Using the Microsoft Dynamics 365 API to Create or Update Account (with Javascript examples)
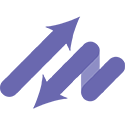
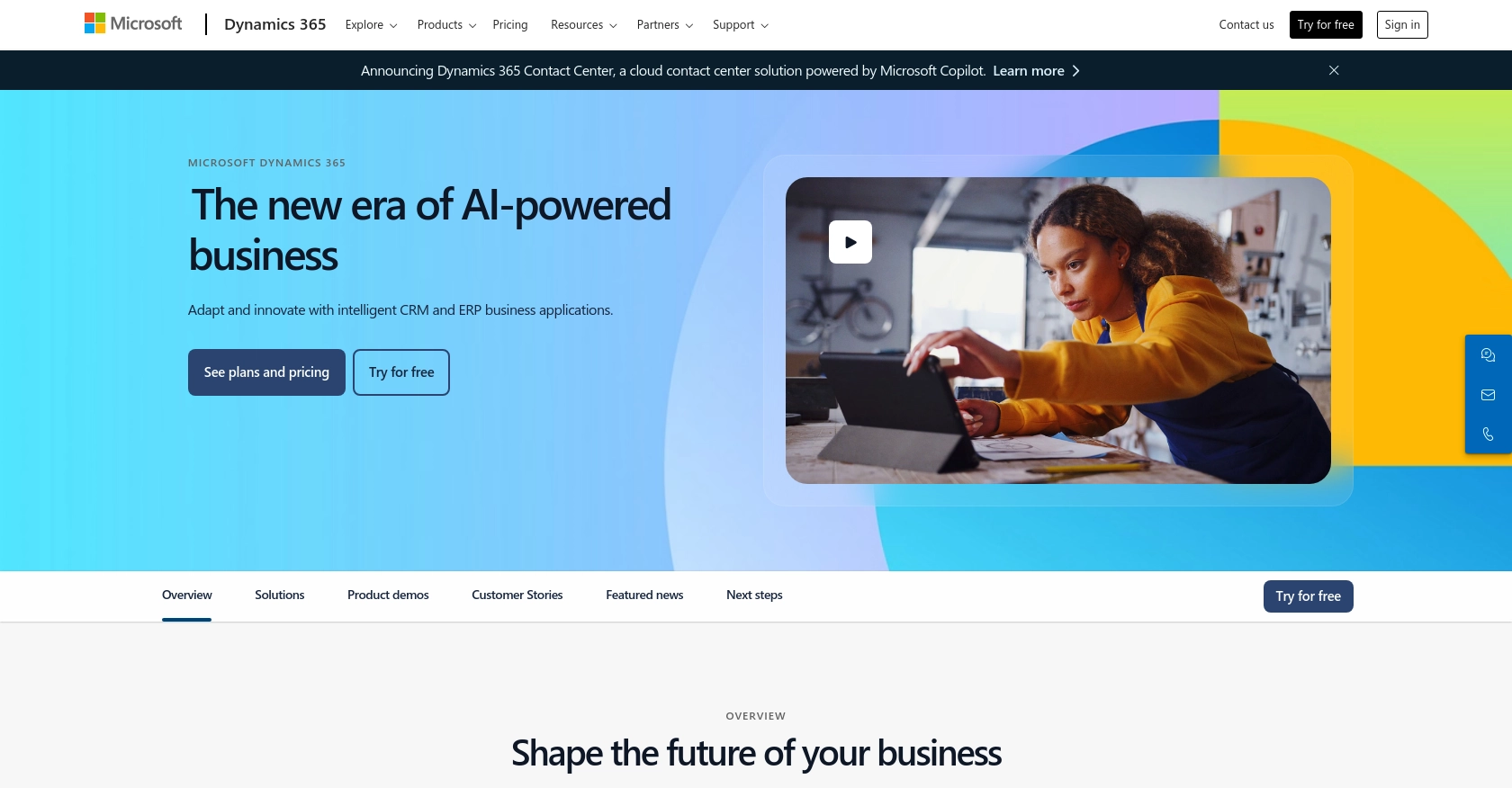
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications that combines CRM and ERP capabilities to streamline business processes and enhance customer engagement. It offers a robust platform for managing sales, customer service, finance, and operations, making it a popular choice for businesses looking to integrate their various functions.
Developers may want to connect with Microsoft Dynamics 365 to automate and enhance their business processes. For example, using the Microsoft Dynamics 365 API, a developer can create or update account information directly from a web application, ensuring that customer data is always up-to-date and accessible across the organization.
This article will guide you through using JavaScript to interact with the Microsoft Dynamics 365 API, specifically focusing on creating or updating account records. By following this tutorial, you will learn how to efficiently manage account data within the Dynamics 365 platform using JavaScript.
Setting Up a Microsoft Dynamics 365 Test/Sandbox Account
Before you begin integrating with the Microsoft Dynamics 365 API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Microsoft Dynamics 365 Free Trial Account
- Visit the Microsoft Dynamics 365 website and click on "Try for free."
- Fill out the registration form with your details and submit it to create your account.
- Once registered, you will receive an email with instructions to access your Dynamics 365 environment.
Register an Application in Microsoft Entra ID
To use OAuth authentication with Microsoft Dynamics 365, you need to register your application in Microsoft Entra ID. This process will provide you with the necessary credentials to authenticate API requests.
- Navigate to the Azure Portal and sign in with your Microsoft account.
- Go to "Azure Active Directory" and select "App registrations."
- Click on "New registration" and fill in the required details, such as the application name and redirect URI.
- After registration, note down the Application (client) ID and Directory (tenant) ID.
Configure API Permissions
To interact with the Microsoft Dynamics 365 API, you need to set the appropriate permissions for your registered application.
- In the Azure Portal, navigate to your registered application and select "API permissions."
- Click on "Add a permission" and choose "Dynamics CRM."
- Select the permissions required for your application, such as "user_impersonation" for accessing data on behalf of a user.
- Click "Add permissions" to apply the changes.
Generate Client Secret for Authentication
To authenticate your application, you need a client secret. Follow these steps to generate one:
- In your registered application, go to "Certificates & secrets."
- Under "Client secrets," click on "New client secret."
- Provide a description and select an expiration period for the secret.
- Click "Add" and copy the generated secret value. Store it securely as it will not be shown again.
Set Up OAuth Authentication in Your Application
With your application registered and permissions set, you can now configure OAuth authentication in your JavaScript application. Use the Microsoft Authentication Library (MSAL) to acquire access tokens for API requests.
// Example using MSAL.js
const msalConfig = {
auth: {
clientId: 'Your_Client_ID',
authority: 'https://login.microsoftonline.com/Your_Tenant_ID',
redirectUri: 'http://localhost'
}
};
const msalInstance = new msal.PublicClientApplication(msalConfig);
async function getAccessToken() {
const loginRequest = {
scopes: ['https://Your_Org.crm.dynamics.com/.default']
};
try {
const loginResponse = await msalInstance.loginPopup(loginRequest);
return loginResponse.accessToken;
} catch (error) {
console.error('Error acquiring access token:', error);
}
}
Replace Your_Client_ID
and Your_Tenant_ID
with your application's client ID and tenant ID. Use the acquired access token to authenticate your API requests.
With your test account and application set up, you're ready to start making API calls to Microsoft Dynamics 365.
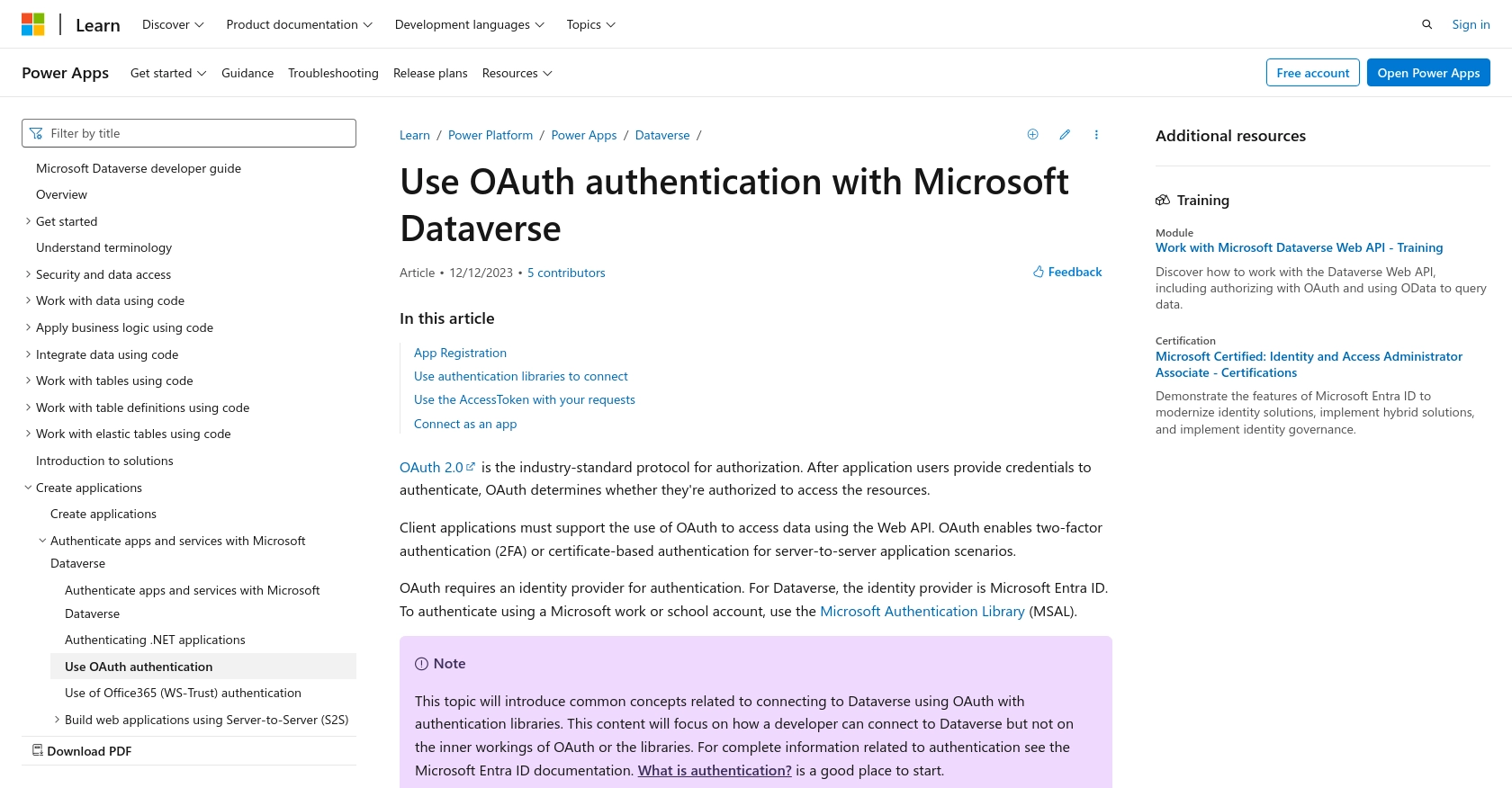
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 for Account Management Using JavaScript
Now that you have set up your Microsoft Dynamics 365 environment and configured OAuth authentication, it's time to make API calls to create or update account records. This section will guide you through the process using JavaScript, ensuring you can efficiently manage account data within Dynamics 365.
Prerequisites for JavaScript Integration with Microsoft Dynamics 365 API
Before proceeding, ensure you have the following prerequisites installed and configured:
- Node.js and npm for managing JavaScript dependencies.
- MSAL.js library for handling OAuth authentication.
- An active Microsoft Dynamics 365 test or sandbox account.
Installing Required JavaScript Libraries
To interact with the Microsoft Dynamics 365 API, you need to install the necessary libraries. Use the following command to install MSAL.js:
npm install @azure/msal-browser
Creating or Updating an Account in Microsoft Dynamics 365
With the setup complete, you can now create or update an account using the Microsoft Dynamics 365 API. Below is an example of how to perform these operations using JavaScript:
// Import the required libraries
import { PublicClientApplication } from '@azure/msal-browser';
// Configure MSAL
const msalConfig = {
auth: {
clientId: 'Your_Client_ID',
authority: 'https://login.microsoftonline.com/Your_Tenant_ID',
redirectUri: 'http://localhost'
}
};
const msalInstance = new PublicClientApplication(msalConfig);
// Function to acquire an access token
async function getAccessToken() {
const loginRequest = {
scopes: ['https://Your_Org.crm.dynamics.com/.default']
};
try {
const loginResponse = await msalInstance.loginPopup(loginRequest);
return loginResponse.accessToken;
} catch (error) {
console.error('Error acquiring access token:', error);
}
}
// Function to create or update an account
async function createOrUpdateAccount(accountData) {
const token = await getAccessToken();
const url = 'https://Your_Org.api.crm.dynamics.com/api/data/v9.2/accounts';
const headers = {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json',
'OData-MaxVersion': '4.0',
'OData-Version': '4.0'
};
try {
const response = await fetch(url, {
method: 'POST', // Use 'PATCH' for updating an existing account
headers: headers,
body: JSON.stringify(accountData)
});
if (response.ok) {
const result = await response.json();
console.log('Account operation successful:', result);
} else {
console.error('Failed to create or update account:', response.statusText);
}
} catch (error) {
console.error('Error during API call:', error);
}
}
// Example account data
const accountData = {
name: 'New Account Name',
accountnumber: '12345',
telephone1: '123-456-7890',
emailaddress1: 'contact@example.com'
};
// Call the function to create or update the account
createOrUpdateAccount(accountData);
Replace Your_Client_ID
, Your_Tenant_ID
, and Your_Org
with your specific application and organization details. The accountData
object should contain the account properties you wish to create or update, as outlined in the Microsoft Dynamics 365 API documentation.
Verifying API Call Success in Microsoft Dynamics 365
After executing the API call, verify the success of the operation by checking the response status and reviewing the account records in your Microsoft Dynamics 365 sandbox environment. Successful creation or update will reflect in the account list.
Handling Errors and Common Issues
When making API calls, you may encounter errors. Common HTTP status codes include:
- 400 Bad Request: Check the request syntax and data format.
- 401 Unauthorized: Ensure the access token is valid and has the required permissions.
- 403 Forbidden: Verify that your application has the necessary API permissions.
For more detailed error handling, refer to the Microsoft Graph API documentation.
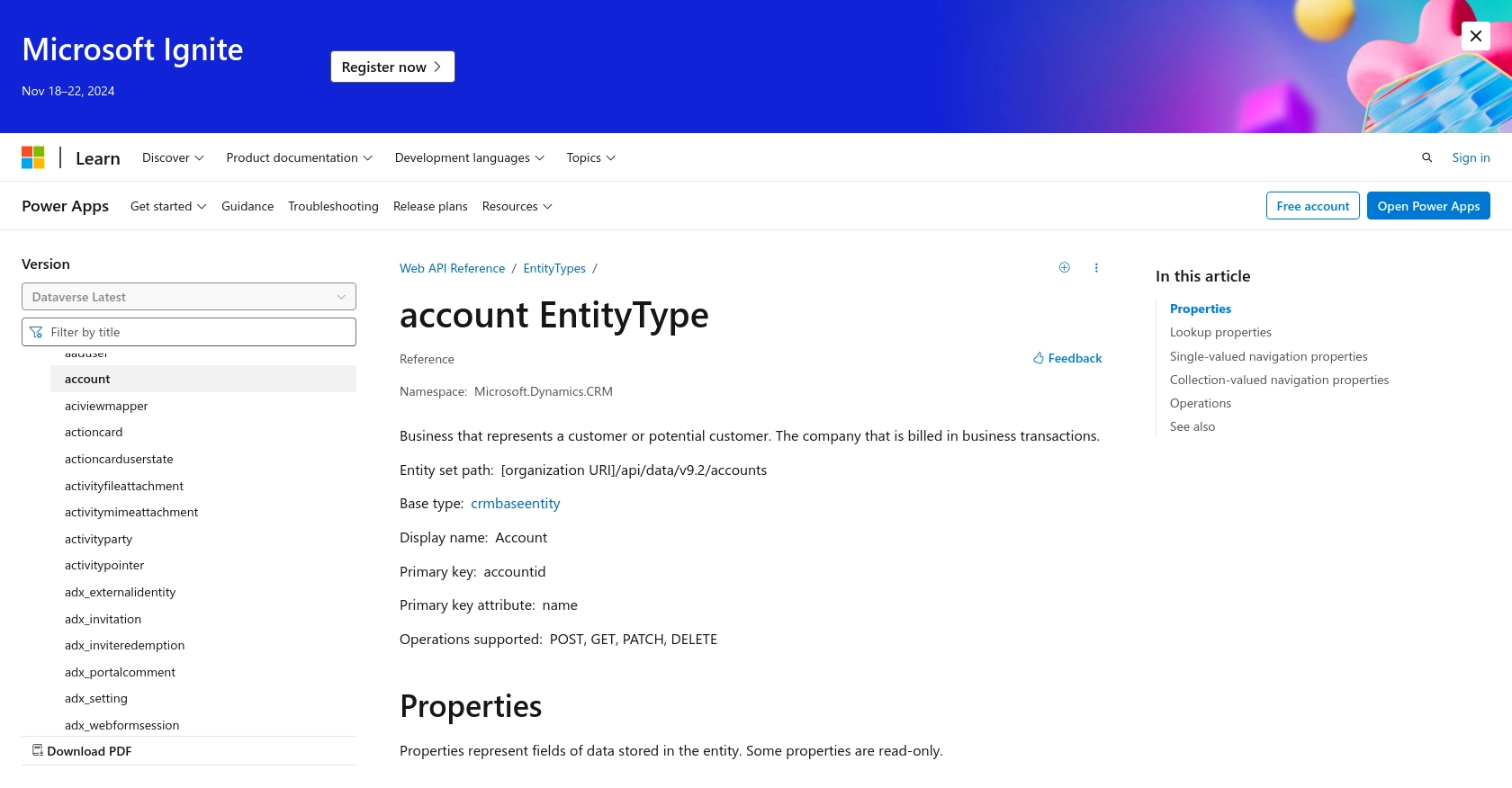
Conclusion and Best Practices for Integrating with Microsoft Dynamics 365 API
Integrating with the Microsoft Dynamics 365 API using JavaScript offers a powerful way to manage account data efficiently. By following the steps outlined in this guide, you can create or update account records seamlessly, ensuring your business processes remain streamlined and effective.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store client secrets and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure data consistency by standardizing fields before sending them to the API. This practice helps maintain data integrity across your systems.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any bottlenecks or issues that may arise.
Streamline Your Integration Process with Endgrate
While integrating with Microsoft Dynamics 365 API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Microsoft Dynamics 365.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/account?view=dataverse-latest
Ready to get started?