Using the Xero API to Create or Update Purchase Orders (with PHP examples)
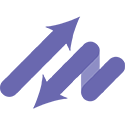
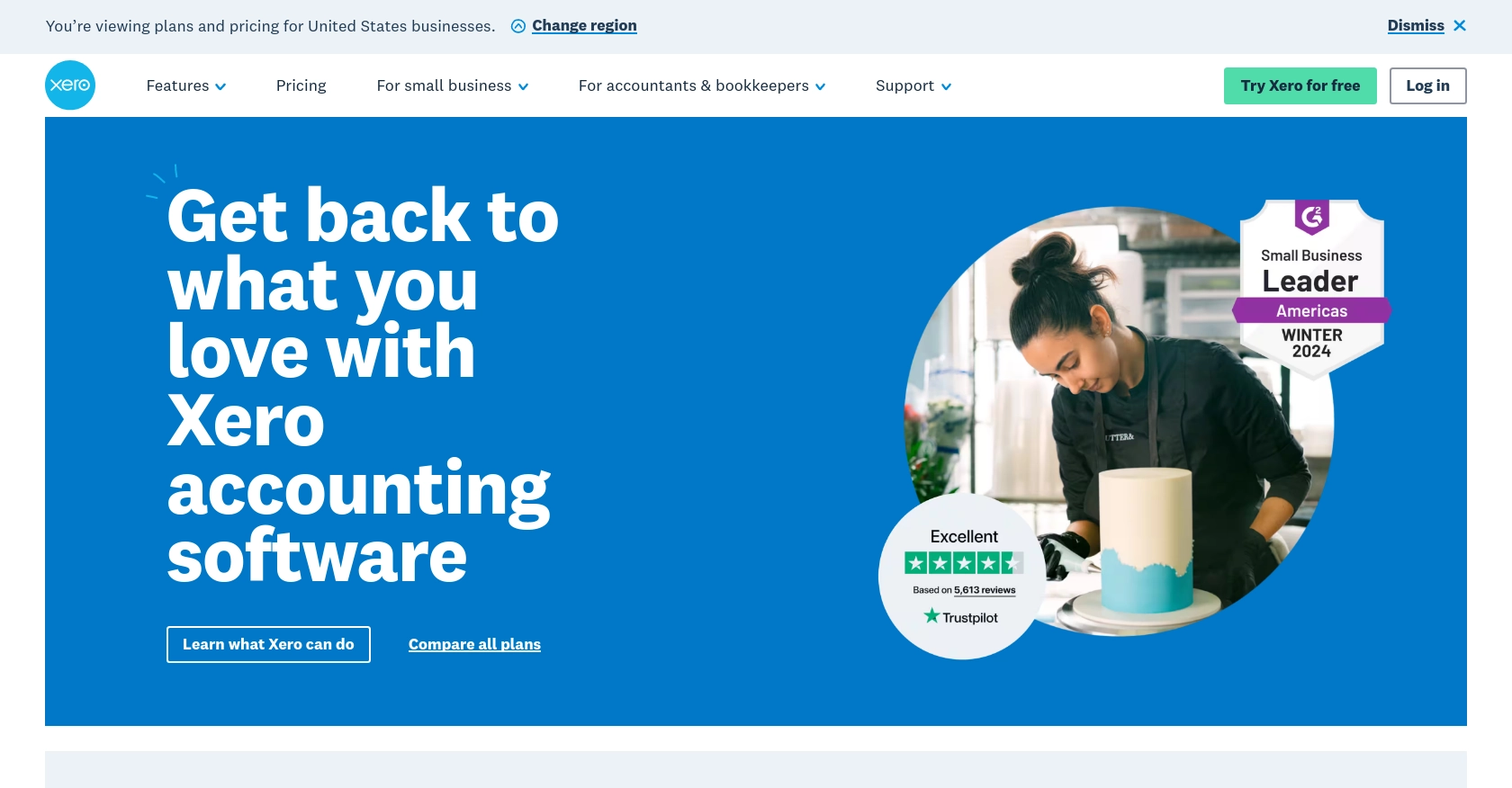
Introduction to Xero API for Purchase Orders
Xero is a powerful cloud-based accounting software platform designed to simplify financial management for small and medium-sized businesses. With its robust set of features, Xero allows businesses to manage invoices, bank reconciliation, inventory, and more, all from a single, user-friendly interface.
For developers, integrating with Xero's API opens up a world of possibilities for automating accounting tasks and enhancing business workflows. One such task is the creation and updating of purchase orders, which can streamline procurement processes and improve financial accuracy.
Imagine a scenario where a developer needs to automate the purchase order process for a business. By leveraging the Xero API, they can programmatically create or update purchase orders, ensuring that inventory levels are maintained and financial records are up-to-date without manual intervention.
This article will guide you through using the Xero API to create or update purchase orders using PHP, providing you with the tools and knowledge to integrate this functionality into your own applications.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start creating or updating purchase orders using the Xero API, you'll need to set up a sandbox account. This allows you to test your integration in a safe environment without affecting live data.
Creating a Xero Sandbox Account
To begin, sign up for a Xero Developer account if you haven't already. This will give you access to the Xero Developer Portal, where you can manage your applications and access sandbox environments.
- Visit the Xero Developer Portal and sign up for an account.
- Once registered, log in and navigate to the "My Apps" section.
- Create a new app by clicking on "New App" and filling in the required details, such as the app name and company URL.
Configuring OAuth 2.0 Authentication
The Xero API uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to configure OAuth 2.0:
- In your app settings, note down the Client ID and Client Secret. These will be used to authenticate your API requests.
- Set the redirect URI to a valid endpoint in your application where Xero will send the authorization code.
- Define the necessary scopes for your app. For purchase orders, ensure you include the appropriate scopes to access and modify purchase orders.
For more details on OAuth 2.0, refer to the Xero OAuth 2.0 documentation.
Generating Access Tokens
With your app configured, you can now generate access tokens to authenticate API requests:
- Direct users to the Xero authorization URL, including your Client ID and requested scopes.
- Once users authorize the app, Xero will redirect them to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to the Xero token endpoint.
Ensure you securely store the access token and refresh token, as they are required for making authenticated API calls.
Connecting to a Xero Tenant
After obtaining an access token, connect to a Xero tenant to access its data:
- Use the access token to make a GET request to the Xero connections endpoint.
- Retrieve the list of tenants associated with the token and select the appropriate tenant ID for your operations.
For more information on managing tenants, visit the Xero Tenants documentation.
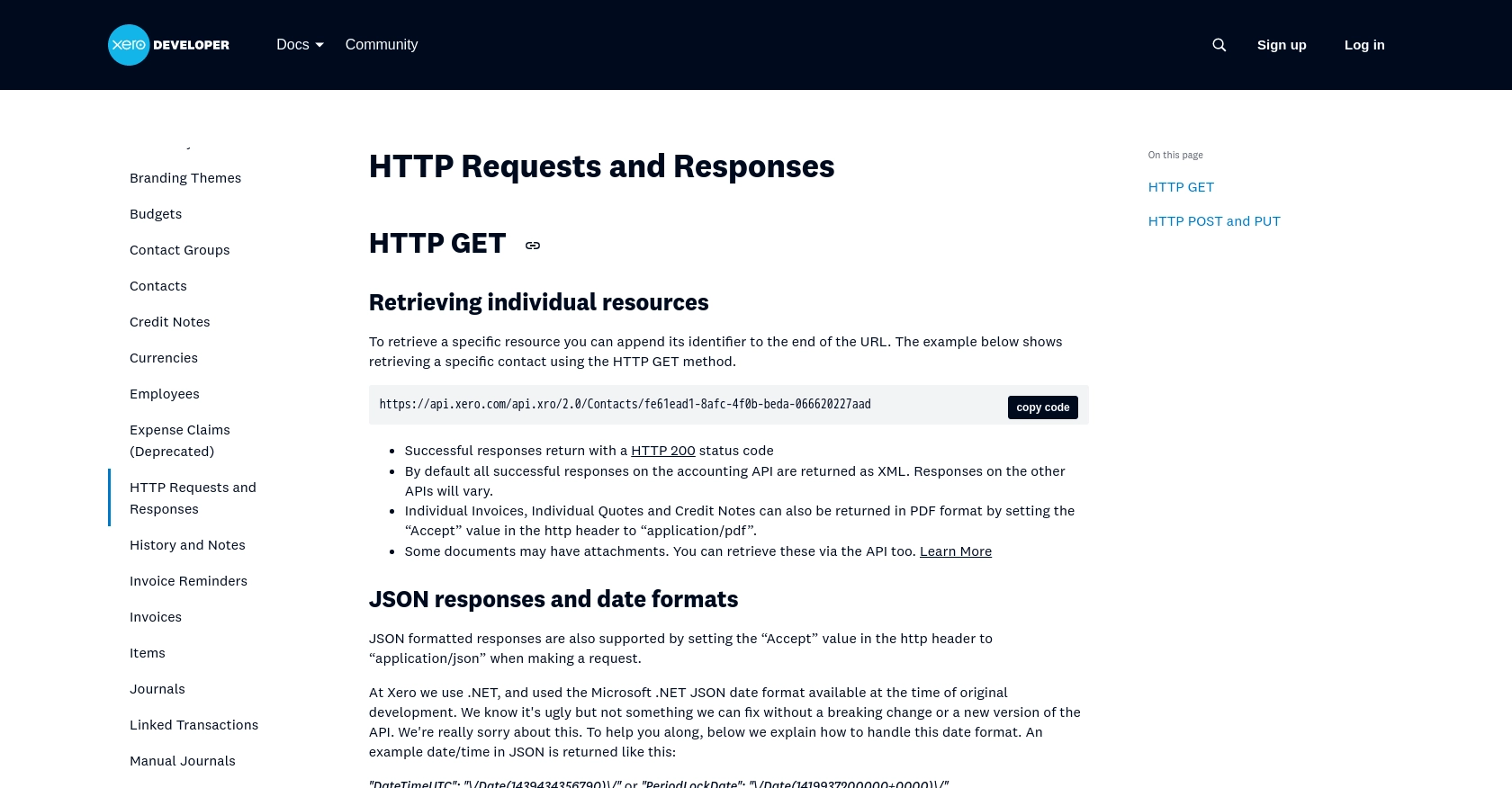
sbb-itb-96038d7
Making API Calls to Xero for Purchase Orders Using PHP
With your Xero sandbox account and OAuth 2.0 authentication set up, you can now proceed to make API calls to create or update purchase orders. This section will guide you through the process using PHP, ensuring you have the necessary tools and knowledge to interact with the Xero API effectively.
Prerequisites for PHP Integration with Xero API
Before diving into the code, ensure you have the following prerequisites installed on your development environment:
- PHP 7.4 or higher
- Composer for dependency management
- The Guzzle HTTP client for making HTTP requests
To install Guzzle, run the following command in your terminal:
composer require guzzlehttp/guzzle
Creating a Purchase Order with Xero API Using PHP
To create a purchase order, you'll need to make a POST request to the Xero API endpoint for purchase orders. Here's a step-by-step guide:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$tenantId = 'Your_Tenant_ID';
$url = 'https://api.xero.com/api.xro/2.0/PurchaseOrders';
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Xero-tenant-id' => $tenantId,
'Content-Type' => 'application/json',
'Accept' => 'application/json'
];
$purchaseOrderData = [
'Type' => 'ACCPAY',
'Contact' => [
'ContactID' => 'Your_Contact_ID'
],
'LineItems' => [
[
'Description' => 'Sample Item',
'Quantity' => 1,
'UnitAmount' => 100.00,
'AccountCode' => '400'
]
],
'Date' => '2023-10-01',
'DeliveryDate' => '2023-10-05',
'Status' => 'DRAFT'
];
$response = $client->post($url, [
'headers' => $headers,
'json' => $purchaseOrderData
]);
echo $response->getBody();
Replace Your_Access_Token
, Your_Tenant_ID
, and Your_Contact_ID
with your actual access token, tenant ID, and contact ID. This code sends a POST request to create a purchase order with specified details.
Updating a Purchase Order with Xero API Using PHP
To update an existing purchase order, make a POST request to the same endpoint with the updated data:
$purchaseOrderId = 'Your_Purchase_Order_ID';
$updateData = [
'Status' => 'AUTHORISED'
];
$response = $client->post($url . '/' . $purchaseOrderId, [
'headers' => $headers,
'json' => $updateData
]);
echo $response->getBody();
Replace Your_Purchase_Order_ID
with the ID of the purchase order you wish to update. This example changes the status to "AUTHORISED".
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Check the response status code to verify success:
- A status code of
200
indicates success. - Handle errors by checking for status codes like
400
(Bad Request) or401
(Unauthorized).
For more details on error codes, refer to the Xero API documentation.
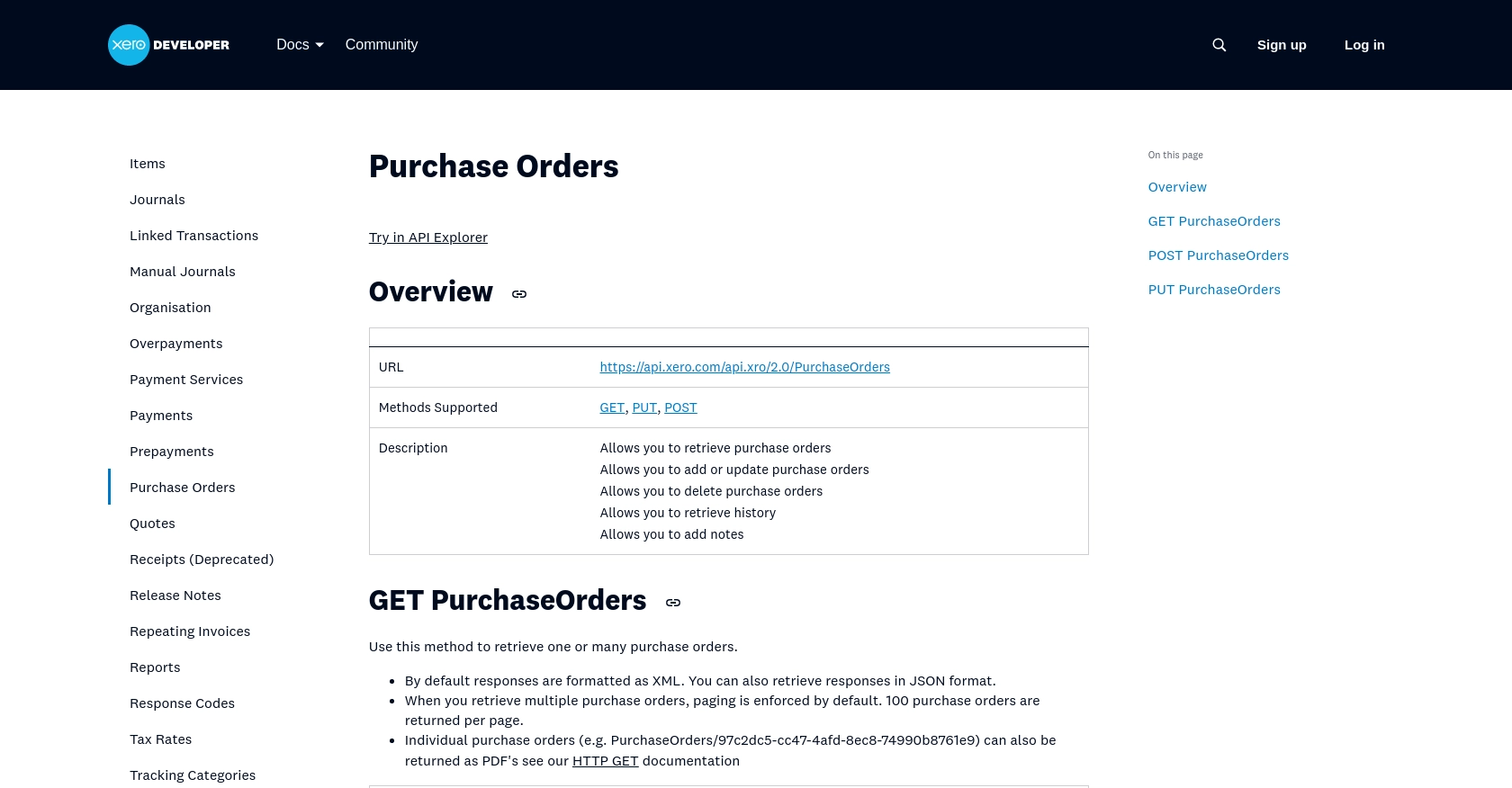
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API to create or update purchase orders using PHP can significantly enhance your business's financial management processes. By automating these tasks, you can ensure accuracy and efficiency in handling procurement and inventory management.
Here are some best practices to consider when working with the Xero API:
- Securely Store Credentials: Always store your Client ID, Client Secret, access tokens, and refresh tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be sure to implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the Xero OAuth 2.0 API limits.
- Standardize Data Fields: Ensure that the data you send to Xero is standardized and validated to prevent errors and maintain data integrity.
- Monitor API Usage: Regularly monitor your API usage and logs to identify any issues or anomalies in your integration.
By following these best practices, you can build a robust integration with Xero that enhances your business operations.
Streamline Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations and focusing on your core product. Build once for each use case instead of multiple times for different integrations, and provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/purchaseorders
Ready to get started?