Using the Endear API to Create Tasks (with PHP examples)
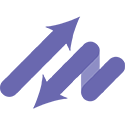
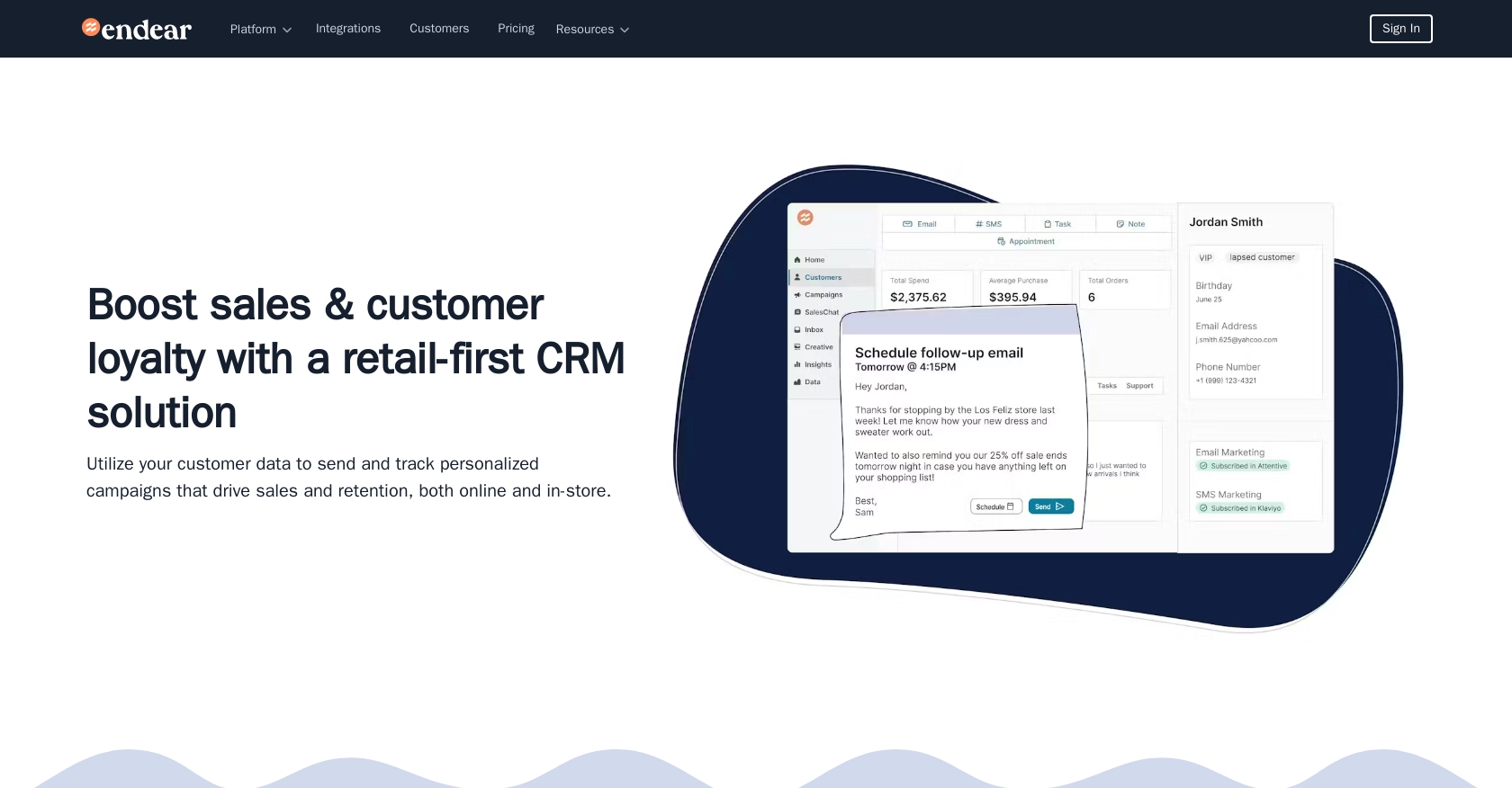
Introduction to Endear API for Task Management
Endear is a powerful CRM platform designed to enhance customer relationships through seamless communication and task management. It offers a robust API that allows developers to integrate and automate various functionalities, including task creation and management, directly within their applications.
Integrating with Endear's API can significantly streamline task management processes for developers working on B2B SaaS products. For example, a developer might want to create tasks automatically based on specific customer interactions or sales milestones, ensuring that teams remain organized and responsive to customer needs.
This article will guide you through using the Endear API to create tasks using PHP, providing step-by-step instructions and code examples to help you efficiently manage tasks within the Endear platform.
Setting Up Your Endear Test Account for API Integration
Before you can start creating tasks using the Endear API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started with your Endear test account:
Creating an Endear Account
If you don't already have an Endear account, you can sign up for a free trial or use a demo account. Visit the Endear website and follow the instructions to create your account. Once your account is set up, log in to access the dashboard.
Generating an API Key for Endear Integration
To interact with the Endear API, you'll need an API key. This key will authenticate your requests and ensure they are associated with your account. Follow these steps to generate your API key:
- Navigate to the Settings section in your Endear dashboard.
- Click on Integrations and then select Add Integration.
- Choose API from the options provided.
- Fill in the necessary details and submit the form to generate your API key.
Once generated, make sure to store your API key securely, as it will be required for authenticating your API requests.
Authenticating API Requests with Your API Key
With your API key in hand, you can now authenticate requests to the Endear API. All API calls should be made to the following endpoint:
https://api.endearhq.com/graphql
Include the following header in your requests to authenticate:
X-Endear-Api-Key: {{API_KEY}}
Replace {{API_KEY}}
with the API key you generated. This header will ensure that your requests are properly authenticated and associated with your account.
For more detailed information on authentication, refer to the Endear Authentication Documentation.
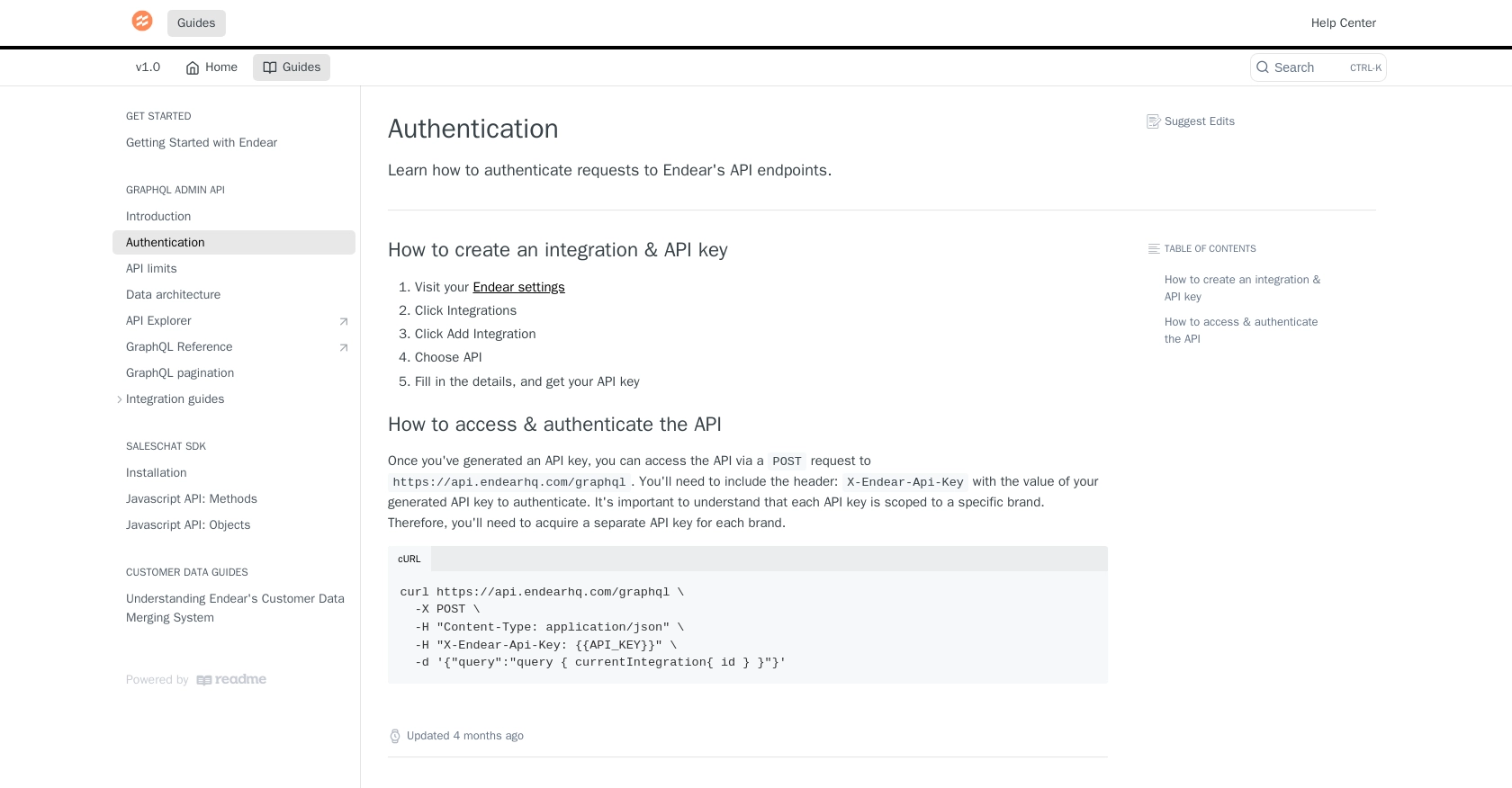
sbb-itb-96038d7
Making API Calls to Create Tasks with Endear Using PHP
To create tasks using the Endear API with PHP, you'll need to set up your environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up PHP, installing dependencies, and writing the code to make API calls.
Setting Up Your PHP Environment for Endear API Integration
Before making API calls, ensure you have PHP installed on your machine. You can download the latest version from the official PHP website. Additionally, you'll need the cURL
extension enabled to make HTTP requests.
To verify that cURL is enabled, create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL section in the output. If it's not enabled, you may need to modify your php.ini
file to enable it.
Installing Required PHP Dependencies for Endear API
To handle HTTP requests, we'll use the GuzzleHTTP
library. Install it using Composer by running the following command in your terminal:
composer require guzzlehttp/guzzle
Ensure Composer is installed on your machine. If not, you can download it from the Composer website.
Writing PHP Code to Create Tasks with Endear API
With your environment set up, you can now write the PHP code to create tasks using the Endear API. Create a new PHP file and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'YOUR_API_KEY';
$endpoint = 'https://api.endearhq.com/graphql';
$query = '
mutation {
createTask(input: {
note: "Follow up with client",
deadline: "2023-12-31T23:59:59Z"
}) {
task {
id
note
deadline
}
}
}';
$response = $client->post($endpoint, [
'headers' => [
'Content-Type' => 'application/json',
'X-Endear-Api-Key' => $apiKey,
],
'body' => json_encode(['query' => $query])
]);
$data = json_decode($response->getBody(), true);
if (isset($data['data']['createTask']['task'])) {
echo 'Task Created Successfully: ' . $data['data']['createTask']['task']['id'];
} else {
echo 'Failed to Create Task';
}
?>
Replace YOUR_API_KEY
with the API key you generated earlier. This script sends a GraphQL mutation to create a new task with a note and deadline. If successful, it will output the task ID.
Verifying Task Creation in Endear
After running the script, log in to your Endear account and navigate to the tasks section to verify that the task was created successfully. You should see the new task listed with the details provided in your API call.
Handling Errors and Rate Limits with Endear API
When making API calls, it's essential to handle potential errors. The Endear API may return HTTP 429 errors if you exceed the rate limit of 120 requests per minute. Implement error handling in your code to manage these scenarios gracefully.
For more information on rate limits and error codes, refer to the Endear Rate Limits and Errors Documentation.
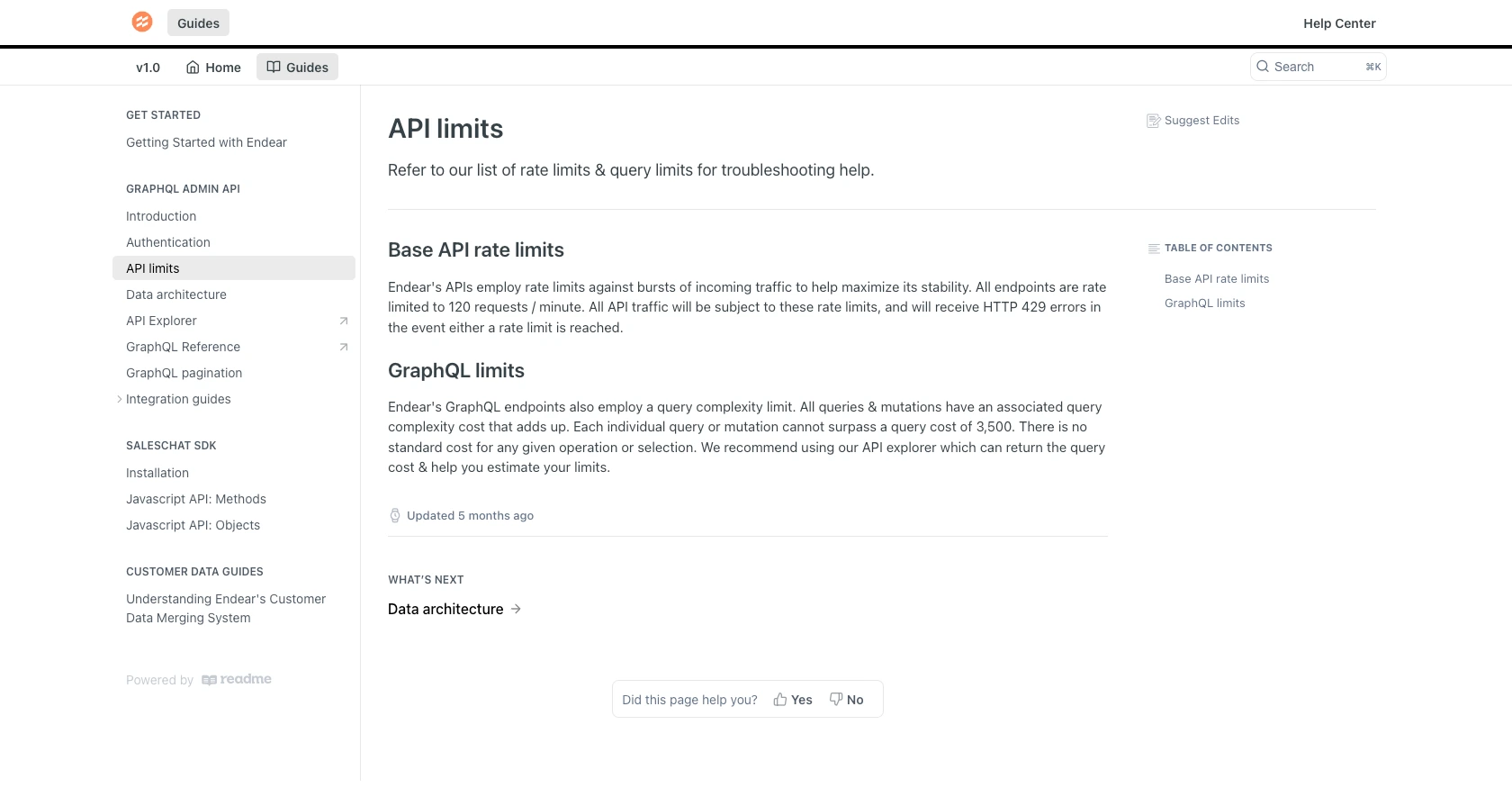
Conclusion and Best Practices for Using Endear API in PHP
Integrating with the Endear API to manage tasks can greatly enhance the efficiency of your B2B SaaS applications. By automating task creation and management, you ensure that your team remains organized and responsive to customer needs. However, it's crucial to follow best practices to maintain security and performance.
Best Practices for Secure API Key Management
- Store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Regularly rotate your API keys to minimize security risks.
- Limit the scope of your API keys to only the necessary permissions required for your application.
Handling Rate Limits and Optimizing API Calls
- Be mindful of Endear's rate limit of 120 requests per minute. Implement retry logic with exponential backoff to handle HTTP 429 errors gracefully.
- Batch API requests where possible to reduce the number of calls and stay within rate limits.
Data Transformation and Standardization
- Ensure data consistency by standardizing fields and formats before sending data to Endear.
- Use unique identifiers to manage data integration and avoid conflicts between different data sources.
Enhancing Integration with Endgrate
While integrating with Endear's API can streamline your task management, managing multiple integrations can be complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Endear. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Task
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?