Using the Xero API to Create or Update vendors in Python
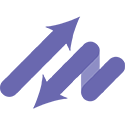
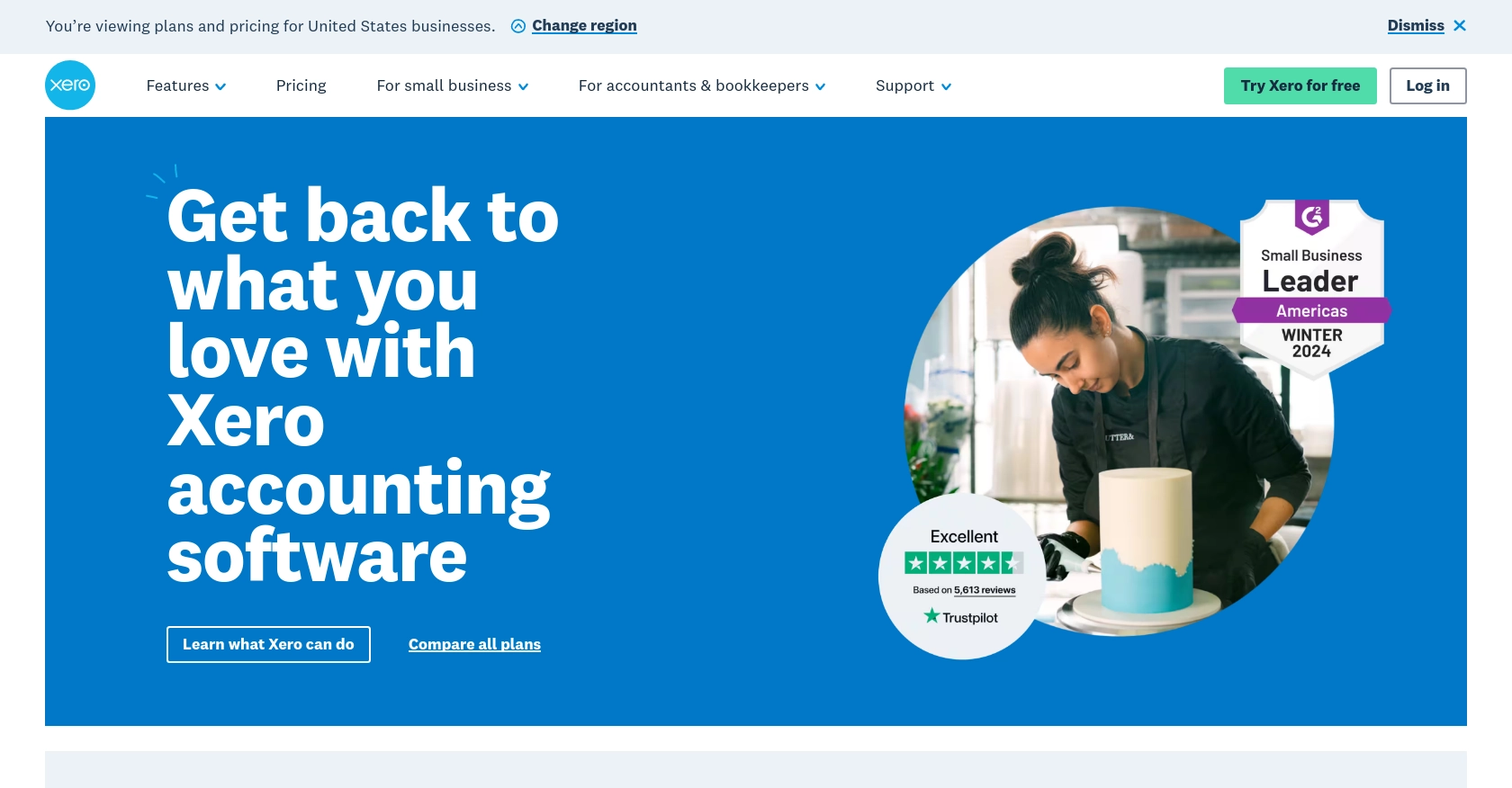
Introduction to Xero API for Vendor Management
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances with ease. It offers a wide range of features, including invoicing, bank reconciliation, and expense tracking, making it a popular choice for businesses looking to streamline their financial operations.
For developers, integrating with Xero's API can unlock the potential to automate and enhance financial processes. One common use case is managing vendor information. By using the Xero API, developers can create or update vendor records programmatically, ensuring that the financial data remains accurate and up-to-date across systems.
In this article, we'll explore how to use Python to interact with the Xero API for creating or updating vendor information. This integration can be particularly useful for businesses that need to synchronize vendor data between Xero and other platforms, reducing manual data entry and minimizing errors.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start integrating with the Xero API, you'll need to set up a sandbox account. This allows you to test your API interactions without affecting live data. Xero provides a demo company that you can use for this purpose.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal and sign up for a developer account.
- Once registered, log in to your developer dashboard.
Accessing the Xero Demo Company
After setting up your developer account, you can access the Xero demo company:
- Navigate to the "My Apps" section in your developer dashboard.
- Select "Try the Demo Company" to access a pre-configured sandbox environment.
Creating a Xero App for OAuth Authentication
Since the Xero API uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials:
- In the "My Apps" section, click on "New App."
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI to a valid URL where Xero will send the authorization code.
- Once the app is created, note down the client ID and client secret, as you'll need these for authentication.
Configuring OAuth 2.0 Scopes
To interact with vendor data, you'll need to configure the appropriate scopes:
- In your app settings, navigate to the "Scopes" section.
- Select the scopes related to contacts and accounting, such as
accounting.contacts
.
Generating an Access Token
With your app set up, you can now generate an access token:
- Use the standard OAuth 2.0 authorization code flow to obtain an access token. Detailed instructions can be found in the Xero OAuth 2.0 documentation.
- Exchange the authorization code for an access token using your client ID and client secret.
With your sandbox account and app configured, you're ready to start making API calls to create or update vendors in Xero using Python.
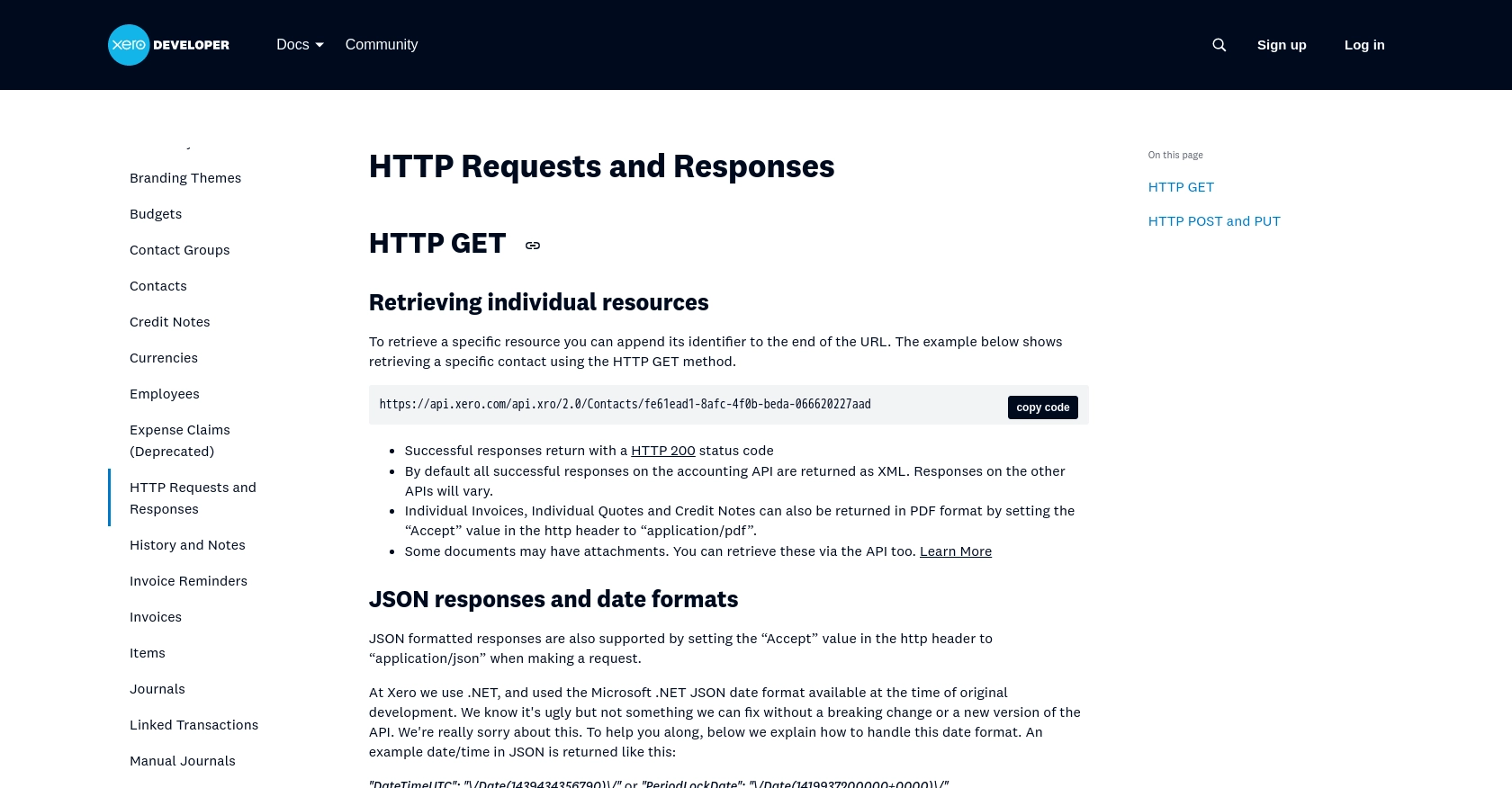
sbb-itb-96038d7
Making API Calls to Xero for Vendor Management Using Python
To interact with the Xero API for creating or updating vendor information, you'll need to write Python code that makes HTTP requests to the appropriate endpoints. This section will guide you through the process of setting up your Python environment and making the necessary API calls.
Setting Up Your Python Environment for Xero API Integration
Before you begin, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Creating or Updating Vendors in Xero Using Python
Now that your environment is set up, you can proceed to write the Python code to create or update vendors in Xero. Follow these steps:
Step 1: Import Required Libraries
Start by creating a new Python file named xero_vendor_management.py
and import the necessary libraries:
import requests
import json
Step 2: Define the API Endpoint and Headers
Set up the API endpoint and headers, including the access token you obtained earlier:
# Define the API endpoint for contacts
url = "https://api.xero.com/api.xro/2.0/Contacts"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
Replace Your_Access_Token
with the access token generated from your Xero app.
Step 3: Create or Update Vendor Data
Define the vendor data you want to create or update. Here's an example of how you might structure this data:
# Define the vendor data
vendor_data = {
"Contacts": [
{
"Name": "Vendor Name",
"EmailAddress": "vendor@example.com",
"ContactNumber": "123456789",
"AccountNumber": "VEND123"
}
]
}
Step 4: Make the API Call
Use the requests.post
method to send the data to Xero:
# Send the request to create or update the vendor
response = requests.post(url, headers=headers, data=json.dumps(vendor_data))
# Check the response status
if response.status_code == 200:
print("Vendor created or updated successfully.")
else:
print(f"Failed to create or update vendor. Status Code: {response.status_code}")
print(response.json())
Step 5: Verify the Request
After running the script, you can verify the vendor creation or update by checking the Xero demo company in your sandbox account. If successful, the vendor should appear in the contacts list.
Handling Errors and Xero API Rate Limits
When working with the Xero API, it's important to handle potential errors and be aware of rate limits. The Xero API documentation provides detailed information on error codes and rate limits, which you can find here.
For example, if you encounter a rate limit error, you may need to implement a retry mechanism or adjust the frequency of your API calls to stay within the allowed limits.
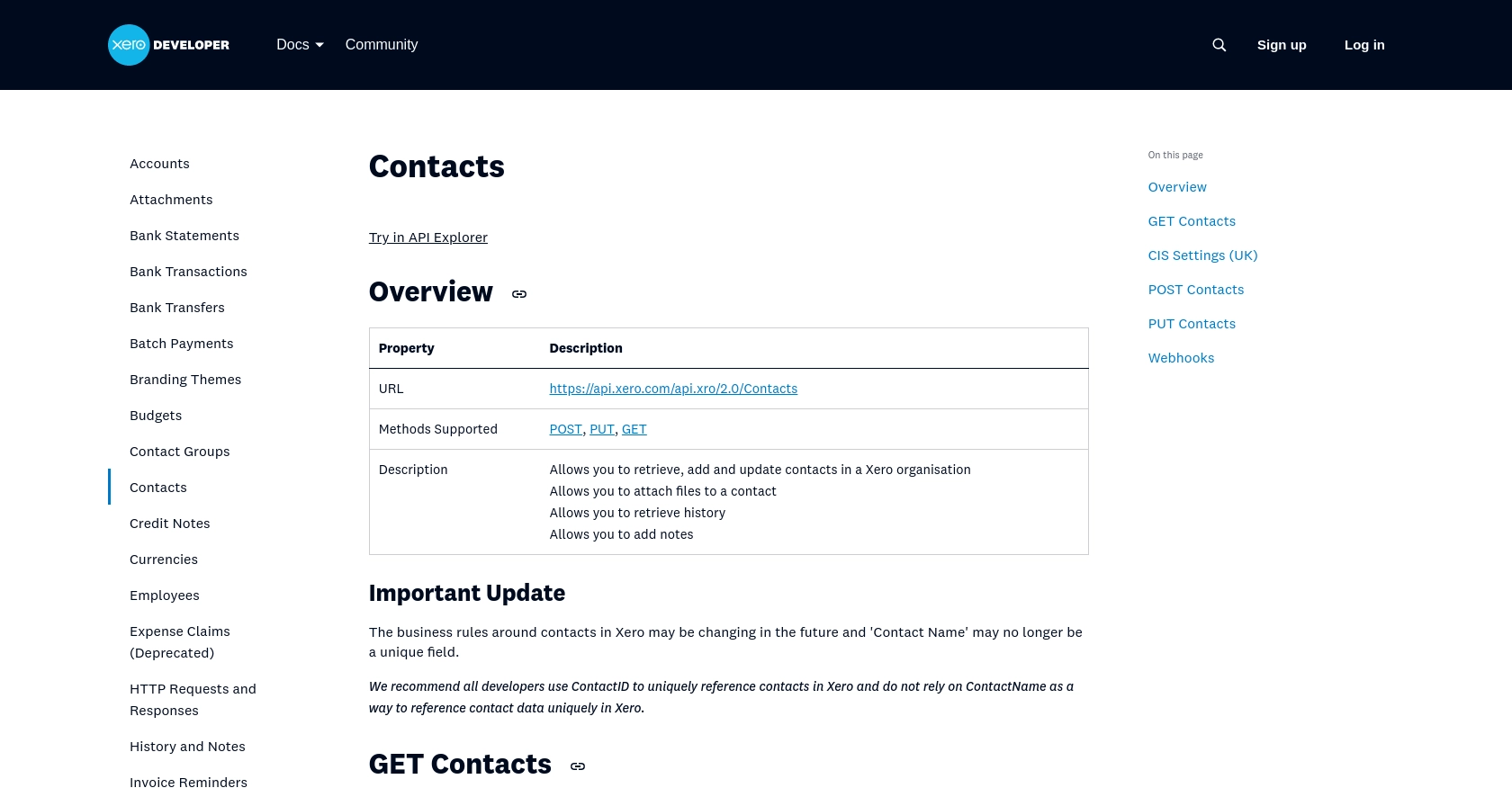
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API to manage vendor information using Python can significantly streamline your business operations by automating data synchronization and reducing manual entry errors. By following the steps outlined in this article, you can efficiently create or update vendor records in Xero, ensuring your financial data remains consistent across platforms.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limits: Be mindful of Xero's API rate limits. Implement retry mechanisms and optimize your API call frequency to avoid hitting these limits. For more details, refer to the Xero API rate limits documentation.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for troubleshooting and provide meaningful feedback to users when issues arise.
- Data Standardization: Ensure that vendor data is standardized before sending it to Xero. This helps maintain data consistency and prevents errors during API interactions.
Enhance Your Integration Strategy with Endgrate
While integrating with Xero's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Xero. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing integrations to streamline your business operations.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/contacts
Ready to get started?