Using the Zoho Recruit API to Create or Update Candidates in Javascript
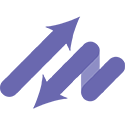
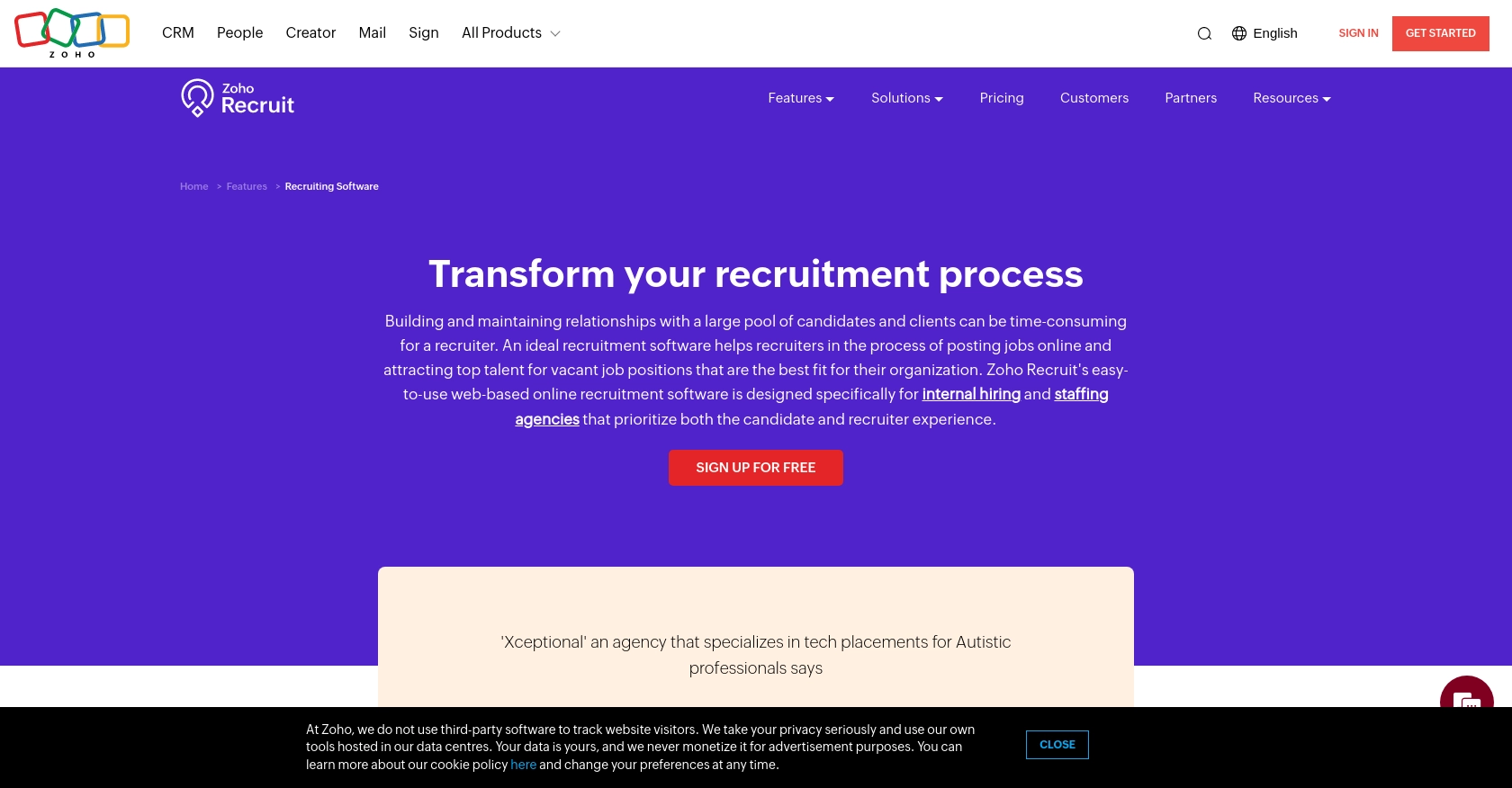
Introduction to Zoho Recruit API
Zoho Recruit is a comprehensive recruitment software solution designed to streamline the hiring process for staffing agencies and corporate HRs. It offers a range of features including candidate sourcing, resume management, and interview scheduling, making it a popular choice for businesses looking to enhance their recruitment efforts.
Integrating with the Zoho Recruit API allows developers to automate and optimize recruitment workflows. For example, you can use the API to create or update candidate records directly from your application, ensuring that your recruitment database is always up-to-date and accurate.
This article will guide you through using JavaScript to interact with the Zoho Recruit API, specifically focusing on creating or updating candidate records. By following this tutorial, developers can efficiently manage candidate data within Zoho Recruit, enhancing the overall recruitment process.
Setting Up Your Zoho Recruit Test Account
Before you can start integrating with the Zoho Recruit API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Zoho Recruit offers a free trial that you can use for this purpose.
Creating a Zoho Recruit Account
- Visit the Zoho Recruit website and sign up for a free trial account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is set up, log in to access the Zoho Recruit dashboard.
Registering Your Application for OAuth Authentication
Zoho Recruit uses OAuth 2.0 for authentication, which is a secure method to authorize API requests. Follow these steps to register your application:
- Go to the Zoho Developer Console.
- Select the appropriate client type for your application. For JavaScript applications, choose "Java Script".
- Enter the required details:
- Client Name: The name of your application.
- Homepage URL: The URL of your application's homepage.
- Authorized Redirect URIs: A valid URL where Zoho will redirect after successful authentication.
- Click "CREATE" to register your application.
- Note down the generated Client ID and Client Secret, as you will need these for authentication.
For more details, refer to the Zoho Recruit OAuth Overview.
Generating Access and Refresh Tokens
Once your application is registered, you need to generate access and refresh tokens to authenticate API requests:
- Make an authorization request to Zoho's OAuth server using your Client ID and Redirect URI.
- Upon successful authentication, you'll receive an authorization code.
- Exchange this authorization code for access and refresh tokens by making a POST request to Zoho's token endpoint.
For detailed steps, refer to the Zoho Recruit Client Registration Guide.
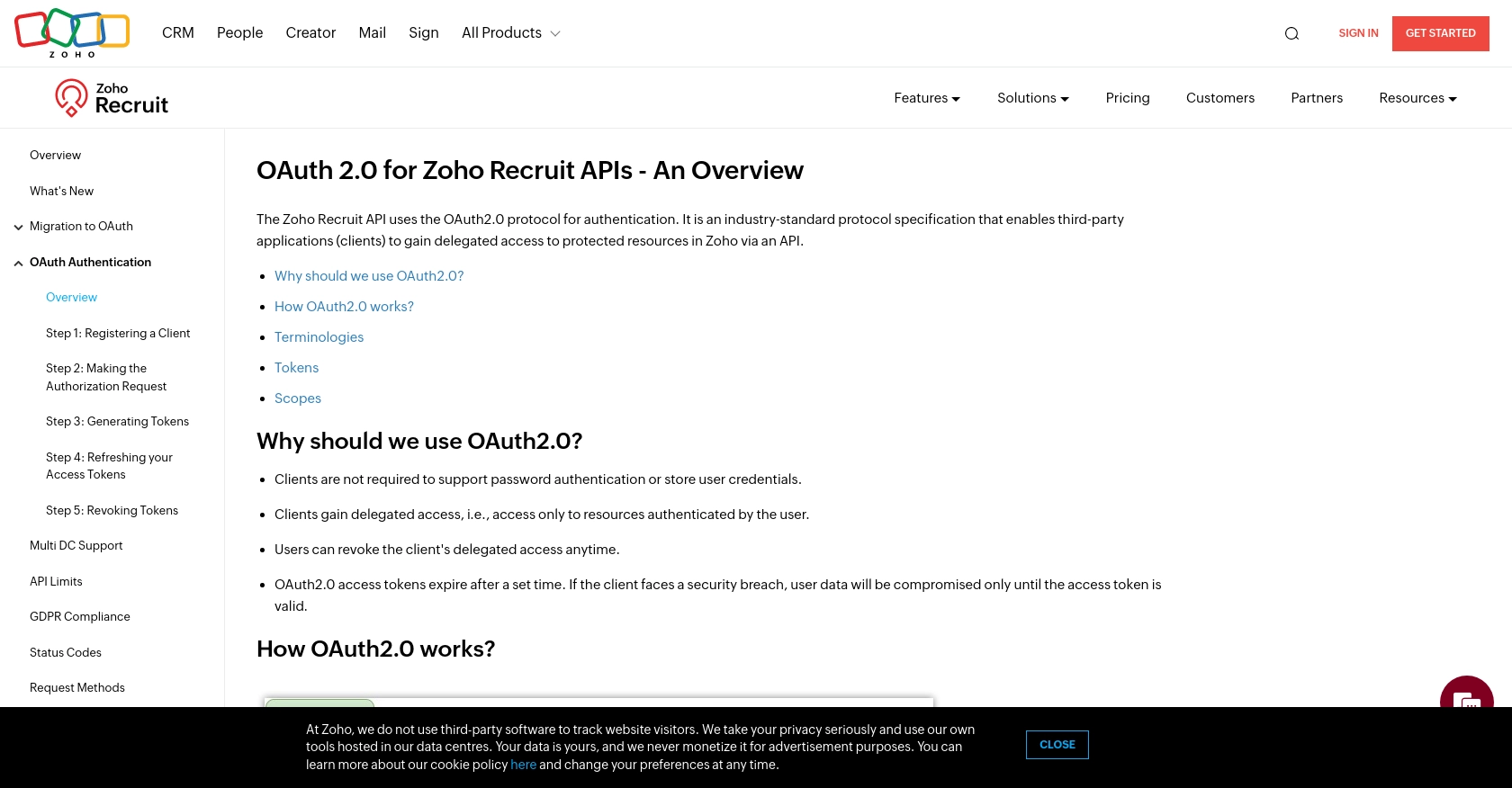
sbb-itb-96038d7
Making API Calls to Zoho Recruit Using JavaScript
To interact with the Zoho Recruit API using JavaScript, you'll need to set up your environment and write code to create or update candidate records. This section will guide you through the process, including setting up your JavaScript environment, writing the necessary code, and handling responses and errors.
Setting Up Your JavaScript Environment for Zoho Recruit API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- Access to the Zoho Recruit API credentials (Client ID, Client Secret, Access Token).
Install the Axios library for making HTTP requests by running the following command in your terminal:
npm install axios
Writing JavaScript Code to Create or Update Candidates in Zoho Recruit
Now, let's write the JavaScript code to create or update candidate records using the Zoho Recruit API. We'll use the Axios library to handle HTTP requests.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://recruit.zoho.com/recruit/v2/Candidates/upsert';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Define the candidate data
const candidateData = {
data: [
{
First_Name: 'John',
Last_Name: 'Doe',
Email: 'john.doe@example.com',
Phone: '1234567890'
}
],
duplicate_check_fields: ['Email']
};
// Make a POST request to the API
axios.post(endpoint, candidateData, { headers })
.then(response => {
console.log('Candidate created/updated successfully:', response.data);
})
.catch(error => {
console.error('Error creating/updating candidate:', error.response.data);
});
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the authentication process. The code above sends a POST request to the Zoho Recruit API to create or update a candidate record. It checks for duplicates using the email field.
Verifying API Call Success in Zoho Recruit
After running the code, verify that the candidate record was created or updated successfully by checking your Zoho Recruit dashboard. Navigate to the Candidates module to see the changes reflected.
Handling Errors and Understanding Zoho Recruit API Status Codes
It's crucial to handle errors gracefully when making API calls. The Zoho Recruit API provides various status codes to indicate the result of your requests:
- 200 OK: The request was successful.
- 201 Created: The candidate was successfully created.
- 400 Bad Request: The request was invalid. Check your input data.
- 401 Authorization Error: Invalid API key or token.
- 429 Too Many Requests: Rate limit exceeded. Try again later.
For more detailed information on status codes, refer to the Zoho Recruit Status Codes Documentation.
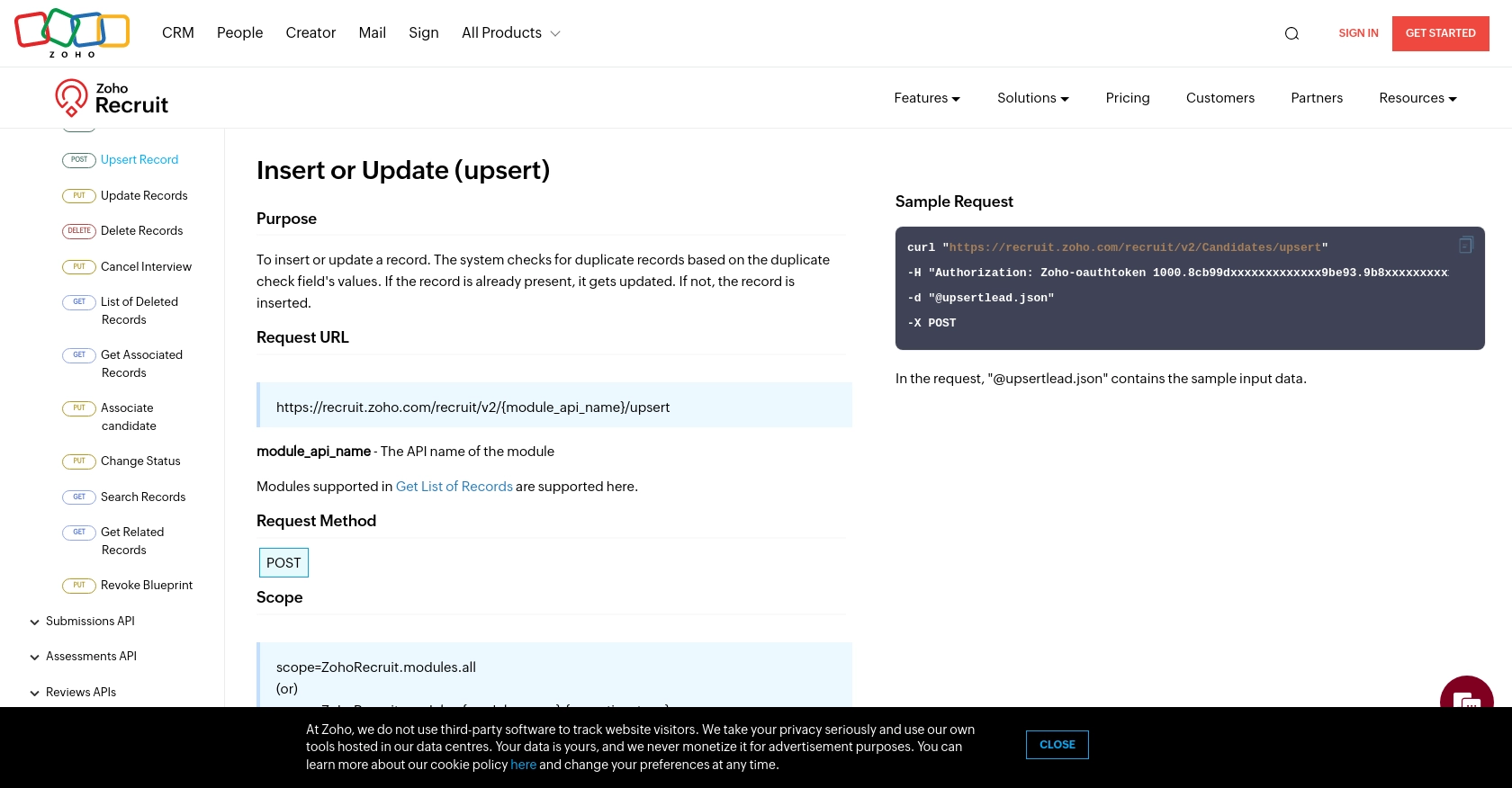
Conclusion and Best Practices for Using Zoho Recruit API in JavaScript
Integrating with the Zoho Recruit API using JavaScript allows developers to efficiently manage candidate data, streamlining recruitment processes and ensuring data accuracy. By following the steps outlined in this guide, you can create or update candidate records seamlessly, enhancing your recruitment workflows.
Best Practices for Zoho Recruit API Integration
- Securely Store Credentials: Always keep your Client ID, Client Secret, and Access Tokens secure. Avoid exposing them in public repositories or client-side code.
- Handle Rate Limits: Be mindful of Zoho Recruit's rate limits to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to handle
429 Too Many Requests
errors gracefully. For more details, refer to the Zoho Recruit API Limits Documentation. - Data Standardization: Ensure that data fields are standardized before making API calls. This helps maintain consistency and accuracy in your recruitment database.
- Error Handling: Implement robust error handling to manage different API response codes effectively. This ensures that your application can gracefully handle unexpected scenarios.
Enhancing Integration with Endgrate
For developers looking to simplify and scale their integration efforts, consider using Endgrate. With Endgrate, you can manage multiple integrations through a single API endpoint, saving time and resources. Focus on your core product while Endgrate handles the complexities of integration, providing an intuitive experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate and discover the benefits of a unified API solution.
Read More
- https://endgrate.com/provider/zohorecruit
- https://www.zoho.com/recruit/developer-guide/apiv2/oauth-overview.html
- https://www.zoho.com/recruit/developer-guide/apiv2/register-client.html
- https://www.zoho.com/recruit/developer-guide/apiv2/limits.html
- https://www.zoho.com/recruit/developer-guide/apiv2/status-codes.html
- https://www.zoho.com/recruit/developer-guide/apiv2/upsert-records.html
Ready to get started?