Using the Sap Business One API to Create or Update Customers in Python
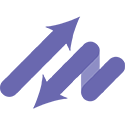
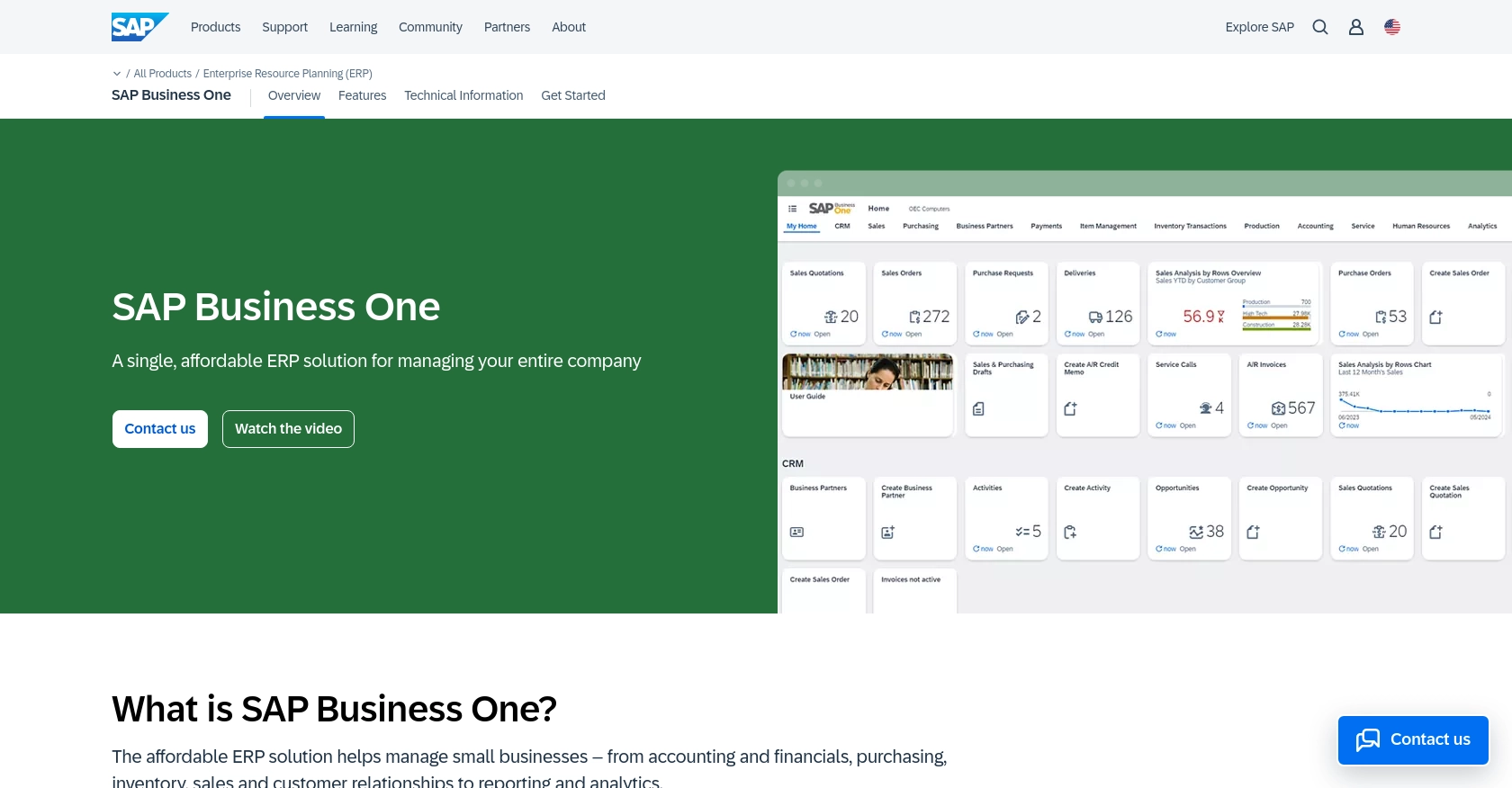
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single platform.
Integrating with the SAP Business One API allows developers to automate and streamline business processes by interacting directly with the system's data. For example, a developer might use the API to create or update customer records, ensuring that customer information is always up-to-date and accessible across various business functions.
This article will guide you through using Python to interact with the SAP Business One API, specifically focusing on creating or updating customer records. By following this tutorial, you'll learn how to efficiently manage customer data within the SAP Business One environment, enhancing your business operations.
Setting Up a Test or Sandbox Account for SAP Business One API
Before you can start integrating with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with the API without affecting your live data. Here's how you can get started:
Creating a SAP Business One Sandbox Account
To begin, you'll need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a sandbox instance from your system administrator. If not, you may need to contact SAP directly to inquire about trial or demo environments.
Generating API Credentials for SAP Business One
Once you have access to the sandbox environment, the next step is to generate the necessary API credentials. SAP Business One uses a custom authentication method, so you'll need to follow these steps:
- Log in to the SAP Business One Service Layer using your sandbox account credentials.
- Navigate to the API Management section within the SAP Business One interface.
- Create a new API application by providing the required details such as application name and description.
- Once the application is created, you will receive a client ID and client secret. Make sure to store these securely, as they will be used to authenticate your API requests.
Configuring OAuth for SAP Business One API
Although SAP Business One uses a custom authentication method, it often involves OAuth-like steps for token generation:
- Use the client ID and client secret to request an access token from the SAP Business One authentication server.
- Include this access token in the header of your API requests to authenticate them.
For detailed instructions, refer to the official SAP Business One documentation: SAP Business One Service Layer Documentation.
Testing Your Setup
After setting up your sandbox account and generating the necessary credentials, it's important to test your configuration:
- Make a simple API call to verify that your credentials are working correctly.
- Check the response to ensure that you have the necessary permissions to create or update customer records.
By following these steps, you'll be ready to start integrating with the SAP Business One API using Python, allowing you to create or update customer records efficiently.
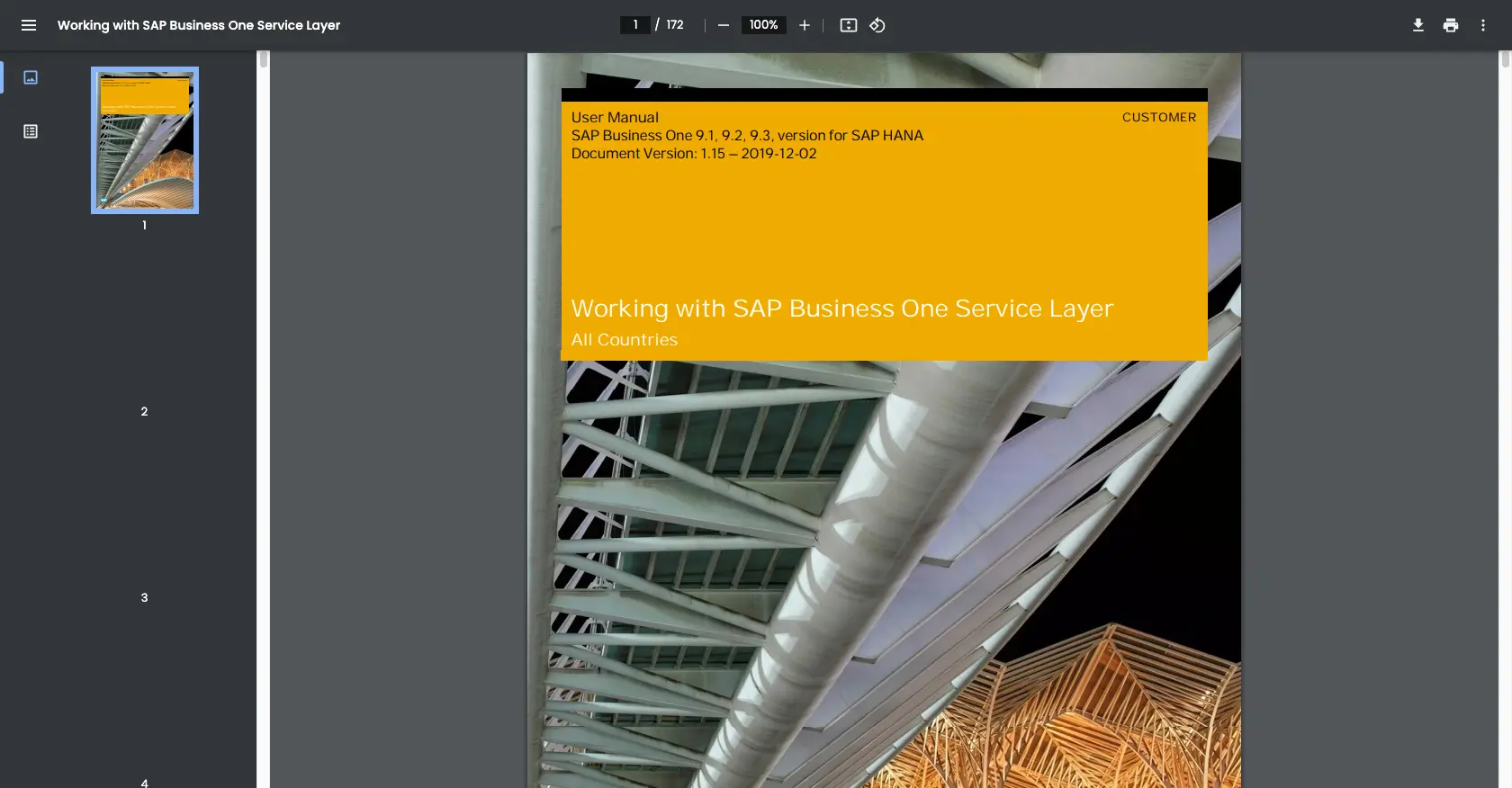
sbb-itb-96038d7
Making API Calls to SAP Business One for Customer Management in Python
To interact with the SAP Business One API for creating or updating customer records, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for SAP Business One API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Install the required dependencies by running the following command in your terminal or command prompt:
pip install requests
The requests
library will help you make HTTP requests to the SAP Business One API.
Writing Python Code to Create or Update Customers in SAP Business One
Create a new Python file named sap_business_one_customer.py
and add the following code:
import requests
# Set the API endpoint for creating or updating customers
url = "https://your-sap-business-one-instance.com/b1s/v1/BusinessPartners"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the customer data
customer_data = {
"CardCode": "C12345",
"CardName": "New Customer",
"CardType": "C",
"EmailAddress": "customer@example.com"
}
# Send the request to create or update the customer
response = requests.post(url, json=customer_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Customer created or updated successfully.")
else:
print(f"Failed to create or update customer: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the token obtained during the authentication setup. The customer_data
dictionary contains the customer details you wish to create or update.
Verifying API Call Success in SAP Business One
After running the script, verify the success of your API call by checking the response status code. A status code of 201
indicates success. You can also log into your SAP Business One sandbox account to confirm that the customer record has been created or updated.
Handling Errors and Troubleshooting SAP Business One API Calls
It's crucial to handle potential errors when making API calls. Common error codes include:
400
- Bad Request: Check your request syntax and data.401
- Unauthorized: Verify your access token.404
- Not Found: Ensure the endpoint URL is correct.500
- Internal Server Error: Contact SAP support if this persists.
For more detailed error handling, refer to the official SAP Business One documentation: SAP Business One Service Layer Documentation.
Conclusion and Best Practices for Integrating with SAP Business One API
Integrating with the SAP Business One API to create or update customer records using Python can significantly enhance your business operations by ensuring that customer data is consistently accurate and accessible. By following the steps outlined in this article, you can efficiently manage customer information within the SAP Business One environment.
Best Practices for Secure and Efficient SAP Business One API Integration
- Securely Store Credentials: Always store your API credentials, such as client ID and client secret, securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement logic to handle rate limit responses and retry requests as needed.
- Data Standardization: Ensure that customer data is standardized before sending it to the API. This includes validating email formats and ensuring consistent data types.
- Error Handling: Implement robust error handling to manage potential issues with API calls. Log errors for further analysis and troubleshooting.
Streamlining Integrations with Endgrate
While integrating with SAP Business One API can be a powerful way to enhance your business processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including SAP Business One.
By using Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. With Endgrate, you build once for each use case, rather than multiple times for different integrations, providing an easy and intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover a more efficient way to manage your business integrations.
Read More
Ready to get started?