Using the Shopify API to Get Collections (with Python examples)
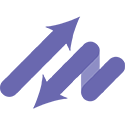
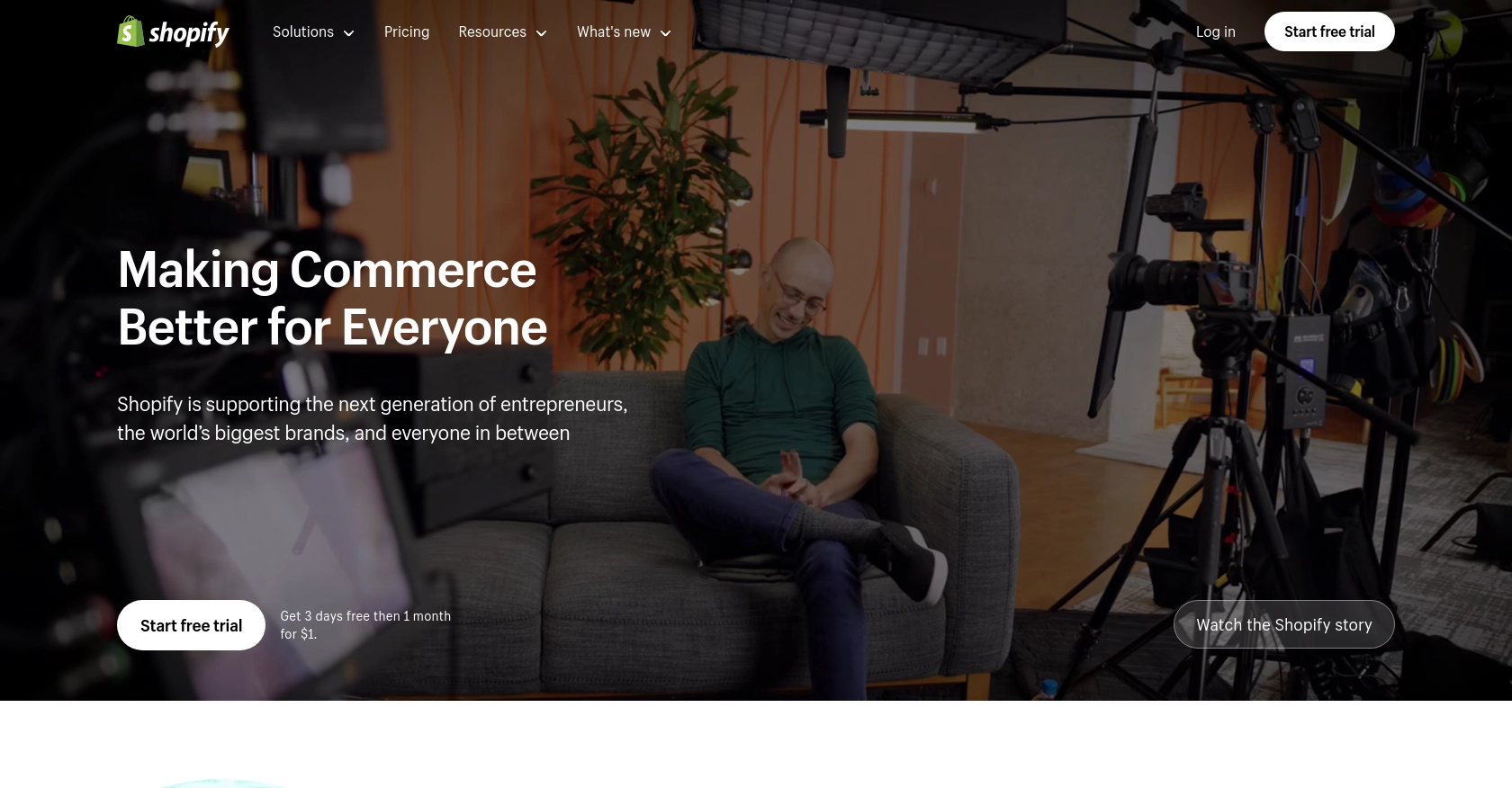
Introduction to Shopify API for Collections
Shopify is a leading e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a robust set of tools and APIs that allow developers to build custom integrations and enhance the functionality of Shopify stores.
Integrating with the Shopify API enables developers to access and manipulate various store resources, such as products, orders, and collections. Collections in Shopify are groupings of products that make it easier for merchants to organize and display their inventory. For example, a developer might use the Shopify API to retrieve a list of collections and display them on a custom storefront, enhancing the shopping experience for customers.
This article will guide you through using Python to interact with the Shopify API, specifically focusing on retrieving collections. By the end of this tutorial, you'll understand how to authenticate with Shopify's API and make API calls to access collection data.
Setting Up Your Shopify Test Account
Before you can start interacting with the Shopify API, you'll need to set up a test account. Shopify provides a development environment that allows developers to create and test apps without affecting live stores. This is essential for ensuring your integration works smoothly before deploying it to production.
Creating a Shopify Partner Account
To begin, you'll need to create a Shopify Partner account. This account will allow you to create development stores where you can test your app integrations.
- Visit the Shopify Partners page and sign up for a free account.
- Fill in the required information and follow the prompts to complete the registration process.
Setting Up a Shopify Development Store
Once your Partner account is ready, you can create a development store:
- Log in to your Shopify Partner dashboard.
- Navigate to the Stores section and click on Add store.
- Select Development store and fill in the necessary details, such as store name and password.
- Click Save to create your development store.
Creating a Shopify App for OAuth Authentication
Shopify uses OAuth for authentication, which requires you to create an app to obtain the necessary credentials.
- In your Shopify Partner dashboard, go to the Apps section and click on Create app.
- Enter your app name and select the development store you created earlier.
- Under App setup, note down the API key and API secret key. These will be used for authenticating API requests.
- Set the App URL and Redirect URL to your development environment's URL.
- Under Scopes, ensure you request the necessary permissions for accessing collections, such as
read_products
andwrite_products
. - Save your app settings.
With your Shopify development store and app set up, you're now ready to authenticate and interact with the Shopify API using Python. In the next section, we'll walk through making API calls to retrieve collection data.
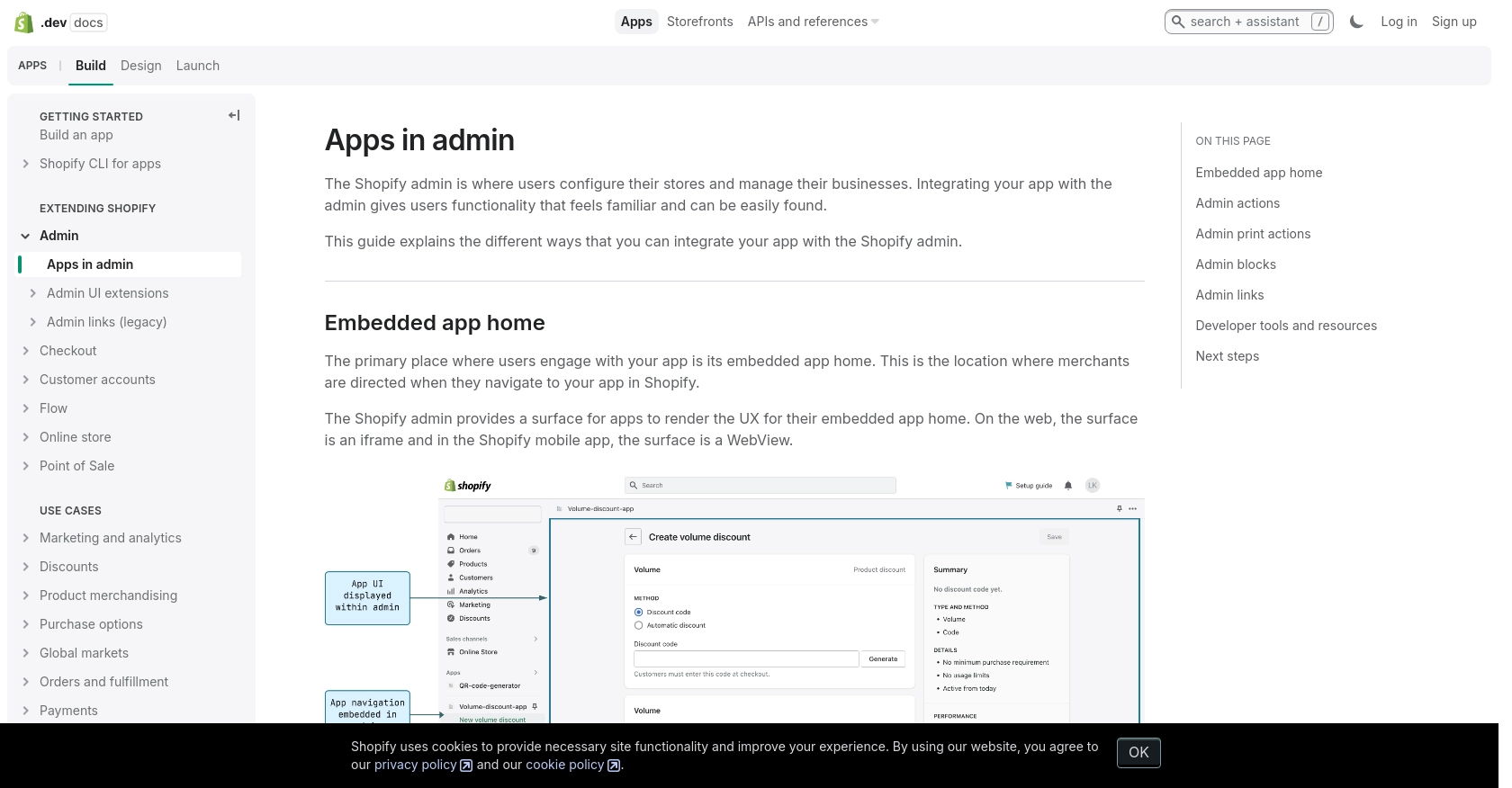
sbb-itb-96038d7
Making API Calls to Retrieve Shopify Collections Using Python
To interact with the Shopify API and retrieve collections, you'll need to use Python. This section will guide you through setting up your Python environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for Shopify API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Open your terminal or command prompt and install the
requests
library using pip:
pip install requests
Writing Python Code to Retrieve Shopify Collections
With your environment ready, you can now write Python code to interact with the Shopify API. The following example demonstrates how to retrieve a list of collections from a Shopify store.
import requests
# Define the API endpoint and headers
store_name = "your-development-store"
api_version = "2024-07"
endpoint = f"https://{store_name}.myshopify.com/admin/api/{api_version}/collections.json"
headers = {
"Content-Type": "application/json",
"X-Shopify-Access-Token": "Your_Access_Token"
}
# Make a GET request to the Shopify API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
collections = response.json()
for collection in collections['collections']:
print(f"Collection ID: {collection['id']}, Title: {collection['title']}")
else:
print(f"Failed to retrieve collections: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained from your Shopify app setup. This script sends a GET request to the Shopify API to retrieve collections and prints their IDs and titles.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of collections printed in your terminal. If the request fails, the script will output an error message with the status code and response text.
Shopify API responses include status codes that indicate the result of your request. Common status codes include:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid authentication credentials.
- 403 Forbidden: Incorrect access scopes.
- 429 Too Many Requests: Rate limit exceeded.
For more information on error codes, refer to the Shopify API documentation.
Handling Shopify API Rate Limits
The Shopify API enforces a rate limit of 40 requests per app per store per minute. If you exceed this limit, you'll receive a 429 error. To handle rate limits, monitor the X-Shopify-Shop-Api-Call-Limit
header in API responses and implement retry logic with exponential backoff.
For detailed information on rate limits, visit the Shopify API rate limits documentation.
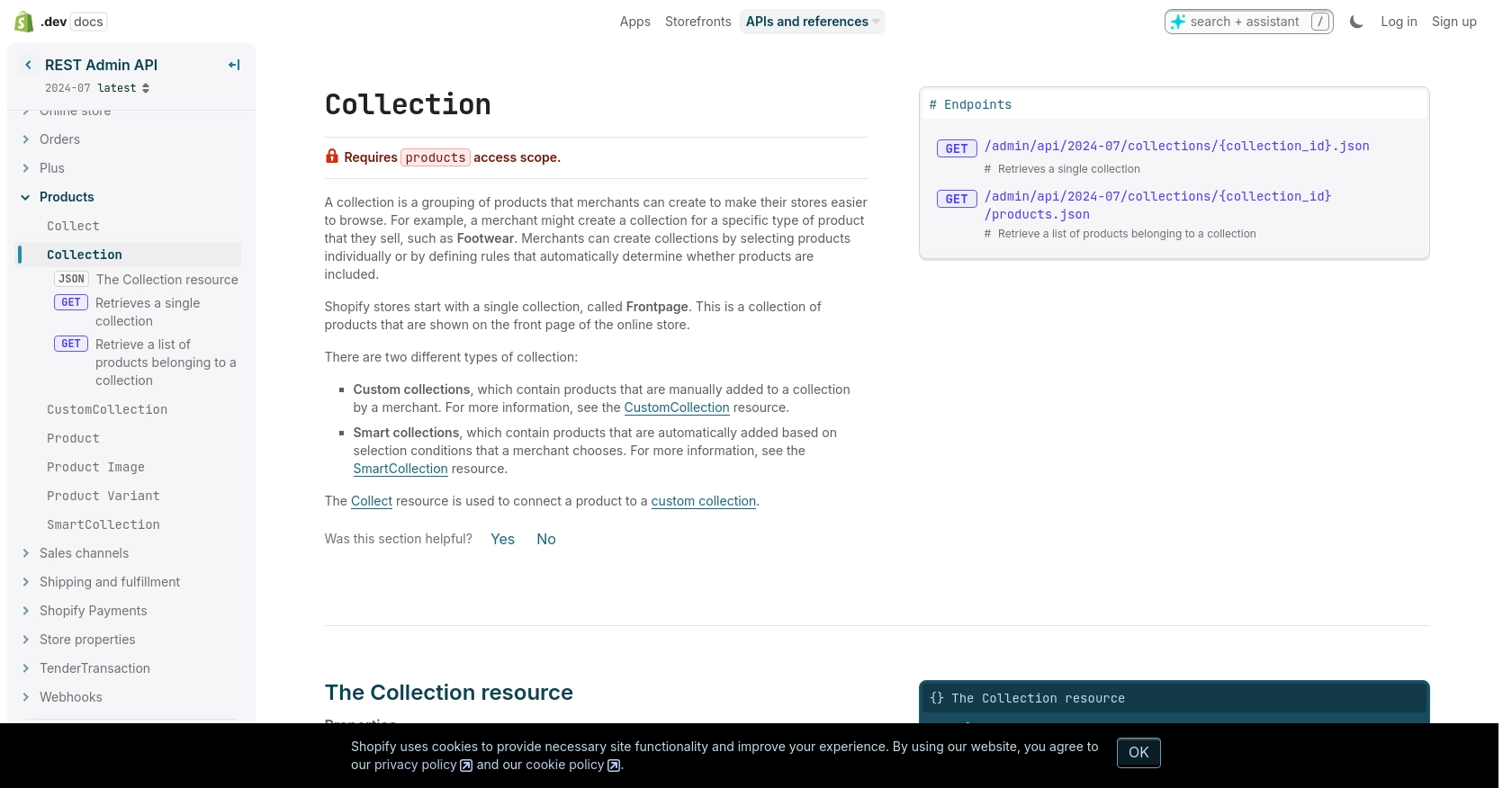
Conclusion and Best Practices for Using Shopify API with Python
Integrating with the Shopify API to retrieve collections using Python can significantly enhance the functionality and user experience of your e-commerce platform. By following the steps outlined in this guide, you can efficiently authenticate and interact with Shopify's robust API to access valuable collection data.
Best Practices for Secure and Efficient Shopify API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or a secure vault to keep your access tokens safe.
- Handle Rate Limits Gracefully: Implement logic to handle Shopify's rate limits by monitoring the
X-Shopify-Shop-Api-Call-Limit
header and using exponential backoff strategies for retries. - Optimize Data Handling: When retrieving large datasets, consider implementing pagination to efficiently manage data and reduce load times.
- Request Only Necessary Scopes: Limit the access scopes requested during app setup to only those necessary for your integration to minimize security risks.
Enhance Your Integration Strategy with Endgrate
While building custom integrations with Shopify can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to multiple platforms, including Shopify. This allows you to focus on your core product while outsourcing the intricacies of integration management.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate's website.
Read More
Ready to get started?