Using the Xero API to Get Accounts in Javascript
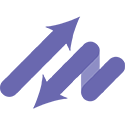
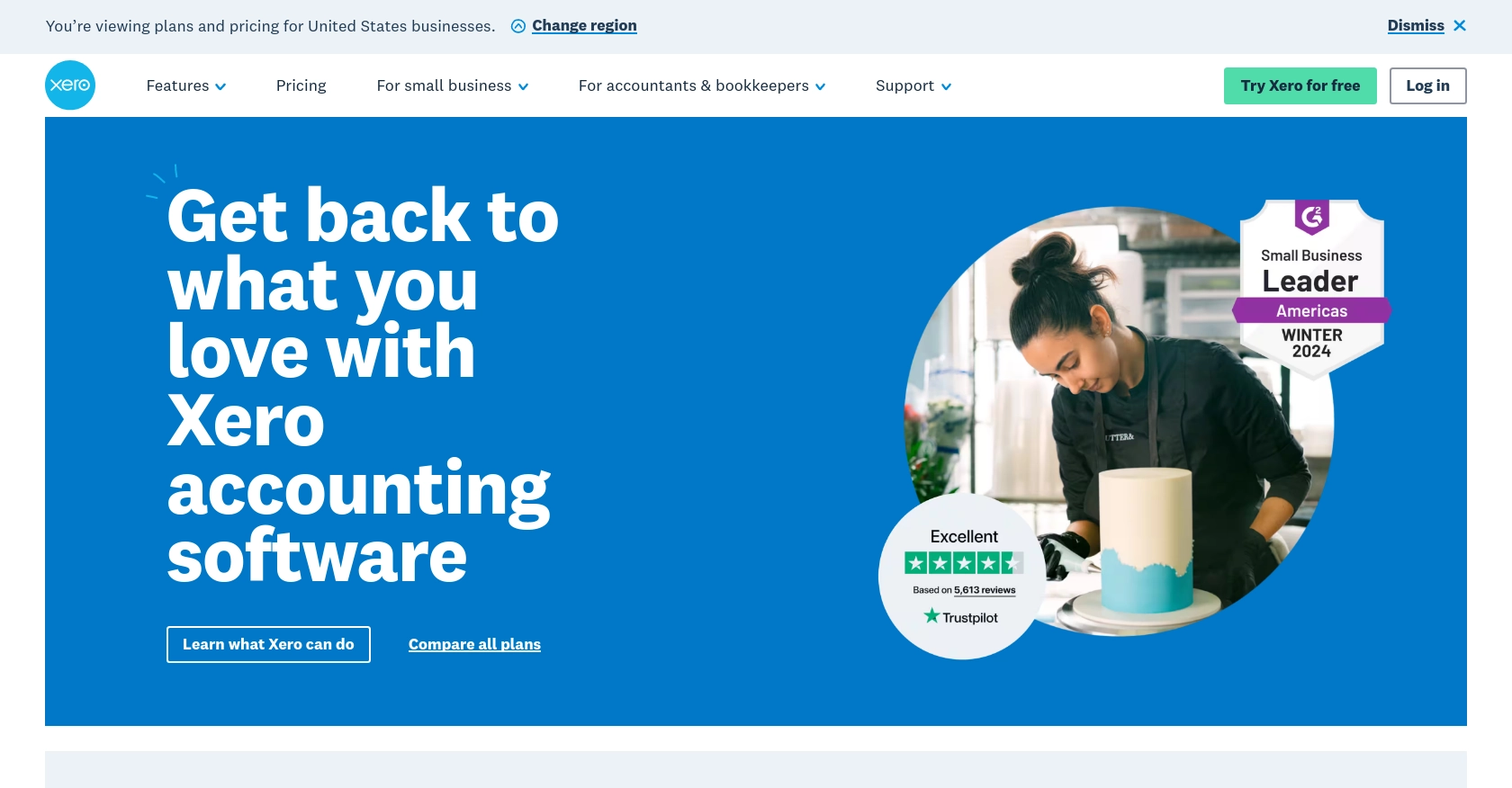
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help businesses manage their finances efficiently. It offers a wide range of features, including invoicing, bank reconciliation, expense tracking, and payroll management, making it a popular choice for small to medium-sized enterprises.
Integrating with Xero's API allows developers to access and manipulate financial data programmatically, enabling seamless automation of accounting tasks. For example, a developer might use the Xero API to retrieve account information, which can be used to generate financial reports or sync with other business applications.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with the Xero API using JavaScript, you'll need to set up a sandbox account. This allows you to test API calls without affecting live data, providing a safe environment for development and experimentation.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal and sign up for a free account.
- Once registered, log in to your developer account.
Setting Up a Xero Sandbox Organization
After creating your developer account, set up a sandbox organization to test your API integrations:
- Navigate to the "My Apps" section in the Xero Developer Portal.
- Create a new organization by selecting "Add a new organization" and choose the demo company option.
- Follow the prompts to complete the setup of your sandbox organization.
Creating a Xero App for OAuth 2.0 Authentication
Xero uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- In the "My Apps" section, click on "New App" to create a new application.
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI to a valid endpoint where you can handle the OAuth callback.
- Save your app to generate the client ID and client secret.
Obtaining OAuth 2.0 Credentials
With your app created, you now have access to the client ID and client secret. These are essential for authenticating API requests:
- Locate your app in the "My Apps" section and click on it to view details.
- Copy the client ID and client secret to a secure location for use in your JavaScript code.
For more detailed information on OAuth 2.0 authentication with Xero, refer to the Xero OAuth 2.0 Overview and Authorization Flow documentation.
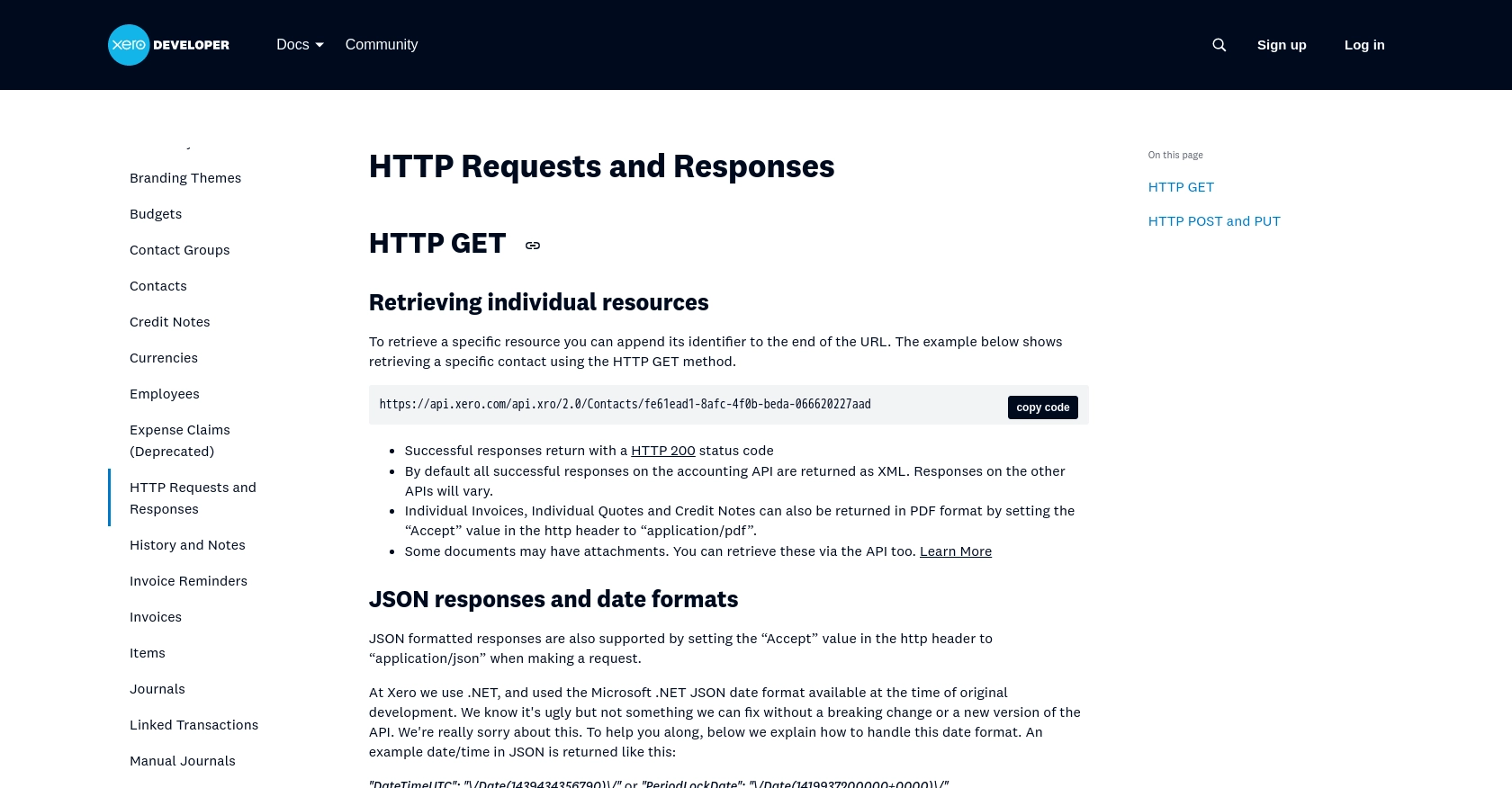
sbb-itb-96038d7
Making API Calls to Xero Using JavaScript
With your Xero sandbox account and OAuth 2.0 credentials ready, you can now proceed to make API calls to retrieve account information using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Xero API Integration
Before making API calls, ensure your development environment is set up correctly:
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Create a new project directory and initialize it using
npm init
. - Install the necessary dependencies by running
npm install axios
to use Axios for making HTTP requests.
Writing JavaScript Code to Retrieve Accounts from Xero
Now, let's write the code to interact with the Xero API and fetch account data:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.xero.com/api.xro/2.0/Accounts';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to get accounts from Xero
async function getXeroAccounts() {
try {
const response = await axios.get(endpoint, { headers });
const accounts = response.data.Accounts;
console.log('Retrieved Accounts:', accounts);
} catch (error) {
console.error('Error fetching accounts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getXeroAccounts();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth 2.0 authentication process. This code uses Axios to send a GET request to the Xero API endpoint for accounts. If successful, it logs the retrieved accounts to the console.
Verifying Successful API Requests in Xero Sandbox
After running the code, you should see the list of accounts printed in your console. To verify the request's success, you can log in to your Xero sandbox organization and check the accounts section to ensure the data matches.
Handling Errors and Xero API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a try-catch
block to catch and log errors. Xero API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. For more details on error codes, refer to the Xero API Requests and Responses documentation.
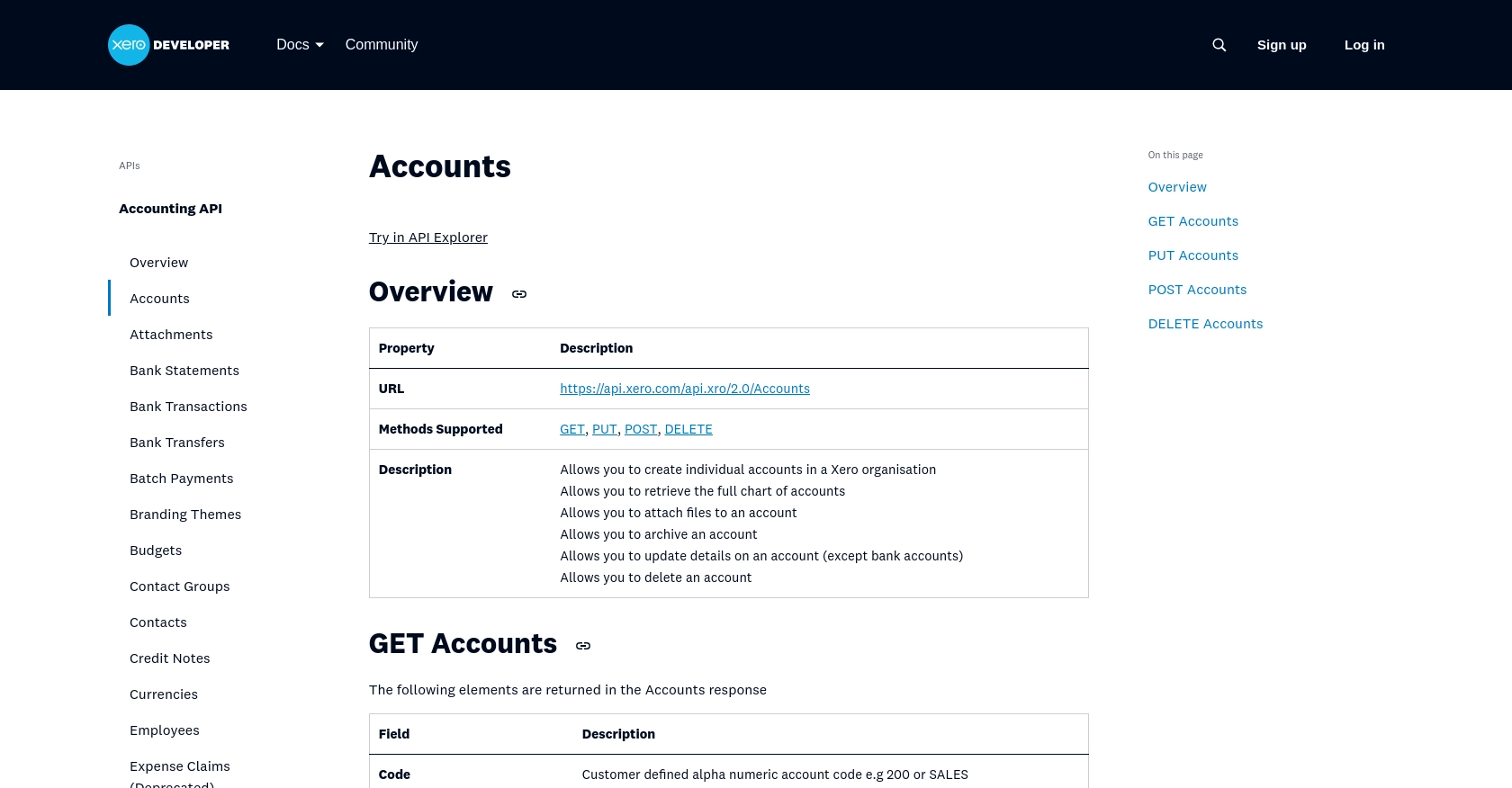
Conclusion and Best Practices for Xero API Integration with JavaScript
Integrating with the Xero API using JavaScript provides a powerful way to automate and streamline accounting processes. By following the steps outlined in this guide, you can efficiently retrieve account information and leverage it for various business applications.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be sure to implement logic to handle rate limiting gracefully. For more details, refer to the Xero API Rate Limits documentation.
- Standardize Data Fields: When integrating with multiple systems, standardize data fields to ensure consistency across platforms.
- Error Handling: Implement robust error handling to manage different error codes returned by the Xero API. This ensures a smooth user experience and helps in debugging issues quickly.
Streamline Your Integrations with Endgrate
While building integrations with Xero and other platforms can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Xero. This allows you to build once for each use case and focus on your core product, saving time and resources.
Explore how Endgrate can simplify your integration process and provide an intuitive experience for your customers. Visit Endgrate to learn more and start optimizing your integration strategy today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/accounts
Ready to get started?