Using the Google Calendar API to Get Calendar Events in PHP
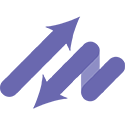
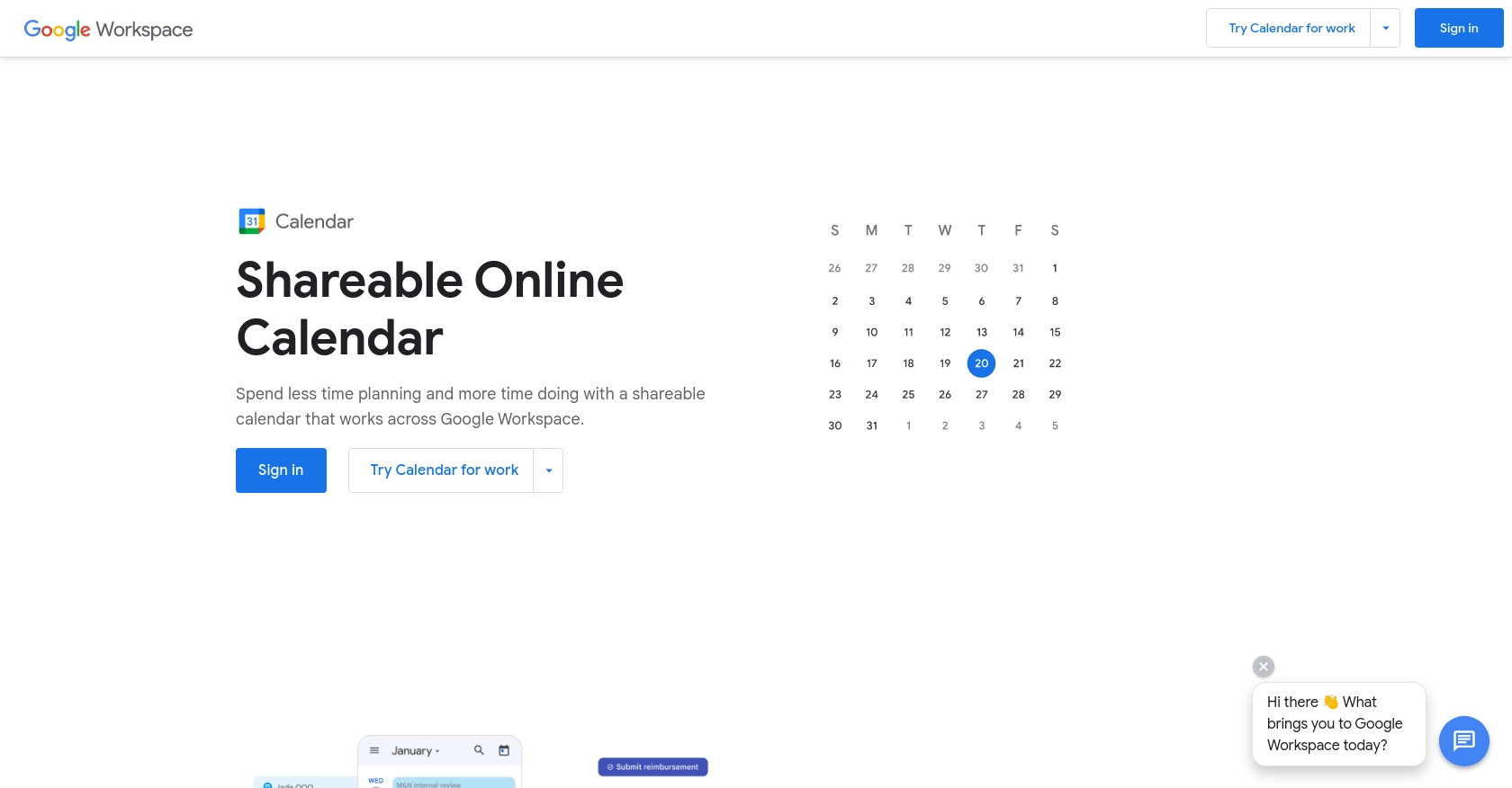
Introduction to Google Calendar API
Google Calendar is a widely used time-management and scheduling service that allows users to create and edit events. It is a part of the Google Workspace suite and is known for its seamless integration with other Google services, making it a popular choice for both personal and professional use.
Integrating with the Google Calendar API enables developers to access and manage calendar events programmatically. This can be particularly useful for applications that need to synchronize events, send reminders, or provide scheduling functionalities. For example, a developer might use the Google Calendar API to fetch upcoming events and display them in a custom dashboard, enhancing user productivity and engagement.
Setting Up a Google Calendar API Test Account
To begin interacting with the Google Calendar API using PHP, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows you to securely access and manage calendar events.
Create a Google Cloud Project
First, you need to create a Google Cloud project. This project will serve as the foundation for enabling APIs and managing credentials.
- Go to the Google Cloud Console.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Calendar API
Once your project is created, you need to enable the Google Calendar API.
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Calendar API" and click on it.
- Click the Enable button.
Configure OAuth Consent Screen
Next, configure the OAuth consent screen to define how your app will request access to user data.
- In the Google Cloud Console, go to Menu > APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the app registration form and click Save and Continue.
Create OAuth 2.0 Credentials
Now, create the OAuth 2.0 credentials needed for authentication.
- In the Google Cloud Console, go to Menu > APIs & Services > Credentials.
- Click Create Credentials > OAuth client ID.
- Select Web application as the application type.
- Enter a name for the credential and add authorized redirect URIs.
- Click Create to generate your Client ID and Client Secret.
Make sure to securely store your Client ID and Client Secret, as they will be used in your PHP application to authenticate API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
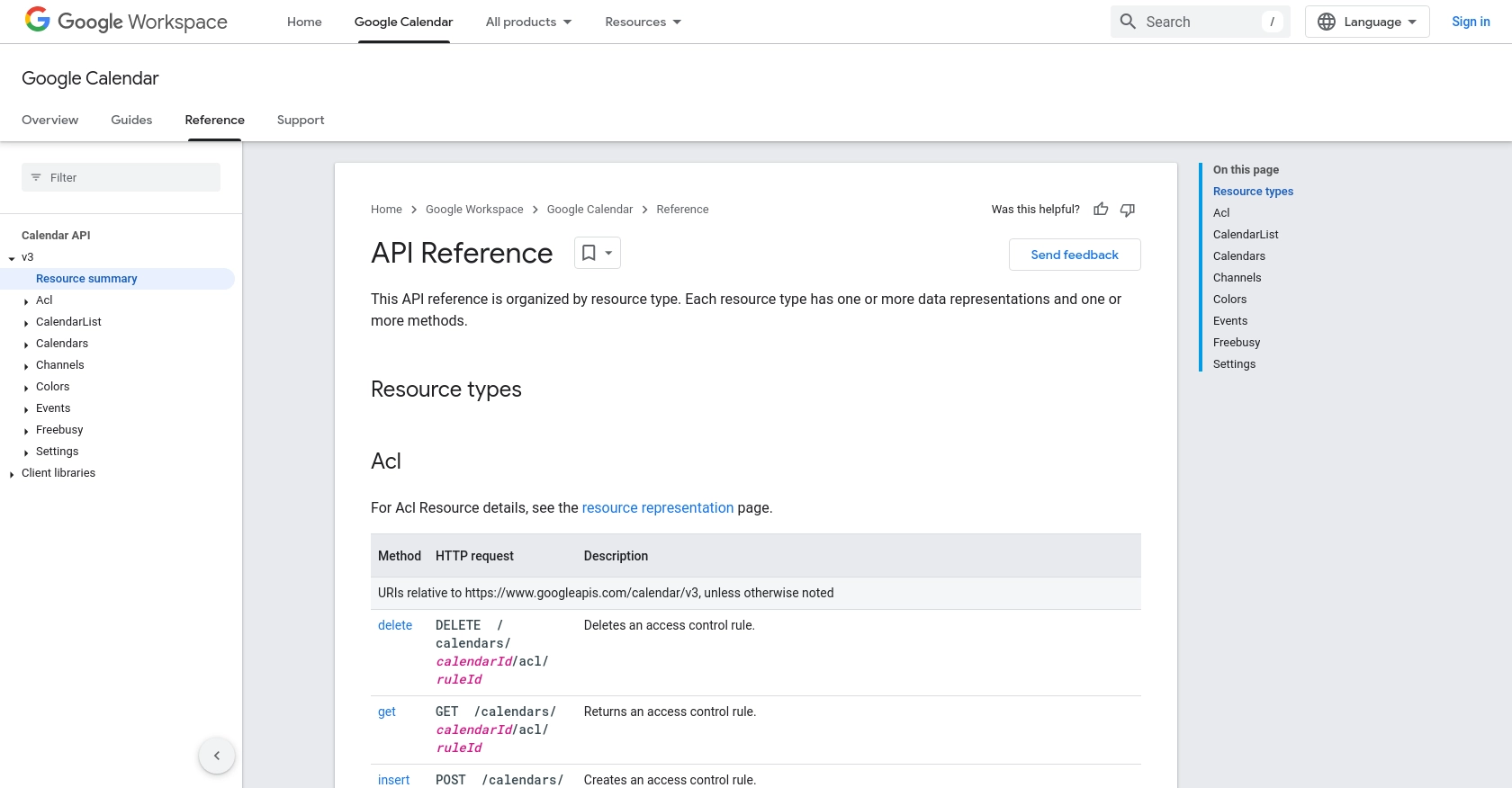
sbb-itb-96038d7
Making API Calls to Retrieve Google Calendar Events Using PHP
To interact with the Google Calendar API and retrieve calendar events using PHP, you need to set up your development environment and write the necessary code to make API requests. This section will guide you through the process, ensuring you can efficiently access calendar data.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, use Composer to install the Google Client Library for PHP by running the following command in your terminal:
composer require google/apiclient:^2.0
Writing PHP Code to Access Google Calendar Events
With the setup complete, you can now write the PHP code to authenticate and fetch events from Google Calendar. Create a file named get_calendar_events.php
and add the following code:
require 'vendor/autoload.php';
session_start();
// Set up the Google Client
$client = new Google_Client();
$client->setAuthConfig('path/to/your/client_credentials.json');
$client->addScope(Google_Service_Calendar::CALENDAR_READONLY);
$client->setRedirectUri('http://localhost/your-redirect-url');
// Handle OAuth 2.0 authorization
if (!isset($_GET['code'])) {
$authUrl = $client->createAuthUrl();
header('Location: ' . filter_var($authUrl, FILTER_SANITIZE_URL));
} else {
$client->authenticate($_GET['code']);
$_SESSION['access_token'] = $client->getAccessToken();
header('Location: ' . filter_var('http://localhost/your-redirect-url', FILTER_SANITIZE_URL));
}
// Set the access token
if (isset($_SESSION['access_token']) && $_SESSION['access_token']) {
$client->setAccessToken($_SESSION['access_token']);
$service = new Google_Service_Calendar($client);
// Fetch events from the primary calendar
$calendarId = 'primary';
$events = $service->events->listEvents($calendarId);
foreach ($events->getItems() as $event) {
echo $event->getSummary() . " (" . $event->getStart()->getDateTime() . ")
";
}
} else {
echo "Authorization required.";
}
Replace path/to/your/client_credentials.json
with the path to your downloaded OAuth 2.0 client credentials JSON file. Also, update http://localhost/your-redirect-url
with your actual redirect URI.
Running the PHP Script and Verifying API Call Success
To execute the script, run the following command in your terminal:
php get_calendar_events.php
Upon successful execution, the script will redirect you to the Google authorization page. After granting access, it will fetch and display the events from your Google Calendar.
Verify the success of the API call by checking the output for your calendar events. If the events are displayed as expected, the integration is successful.
Handling Errors and Debugging
While making API calls, you may encounter errors. Common issues include invalid credentials, incorrect scopes, or network problems. Handle errors by implementing try-catch blocks and logging error messages for debugging. Refer to the Google Calendar API documentation for detailed error codes and troubleshooting tips.
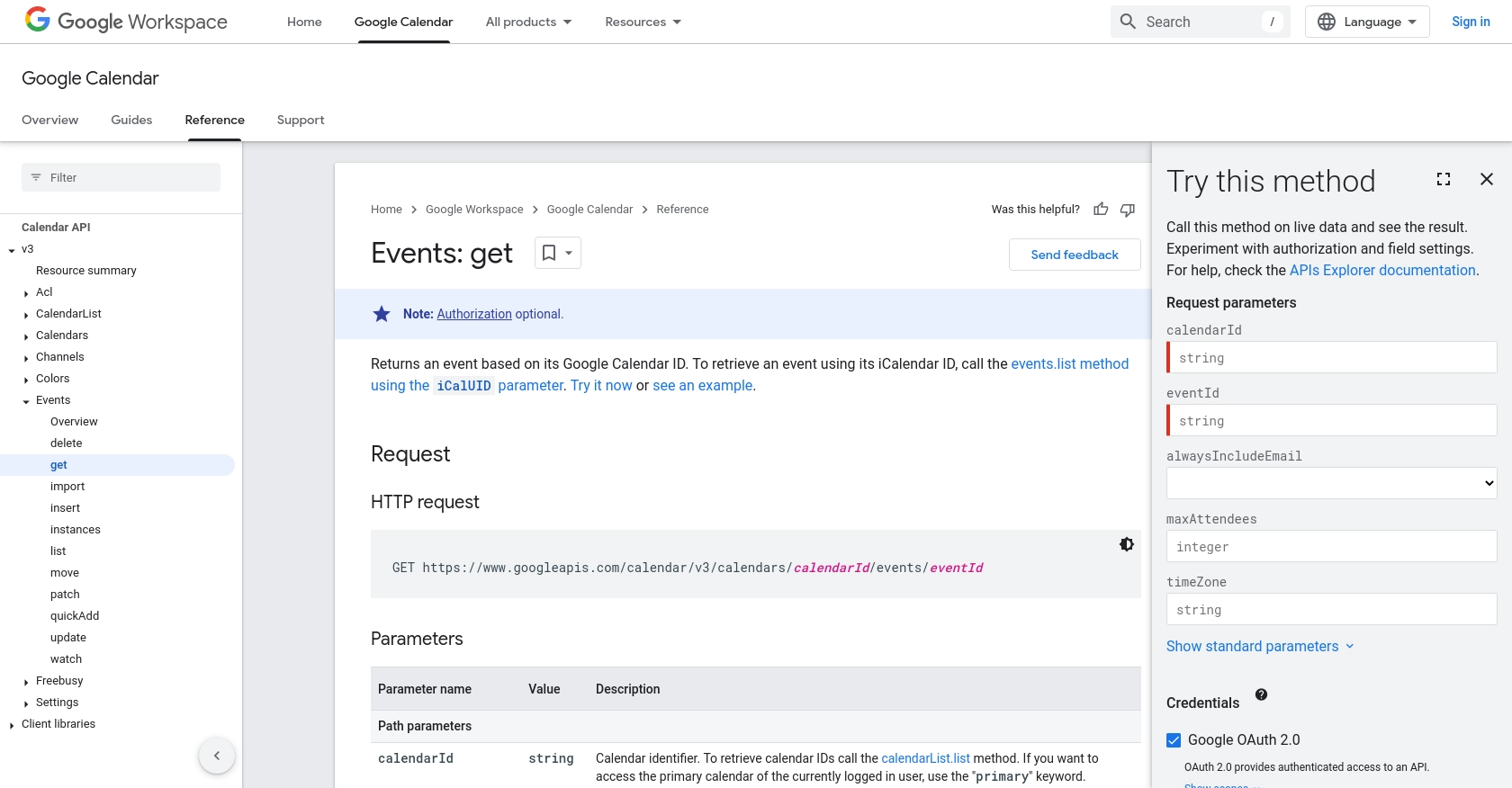
Conclusion and Best Practices for Using Google Calendar API with PHP
Integrating with the Google Calendar API using PHP provides a powerful way to manage and interact with calendar events programmatically. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth 2.0, and retrieve calendar events to enhance your application's functionality.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your OAuth 2.0 Client ID and Client Secret securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of Google's API rate limits to avoid exceeding the allowed number of requests. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Optimize Data Handling: When fetching events, consider filtering and paginating results to minimize data transfer and improve performance.
- Regularly Update Dependencies: Keep your PHP and Google Client Library dependencies up to date to benefit from the latest features and security patches.
Streamlining Integrations with Endgrate
While integrating with Google Calendar API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Google Calendar. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of outsourcing integrations to focus on what truly matters—your core product.
Read More
- https://endgrate.com/provider/googlecalendar
- https://developers.google.com/calendar/api/v3/reference
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/calendar/api/v3/reference/events/get
Ready to get started?