How to Create or Update Companies with the Zoho CRM API in Python
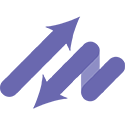
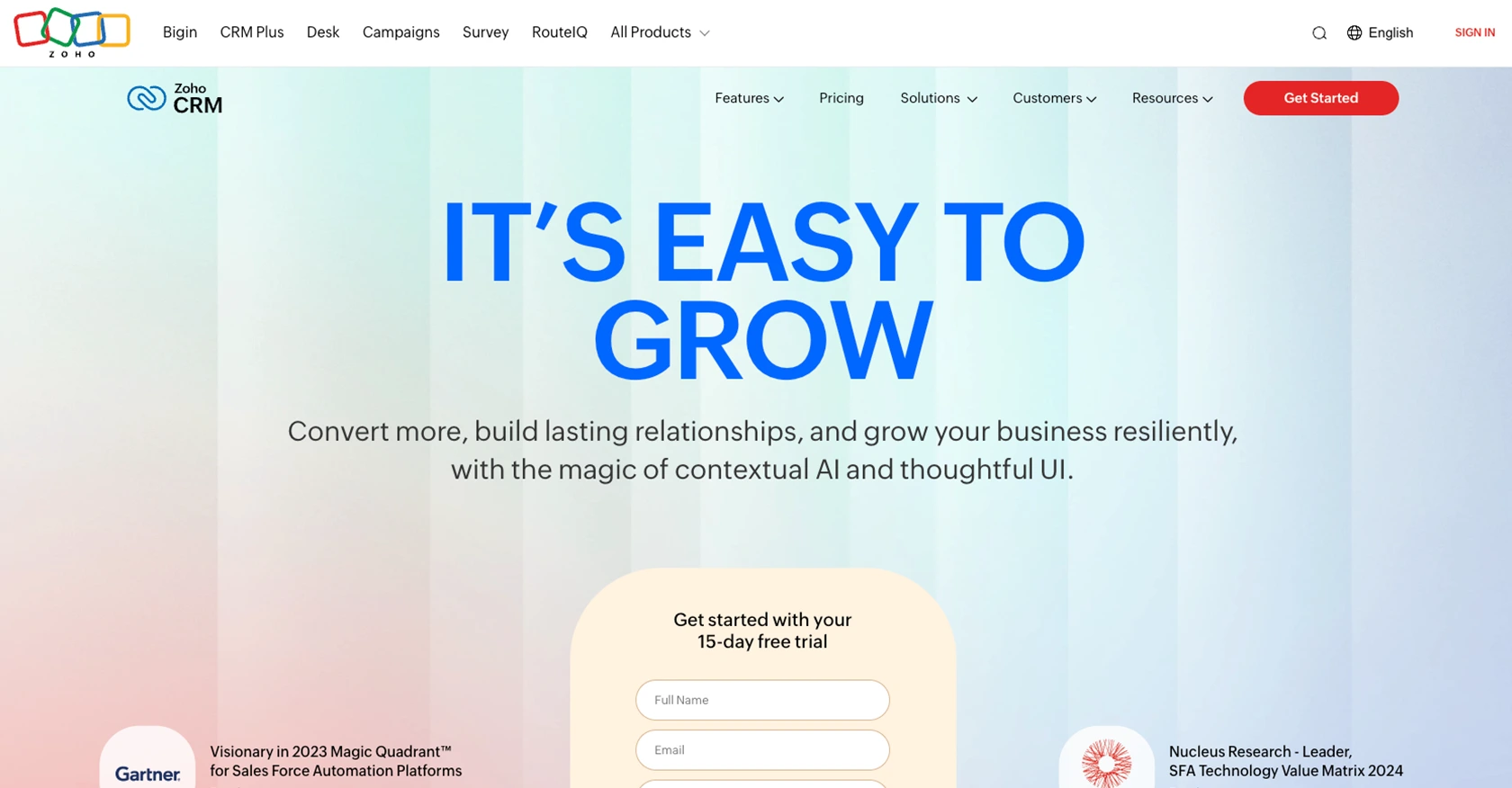
Introduction to Zoho CRM API Integration
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a unified manner. Known for its flexibility and extensive feature set, Zoho CRM is a popular choice for organizations looking to enhance their customer engagement and streamline operations.
Integrating with the Zoho CRM API allows developers to automate and optimize various business processes. For example, you can create or update company records directly from your application, ensuring that your CRM data is always up-to-date and accurate. This integration is particularly useful for businesses that need to synchronize data between Zoho CRM and other platforms, enhancing efficiency and reducing manual data entry.
Setting Up Your Zoho CRM Sandbox Account for API Integration
Before you can start integrating with the Zoho CRM API, you need to set up a sandbox account. This allows you to test your API calls without affecting your live data. Zoho CRM offers a developer sandbox environment that mimics your production environment, providing a safe space for testing and development.
Steps to Create a Zoho CRM Sandbox Account
- Sign Up for Zoho CRM: If you don't have a Zoho CRM account, visit the Zoho CRM website and sign up for a free trial or a developer account.
- Access the Developer Console: Once logged in, navigate to the Developer Console from the Zoho CRM dashboard. This is where you can manage your API integrations and sandbox settings.
- Create a Sandbox: In the Developer Console, select the option to create a new sandbox. Follow the prompts to configure your sandbox environment, ensuring it mirrors your production setup.
Configuring OAuth Authentication for Zoho CRM API
Zoho CRM uses OAuth 2.0 for secure API authentication. Follow these steps to set up OAuth for your application:
- Register Your Application: In the Developer Console, register your application by providing necessary details such as the application name, homepage URL, and authorized redirect URIs. This will generate a Client ID and Client Secret for your app.
-
Set Scopes: Define the scopes for your application to specify the level of access it requires. For creating or updating companies, you might need scopes like
ZohoCRM.modules.accounts.CREATE
andZohoCRM.modules.accounts.UPDATE
. - Generate Access Tokens: Use the Client ID and Client Secret to generate access tokens. This involves making an authorization request and exchanging the authorization code for access and refresh tokens. Refer to the Zoho CRM OAuth documentation for detailed steps.
Obtaining API Credentials
After setting up OAuth, you will have the following credentials:
- Client ID: The unique identifier for your application.
- Client Secret: A secret key used to authenticate your application.
- Access Token: A token used to access the Zoho CRM API.
- Refresh Token: A token used to obtain new access tokens when the current one expires.
Ensure you store these credentials securely and do not expose them in public repositories or client-side code.
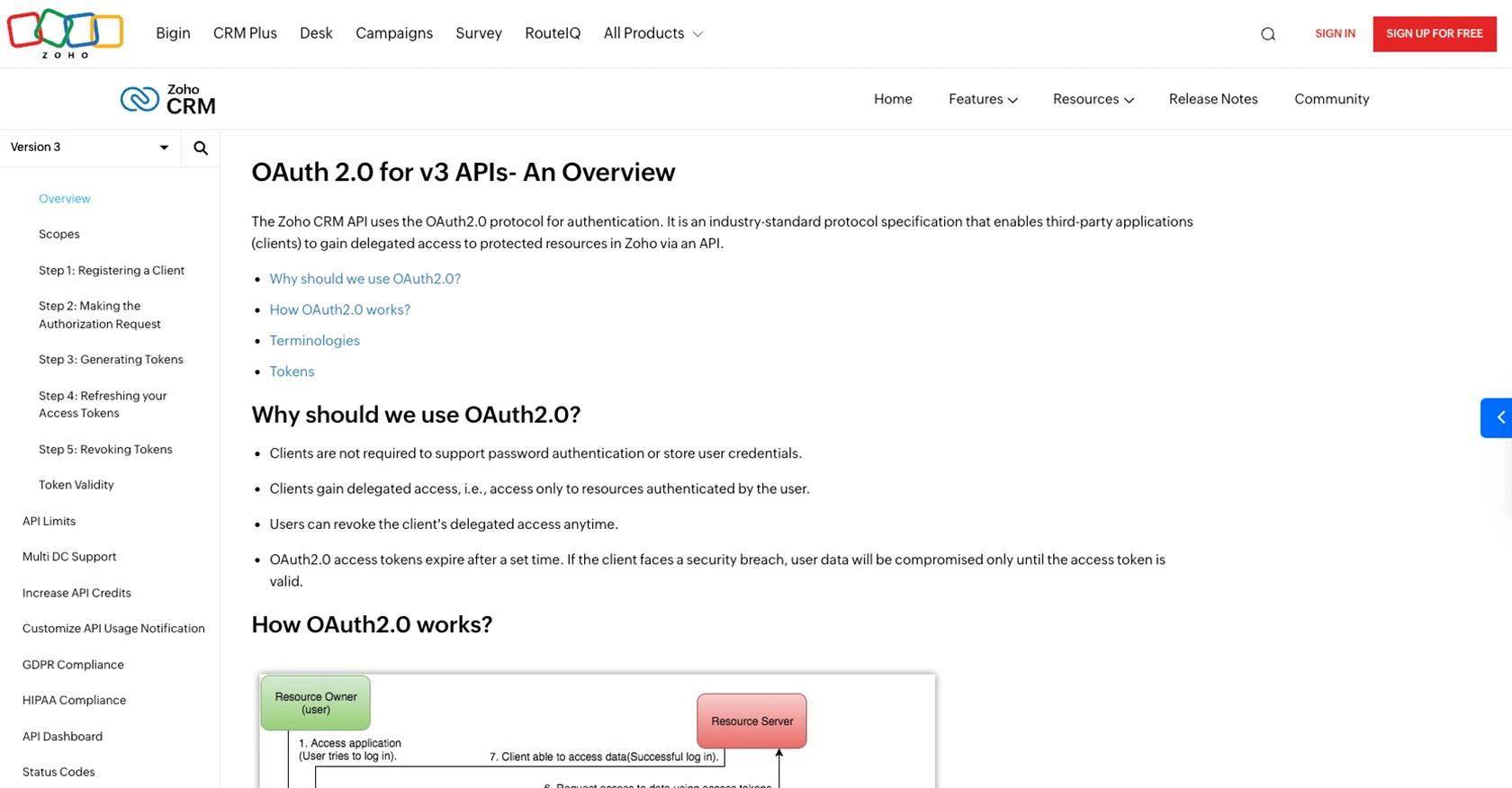
sbb-itb-96038d7
How to Make API Calls to Create or Update Companies in Zoho CRM Using Python
To interact with the Zoho CRM API for creating or updating company records, you'll need to use Python. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for Zoho CRM API Integration
Before making API calls, ensure you have Python 3.11.1 installed on your machine. Additionally, you'll need the requests
library to handle HTTP requests.
pip install requests
Creating or Updating Companies with Zoho CRM API in Python
Below is a sample Python script to create or update a company record in Zoho CRM. Replace Your_Access_Token
with your actual access token obtained from the OAuth setup.
import requests
# Define the API endpoint
url = "https://www.zohoapis.com/crm/v3/Accounts"
# Set the request headers
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token",
"Content-Type": "application/json"
}
# Define the company data
company_data = {
"data": [
{
"Account_Name": "New Company",
"Website": "https://newcompany.com",
"Industry": "Technology"
}
]
}
# Make the API request
response = requests.post(url, json=company_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Company created successfully.")
elif response.status_code == 200:
print("Company updated successfully.")
else:
print("Failed to create or update company:", response.json())
Verifying API Call Success in Zoho CRM Sandbox
After running the script, verify the success of your API call by checking the Zoho CRM sandbox. If a new company was created, it should appear in the Accounts module. If an existing company was updated, the changes should reflect accordingly.
Handling Errors and Zoho CRM API Error Codes
When making API calls, it's crucial to handle potential errors. Zoho CRM provides detailed error codes that can help diagnose issues. For example, a 400
status code indicates a bad request, while a 401
status code signifies unauthorized access. Refer to the Zoho CRM API Status Codes documentation for more details.
By following these steps, you can efficiently create or update company records in Zoho CRM using Python, ensuring your CRM data remains accurate and up-to-date.
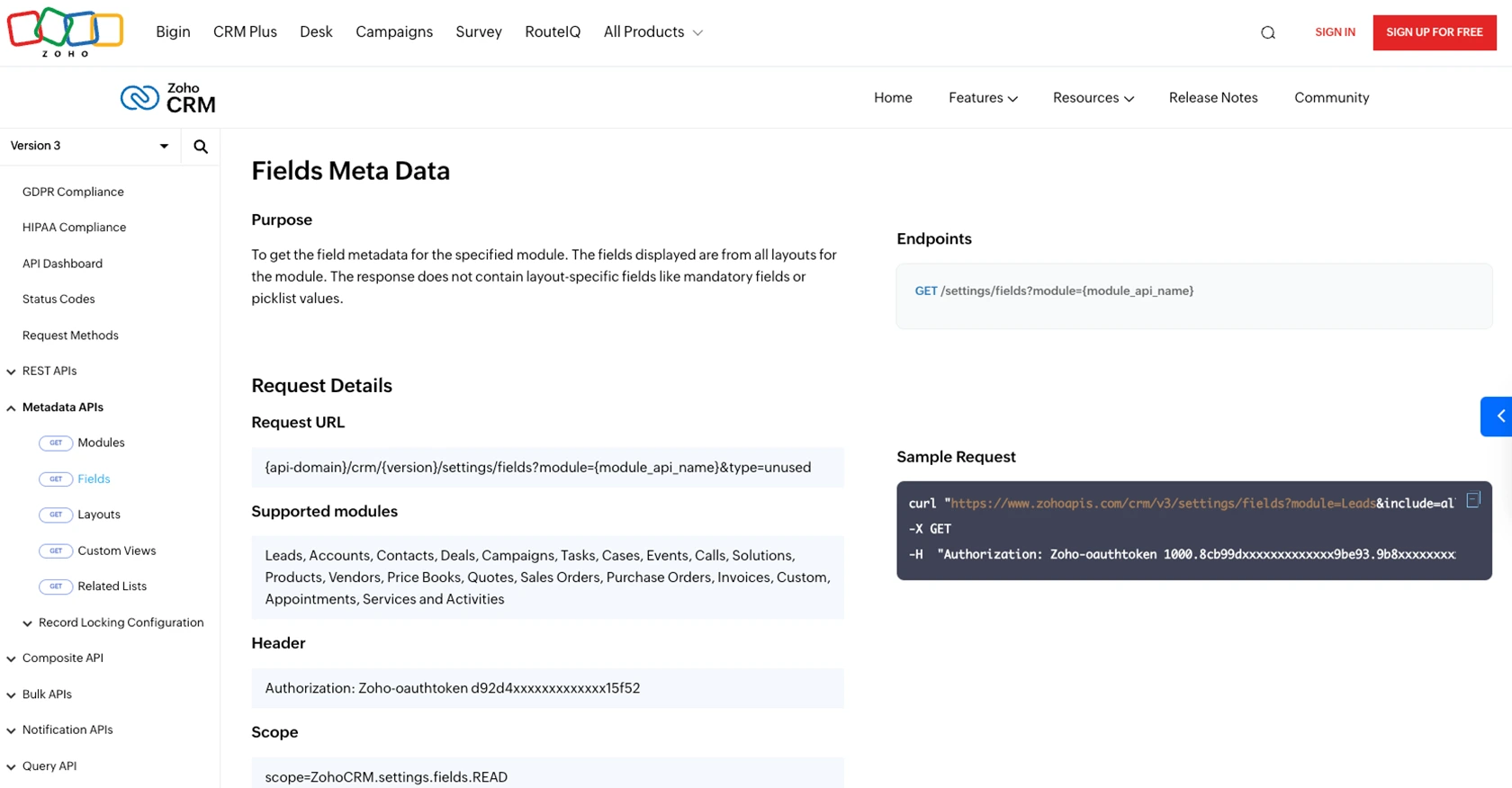
Best Practices for Zoho CRM API Integration
When integrating with the Zoho CRM API, it's essential to follow best practices to ensure security, efficiency, and reliability. Here are some recommendations:
- Securely Store Credentials: Always store your Client ID, Client Secret, and tokens securely. Avoid exposing them in public repositories or client-side code.
- Handle Rate Limiting: Zoho CRM imposes rate limits on API requests. Be sure to implement logic to handle rate limiting gracefully, such as retrying requests after a delay. Refer to the API Limits documentation for more details.
- Standardize Data Fields: Ensure that data fields are standardized and consistent across your applications to avoid discrepancies and data integrity issues.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
Streamlining Zoho CRM Integrations with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?