Using the BigCommerce API to Create Or Update Products (with Javascript examples)
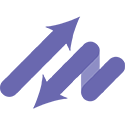
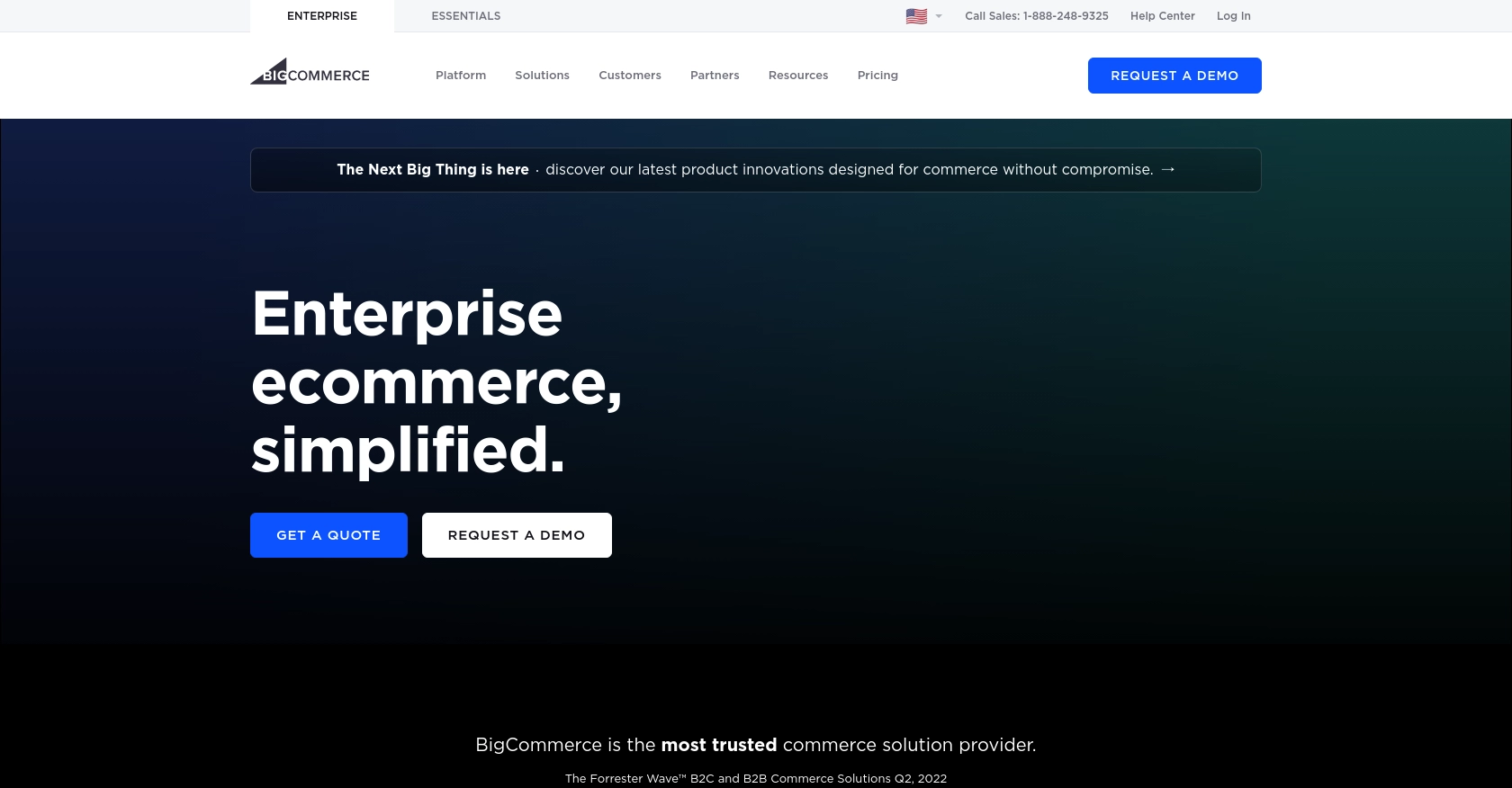
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a comprehensive suite of tools for managing products, orders, and customer relationships, making it a popular choice for businesses looking to scale their online presence.
Integrating with the BigCommerce API allows developers to automate and streamline various e-commerce operations. For example, you can use the API to create or update products in your store, ensuring that your inventory is always up-to-date and accurate. This can be particularly useful for businesses that manage large product catalogs or frequently update their offerings.
In this article, we'll explore how to use JavaScript to interact with the BigCommerce API, focusing on creating and updating products. By the end of this guide, you'll have a clear understanding of how to leverage the BigCommerce API to enhance your e-commerce operations.
Setting Up Your BigCommerce Test or Sandbox Account
Before you can start integrating with the BigCommerce API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live store data.
Creating a BigCommerce Sandbox Account
To get started, follow these steps to create a sandbox account:
- Visit the BigCommerce Sandbox page and sign up for a free sandbox account.
- Fill out the required information, including your email and store details, to create your sandbox store.
- Once your account is set up, you'll receive an email with your store's URL and login credentials.
Generating API Credentials for BigCommerce
After setting up your sandbox account, you'll need to generate API credentials to authenticate your requests:
- Log in to your BigCommerce control panel using your sandbox account credentials.
- Navigate to Advanced Settings > API Accounts.
- Click on Create API Account and select Create V2/V3 API Token.
- Fill in the details for your API account, including the name and permissions required for your integration.
- Click Save to generate your API credentials, including the
X-Auth-Token
. - Make sure to copy and securely store your
Client ID
,Client Secret
, andAccess Token
as they will be needed for API authentication.
Understanding BigCommerce API Authentication
BigCommerce uses OAuth-based authentication for API requests. You'll need to include the X-Auth-Token
in the header of your API calls to authenticate successfully. For more details, refer to the BigCommerce Authentication Documentation.
With your sandbox account and API credentials set up, you're ready to start making API calls to create or update products in your BigCommerce store using JavaScript.
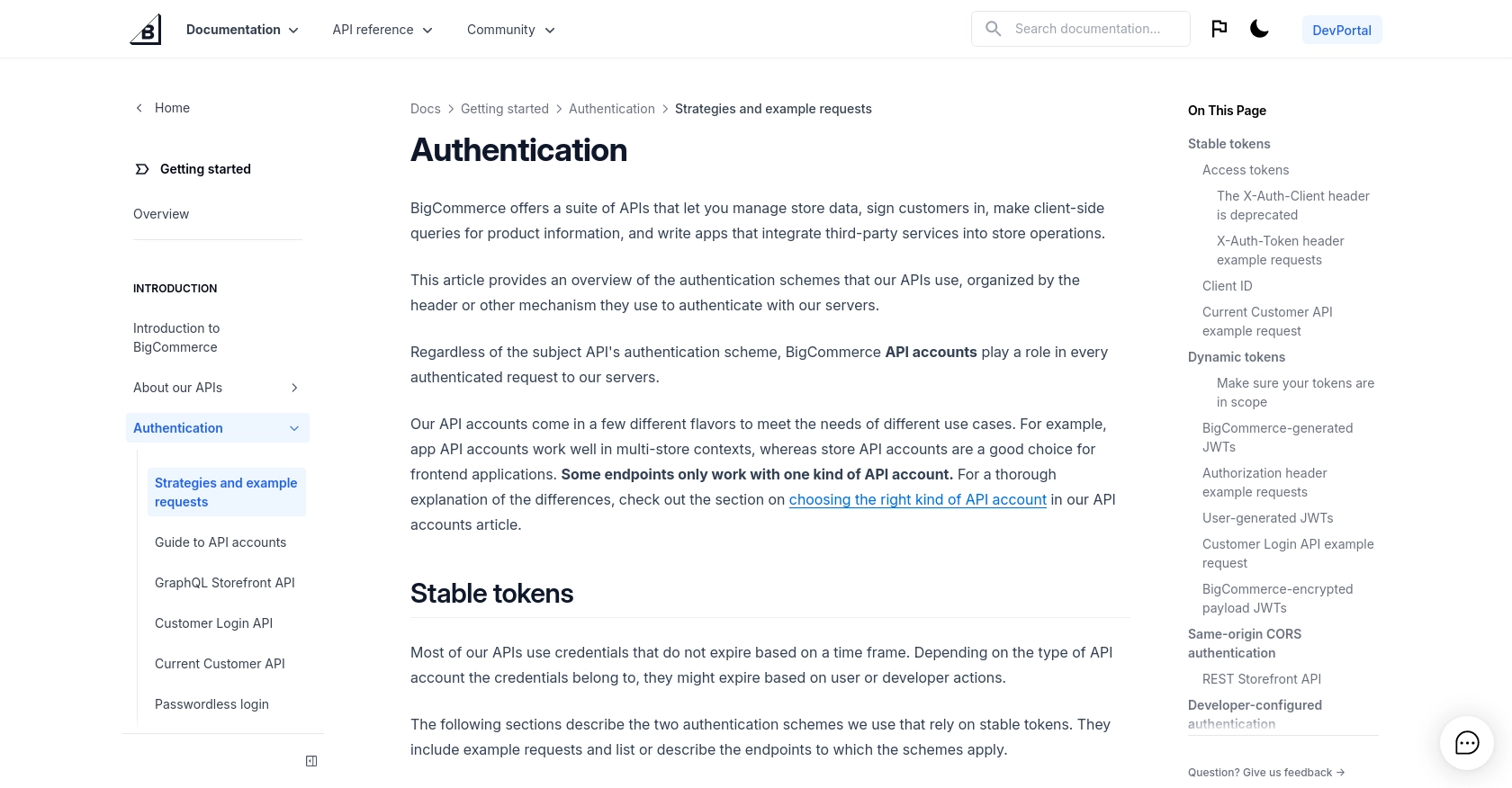
sbb-itb-96038d7
How to Make API Calls to BigCommerce for Product Management Using JavaScript
To interact with the BigCommerce API for creating or updating products, you'll need to use JavaScript to send HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for BigCommerce API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
- Familiarity with JavaScript and HTTP requests.
You'll also need to install the axios
library, which simplifies making HTTP requests. Run the following command in your terminal:
npm install axios
Creating a Product in BigCommerce Using JavaScript
To create a product in BigCommerce, you'll send a POST request to the API endpoint. Here's an example of how to do this using JavaScript and the axios
library:
const axios = require('axios');
const createProduct = async () => {
const url = 'https://api.bigcommerce.com/stores/{store_hash}/v3/catalog/products';
const headers = {
'X-Auth-Token': 'your_access_token',
'Content-Type': 'application/json',
'Accept': 'application/json'
};
const productData = {
name: 'New Product',
type: 'physical',
weight: 1,
price: 19.99
};
try {
const response = await axios.post(url, productData, { headers });
console.log('Product Created:', response.data);
} catch (error) {
console.error('Error creating product:', error.response.data);
}
};
createProduct();
Replace your_access_token
and {store_hash}
with your actual access token and store hash. This code sends a request to create a new product with specified attributes.
Updating a Product in BigCommerce Using JavaScript
To update an existing product, you'll use a PUT request. Here's how you can update a product's details:
const updateProduct = async (productId) => {
const url = `https://api.bigcommerce.com/stores/{store_hash}/v3/catalog/products/${productId}`;
const headers = {
'X-Auth-Token': 'your_access_token',
'Content-Type': 'application/json',
'Accept': 'application/json'
};
const updatedData = {
price: 24.99,
description: 'Updated product description'
};
try {
const response = await axios.put(url, updatedData, { headers });
console.log('Product Updated:', response.data);
} catch (error) {
console.error('Error updating product:', error.response.data);
}
};
updateProduct(123);
Replace 123
with the ID of the product you wish to update. This code modifies the price and description of the specified product.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Successful requests will return a status code of 200
or 201
, while errors might return codes like 404
or 422
. Always check the response data and handle errors gracefully to ensure a robust integration.
For more details on error codes, refer to the BigCommerce API documentation.
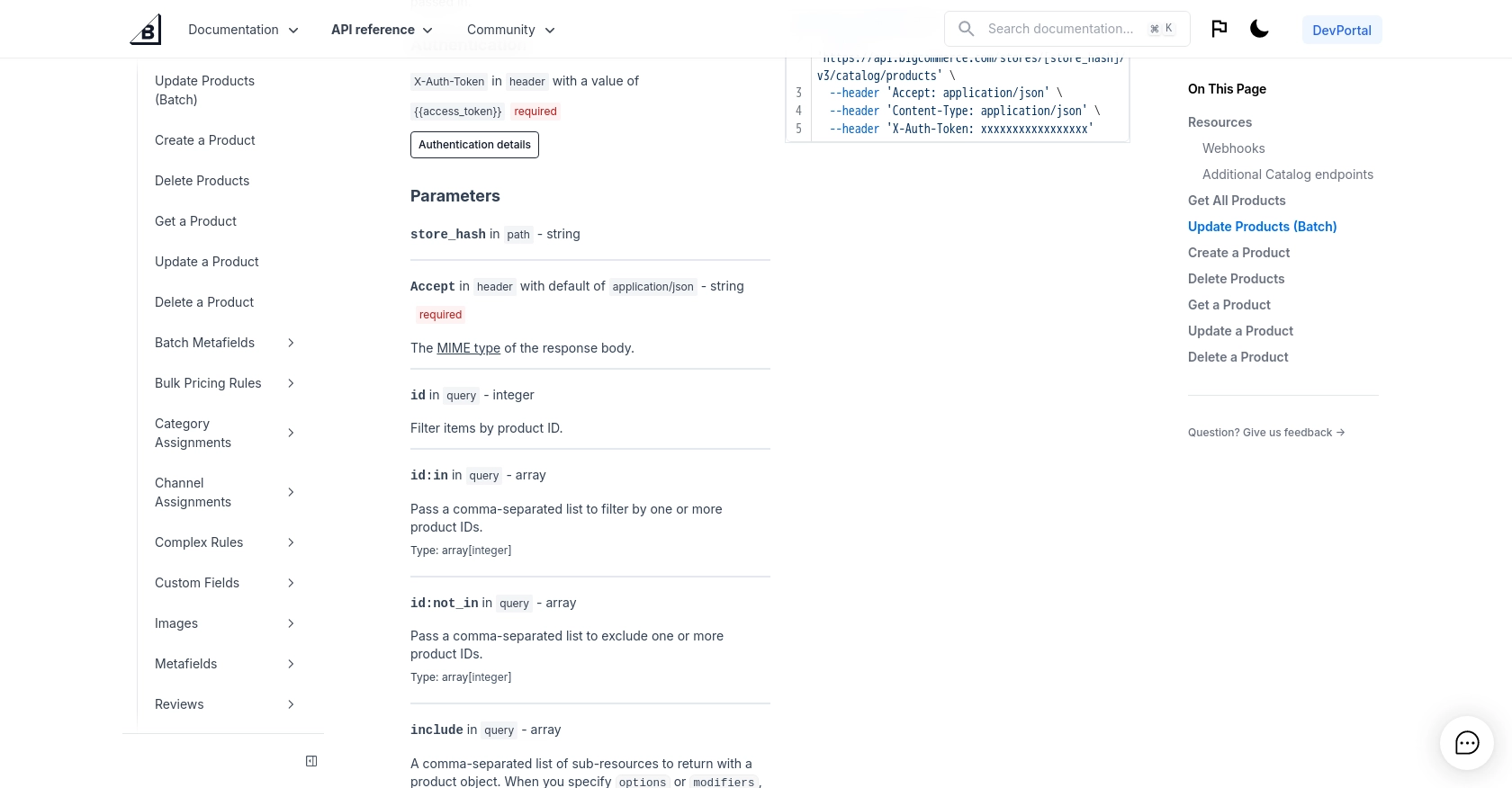
Conclusion and Best Practices for BigCommerce API Integration
Integrating with the BigCommerce API using JavaScript provides a powerful way to automate and manage your e-commerce operations. By following the steps outlined in this guide, you can efficiently create and update products, ensuring your store's inventory remains accurate and up-to-date.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and consistent across your application to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling to manage API response codes effectively. Refer to the BigCommerce API documentation for detailed error code information.
Enhance Your Integration Strategy with Endgrate
While integrating with the BigCommerce API can significantly enhance your e-commerce operations, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including BigCommerce.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can simplify your integration processes.
Read More
Ready to get started?