How to Create or Update Invoices with the Zoho Books API in Python
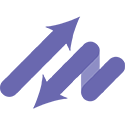
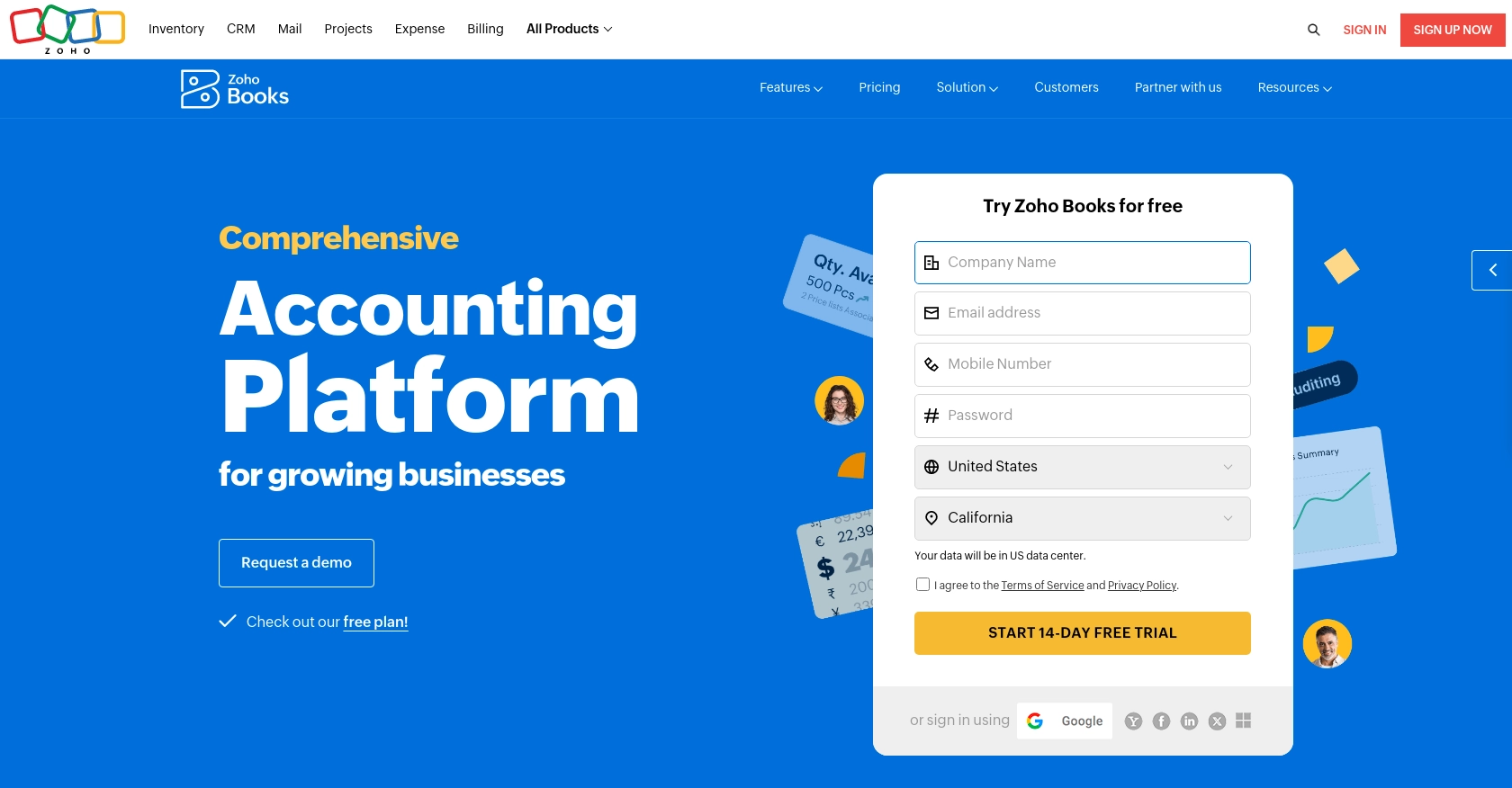
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive online accounting software designed to streamline financial management for businesses. It offers a wide range of features including invoicing, expense tracking, and financial reporting, making it an essential tool for businesses looking to manage their finances efficiently.
Integrating with the Zoho Books API allows developers to automate and enhance financial processes. By connecting with Zoho Books, developers can create or update invoices programmatically, ensuring seamless financial operations. For example, a developer might automate the creation of invoices based on sales data from an e-commerce platform, reducing manual entry and minimizing errors.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting real data. Zoho Books offers a free trial that you can use for this purpose.
Creating a Zoho Books Account
- Visit the Zoho Books website and sign up for a free trial account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the Zoho Books dashboard.
Setting Up OAuth for Zoho Books API
Zoho Books uses OAuth 2.0 for authentication, ensuring secure access to your data. Follow these steps to set up OAuth:
- Go to the Zoho Developer Console.
- Click on Add Client ID to register your application.
- Fill in the required details and submit the form to receive your Client ID and Client Secret.
Generating OAuth Tokens
With your Client ID and Client Secret, you can generate OAuth tokens:
- Redirect users to the following URL to obtain a grant token:
- After user consent, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.invoices.CREATE,ZohoBooks.invoices.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
import requests
url = "https://accounts.zoho.com/oauth/v2/token"
payload = {
"code": "YOUR_CODE",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"redirect_uri": "YOUR_REDIRECT_URI",
"grant_type": "authorization_code"
}
response = requests.post(url, data=payload)
print(response.json())
For more details on OAuth setup, refer to the Zoho Books OAuth documentation.
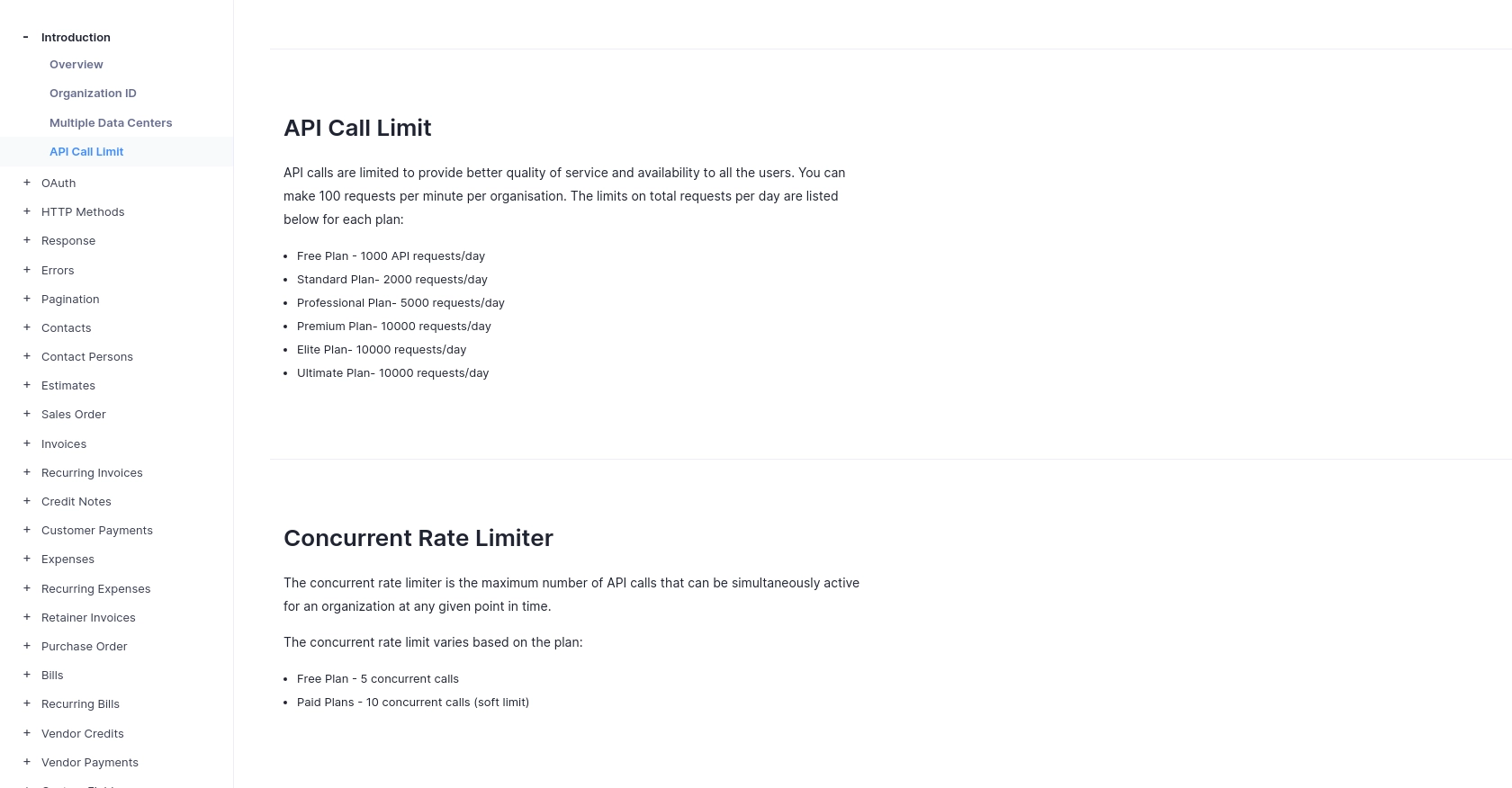
sbb-itb-96038d7
Making API Calls to Create or Update Invoices with Zoho Books Using Python
Setting Up Your Python Environment for Zoho Books API Integration
To interact with the Zoho Books API using Python, ensure you have Python 3.11.1 installed. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Creating an Invoice with Zoho Books API in Python
To create an invoice, you'll need to make a POST request to the Zoho Books API. Here's a step-by-step guide:
- Define the API endpoint and headers. The endpoint for creating an invoice is
/books/v3/invoices
. - Prepare the request payload with necessary invoice details such as
customer_id
,line_items
, and other relevant fields. - Send the POST request and handle the response.
import requests
# Define the API endpoint and headers
url = "https://www.zohoapis.com/books/v3/invoices?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the invoice data
invoice_data = {
"customer_id": "982000000567001",
"line_items": [
{
"item_id": "982000000030049",
"quantity": 1,
"rate": 120
}
],
"date": "2023-10-01",
"due_date": "2023-10-15"
}
# Make the POST request
response = requests.post(url, headers=headers, json=invoice_data)
# Print the response
print(response.json())
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token.
Updating an Invoice with Zoho Books API in Python
To update an existing invoice, use the PUT method. Here's how you can do it:
- Specify the invoice ID you want to update in the endpoint URL.
- Prepare the payload with updated invoice details.
- Send the PUT request and handle the response.
import requests
# Define the API endpoint and headers
invoice_id = "982000000567114"
url = f"https://www.zohoapis.com/books/v3/invoices/{invoice_id}?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the updated invoice data
updated_data = {
"line_items": [
{
"item_id": "982000000030049",
"quantity": 2,
"rate": 120
}
]
}
# Make the PUT request
response = requests.put(url, headers=headers, json=updated_data)
# Print the response
print(response.json())
Ensure to replace invoice_id
, YOUR_ORG_ID
, and YOUR_ACCESS_TOKEN
with the correct values.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Zoho Books API uses HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: The invoice was successfully created.
- 400 Bad Request: The request was invalid. Check your payload.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 429 Rate Limit Exceeded: Too many requests. Respect the rate limit of 100 requests per minute.
For more details on error handling, refer to the Zoho Books Error Documentation.
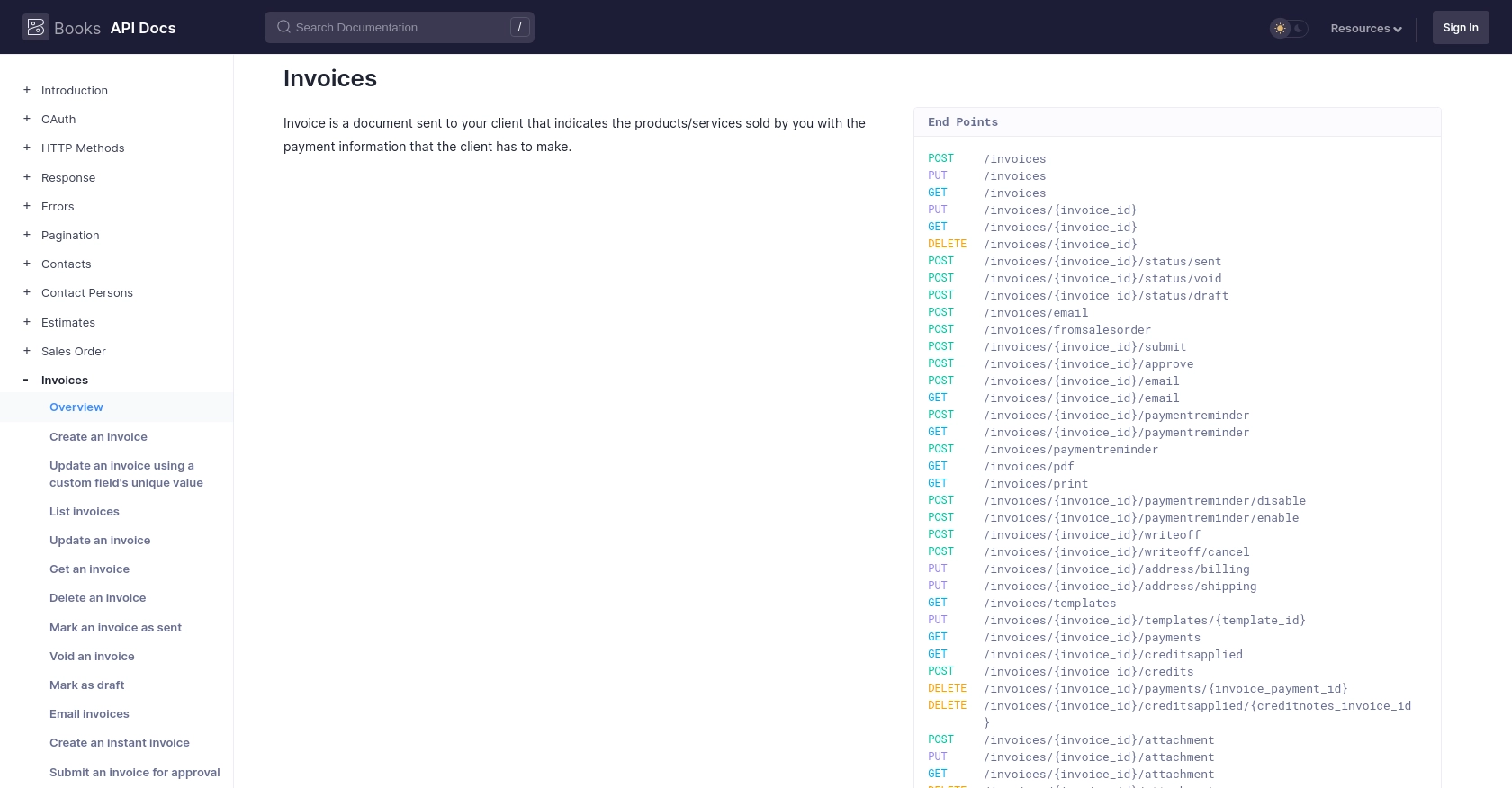
Best Practices for Zoho Books API Integration
When integrating with the Zoho Books API, it's essential to follow best practices to ensure security, efficiency, and reliability. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Zoho Books API allows up to 100 requests per minute. Implement logic to handle rate limits gracefully, such as retry mechanisms or request throttling.
- Standardize Data Fields: Ensure that data fields are consistent and standardized across your application to avoid discrepancies and errors during API interactions.
Leveraging Endgrate for Seamless Zoho Books Integrations
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Zoho Books. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and loyalty.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/invoices/#overview
Ready to get started?