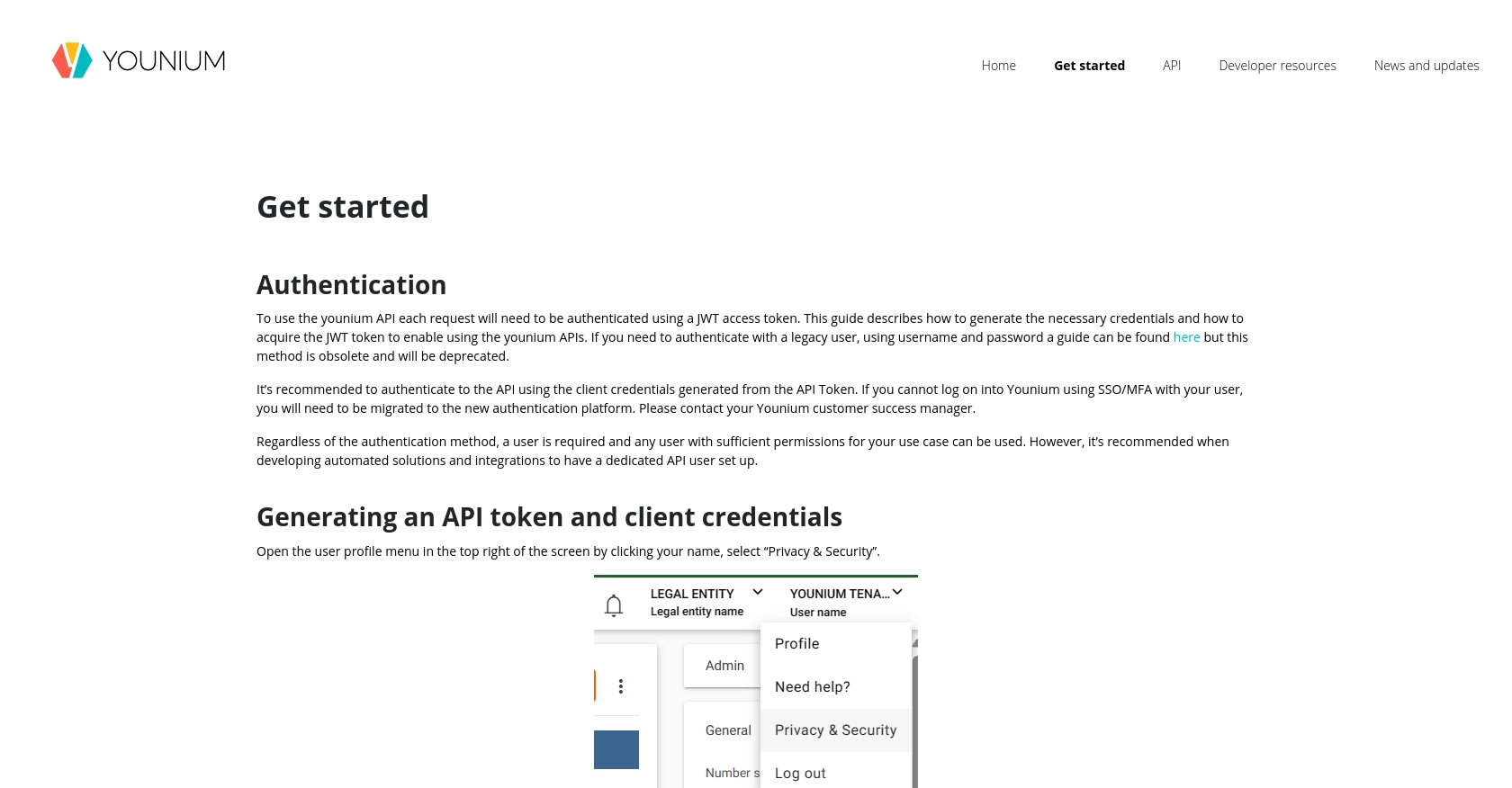
sbb-itb-96038d7
Making API Calls to Create or Update Sales Orders with Younium API in Python
In this section, we'll explore how to create or update sales orders using the Younium API with Python. This involves setting up the necessary environment, writing the code to interact with the API, and handling potential errors.
Setting Up Your Python Environment for Younium API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Creating or Updating Sales Orders with Younium API
Once your environment is ready, you can proceed to create or update sales orders. Below is a sample code snippet to guide you through the process:
import requests
# Define the API endpoint for creating or updating sales orders
url = "https://api.sandbox.younium.com/salesorders"
# Set the request headers
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1"
}
# Define the sales order data
sales_order_data = {
"customerId": "12345",
"orderDate": "2023-10-01",
"orderLines": [
{
"productId": "67890",
"quantity": 10,
"price": 100.0
}
]
}
# Make a POST request to create or update the sales order
response = requests.post(url, headers=headers, json=sales_order_data)
# Check if the request was successful
if response.status_code == 201:
print("Sales Order Created Successfully")
elif response.status_code == 200:
print("Sales Order Updated Successfully")
else:
print("Failed to Create or Update Sales Order:", response.json())
Replace Your_Access_Token
with the JWT access token you obtained earlier. The code above sends a POST request to the Younium API to create or update a sales order. The response status code will indicate whether the operation was successful.
Verifying API Request Success in Younium Sandbox
After executing the code, verify the success of your request by checking the Younium sandbox environment. If a sales order was created or updated, it should appear in the sandbox.
Handling Errors and Common Error Codes
While interacting with the Younium API, you may encounter errors. Here are some common error codes and their meanings:
- 400 Bad Request: Indicates a malformed request. Check the request body and headers.
- 401 Unauthorized: Indicates an expired or invalid access token. Obtain a new token.
- 403 Forbidden: Indicates insufficient permissions or incorrect legal entity.
For more details on error handling, refer to the Younium API documentation.
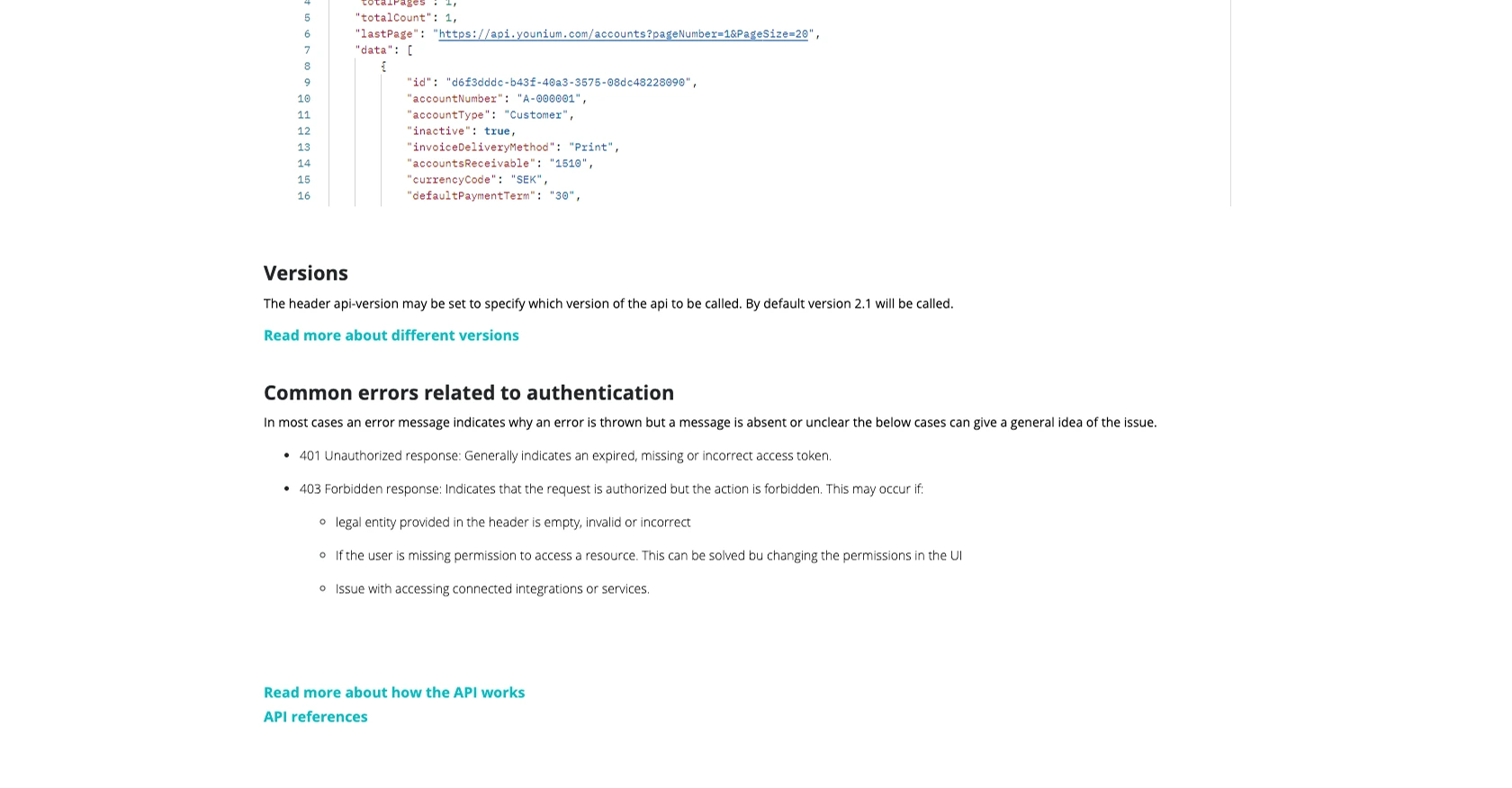
Conclusion and Best Practices for Using Younium API in Python
Integrating with the Younium API to create or update sales orders can significantly enhance your business operations by automating order processing and ensuring data consistency across platforms. By following the steps outlined in this guide, you can efficiently manage sales orders using Python.
Best Practices for Secure and Efficient Younium API Integration
- Secure Storage of Credentials: Always store your Client ID, Secret Key, and JWT access token securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handling Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that data fields are standardized and validated before making API calls to prevent errors and maintain data integrity.
- Regular Token Refresh: Since the JWT access token is valid for 24 hours, implement a mechanism to refresh the token automatically to maintain uninterrupted API access.
Streamlining Integrations with Endgrate
While integrating with the Younium API can be straightforward, managing multiple integrations across different platforms can be complex and time-consuming. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Younium. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?