Using the Freshsales API to Create or Update Accounts in Javascript
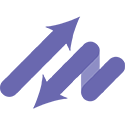
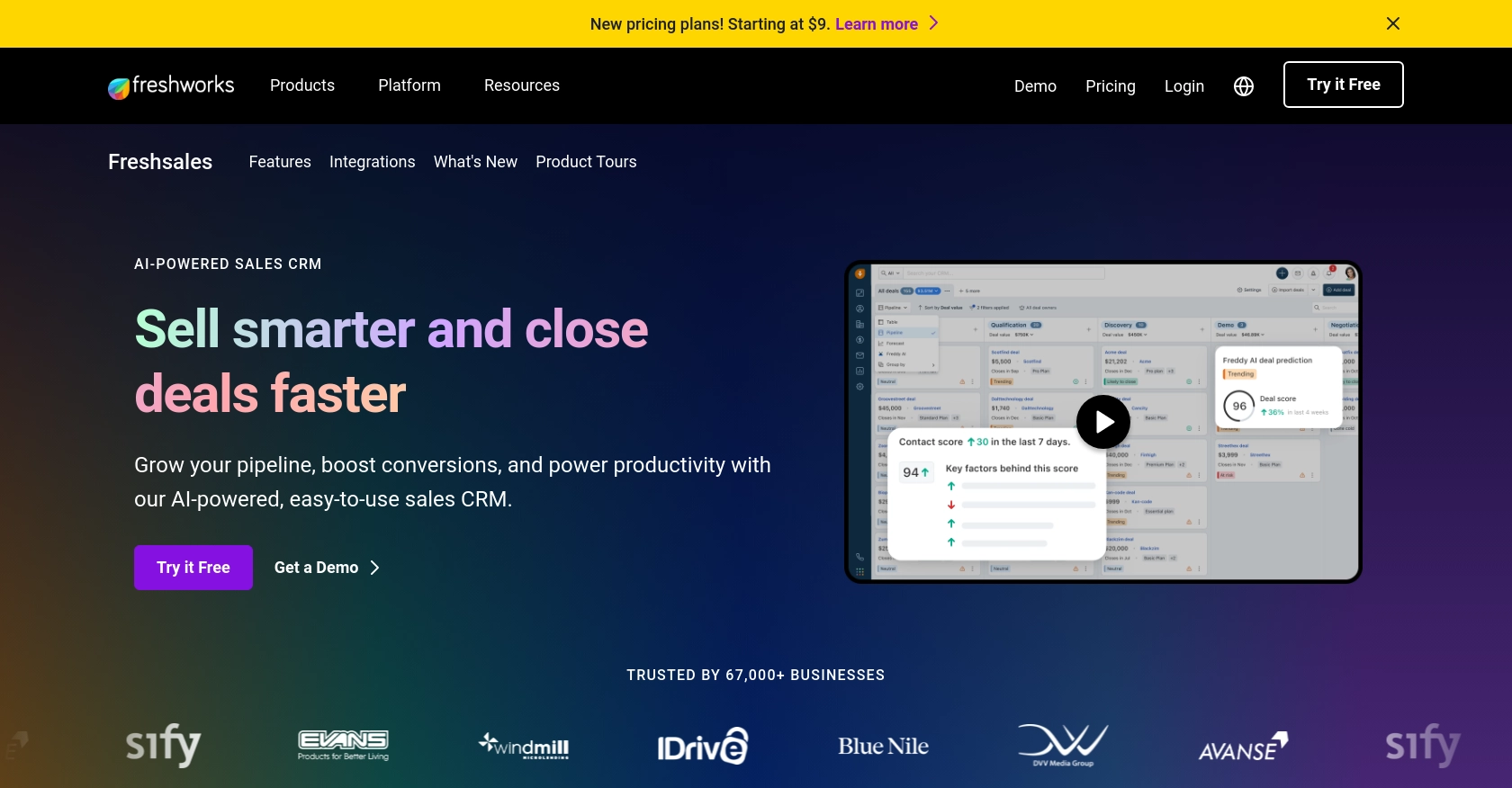
Introduction to Freshsales API
Freshsales is a powerful customer relationship management (CRM) platform designed to help businesses manage their sales processes efficiently. With features like lead scoring, email tracking, and built-in phone capabilities, Freshsales provides a comprehensive solution for sales teams to enhance their productivity and close deals faster.
Integrating with the Freshsales API allows developers to automate and streamline various sales operations, such as creating or updating account information. For example, a developer might use the Freshsales API to automatically update account details when a customer makes a purchase, ensuring that the sales team always has the most current information.
Setting Up a Freshsales Test or Sandbox Account for API Integration
Before diving into the Freshsales API, it's crucial to set up a test or sandbox account. This environment allows developers to experiment with API calls without affecting live data, ensuring a safe and controlled testing process.
Creating a Freshsales Account
If you don't already have a Freshsales account, you can start by signing up for a free trial on the Freshsales website. This trial provides access to all features, allowing you to explore the platform's capabilities fully.
- Visit the Freshsales website and click on the "Free Trial" button.
- Fill out the registration form with your details and submit it.
- Once registered, you'll receive an email with instructions to activate your account.
Generating an API Key for Freshsales
Freshsales uses a custom authentication method involving an API key. Follow these steps to generate your API key:
- Log in to your Freshsales account.
- Navigate to the "Settings" section in the top-right corner of the dashboard.
- Under "API Settings," locate the option to generate an API key.
- Click "Generate" and copy the API key. Store it securely, as you'll need it for authentication in your API requests.
Configuring OAuth for Freshsales API Access
For more advanced integrations, you might need to set up OAuth. Here's a brief overview of the process:
- In the "Settings" section, navigate to "API Settings" and select "OAuth Apps."
- Click "Create App" and fill in the required details, such as the app name and redirect URL.
- Once created, you'll receive a client ID and client secret. These credentials are essential for OAuth-based authentication.
For detailed instructions, refer to the official Freshsales API documentation at Freshsales API Documentation.
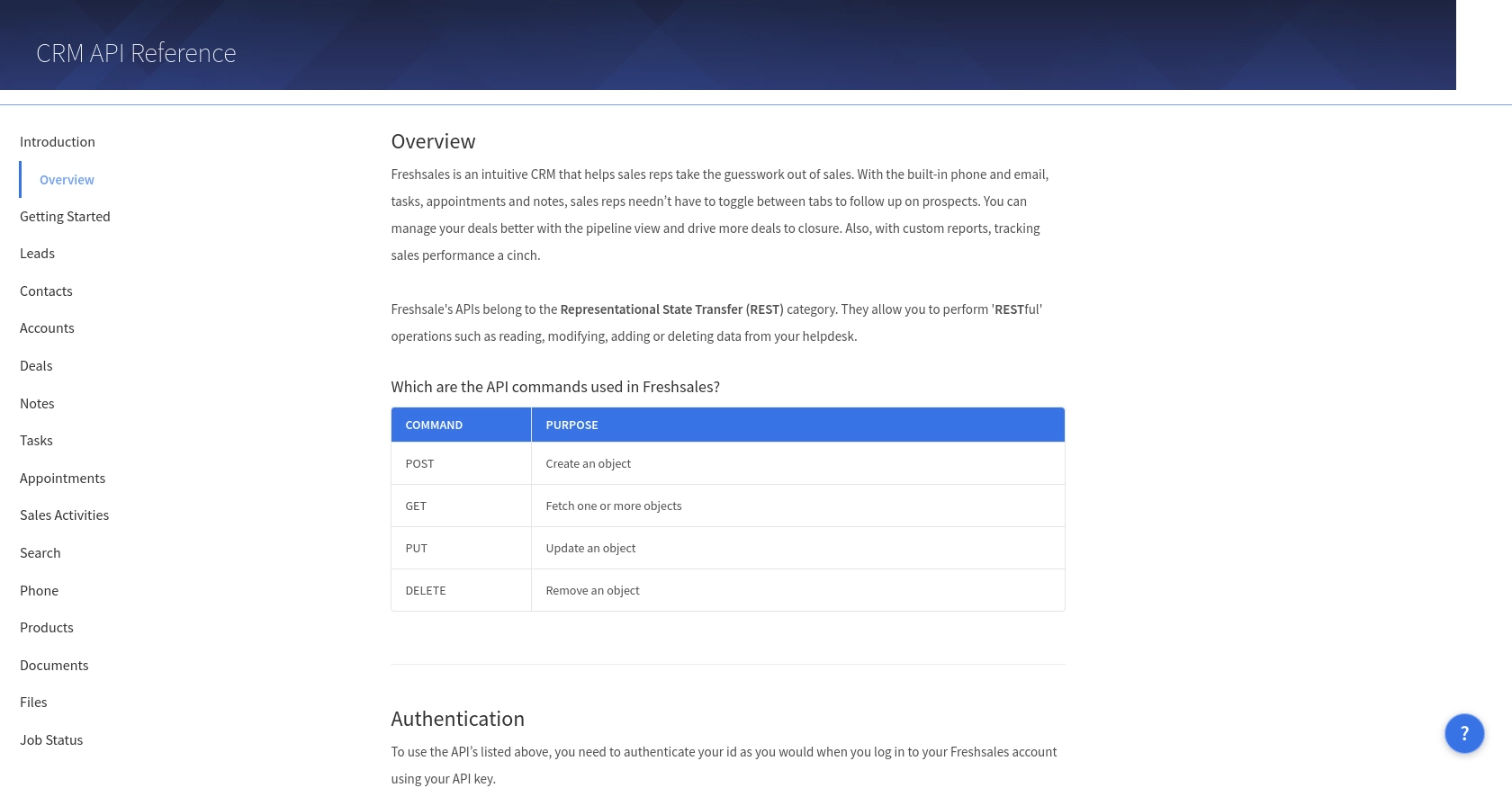
sbb-itb-96038d7
Making API Calls to Freshsales Using JavaScript
To interact with the Freshsales API using JavaScript, you'll need to set up your development environment and write code to create or update accounts. This section will guide you through the necessary steps, including setting up your JavaScript environment, writing the API call, and handling responses.
Setting Up Your JavaScript Environment for Freshsales API
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js for server-side scripting or a browser-based environment for client-side scripting. For this tutorial, we'll use Node.js.
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library for making HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Create or Update Accounts in Freshsales
With your environment set up, you can now write the JavaScript code to interact with the Freshsales API. The following example demonstrates how to create or update an account using the API key for authentication.
const axios = require('axios');
// Set your Freshsales domain and API key
const domain = 'yourdomain.freshsales.io';
const apiKey = 'Your_API_Key';
// Function to create or update an account
async function createOrUpdateAccount(accountData) {
try {
const response = await axios({
method: 'post', // Use 'put' for updating an existing account
url: `https://${domain}/api/accounts`,
headers: {
'Authorization': `Token token=${apiKey}`,
'Content-Type': 'application/json'
},
data: accountData
});
console.log('Account created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating account:', error.response ? error.response.data : error.message);
}
}
// Example account data
const accountData = {
account: {
name: 'Example Company',
industry: 'Technology'
}
};
// Call the function
createOrUpdateAccount(accountData);
Replace Your_API_Key
with the API key you generated earlier. The accountData
object contains the account details you wish to create or update. You can modify this object to include additional fields as needed.
Verifying API Request Success and Handling Errors
After executing the API call, it's essential to verify the success of the request and handle any potential errors. The code above logs the response data upon a successful request and logs an error message if the request fails.
To ensure the account was created or updated, you can check the Freshsales dashboard for the new or modified account entry. Additionally, handle errors gracefully by checking the error response and providing informative messages to users or developers.
For more information on error codes and handling, refer to the Freshsales API Documentation.
Best Practices for Using Freshsales API in JavaScript
When working with the Freshsales API, it's essential to follow best practices to ensure efficient and secure integration. Here are some key recommendations:
- Securely Store API Credentials: Always store your API key and OAuth credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Freshsales API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limits effectively.
- Standardize Data Fields: Ensure that data fields are standardized across your application to maintain consistency and avoid errors during data processing.
- Error Handling: Implement robust error handling to manage API errors gracefully. Log errors for debugging and provide informative messages to users.
Enhance Your Integration Experience with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Freshsales. With Endgrate, you can:
- Save Time and Resources: Focus on your core product by outsourcing integrations to Endgrate, reducing development time and costs.
- Build Once, Use Everywhere: Create a single integration for each use case and leverage it across multiple platforms without additional effort.
- Offer a Seamless Experience: Provide your customers with an intuitive and efficient integration experience, enhancing user satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate today.
Read More
Ready to get started?