Using the Salesloft API to Get Notes (with Javascript examples)
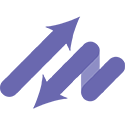
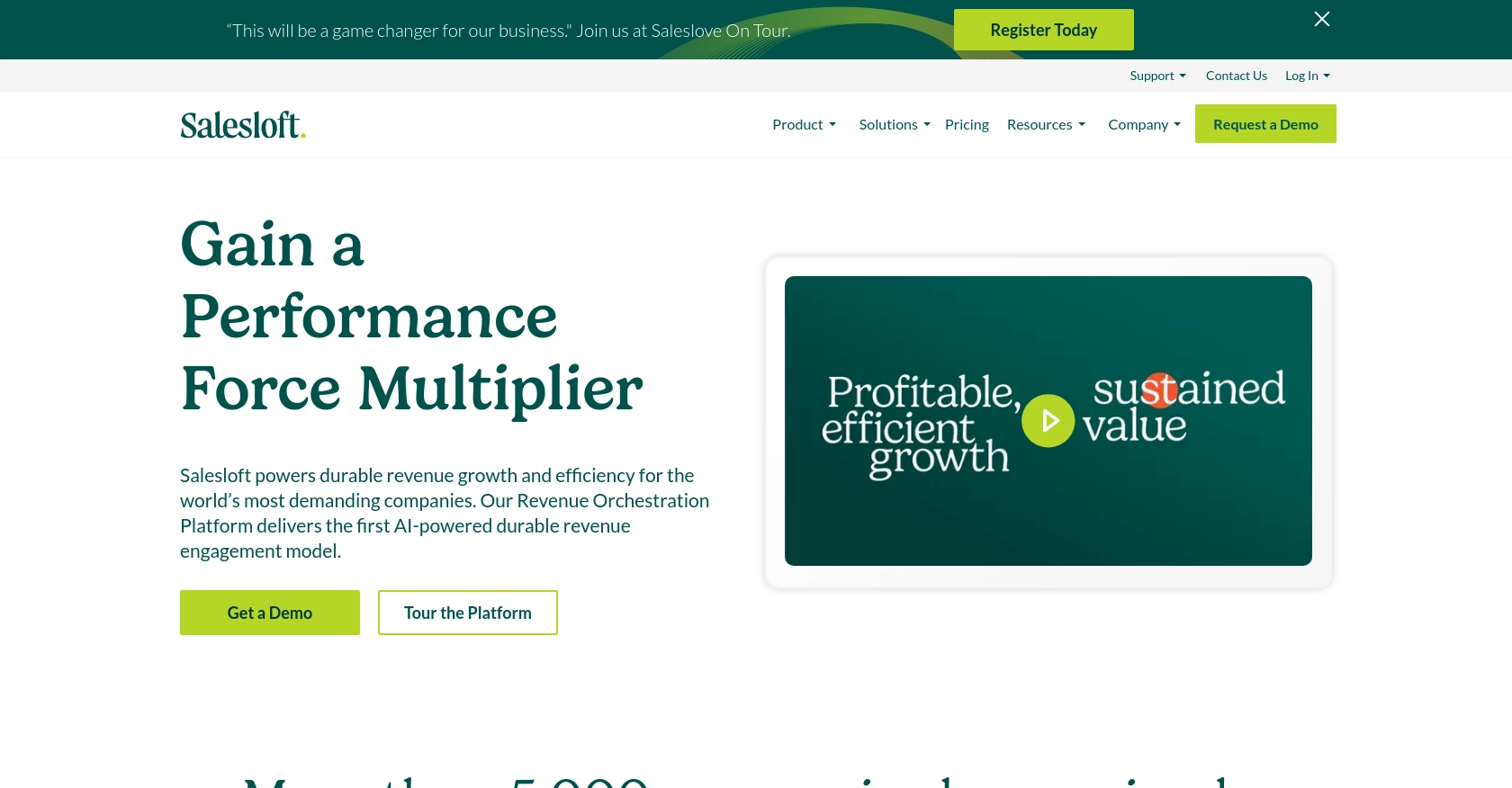
Introduction to Salesloft API Integration
Salesloft is a premier sales engagement platform that empowers sales teams to enhance their communication and streamline their workflows. By offering a suite of tools designed to optimize sales processes, Salesloft enables businesses to connect with prospects more effectively and close deals faster.
Developers may want to integrate with the Salesloft API to access and manage sales data, such as notes, which can be crucial for tracking interactions and insights. For example, a developer might use the Salesloft API to retrieve notes associated with specific sales activities, allowing for better analysis and reporting of sales performance.
Setting Up Your Salesloft Test Account for API Integration
Before diving into the Salesloft API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Salesloft provides a sandbox environment through its OAuth authentication process, which is essential for secure API interactions.
Creating a Salesloft Sandbox Account
If you don't have a Salesloft account, start by signing up for a free trial or demo account on the Salesloft website. This will give you access to the necessary features for testing API integrations.
- Visit the Salesloft website and navigate to the sign-up page.
- Follow the instructions to create your account. Once completed, log in to your Salesloft dashboard.
Setting Up OAuth Authentication for Salesloft API
Salesloft uses OAuth 2.0 for authentication, which involves creating an app within your Salesloft account. This process will provide you with the client ID and client secret needed for API access.
- Navigate to Your Applications in your Salesloft account settings.
- Select OAuth Applications and click on Create New.
- Fill in the required fields and save your application. You will receive your Application ID (Client ID) and Secret (Client Secret).
Obtaining Authorization Code and Access Tokens
To interact with the Salesloft API, you need to obtain an access token. Follow these steps to complete the OAuth flow:
- Generate a request to the authorization endpoint using your client ID and redirect URI:
- Direct users to this URL to authorize your application. Upon approval, you'll receive a code in the redirect URI.
- Exchange this code for an access token by making a POST request:
const authUrl = `https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code`;
fetch('https://accounts.salesloft.com/oauth/token', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
client_id: 'YOUR_CLIENT_ID',
client_secret: 'YOUR_CLIENT_SECRET',
code: 'AUTHORIZATION_CODE',
grant_type: 'authorization_code',
redirect_uri: 'YOUR_REDIRECT_URI'
})
})
.then(response => response.json())
.then(data => console.log(data));
You'll receive a JSON response containing the access_token and refresh_token. Store these securely as they are required for API requests.
For more details on OAuth authentication, refer to the Salesloft OAuth documentation.
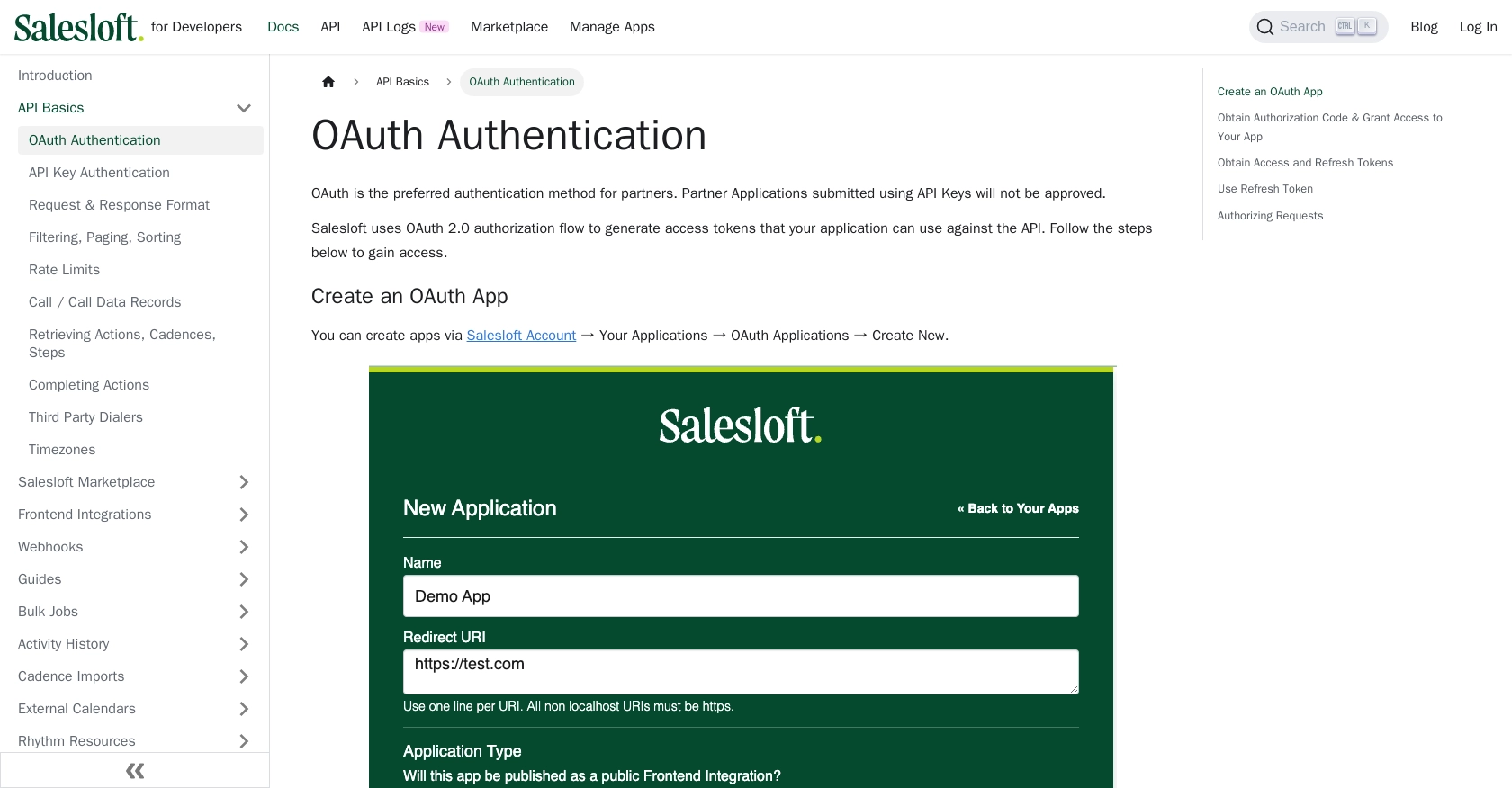
sbb-itb-96038d7
Making API Calls to Retrieve Notes from Salesloft Using JavaScript
To interact with the Salesloft API and retrieve notes, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment and executing the necessary API calls.
JavaScript Environment Setup for Salesloft API
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js.
- Ensure Node.js is installed on your machine. You can download it from the official Node.js website.
- Initialize a new Node.js project and install the
node-fetch
package to make HTTP requests:
npm init -y
npm install node-fetch
Executing the API Call to Get Notes from Salesloft
With your environment ready, you can now make a GET request to the Salesloft API to retrieve notes. Here's how you can do it using JavaScript:
const fetch = require('node-fetch');
// Set the API endpoint and headers
const endpoint = 'https://api.salesloft.com/v2/notes';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Make a GET request to the API
fetch(endpoint, { headers })
.then(response => response.json())
.then(data => {
// Loop through the notes and print their content
data.data.forEach(note => {
console.log(`Note ID: ${note.id}, Content: ${note.content}`);
});
})
.catch(error => console.error('Error fetching notes:', error));
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth process. This code fetches notes from Salesloft and logs their IDs and content to the console.
Verifying API Call Success and Handling Errors
After running the code, you should see the notes printed in your console. To verify the request's success, check the response status code. A status code of 200 indicates success.
If you encounter errors, handle them gracefully by checking the response status and error messages. Common error codes include:
- 403 Forbidden: Check your access token and permissions.
- 404 Not Found: Ensure the endpoint URL is correct.
- 422 Unprocessable Entity: Review the request parameters for any invalid values.
For more details on error handling, refer to the Salesloft Request & Response Format documentation.
Optimizing API Calls with Salesloft's Rate Limits
Salesloft imposes a rate limit of 600 requests per minute. To avoid hitting this limit, consider implementing rate limiting strategies such as:
- Batching requests to reduce the number of API calls.
- Implementing exponential backoff for retrying failed requests.
For more information on rate limits, visit the Salesloft Rate Limits documentation.
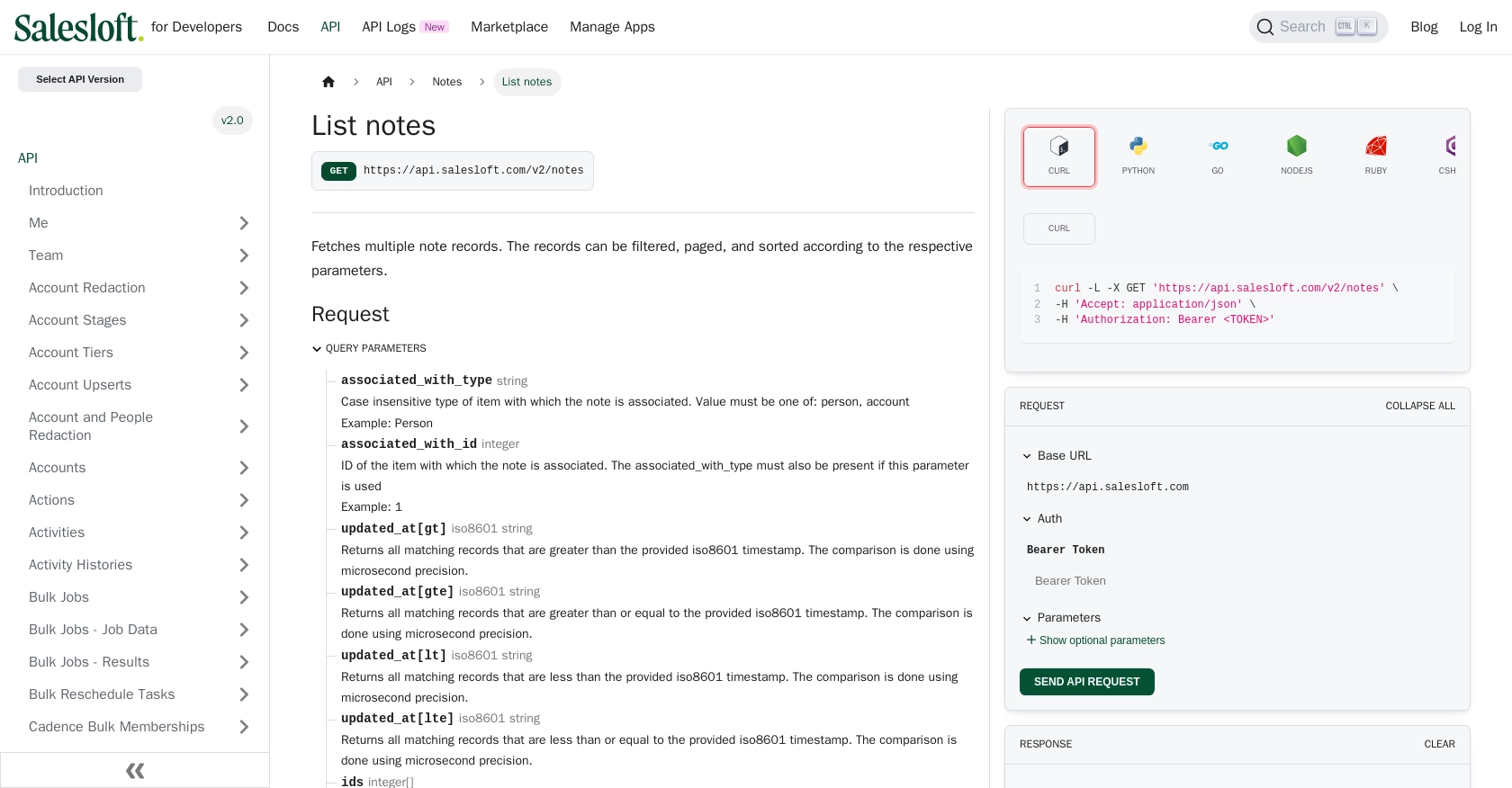
Conclusion and Best Practices for Using Salesloft API with JavaScript
Integrating with the Salesloft API to retrieve notes using JavaScript can significantly enhance your sales data management and analysis capabilities. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth, and make API calls to access valuable sales insights.
Best Practices for Secure and Efficient Salesloft API Integration
- Securely Store Credentials: Always store your access and refresh tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Salesloft's rate limit of 600 requests per minute. Implement strategies like request batching and exponential backoff to manage your API usage effectively.
- Data Standardization: Ensure that the data retrieved from Salesloft is standardized and transformed as needed to fit your application's requirements.
Leverage Endgrate for Simplified Integration Management
While building integrations with the Salesloft API can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Salesloft.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. With Endgrate's intuitive integration experience, you can build once for each use case and easily manage multiple integrations without the hassle of maintaining separate connections.
Explore how Endgrate can simplify your integration process by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/notes-index/
Ready to get started?