How to Get Subscriptions with the Chargebee API in Python
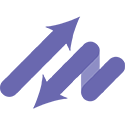
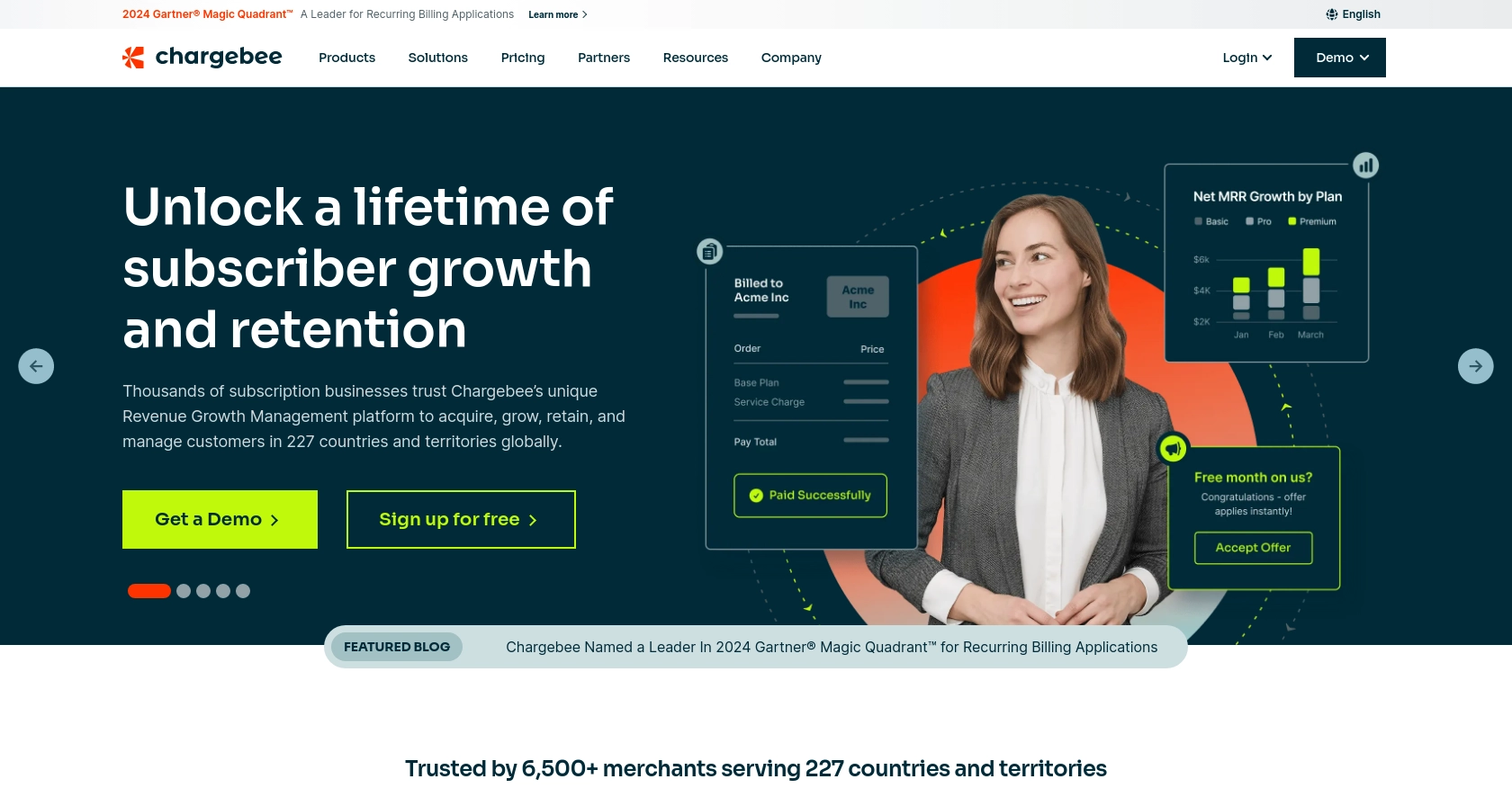
Introduction to Chargebee API Integration
Chargebee is a robust subscription management platform that simplifies billing, invoicing, and revenue operations for businesses. It offers a comprehensive suite of tools to manage subscriptions, automate recurring billing, and streamline financial workflows.
Integrating with Chargebee's API allows developers to efficiently manage subscription data and automate billing processes. For example, you can use the Chargebee API to retrieve subscription details, enabling seamless integration with your existing systems to enhance customer management and billing accuracy.
Setting Up Your Chargebee Test/Sandbox Account
Before you can start integrating with the Chargebee API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Chargebee Sandbox Account
- Visit the Chargebee signup page and register for a new account.
- Select the option to create a sandbox account during the signup process. This will provide you with a test environment to explore Chargebee's features.
- Once registered, log in to your Chargebee dashboard.
Generate API Keys for Chargebee Integration
Chargebee uses HTTP Basic authentication for API calls, where the username is your API key and the password is left empty. Follow these steps to generate your API keys:
- Navigate to the Settings section in your Chargebee dashboard.
- Under API & Webhooks, click on API Keys.
- Click on Create a Key and provide a name for your API key.
- Copy the generated API key and store it securely. You will use this key to authenticate your API requests.
Configure API Access and Permissions
Ensure that your API key has the necessary permissions to access subscription data:
- In the API Keys section, click on the key you created.
- Under Permissions, ensure that the key has access to subscription-related resources.
- Save any changes to update the permissions.
Test Your Chargebee API Setup
To verify that your setup is correct, you can make a simple API call to list subscriptions:
import requests
# Set the API endpoint
url = "https://{site}.chargebee.com/api/v2/subscriptions"
# Set the request headers
headers = {
"Authorization": "Basic {api_key}:"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Print the response
print(response.json())
Replace {site}
with your Chargebee site name and {api_key}
with your actual API key. If the setup is correct, you should receive a JSON response with subscription data.
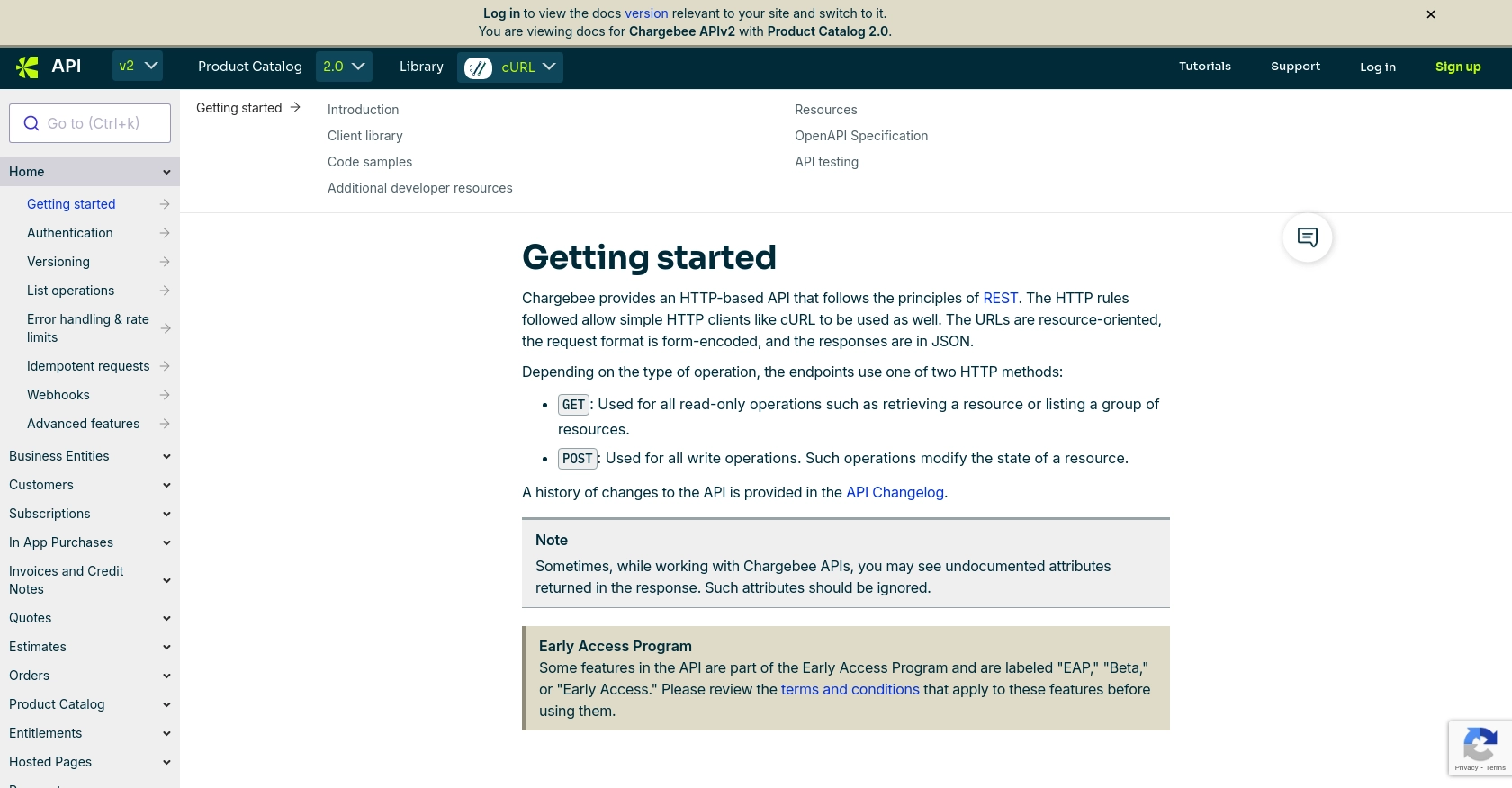
sbb-itb-96038d7
Making API Calls to Retrieve Subscriptions with Chargebee API in Python
To interact with the Chargebee API using Python, you'll need to ensure your environment is set up correctly. This involves using the appropriate Python version and installing necessary dependencies. Let's walk through the steps to make API calls to retrieve subscription data from Chargebee.
Setting Up Your Python Environment for Chargebee API Integration
Before making API calls, ensure you have Python installed on your machine. This guide uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Verify your Python version by running
python --version
in your terminal. - Install the
requests
library using pip:
pip install requests
Writing Python Code to Retrieve Subscriptions from Chargebee
With your environment ready, you can now write a Python script to retrieve subscriptions from Chargebee. Follow the example code below:
import requests
# Set the API endpoint and your Chargebee site name
site = "your_site_name"
url = f"https://{site}.chargebee.com/api/v2/subscriptions"
# Set the request headers with your API key
headers = {
"Authorization": "Basic your_api_key:"
}
# Make a GET request to the Chargebee API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse and print the JSON response
subscriptions = response.json()
for subscription in subscriptions['list']:
print(subscription['subscription'])
else:
print(f"Failed to retrieve subscriptions: {response.status_code} - {response.text}")
Replace your_site_name
with your actual Chargebee site name and your_api_key
with your API key. This script makes a GET request to the Chargebee API to list subscriptions and prints the subscription details if the request is successful.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The Chargebee API uses standard HTTP status codes to indicate success or failure:
- 2XX: Success
- 4XX: Client-side error (e.g., invalid request)
- 5XX: Server-side error
If you encounter a 429 Too Many Requests
error, it means you've exceeded the API rate limit. Chargebee's rate limits are approximately 750 API calls per 5 minutes for test sites and 150 calls per minute for live sites. Implementing exponential backoff with jitter can help manage rate limits effectively.
For more detailed error handling, refer to the Chargebee API error handling documentation.
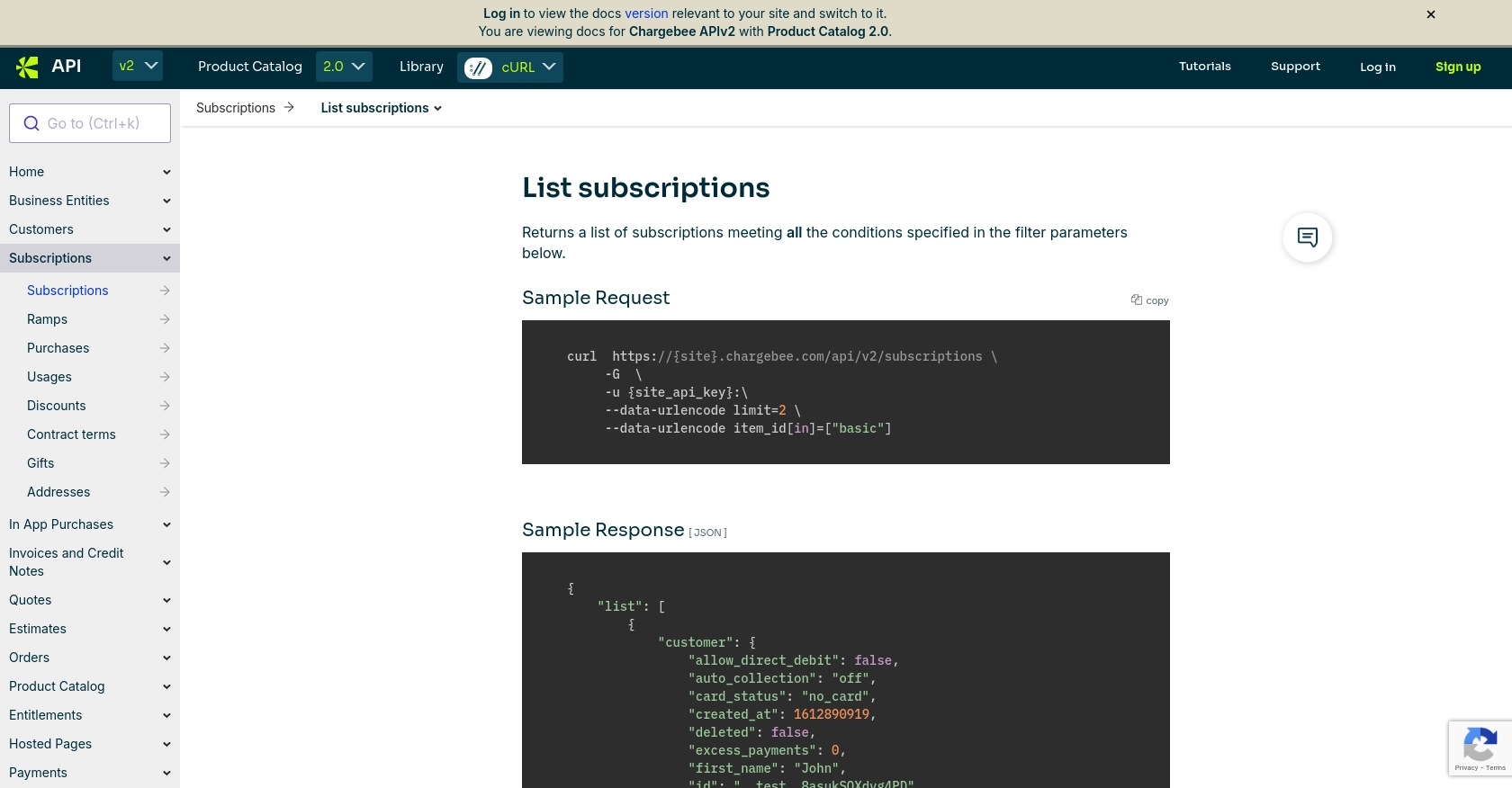
Conclusion and Best Practices for Chargebee API Integration
Integrating with the Chargebee API using Python provides a powerful way to manage subscriptions and automate billing processes. By following the steps outlined in this guide, you can efficiently retrieve subscription data and integrate it with your existing systems.
Best Practices for Secure and Efficient Chargebee API Usage
- Secure API Keys: Store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Implement exponential backoff with jitter to manage API rate limits effectively. Chargebee's rate limits are approximately 750 API calls per 5 minutes for test sites and 150 calls per minute for live sites.
- Error Handling: Use Chargebee's error codes to handle errors gracefully. Refer to the Chargebee API error handling documentation for detailed guidance.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your systems.
Streamline Your Integration Process with Endgrate
For developers looking to simplify and accelerate their integration efforts, consider using Endgrate. Endgrate offers a unified API endpoint that connects to multiple platforms, including Chargebee, allowing you to build integrations once and reuse them across different services. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate and discover the benefits of a streamlined integration process.
Read More
Ready to get started?