Using the Applied Epic API to Get Clients in Javascript
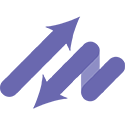
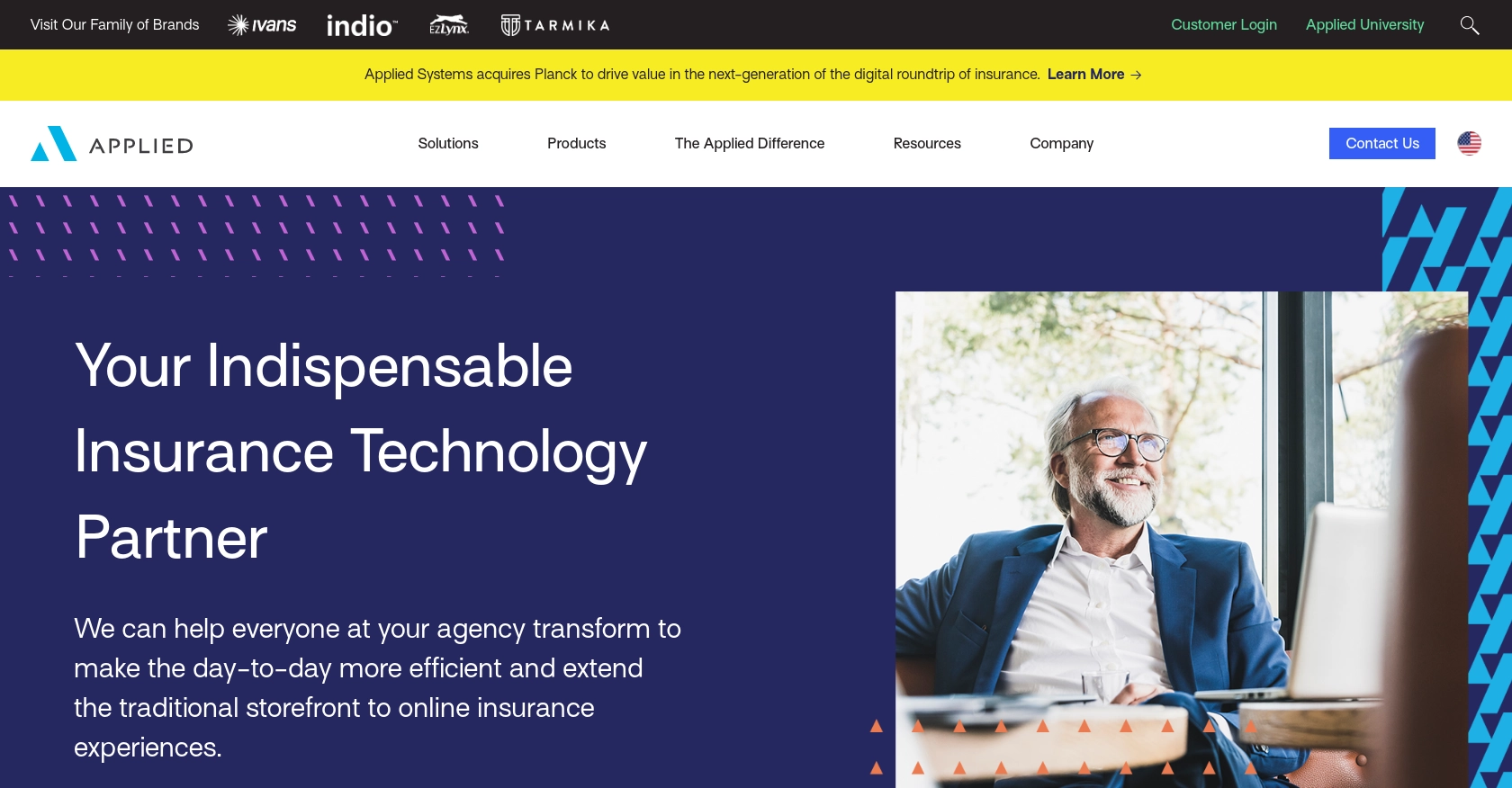
Introduction to Applied Epic API
Applied Epic is a comprehensive insurance management platform that streamlines operations for insurance agencies and brokerages. It offers a suite of tools to manage client relationships, policies, and claims efficiently. By integrating with the Applied Epic API, developers can automate and enhance various processes, such as retrieving client data, which is crucial for maintaining up-to-date records and providing personalized services.
Connecting with the Applied Epic API allows developers to access client information programmatically, enabling seamless integration with other systems. For example, a developer might use the API to fetch client details and synchronize them with a CRM system, ensuring that sales and support teams have the most current information at their fingertips.
Setting Up Your Applied Epic Test Account
Before you can start using the Applied Epic API to retrieve client data, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting real data. Follow these steps to get started:
Sign Up for an Applied Epic Developer Account
To access the Applied Epic API, you'll first need to sign up for a developer account. Visit the Applied Developer Portal and follow the instructions to create your account. This will grant you access to the necessary tools and resources for API integration.
Create an Application for API Access
Once your developer account is set up, you'll need to create an application within the Applied Developer Portal. This application will provide you with the credentials required for API authentication.
- Log in to your developer account.
- Navigate to the "Applications" section and click on "Create New Application."
- Fill in the required details, such as the application name and description.
- Save your application to generate the client ID and client secret.
Configure Custom Authentication for Applied Epic API
The Applied Epic API uses a custom authentication method. You'll need to configure your application to handle this authentication process:
- Use the client ID and client secret obtained from your application to authenticate API requests.
- Ensure that your application is set up to handle OAuth scopes such as
epic/clients:read
andepic/clients:all
.
For more details on authentication, refer to the Applied Epic API Overview.
Testing Your Setup
With your test account and application configured, you can now begin testing API calls. Ensure that your setup is correct by making a simple API request to retrieve client data. This will confirm that your authentication and application settings are properly configured.
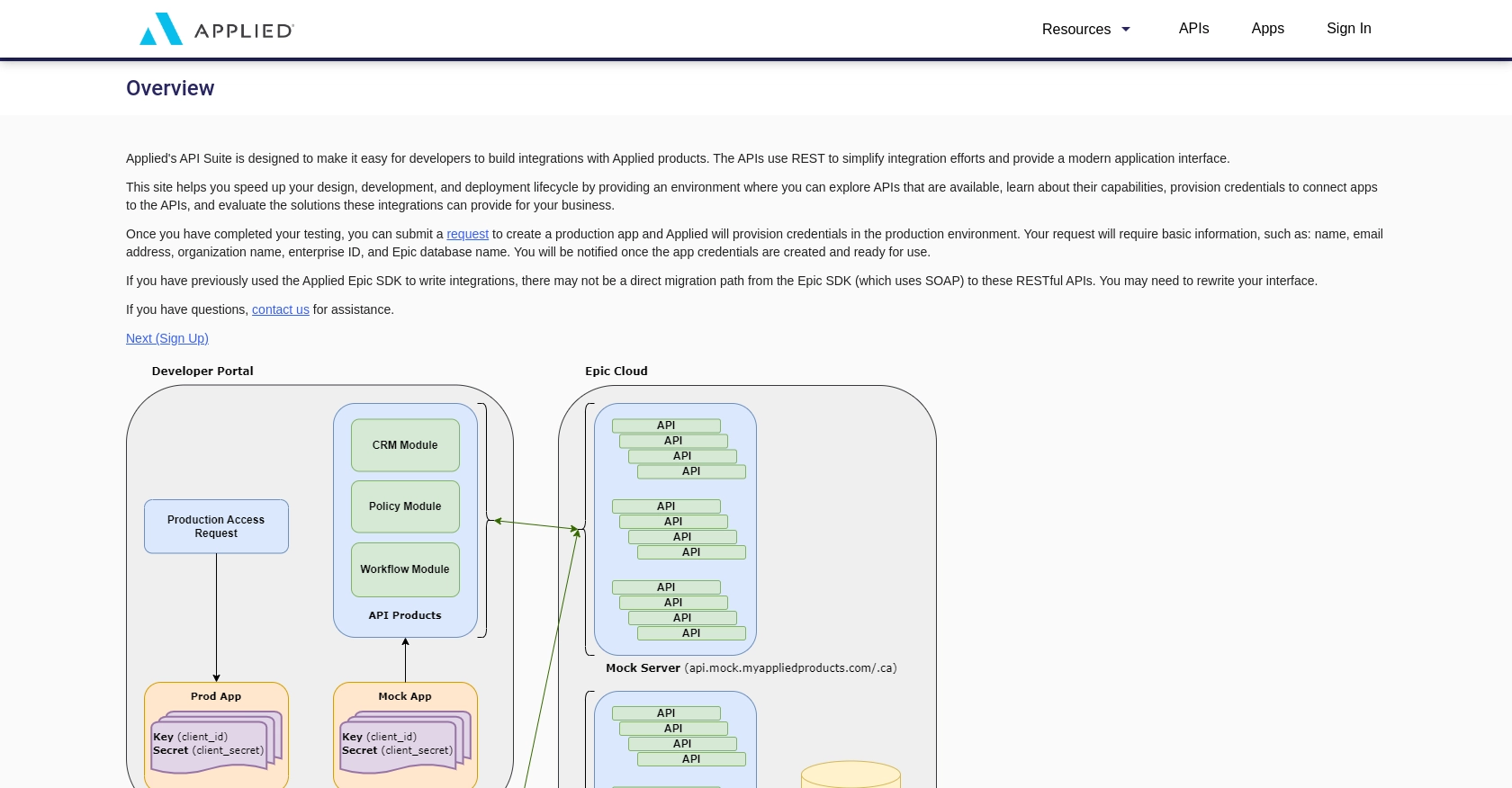
sbb-itb-96038d7
Making API Calls to Retrieve Clients Using Applied Epic API in JavaScript
To interact with the Applied Epic API and retrieve client data, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have a modern JavaScript environment set up. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js. Make sure you have Node.js installed on your machine. You can download it from the official Node.js website.
Next, initialize a new Node.js project and install the necessary dependencies:
mkdir applied-epic-client
cd applied-epic-client
npm init -y
npm install axios
We will use Axios, a popular HTTP client for JavaScript, to make API requests.
Writing the JavaScript Code to Fetch Clients
Create a new file named getClients.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.myappliedproducts.com/crm/v1/clients';
const headers = {
'Authorization': 'Bearer Your_Token',
'Content-Type': 'application/json'
};
// Function to get clients
async function getClients() {
try {
const response = await axios.get(endpoint, { headers });
const clients = response.data._embedded.clients;
console.log('Clients:', clients);
} catch (error) {
console.error('Error fetching clients:', error.response ? error.response.data : error.message);
}
}
getClients();
Replace Your_Token
with the token obtained from your Applied Epic application setup. This code sets up the API endpoint and headers, makes a GET request to the Applied Epic API, and logs the client data to the console.
Running the Code and Verifying the Output
Run the code using the following command:
node getClients.js
If the request is successful, you should see a list of clients printed in the console. This confirms that your API call is working correctly and that you have access to the client data.
Handling Errors and Common Error Codes
When making API calls, it's essential to handle potential errors gracefully. The Applied Epic API may return various error codes, such as:
- 400 Bad Request: The request was malformed or invalid.
- 401 Unauthorized: Authentication failed. Check your token.
- 403 Forbidden: You do not have permission to access the resource.
Ensure your application handles these errors by logging them and providing meaningful feedback to the user.
For more detailed information on error handling, refer to the Applied Epic API Documentation.
Conclusion and Best Practices for Using Applied Epic API in JavaScript
Integrating with the Applied Epic API allows developers to efficiently manage client data, enhancing the capabilities of insurance management systems. By following the steps outlined in this guide, you can successfully retrieve client information using JavaScript, ensuring your applications are both robust and reliable.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your API credentials, such as client ID and client secret, securely. Consider using environment variables or a secure vault service to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your existing systems. This can help maintain data integrity and consistency across platforms.
- Error Handling: Implement comprehensive error handling to manage different error codes effectively. Provide meaningful feedback to users and log errors for further analysis.
Streamlining Integration with Endgrate
While integrating with the Applied Epic API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
By using Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case, reducing the need for multiple integration efforts.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?