Using the Sage 100 API to Create or Update Purchase Orders in Python
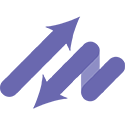
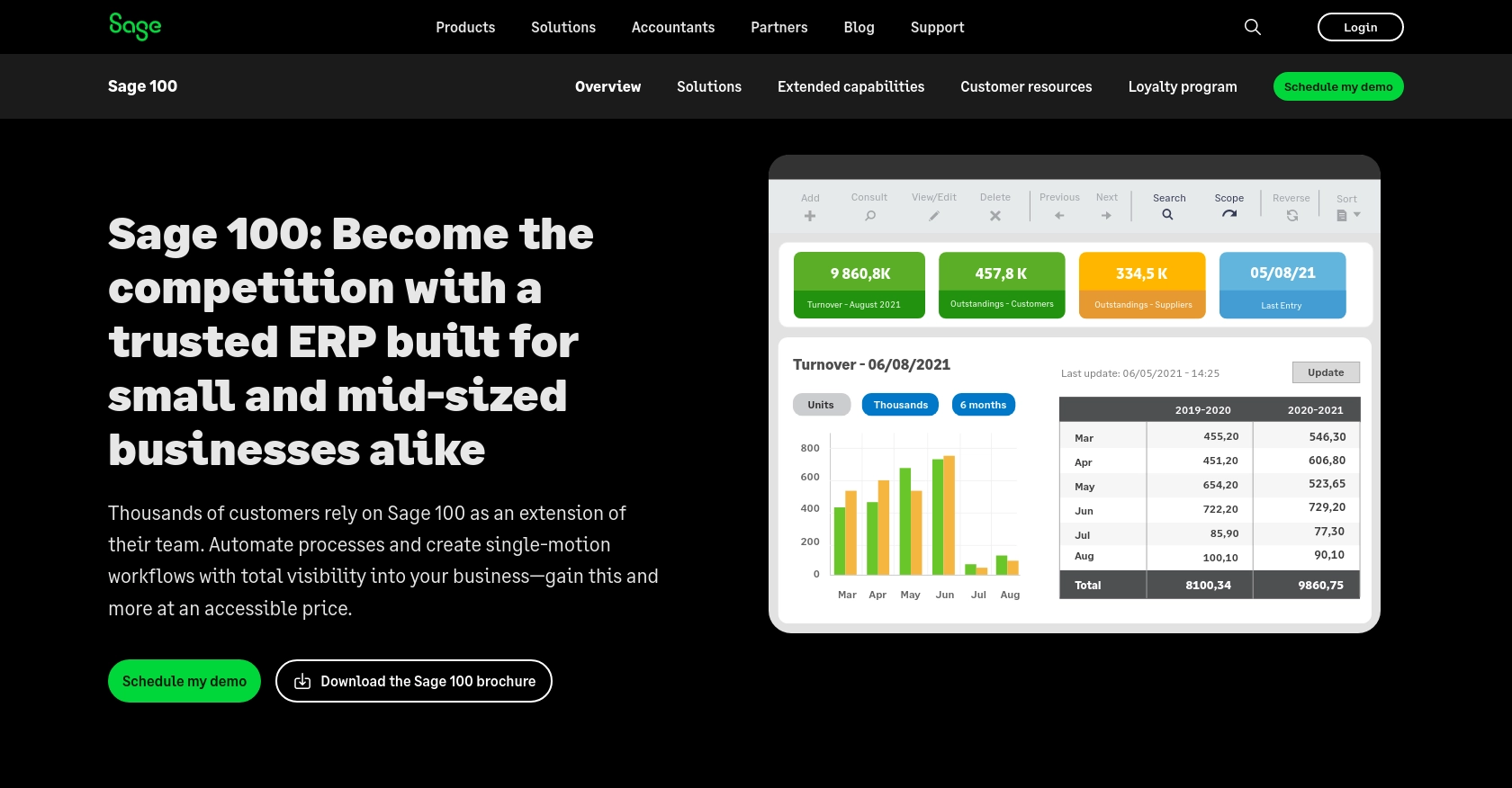
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution designed to streamline business operations, including accounting, inventory management, and purchasing. It is widely used by businesses to enhance efficiency and manage complex processes with ease.
Integrating with the Sage 100 API allows developers to automate and manage purchase orders, a critical component of supply chain management. For example, a developer might use the Sage 100 API to create or update purchase orders directly from a custom application, ensuring seamless data flow and reducing manual entry errors.
This article will guide you through using Python to interact with the Sage 100 API, focusing on creating and updating purchase orders. By following this tutorial, you will learn how to efficiently connect to Sage 100 and perform essential operations on purchase orders.
Setting Up Your Sage 100 Test Environment
Before you can begin integrating with the Sage 100 API, it's essential to set up a test environment. This will allow you to safely experiment with API calls without affecting live data. Sage 100 provides a robust ODBC driver that facilitates connections to its database, enabling developers to perform necessary operations.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, you need to install and configure the Sage 100 ODBC driver. Follow these steps to set up the driver:
- Ensure that the Sage 100 ODBC driver is installed on your system. You can download it from the Sage 100 website or contact your system administrator for assistance.
- Access the ODBC Data Source Administrator on your system. This can typically be found in the Control Panel under Administrative Tools.
- Create a new DSN (Data Source Name) that connects to the Sage 100 ERP system. Provide the correct server, database, and authentication settings as required.
For detailed instructions on configuring the ODBC driver, refer to the official Sage 100 documentation.
Creating a Sage 100 Test Account
If you don't already have a Sage 100 account, you may need to set up a test account to access the API. Contact your Sage 100 representative to inquire about obtaining a test or sandbox account. This will allow you to perform API operations without impacting your production environment.
Configuring Authentication for Sage 100 API Access
Sage 100 uses a custom authentication method via the ODBC driver. Follow these steps to configure authentication:
- Open the Sage 100 Advanced server and navigate to the Library Master System Configuration task.
- In the System Configuration window, select the ODBC Driver tab and enable the C/S ODBC Driver.
- Enter the server name or IP address where the ODBC application or service is running.
- Test the ODBC data source to ensure the connection is successful.
For more detailed guidance, consult the Sage 100 ODBC configuration guide.
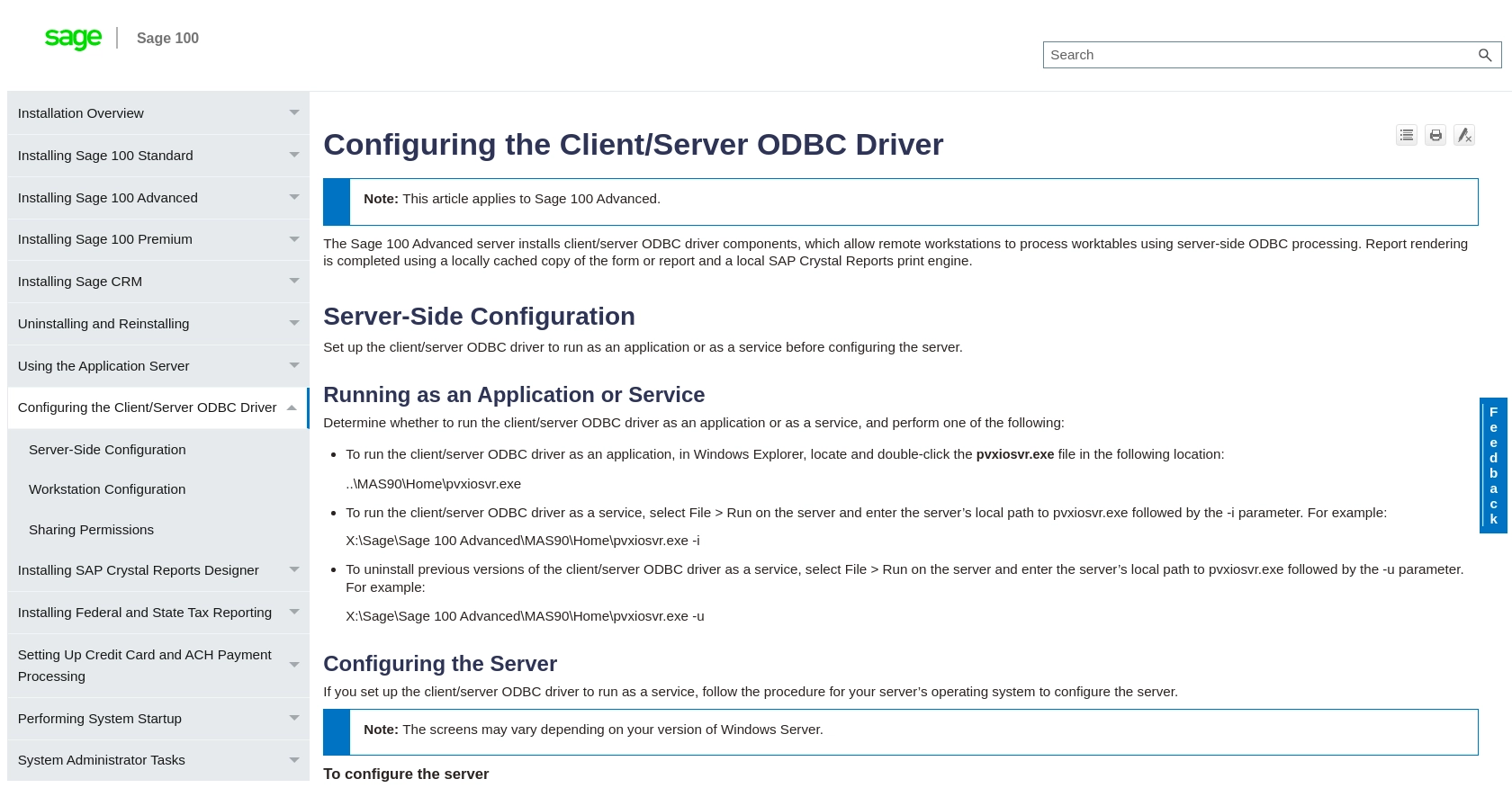
sbb-itb-96038d7
Making API Calls to Sage 100 for Purchase Orders Using Python
To interact with the Sage 100 API for creating or updating purchase orders, you'll need to use Python along with the ODBC driver. This section will guide you through setting up your Python environment and executing API calls to manage purchase orders efficiently.
Setting Up Python Environment for Sage 100 API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- ODBC Driver for Sage 100
- Python package
pyodbc
for ODBC connections
Install the pyodbc
package using pip:
pip install pyodbc
Creating or Updating Purchase Orders with Sage 100 API
Once your environment is set up, you can proceed to create or update purchase orders. Below is an example code snippet to demonstrate how to connect to Sage 100 and perform these operations:
import pyodbc
# Define the DSN and connection parameters
dsn = 'Your_DSN_Name'
user = 'Your_Username'
password = 'Your_Password'
# Establish a connection to the Sage 100 database
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
# Create a cursor object to execute SQL queries
cursor = connection.cursor()
# Example SQL query to create a new purchase order
sql_create_po = """
INSERT INTO PO_PurchaseOrderHeader (PurchaseOrderNo, VendorNo, OrderDate)
VALUES (?, ?, ?)
"""
# Execute the query with sample data
cursor.execute(sql_create_po, ('PO12345', 'VENDOR001', '2023-10-01'))
# Commit the transaction
connection.commit()
# Example SQL query to update an existing purchase order
sql_update_po = """
UPDATE PO_PurchaseOrderHeader
SET OrderDate = ?
WHERE PurchaseOrderNo = ?
"""
# Execute the query with updated data
cursor.execute(sql_update_po, ('2023-10-15', 'PO12345'))
# Commit the transaction
connection.commit()
# Close the connection
cursor.close()
connection.close()
Replace Your_DSN_Name
, Your_Username
, and Your_Password
with your actual Sage 100 credentials. The above code demonstrates how to insert a new purchase order and update an existing one using SQL queries.
Verifying API Call Success in Sage 100
After executing the API calls, verify the success of your operations by checking the Sage 100 test environment. You should see the newly created or updated purchase orders reflected in the system.
Handling Errors and Exceptions in Sage 100 API Calls
It's crucial to handle potential errors during API interactions. Use try-except blocks to manage exceptions such as connection errors or SQL execution issues:
try:
# Your database operations here
except pyodbc.Error as e:
print("An error occurred:", e)
finally:
# Ensure the connection is closed
if connection:
connection.close()
By implementing error handling, you can ensure robust and reliable integration with the Sage 100 API.
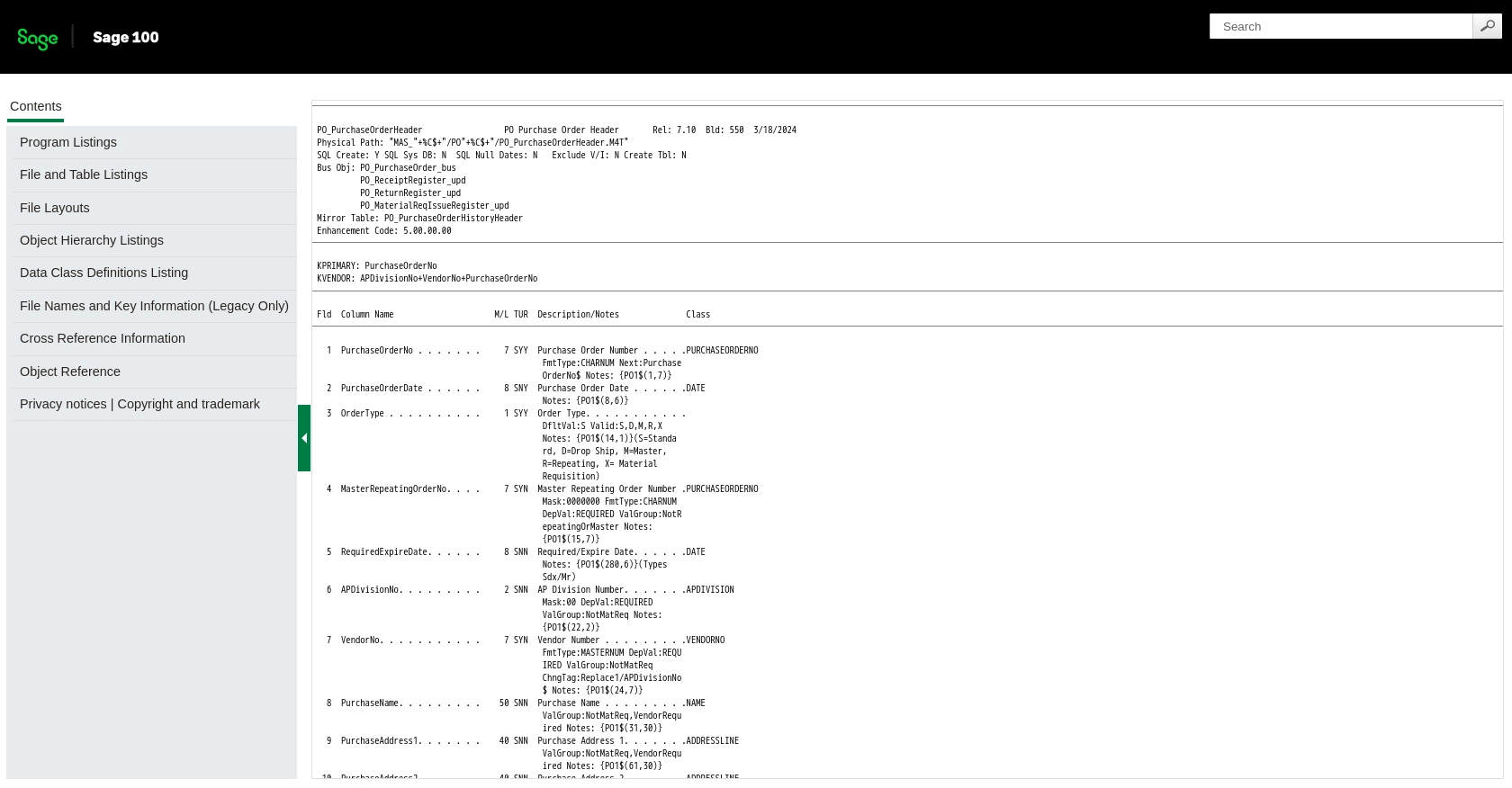
Conclusion and Best Practices for Sage 100 API Integration
Integrating with the Sage 100 API using Python provides a powerful way to automate and manage purchase orders, enhancing efficiency and reducing manual errors. By following the steps outlined in this guide, you can seamlessly connect to Sage 100 and perform essential operations on purchase orders.
Best Practices for Secure and Efficient Sage 100 API Usage
- Secure Credential Storage: Always store user credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure consistent data formats when interacting with the API to maintain data integrity across systems.
- Error Handling: Implement robust error handling to manage exceptions and ensure reliable API interactions.
Streamline Your Integrations with Endgrate
While integrating with Sage 100 can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sage 100. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover the benefits of a streamlined, intuitive integration solution.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Purchase_Order/PO_PurchaseOrderHeader.htm?Highlight=PO_PurchaseOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Purchase_Order/PO_PurchaseOrderDetail.htm?Highlight=PO_PurchaseOrderDetail
Ready to get started?