Using the Xero API to Create or Update Invoices in PHP
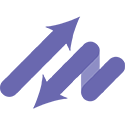
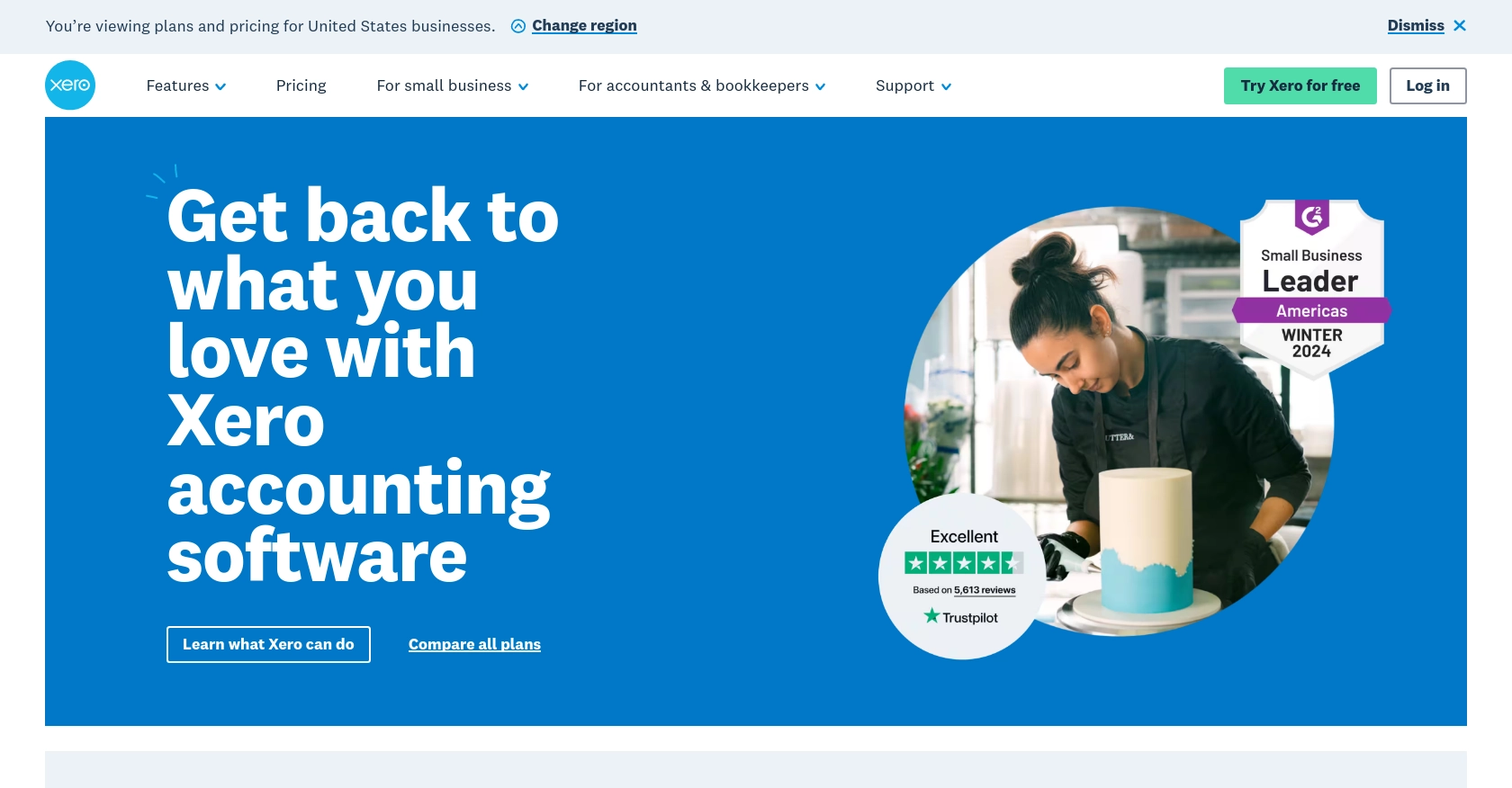
Introduction to Xero Accounting Software
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, bank reconciliation, and financial reporting, Xero provides a comprehensive solution for businesses looking to streamline their accounting processes.
Integrating with Xero's API allows developers to automate financial tasks, such as creating or updating invoices, directly from their applications. For example, a developer might use the Xero API to automatically generate invoices for completed sales transactions, ensuring that financial records are always up-to-date and accurate.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start integrating with the Xero API to create or update invoices, you need to set up a Xero sandbox account. This allows you to test your application in a controlled environment without affecting live data.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. If you don't have one, follow these steps:
- Visit the Xero Developer Portal.
- Click on "Sign Up" and fill in the required details to create your developer account.
- Once registered, log in to your developer account.
Setting Up a Xero Sandbox Organization
After creating your developer account, you need to set up a sandbox organization:
- Navigate to the "My Apps" section in the Xero Developer Portal.
- Click on "Create App" and provide the necessary information, such as the app name and company URL.
- Select "Web App" as the app type and click "Create App."
- Once the app is created, you'll be directed to the app's dashboard. Here, you can find your client ID and client secret, which are essential for OAuth authentication.
Configuring OAuth 2.0 Authentication
Xero uses OAuth 2.0 for authentication. Follow these steps to configure it:
- In your app's dashboard, navigate to the "OAuth 2.0" section.
- Set up your redirect URI, which is the URL where users will be redirected after authentication.
- Note down the client ID and client secret, as you'll need them to authenticate API requests.
For more detailed information on OAuth 2.0, refer to the Xero OAuth 2.0 Overview.
Obtaining Access and Refresh Tokens
To interact with the Xero API, you'll need to obtain access and refresh tokens:
- Use the client ID and client secret to request an authorization code.
- Exchange the authorization code for access and refresh tokens.
- These tokens will allow your application to make authorized API requests.
For a step-by-step guide, visit the Xero OAuth 2.0 Authorization Flow.
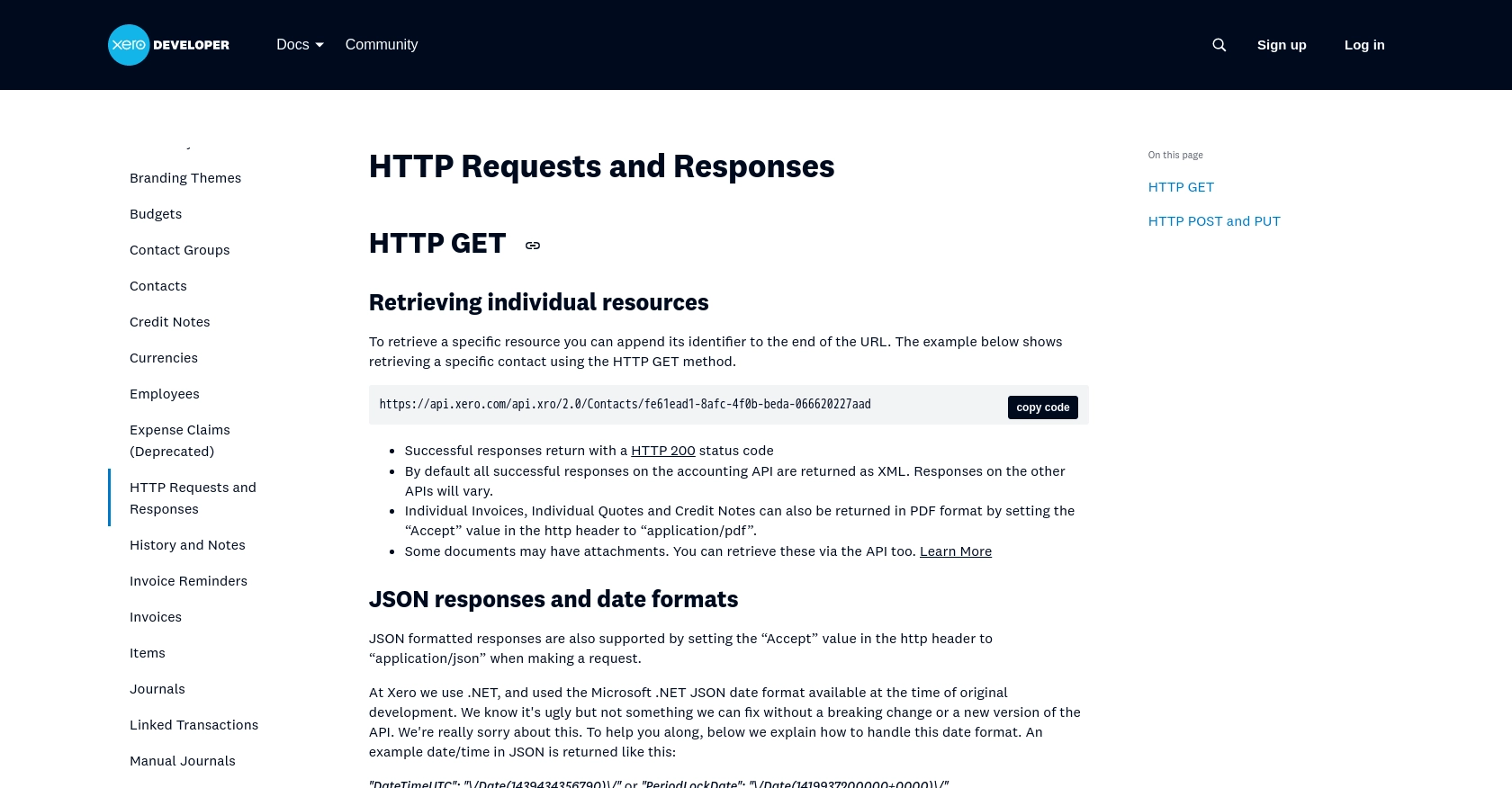
sbb-itb-96038d7
Making API Calls to Xero for Invoice Management Using PHP
To create or update invoices using the Xero API in PHP, you'll need to set up your development environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up PHP, installing dependencies, and writing code to make API requests.
Setting Up Your PHP Environment for Xero API Integration
Before making API calls, ensure you have the following prerequisites installed:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, use Composer to install the Guzzle HTTP client, which will help you make HTTP requests to the Xero API:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Invoices in Xero
With your environment set up, you can now write the PHP code to interact with the Xero API. Below is an example of how to create or update an invoice:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$tenantId = 'Your_Tenant_Id';
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Xero-tenant-id' => $tenantId,
'Content-Type' => 'application/json',
'Accept' => 'application/json',
];
$invoiceData = [
'Type' => 'ACCREC',
'Contact' => [
'ContactID' => 'Contact_ID'
],
'LineItems' => [
[
'Description' => 'Consulting services',
'Quantity' => 10,
'UnitAmount' => 100,
'AccountCode' => '200'
]
],
'Date' => '2023-10-01',
'DueDate' => '2023-10-15',
'InvoiceNumber' => 'INV-001'
];
$response = $client->post('https://api.xero.com/api.xro/2.0/Invoices', [
'headers' => $headers,
'json' => $invoiceData
]);
if ($response->getStatusCode() == 200) {
echo "Invoice created or updated successfully.";
} else {
echo "Failed to create or update invoice.";
}
Replace Your_Access_Token
and Your_Tenant_Id
with your actual access token and tenant ID. The ContactID
should be replaced with the ID of the contact you are invoicing.
Verifying API Request Success in Xero Sandbox
After running your PHP script, verify the success of the API request by checking the Xero sandbox account. Navigate to the invoices section to see if the invoice has been created or updated as expected.
Handling Errors and Common Error Codes in Xero API
When making API calls, it's crucial to handle potential errors. The Xero API may return various HTTP status codes indicating the result of your request:
- 200 OK: The request was successful.
- 400 Bad Request: The request was malformed or contains invalid data.
- 401 Unauthorized: Authentication failed. Check your access token.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The requested resource does not exist.
For more details on error handling, refer to the Xero API documentation.
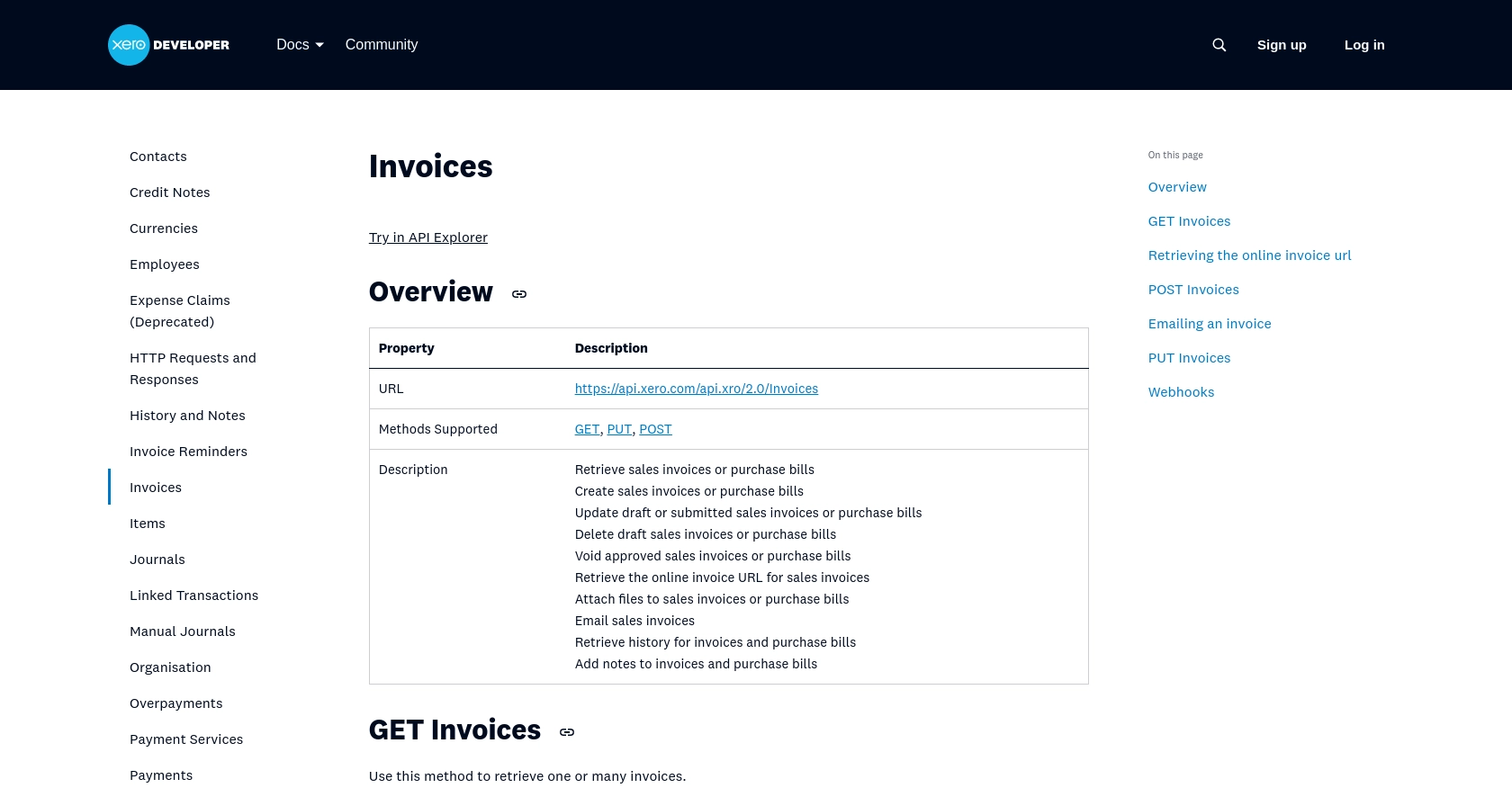
Conclusion and Best Practices for Xero API Integration in PHP
Integrating with the Xero API to create or update invoices in PHP can significantly streamline financial processes for businesses. By automating these tasks, developers can ensure that financial records are accurate and up-to-date, reducing manual effort and minimizing errors.
Best Practices for Secure and Efficient Xero API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be sure to implement logic to handle rate limit errors gracefully. For more details, refer to the Xero OAuth 2.0 API limits.
- Data Standardization: Ensure that the data you send to Xero is standardized and validated to prevent errors and maintain consistency across your applications.
- Regularly Refresh Tokens: Implement a mechanism to refresh your access tokens regularly to maintain uninterrupted API access.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Xero. By using Endgrate, you can save time and resources, allowing you to focus on your core product development. With Endgrate, you build once for each use case, providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/invoices
Ready to get started?