Using the Younium API to Create or Update Products in Javascript
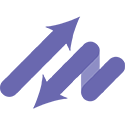
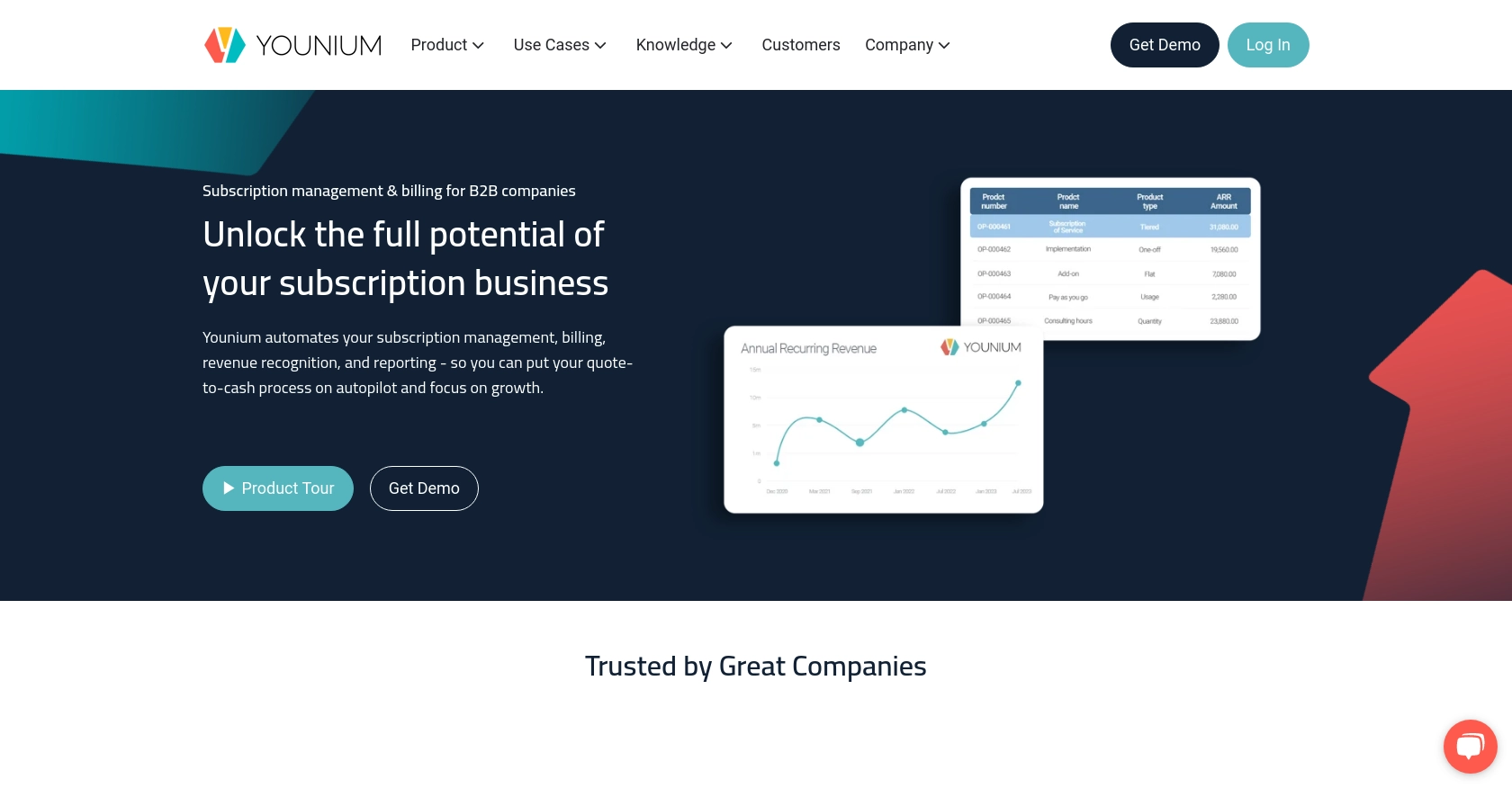
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to help businesses streamline their billing, invoicing, and financial operations. It offers robust tools for managing subscriptions, financial reporting, and customer accounts, making it an essential solution for B2B SaaS companies.
Integrating with the Younium API allows developers to automate and enhance their product management processes. For example, you can create or update product information directly from your application, ensuring that your product catalog remains synchronized with your billing system. This integration can significantly reduce manual data entry and improve operational efficiency.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start integrating with the Younium API, it's crucial to set up a sandbox account. This allows you to test your API interactions without affecting your live data. Younium provides a sandbox environment where developers can experiment and validate their integration processes.
Creating a Younium Sandbox Account
If you don't have a Younium account, you can sign up for a free trial or request access to a sandbox account through the Younium website. Follow these steps to get started:
- Visit the Younium Developer Portal.
- Sign up for a new account or log in if you already have one.
- Request access to the sandbox environment through your account dashboard.
Generating API Credentials for Younium
To authenticate your API requests, you'll need to generate API credentials. Younium uses JWT access tokens for authentication. Follow these steps to create the necessary credentials:
- Navigate to your user profile by clicking your name in the top right corner.
- Select "Privacy & Security" from the dropdown menu.
- Click on "Personal Tokens" in the left panel and then "Generate Token."
- Provide a relevant description and click "Create."
- Copy the Client ID and Secret Key as they will not be visible again.
Acquiring a JWT Access Token
With your client credentials, you can now generate a JWT access token. This token will be used to authenticate your API calls:
// Example POST request to acquire JWT token
const axios = require('axios');
const getToken = async () => {
try {
const response = await axios.post('https://api.sandbox.younium.com/auth/token', {
clientId: 'Your_Client_ID',
secret: 'Your_Secret_Key'
}, {
headers: {
'Content-Type': 'application/json'
}
});
console.log('Access Token:', response.data.accessToken);
} catch (error) {
console.error('Error acquiring token:', error.response.data);
}
};
getToken();
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated. This script will return an access token valid for 24 hours.
For more details on authentication, refer to the Younium Authentication Guide.
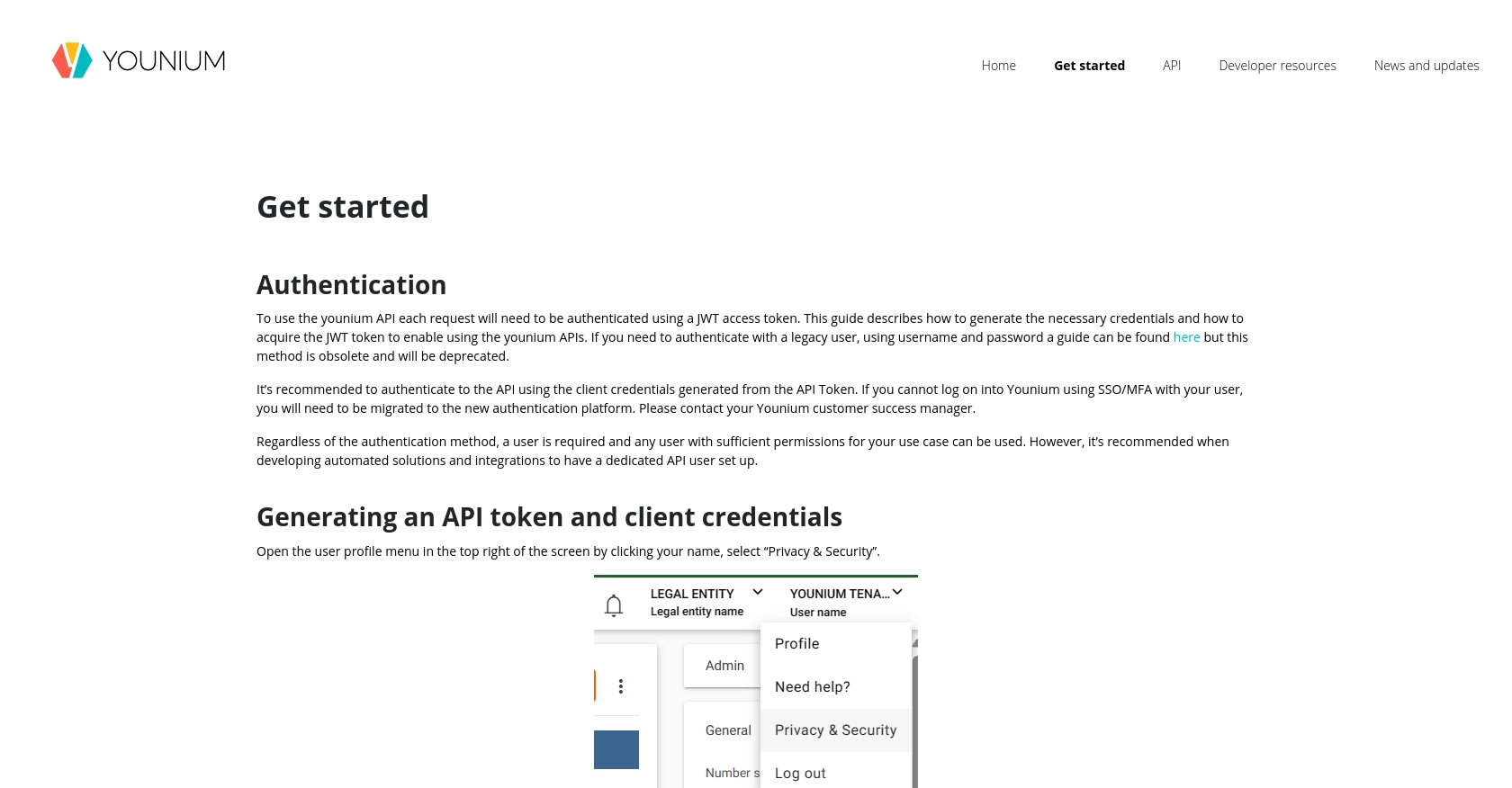
sbb-itb-96038d7
Making API Calls to Younium for Product Management in JavaScript
To interact with the Younium API for creating or updating products, you need to make authenticated API calls using JavaScript. This section will guide you through setting up your environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Younium API Integration
Before making API calls, ensure you have Node.js installed on your machine. You will also need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Creating or Updating Products with Younium API
Once your environment is set up, you can proceed to create or update products using the Younium API. Below is a sample code snippet demonstrating how to perform these operations:
// Import axios for making HTTP requests
const axios = require('axios');
// Function to create or update a product
const manageProduct = async (productData) => {
try {
// Define the API endpoint
const endpoint = 'https://api.sandbox.younium.com/products';
// Set the headers with the JWT token
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
// Make a POST request to create or update the product
const response = await axios.post(endpoint, productData, { headers });
// Log the response data
console.log('Product Response:', response.data);
} catch (error) {
// Handle errors and log the error message
console.error('Error managing product:', error.response.data);
}
};
// Example product data
const productData = {
name: 'New Product',
description: 'A description of the new product',
price: 100.00,
currency: 'USD'
};
// Call the function with the product data
manageProduct(productData);
Replace Your_Access_Token
with the JWT token you obtained earlier. The productData
object should include the necessary fields for your product, such as name
, description
, price
, and currency
.
Verifying API Call Success and Handling Errors
After executing the API call, verify the success of the operation by checking the response data. A successful product creation or update will return relevant product details. If the request fails, the error handling block will log the error message, which can help diagnose issues such as invalid tokens or incorrect data formats.
Common error codes include:
- 400 Bad Request: Indicates malformed request syntax or invalid data.
- 401 Unauthorized: Suggests an expired or incorrect access token.
- 403 Forbidden: Occurs if the user lacks necessary permissions or the legal entity is incorrect.
For more detailed error handling, refer to the Younium API Documentation.
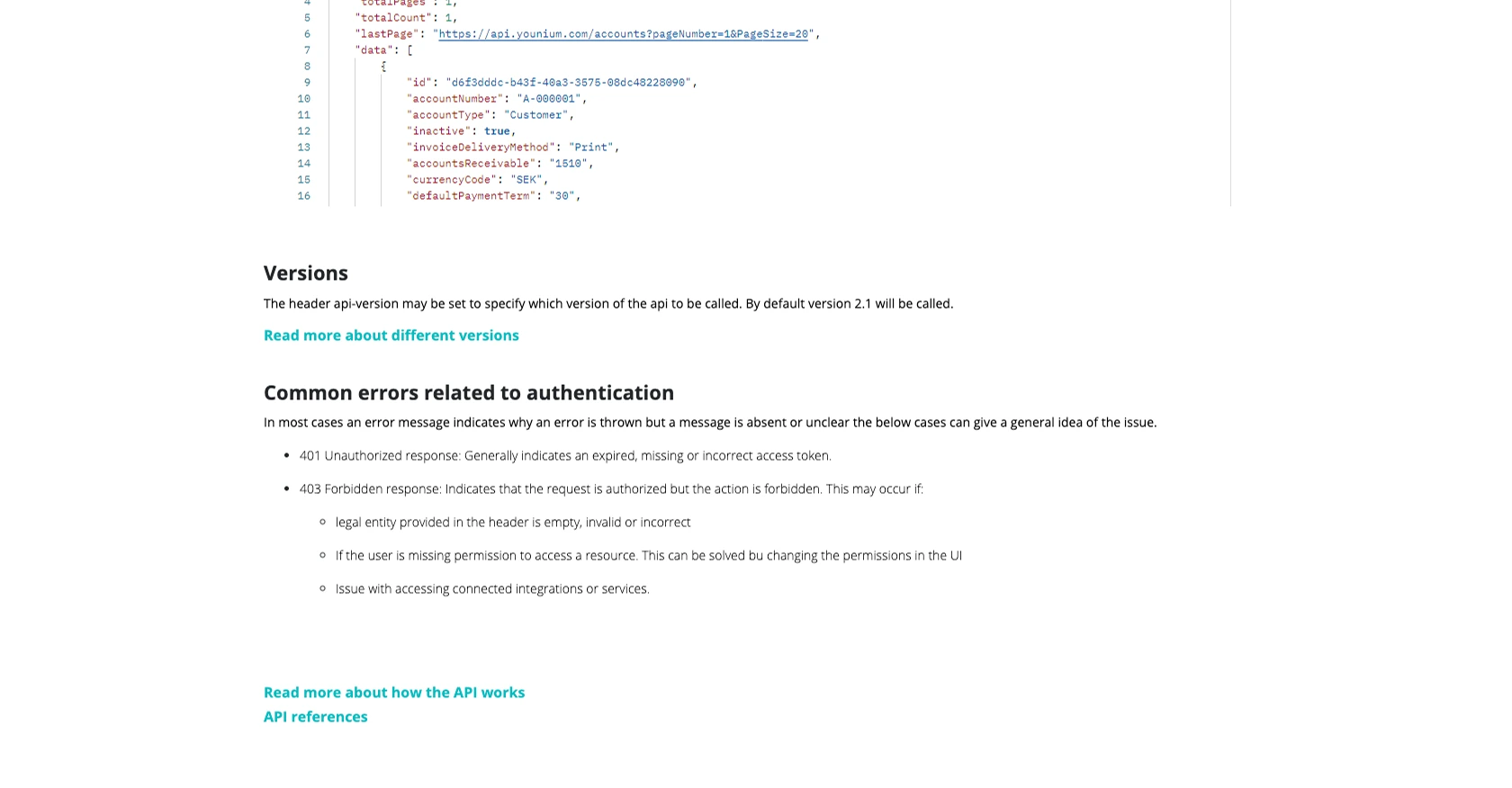
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API using JavaScript can significantly enhance your product management capabilities by automating the creation and updating of product information. This not only streamlines your operations but also ensures data consistency across your systems.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to manage sensitive information like Client IDs and Secret Keys.
- Handling Rate Limits: Be aware of any rate limits imposed by the Younium API to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that your product data is standardized before making API calls. This helps in maintaining consistency and avoiding errors related to data formats.
- Error Handling: Implement robust error handling to manage different HTTP response codes effectively. This will help in diagnosing issues quickly and maintaining a smooth integration process.
Streamlining Integrations with Endgrate
While integrating with Younium directly offers powerful capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Younium.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate, you build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?