Using the Zoho Recruit API to Get Candidates in Javascript
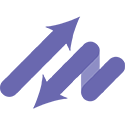
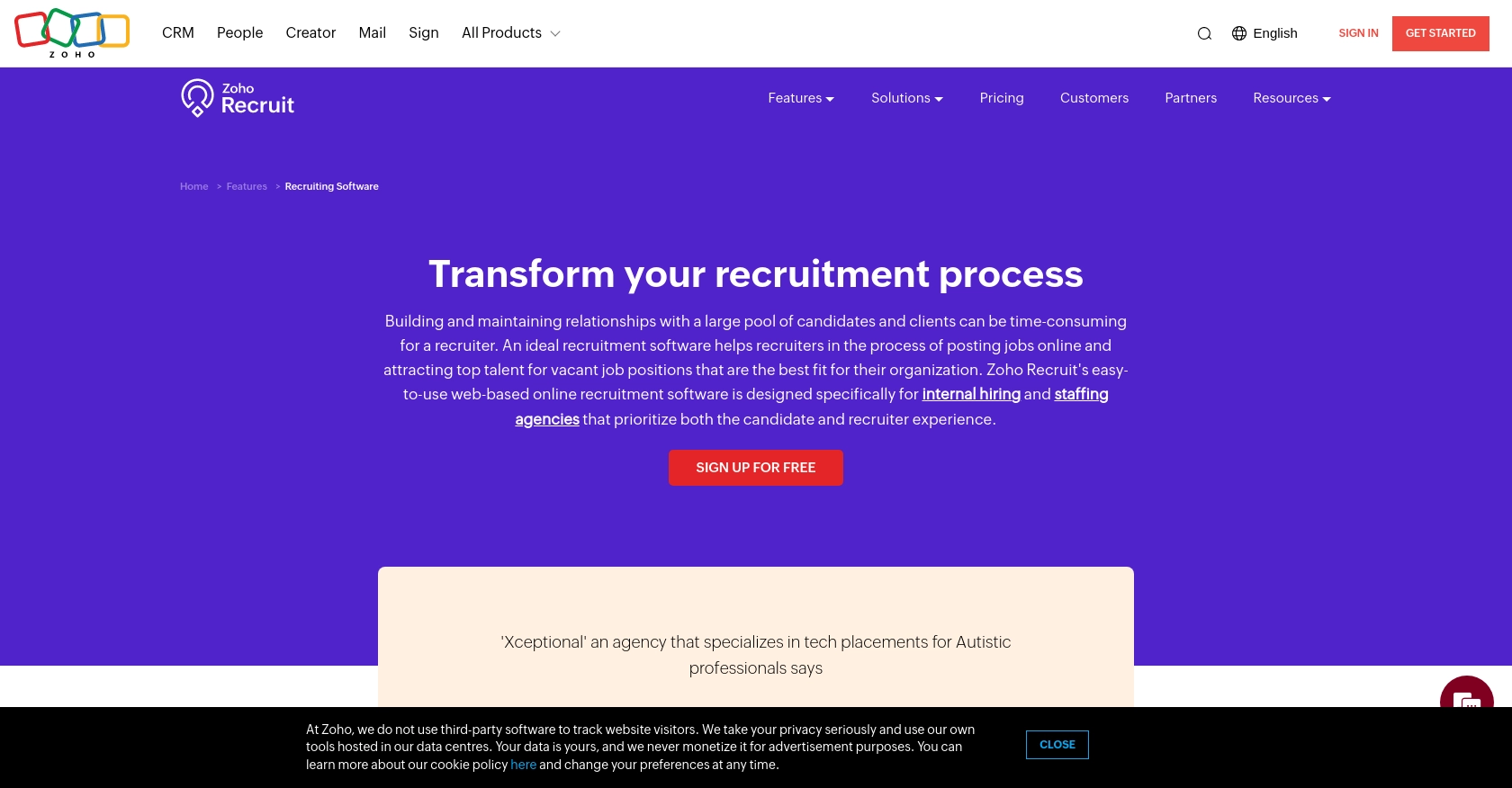
Introduction to Zoho Recruit API
Zoho Recruit is a comprehensive recruitment software solution designed to streamline the hiring process for businesses of all sizes. It offers a robust platform with features for candidate sourcing, resume management, and interview scheduling, making it an ideal choice for staffing agencies and corporate HR teams.
Integrating with the Zoho Recruit API allows developers to automate and enhance recruitment workflows. For example, you can use the API to retrieve candidate information, enabling seamless integration with other HR systems or custom applications. This can help in creating a unified recruitment experience, reducing manual data entry, and improving efficiency.
Setting Up Your Zoho Recruit Test/Sandbox Account
Before you can start using the Zoho Recruit API to retrieve candidate information, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Zoho Recruit Account
If you don't already have a Zoho Recruit account, you can sign up for a free trial or use the free version. Visit the Zoho Recruit website and follow the instructions to create your account. Once your account is set up, log in to access the Zoho Recruit dashboard.
Registering a Client Application for OAuth Authentication
Zoho Recruit uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials.
- Go to the Zoho Developer Console.
- Select the client type as "Java Script" since you'll be working with JavaScript.
- Enter the required details:
- Client Name: The name of your application.
- Homepage URL: The URL of your web application.
- Authorized Redirect URIs: A valid URL where Zoho will redirect after successful authentication.
- Click "CREATE" to register your application.
Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for making API calls.
Generating Access and Refresh Tokens
To interact with the Zoho Recruit API, you'll need to generate access and refresh tokens. Follow these steps:
- Make an authorization request to Zoho's OAuth server using the following URL format:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoRecruit.modules.ALL&client_id=YOUR_CLIENT_ID&response_type=code&access_type=offline&redirect_uri=YOUR_REDIRECT_URI
- After successful authorization, you'll receive an authorization code at your redirect URI.
- Exchange the authorization code for access and refresh tokens by making a POST request to:
https://accounts.zoho.com/oauth/v2/token?grant_type=authorization_code&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&code=AUTHORIZATION_CODE
Store the access and refresh tokens securely. The access token is valid for one hour, while the refresh token can be used to obtain new access tokens.
For more detailed information, refer to the Zoho Recruit OAuth Overview and Register Client with Zoho documentation.
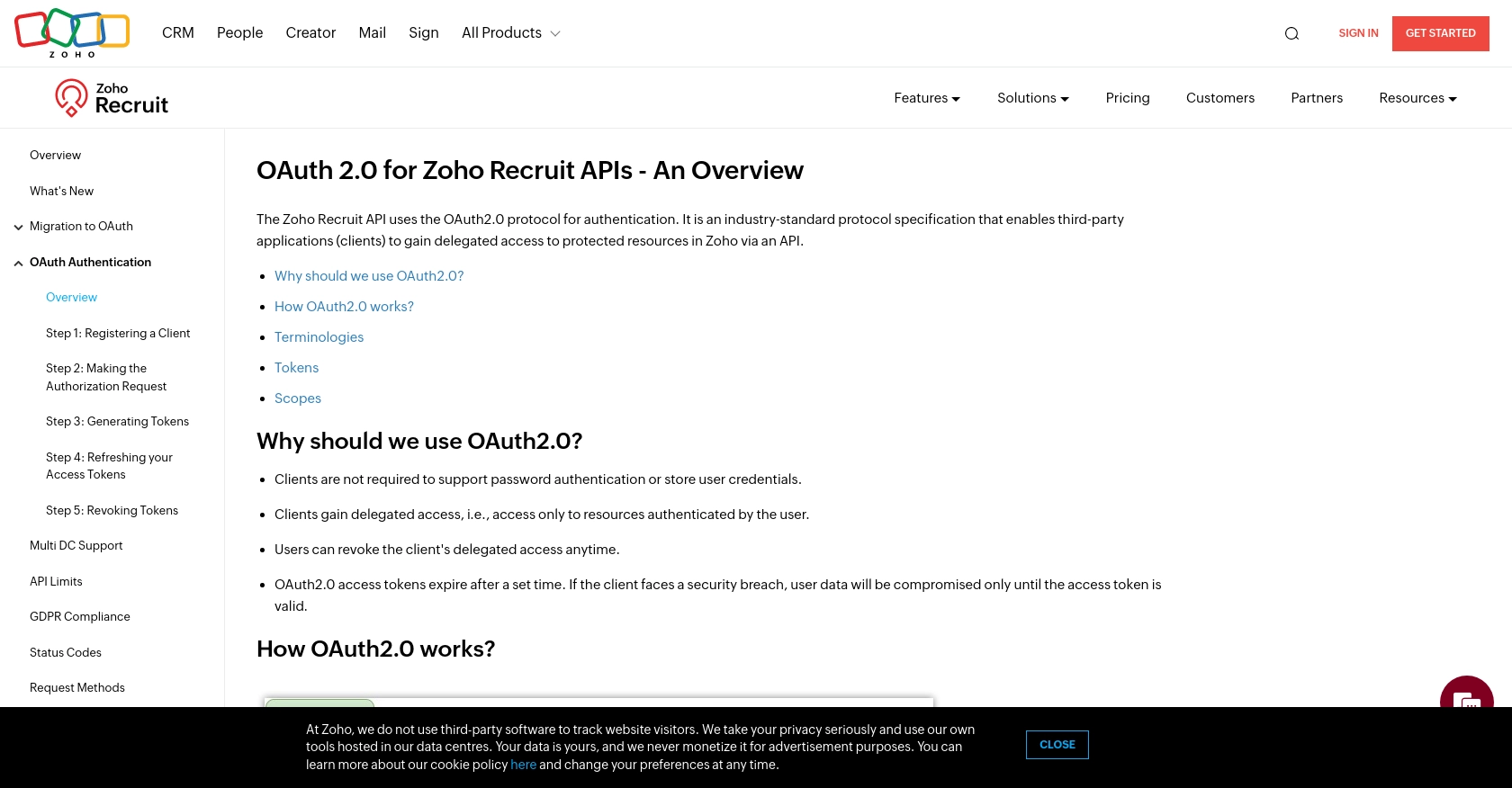
sbb-itb-96038d7
Making API Calls to Retrieve Candidates from Zoho Recruit Using JavaScript
Once you have set up your Zoho Recruit account and obtained the necessary OAuth credentials, you can start making API calls to retrieve candidate information. This section will guide you through the process of using JavaScript to interact with the Zoho Recruit API.
Prerequisites for Using JavaScript with Zoho Recruit API
Before proceeding, ensure you have the following:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Example Code to Retrieve Candidates from Zoho Recruit
Below is a sample JavaScript code snippet to fetch candidate records from Zoho Recruit:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://recruit.zoho.com/recruit/v2/Candidates';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
};
// Function to get candidates
async function getCandidates() {
try {
const response = await axios.get(endpoint, { headers });
const candidates = response.data.data;
console.log('Candidates:', candidates);
} catch (error) {
console.error('Error fetching candidates:', error.response.data);
}
}
// Call the function
getCandidates();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup.
Understanding the API Response and Handling Errors
When the API call is successful, you will receive a JSON response containing the candidate data. You can access this data and manipulate it as needed in your application.
It's crucial to handle potential errors when making API calls. Common error codes include:
- 400 BAD REQUEST: Invalid request or authentication.
- 401 AUTHORIZATION ERROR: Invalid API key provided.
- 403 FORBIDDEN: No permission to perform the operation.
- 429 TOO MANY REQUESTS: API request limit exceeded.
For more detailed error information, refer to the Zoho Recruit Status Codes documentation.
Verifying API Call Success in Zoho Recruit Sandbox
After executing the API call, verify the retrieved data by checking the candidate records in your Zoho Recruit sandbox account. Ensure the data matches the expected output.
For additional details on making API calls, refer to the Zoho Recruit Get Records API documentation.
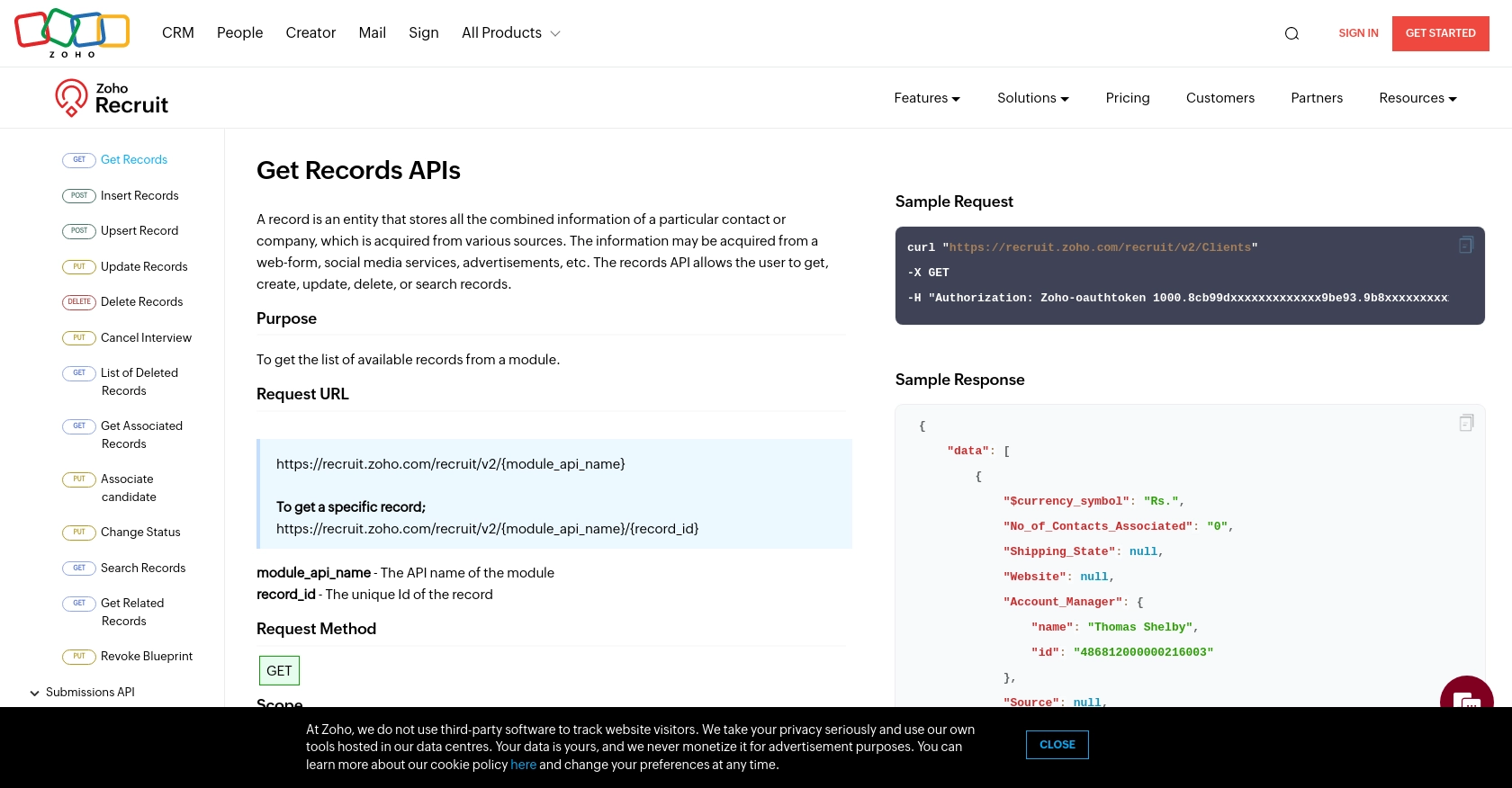
Conclusion and Best Practices for Using Zoho Recruit API with JavaScript
Integrating with the Zoho Recruit API using JavaScript can significantly enhance your recruitment processes by automating tasks and seamlessly connecting with other HR systems. By following the steps outlined in this guide, you can efficiently retrieve candidate data and incorporate it into your applications.
Best Practices for Secure and Efficient API Integration with Zoho Recruit
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and tokens securely. Avoid exposing them in public repositories or client-side code.
- Handling Rate Limits: Be mindful of Zoho Recruit's API rate limits. The API allows a specific number of requests per 24-hour period, so plan your calls accordingly to avoid hitting the limit. For more details, refer to the Zoho Recruit API Limits documentation.
- Refreshing Tokens: Remember that access tokens expire after one hour. Use the refresh token to obtain new access tokens without requiring user re-authentication.
- Error Handling: Implement robust error handling to manage potential issues such as invalid requests or exceeded rate limits. Refer to the Zoho Recruit Status Codes for guidance.
- Data Standardization: Ensure that data retrieved from Zoho Recruit is standardized and transformed as needed to fit your application's requirements.
Streamlining Integrations with Endgrate
While integrating with Zoho Recruit can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho Recruit. This allows you to focus on your core product while outsourcing integration management.
With Endgrate, you can build once for each use case, reducing the need for multiple integrations and offering an intuitive experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohorecruit
- https://www.zoho.com/recruit/developer-guide/apiv2/oauth-overview.html
- https://www.zoho.com/recruit/developer-guide/apiv2/register-client.html
- https://www.zoho.com/recruit/developer-guide/apiv2/limits.html
- https://www.zoho.com/recruit/developer-guide/apiv2/status-codes.html
- https://www.zoho.com/recruit/developer-guide/apiv2/get-records.html
Ready to get started?