How to Get Users with the PipelineCRM API in PHP
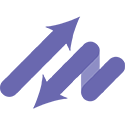
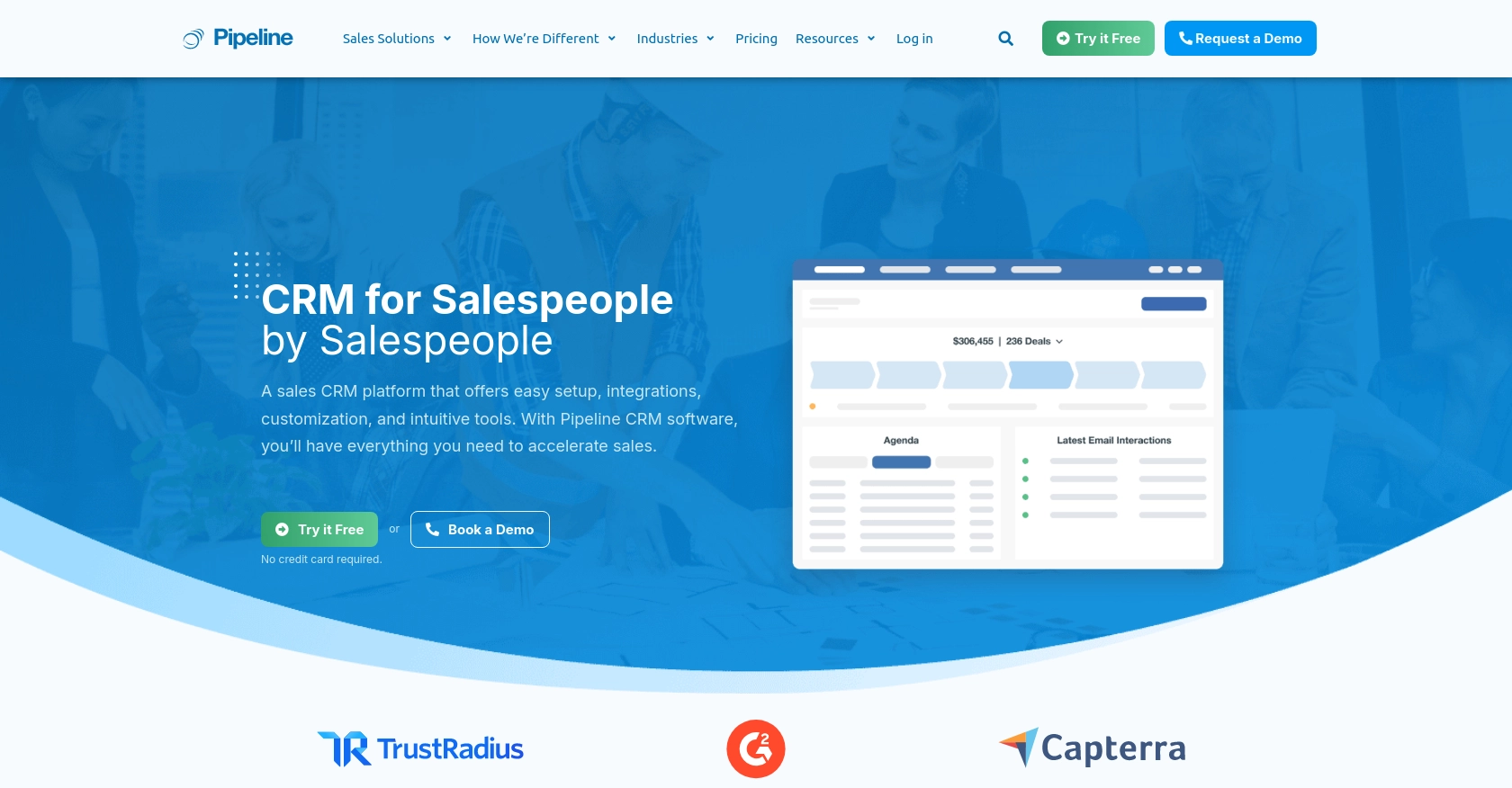
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to enhance sales processes and improve customer interactions for businesses. It offers a suite of tools that help sales teams manage leads, track deals, and analyze performance, all within an intuitive interface.
Developers may want to integrate with PipelineCRM's API to automate and streamline sales operations. For example, by using the PipelineCRM API, a developer can retrieve user data to synchronize with other business systems, ensuring that sales teams have up-to-date information across platforms.
Setting Up Your PipelineCRM Test Account
Before you can start interacting with the PipelineCRM API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on the PipelineCRM website. Follow the instructions to create your account and gain access to the platform's features.
Generating Your API Key for PipelineCRM
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account.
- Navigate to the API settings section, which can typically be found under the account or settings menu.
- Locate the option to generate a new API key. Click on it and follow the prompts to create your key.
- Once generated, copy the API key and store it securely. You will need this key to authenticate your API requests.
With your API key ready, you can now proceed to make API calls to retrieve user data from PipelineCRM. Ensure that you keep your API key confidential and do not expose it in public repositories or client-side code.
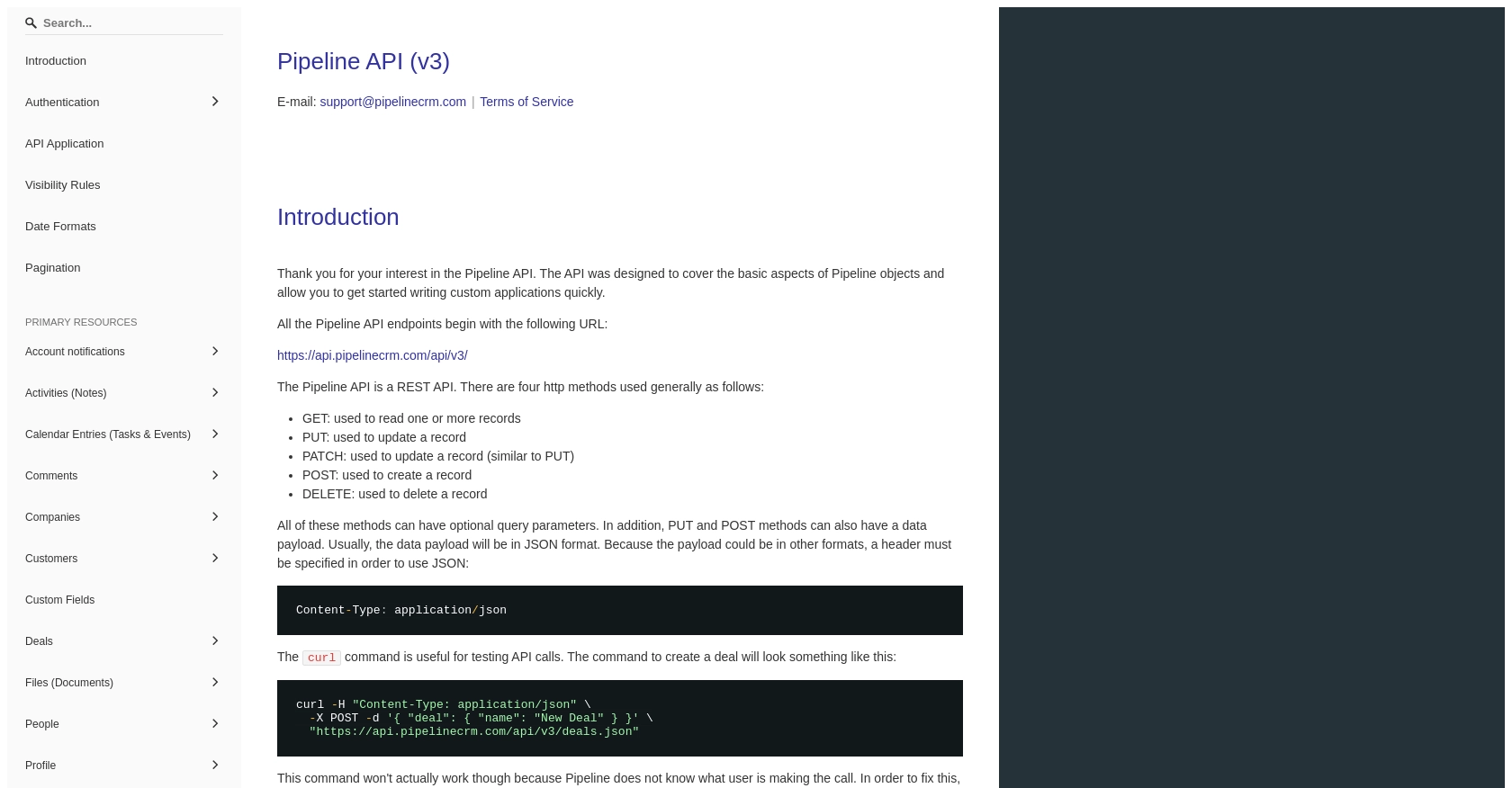
sbb-itb-96038d7
Making API Calls to Retrieve Users from PipelineCRM Using PHP
To interact with the PipelineCRM API and retrieve user data, you need to set up your PHP environment and make HTTP requests to the appropriate endpoints. This section will guide you through the process of making API calls using PHP, ensuring you can efficiently access user information from PipelineCRM.
Setting Up Your PHP Environment for PipelineCRM API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer (PHP dependency manager)
Once you have these installed, use Composer to install the Guzzle HTTP client, which simplifies making HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Users from PipelineCRM
Create a new PHP file named get_pipelinecrm_users.php
and add the following code to it:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key'; // Replace with your actual API key
try {
$response = $client->request('GET', 'https://api.pipelinecrm.com/api/v3/users', [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Accept' => 'application/json',
],
]);
$data = json_decode($response->getBody(), true);
foreach ($data['users'] as $user) {
echo 'User ID: ' . $user['id'] . ', Name: ' . $user['name'] . '<br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you generated earlier. This code uses Guzzle to send a GET request to the PipelineCRM API endpoint for users. It then decodes the JSON response and prints out the user IDs and names.
Running Your PHP Script to Fetch Users from PipelineCRM
Execute the script from the command line using the following command:
php get_pipelinecrm_users.php
If successful, you should see a list of user IDs and names printed to the console. This confirms that your API call to PipelineCRM was successful.
Handling Errors and Verifying API Call Success
To ensure your API calls are successful, check the HTTP status code returned by the API. A status code of 200 indicates success, while other codes may indicate errors. The example code above includes basic error handling to catch exceptions and display error messages.
For more detailed error information, refer to the PipelineCRM API documentation.
Conclusion and Best Practices for PipelineCRM API Integration
Integrating with the PipelineCRM API using PHP allows developers to efficiently manage and synchronize user data across various platforms. By following the steps outlined in this guide, you can retrieve user information and ensure your sales teams have access to the most current data.
Best Practices for Secure and Efficient API Usage
- Secure API Key Storage: Always store your API keys securely and avoid exposing them in public repositories or client-side code. Consider using environment variables or secure vaults for storage.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the API to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from the API is transformed and standardized to match your internal data structures for seamless integration.
- Error Handling: Implement robust error handling to manage different HTTP status codes and API errors. This will help maintain the stability of your application.
Streamlining Integrations with Endgrate
While integrating with individual APIs like PipelineCRM can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including PipelineCRM.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a seamless integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?